


When would you use a trait versus an abstract class or interface in PHP?
In PHP, trait is suitable for situations where method reuse is required but not suitable for inheritance. 1) trait allows multiplexing methods in classes to avoid multiple inheritance complexity. 2) When using trait, you need to pay attention to method conflicts, which can be resolved through the insteadof and as keywords. 3) Overuse of trait should be avoided and its single responsibility should be maintained to optimize performance and improve code maintainability.
introduction
In PHP programming, choosing to use trait, abstract classes or interfaces is often a headache. Today we will talk about under what circumstances should trait be used and how it differs from abstract classes and interfaces. Through this article, you will learn how to make the best choices in real projects, avoid common misunderstandings, and master some small tips for performance optimization.
Review of basic knowledge
In PHP, trait, abstract classes and interfaces are tools used to implement code reuse, but they each have different uses and limitations. trait can be regarded as a collection of code snippets, allowing developers to reuse methods without using inheritance. An abstract class can contain abstract methods and concrete methods, which are suitable for defining the basic structure of a class. The interface defines a set of methods signatures, and the forced implementation class must implement these methods.
Core concept or function analysis
The definition and function of Trait
Trait is a method to implement cross-cutting concerns in a single inheritance language. They allow developers to reuse methods in classes without implementing them through inheritance. Using trait can avoid the complexity of multiple inheritance while keeping the code concise and maintainability.
trait Logger { public function log($message) { echo "Log: $message\n"; } } class UserService { use Logger; public function doSomething() { $this->log("Doing something..."); } }
In this example, Logger
trait is used by UserService
class, allowing UserService
class to call log
method.
How Trait works
Trait works by copying trait's methods to the class that uses it at compile time. This means that methods in trait are actually part of the class, not called by reference. This mechanism makes trait very flexible, but also needs to pay attention to naming conflicts.
trait A { public function smallTalk() { echo "A: Let's talk about something small.\n"; } } trait B { public function smallTalk() { echo "B: Let's talk about something big.\n"; } } class Talker { use A, B { B::smallTalk instead A; } }
In this example, Talker
class uses two traits A
and B
, and resolves method conflicts through the insteadof
keyword.
Example of usage
Basic usage
The most common usage of Trait is to extract some common methods for use by multiple classes. For example, logging, verification and other functions can be implemented through trait.
trait Validator { public function validateEmail($email) { return filter_var($email, FILTER_VALIDATE_EMAIL); } } class User { use Validator; public function register($email) { if ($this->validateEmail($email)) { echo "Email is valid.\n"; } else { echo "Email is invalid.\n"; } } }
In this example, Validator
trait provides a validateEmail
method, which User
class uses through use
keyword.
Advanced Usage
Trait can also be used to implement more complex functions, such as combining multiple traits to build a fully functional class.
trait Logger { public function log($message) { echo "Log: $message\n"; } } trait Validator { public function validateEmail($email) { return filter_var($email, FILTER_VALIDATE_EMAIL); } } class UserService { use Logger, Validator; public function registerUser($email) { if ($this->validateEmail($email)) { $this->log("User registered with email: $email"); return true; } $this->log("Invalid email: $email"); return false; } }
In this example, UserService
class combines two traits Logger
and Validator
to implement the user registration function.
Common Errors and Debugging Tips
The most common error when using trait is method conflict. These conflicts can be resolved through insteadof
and as
keywords.
trait A { public function smallTalk() { echo "A: Let's talk about something small.\n"; } } trait B { public function smallTalk() { echo "B: Let's talk about something big.\n"; } } class Talker { use A, B { B::smallTalk instead A; A::smallTalk as protected smallTalkA; } public function talk() { $this->smallTalk(); // Calls B::smallTalk $this->smallTalkA(); // Calls A::smallTalk } }
In this example, we resolved method conflicts through insteadof
and renamed the smallTalk
method in A
through as
.
Performance optimization and best practices
When using trait, the following points need to be paid attention to to optimize performance and maintain best practices:
- Avoid overuse of trait : While trait can improve code reusability, overuse can make the code difficult to understand and maintain. Try to use trait for features that really need to be reused.
- Note naming conflicts : When using multiple traits, make sure that the method names do not conflict. If conflicts are conflicted, use
insteadof
andas
keywords in time to resolve them. - Keeping a single responsibility of trait : Each trait should be responsible for only one function, which can improve the readability and maintainability of the code.
In terms of performance, the use of trait will not directly affect the execution efficiency of the code, because trait methods are copied into the class at compile time. However, when designing, you should consider whether the use of trait will increase the complexity of the class, thereby affecting the maintainability of the code.
Overall, trait is a powerful tool that enables code reuse without using multiple inheritance. By using trait rationally, the readability and maintainability of the code can be improved while avoiding common misunderstandings and performance problems.
The above is the detailed content of When would you use a trait versus an abstract class or interface in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


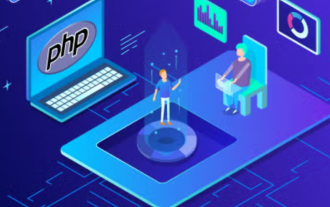
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
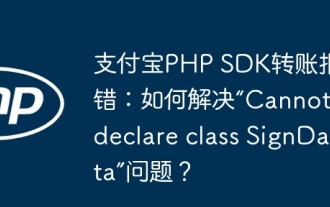
Alipay PHP...
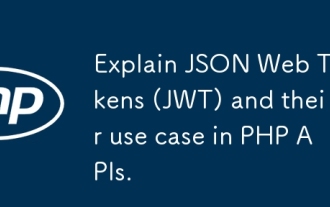
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
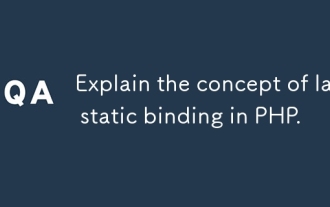
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
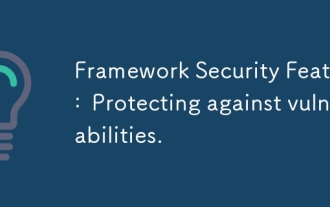
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
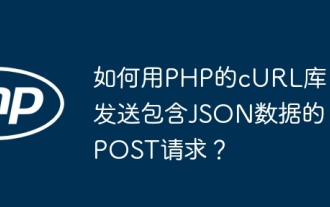
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
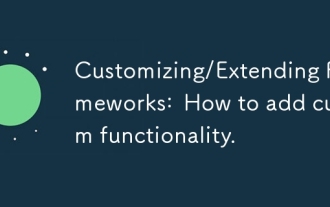
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
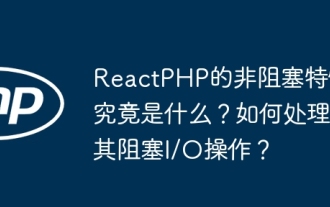
An official introduction to the non-blocking feature of ReactPHP in-depth interpretation of ReactPHP's non-blocking feature has aroused many developers' questions: "ReactPHPisnon-blockingbydefault...
