How does Redis cluster handle memory issues?
Redis memory problems stem from the amount of data exceeding available memory. Solutions include: expanding the memory capacity of Redis instances, using Redis clusters, scattering data across multiple instances to optimize data, deleting unnecessary storage or using more compact data structures to use memory phasing strategies, and controlling memory usage, such as LRU or LFU
Redis cluster handles memory issues? This is a good question, which is directly related to the stability and performance of the system. Many developers think that Redis memory is simple, and it will explode after using it. In fact, this is not the case. Only by understanding Redis's memory management mechanism can we deal with it calmly.
Let’s talk about the conclusion first: Redis’s memory problem is essentially that the amount of data exceeds the available memory. The solution, ultimately, is to control the amount of data or increase memory. But there are many specific operating methods, each with its advantages and disadvantages, so we have to talk about it carefully.
Redis itself is a memory database, which stores all data in memory. This brings extremely high read and write speeds, but at the cost of limited memory. When the amount of data exceeds the memory capacity, various problems will occur, with performance degradation at the least and downtime at the worst.
Let’s start with Redis’ memory mechanism. Redis mainly uses jemalloc for memory allocation, which is more efficient than the system's malloc and is more suitable for high-throughput applications such as Redis. But no matter how good jemalloc is, it cannot create memory out of thin air. Redis's memory usage depends largely on the persistence policy (RDB or AOF) you choose and the data type. RDB will periodically snap the data, occupying extra memory, while AOF will record each command, which will consume more memory, but the data will be safer. Which strategy to choose needs to be weighed based on your business needs and fault tolerance requirements.
For example, if you use Redis to store a large number of strings, the memory consumption will be much greater than the small number of hash tables. The memory usage of different data structures varies greatly, which requires you to have a deep understanding of Redis's data structure in order to choose the most appropriate type to optimize memory usage.
Next, let’s take a look at the actual operation.
The most direct way is of course expansion. Increase the memory of Redis instances, which is simple and crude, but it is expensive. Moreover, stand-alone memory is always limited, and when the amount of data continues to grow, the same problem will still be faced.
A more elegant approach is to adopt clusters. Spread the data across multiple Redis instances, reducing the memory pressure on a single instance. This requires you to carefully plan the sharding strategy to avoid data skew. A good sharding strategy can ensure that data is evenly distributed on each node and maximize the use of cluster resources. But cluster management itself also increases complexity, and you need to consider node failover, data synchronization and other issues.
Another method is to optimize data. This requires you to analyze your business data in depth to see if you can reduce unnecessary storage. For example, you can clean out expiration data regularly, or use a more compact data structure. This requires you to have a good understanding of your business in order to be targeted.
To be more advanced, you can consider using the memory ed out strategy. Redis provides a variety of memory elimination strategies, such as LRU, LFU, etc. Selecting the right strategy can effectively control memory usage. However, different strategies have different advantages and disadvantages, and you need to carefully weigh them when choosing. For example, the LRU (mostly used recently) strategy is simple and efficient, but it may accidentally delete important data; the LFU (mostly used recently) strategy is more accurate, but the implementation is more complex.
Finally, what I want to say is that there is no one-time solution to deal with Redis memory problems. You need to choose the appropriate strategy according to your specific situation, and continuously monitor memory usage and make timely adjustments. Don't wait until the problem breaks out before dealing with it, it will often cost you a greater price. This requires you to have a certain system operation and maintenance capabilities and a deep understanding of Redis. Remember, monitoring and early warning are the key! My code style is relatively casual and does not pursue fancy things, just be practical, such as a simple LRU cache implementation (for reference only, it is not recommended to be used directly in production environment):
<code class="python">class LRUCache: def __init__(self, capacity): self.capacity = capacity self.cache = {} self.queue = [] def get(self, key): if key in self.cache: self.queue.remove(key) self.queue.append(key) return self.cache[key] return -1 def put(self, key, value): if key in self.cache: self.queue.remove(key) elif len(self.queue) == self.capacity: del self.cache[self.queue.pop(0)] self.cache[key] = value self.queue.append(key) #Example cache = LRUCache(2) cache.put(1, 1) cache.put(2, 2) print(cache.get(1)) # returns 1 cache.put(3, 3) # evicts key 2 print(cache.get(2)) # returns -1 print(cache.get(3)) # returns 3</code>
This is just a simple example. In actual applications, you need to consider thread safety, concurrent control and other issues. In short, dealing with Redis memory problems is a system project that requires you to consider and learn from multiple aspects.
The above is the detailed content of How does Redis cluster handle memory issues?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


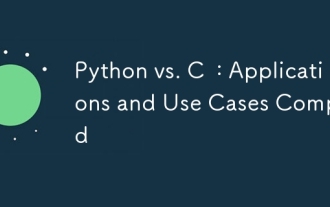
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
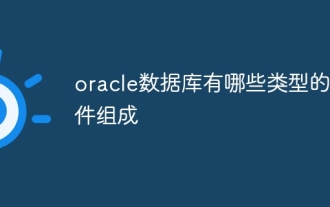
Oracle database file structure includes: data file: storing actual data. Control file: Record database structure information. Redo log files: record transaction operations to ensure data consistency. Parameter file: Contains database running parameters to optimize performance. Archive log file: Backup redo log file for disaster recovery.
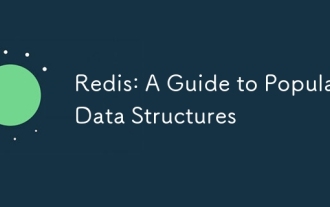
Redis supports a variety of data structures, including: 1. String, suitable for storing single-value data; 2. List, suitable for queues and stacks; 3. Set, used for storing non-duplicate data; 4. Ordered Set, suitable for ranking lists and priority queues; 5. Hash table, suitable for storing object or structured data.
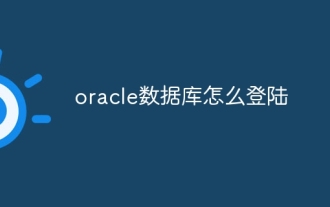
Oracle database login involves not only username and password, but also connection strings (including server information and credentials) and authentication methods. It supports SQL*Plus and programming language connectors and provides authentication options such as username and password, Kerberos and LDAP. Common errors include connection string errors and invalid username/passwords, while best practices focus on connection pooling, parameterized queries, indexing, and security credential handling.
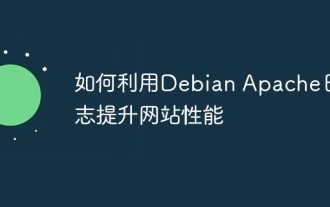
This article will explain how to improve website performance by analyzing Apache logs under the Debian system. 1. Log Analysis Basics Apache log records the detailed information of all HTTP requests, including IP address, timestamp, request URL, HTTP method and response code. In Debian systems, these logs are usually located in the /var/log/apache2/access.log and /var/log/apache2/error.log directories. Understanding the log structure is the first step in effective analysis. 2. Log analysis tool You can use a variety of tools to analyze Apache logs: Command line tools: grep, awk, sed and other command line tools.

The comparison between Laravel and Python in the development environment and ecosystem is as follows: 1. The development environment of Laravel is simple, only PHP and Composer are required. It provides a rich range of extension packages such as LaravelForge, but the extension package maintenance may not be timely. 2. The development environment of Python is also simple, only Python and pip are required. The ecosystem is huge and covers multiple fields, but version and dependency management may be complex.
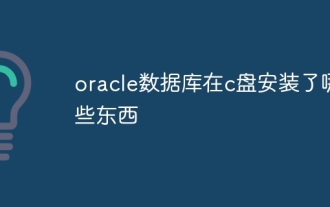
The hiding place of Oracle database on the C drive: Registry: Use the registry editor to search for "Oracle" to find information including installation path, service name, etc. File system: Oracle files are scattered in multiple locations in the C drive, including home directory, system files, temporary files, etc. Environment variables: The environment variables set by Oracle (such as ORACLE_HOME, ORACLE_SID) point to the installation directory and instance name. Careful action: When uninstalling Oracle, you not only need to delete files, but also clean the registry and services. It is recommended to use the official uninstall tool or seek professional help. Space management: Optimize disk space to avoid installing Oracle on C drive; clean temporary files regularly
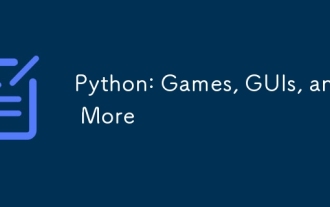
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
