The Continued Use of C : Reasons for Its Endurance
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
introduction
C, the name has been known for decades in the programming world. Why can it still stand firm under the impact of many emerging languages? This article will take you into the deeper discussion of the reasons for C's continuous use, from its powerful performance to a wide range of applications, to its ever-evolving features. After reading this article, you will have a deeper understanding of the lasting charm of C.
Review of basic knowledge
C, first released by Bjarne Stroustrup in 1983, is a statically typed, compiled general programming language. It was originally designed as an extension of C language, adding the features of object-oriented programming. The core advantage of C lies in its efficient performance and direct control of the underlying hardware, which makes it shine in the fields of system programming, game development, embedded systems, etc.
Core concept or function analysis
Performance and efficiency of C
The performance of C has always been one of its most eye-catching features. By directly manipulating memory and hardware resources, C can achieve extremely high execution efficiency. This is crucial for applications that require real-time processing and high-performance computing.
// Performance example: quick sorting algorithm void quickSort(int arr[], int low, int high) { if (low int partition(int arr[], int low, int high) { int pivot = arr[high]; int i = (low - 1);<pre class='brush:php;toolbar:false;'> for (int j = low; j <= high - 1; j ) { if (arr[j] < pivot) { i ; swap(&arr[i], &arr[j]); } } swap(&arr[i 1], &arr[high]); return (i 1);
}
void swap(int a, int b) { int t = a; a = b; b = t; }
This quick sorting algorithm demonstrates the performance advantages of C. By directly manipulating array elements and pointers, C can achieve efficient sorting with minimal overhead.
Object-Oriented Programming and Polymorphism
Another core feature of C is its support for object-oriented programming (OOP). Polymorphism is an important concept in OOP, which allows calling methods of derived classes using base class pointers or references, thus achieving a more flexible code structure.
// Polymorphic example class Shape { public: virtual void draw() { std::cout << "Drawing a shape" << std::endl; } }; <p>class Circle : public Shape { public: void draw() override { std::cout << "Drawing a circle" << std::endl; } };</p><p> class Rectangle : public Shape { public: void draw() override { std::cout << "Drawing a rectangle" << std::endl; } };</p><p> int main() { Shape <em>shape1 = new Circle(); Shape</em> shape2 = new Rectangle();</p><pre class='brush:php;toolbar:false;'> shape1->draw(); // Output: Drawing a circle shape2->draw(); // Output: Drawing a rectangle delete shape1; delete shape2; return 0;
}
This example shows how C can implement flexible code design through virtual functions and polymorphisms. It should be noted that memory needs to be managed carefully when using polymorphism to avoid memory leaks.
Example of usage
Basic usage
The basic usage of C includes variable declarations, function definitions, and control structures. Here is a simple example showing how to write a calculator program using C.
// Basic usage example: Simple calculator #include<iostream><p> int main() { double num1, num2; char op;</p><pre class='brush:php;toolbar:false;'> std::cout << "Enter first number: "; std::cin >> num1; std::cout << "Enter operator ( , -, *, /): "; std::cin >> op; std::cout << "Enter second number: "; std::cin >> num2; switch(op) { case ' ': std::cout << num1 << " " << num2 << " = " << num1 num2 << std::endl; break; case '-': std::cout << num1 << " - " << num2 << " = " << num1 - num2 << std::endl; break; case '*': std::cout << num1 << " * " << num2 << " = " << num1 * num2 << std::endl; break; case '/': if(num2 != 0) std::cout << num1 << " / " << num2 << " = " << num1 / num2 << std::endl; else std::cout << "Error: Division by zero" << std::endl; break; default: std::cout << "Error: Invalid operator" << std::endl; break; } return 0;
}
This example shows the basic syntax and control structure of C. It should be noted that the input and output operations of C need to use std::cin
and std::cout
, and need to include the <iostream>
header file.
Advanced Usage
Advanced usage of C includes template programming, smart pointer, and concurrent programming. The following is an example of implementing a general exchange function using template programming.
// Advanced usage example: template programming template<typename T> void swap(T& a, T& b) { T temp = a; a = b; b = temp; } <p>int main() { int x = 5, y = 10; std::cout << "Before swap: x = " << x << ", y = " << y << std::endl; swap(x, y); std::cout << "After swap: x = " << x << ", y = " << y << std::endl;</p><pre class='brush:php;toolbar:false;'> double a = 3.14, b = 2.71; std::cout << "Before swap: a = " << a << ", b = " << b << std::endl; swap(a, b); std::cout << "After swap: a = " << a << ", b = " << b << std::endl; return 0;
}
This example shows how C template programming implements common code reuse. Template programming can greatly improve the flexibility and maintainability of code, but you also need to pay attention to the performance overhead of template instantiation.
Common Errors and Debugging Tips
Common errors when using C include memory leaks, null pointer dereferences, and array out of bounds. Here are some debugging tips:
- Use smart pointers such as
std::unique_ptr
andstd::shared_ptr
) to manage memory to avoid errors caused by manually managing memory. - Use debugging tools (such as GDB) to track program execution and find error locations.
- Write unit tests to ensure the correctness of each function.
Performance optimization and best practices
In practical applications, performance optimization of C is a key issue. Here are some optimization tips:
- Use the
const
keyword to optimize the compiler's optimization capabilities. - Avoid unnecessary copy operations and use moving semantics to improve efficiency.
- Use
std::vector
instead of C-style arrays for better memory management and performance.
// Performance optimization example: Using mobile semantics #include<iostream> #include<vector><p> class MyClass { public: MyClass() { std::cout << "Constructor called" << std::endl; } MyClass(const MyClass&) { std::cout << "Copy constructor called" << std::endl; } MyClass(MyClass&&) noexcept { std::cout << "Move constructor called" << std::endl; } };</p><p> int main() { std::vector<MyClass> vec; vec.push_back(MyClass()); // Trigger the move constructor</p><pre class='brush:php;toolbar:false;'> return 0;
}
This example shows how to use mobile semantics to optimize performance. By avoiding unnecessary copy operations, the execution efficiency of the program can be significantly improved.
In programming practice, the use of C needs to follow some best practices:
- Write clear, readable code, using meaningful variable and function names.
- Follow the RAII (Resource Acquisition Is Initialization) principle to ensure the correct management of resources.
- Use modern C features (such as auto, lambda expressions, etc.) to simplify code and improve maintainability.
In general, the continuous use of C is due to its powerful performance, flexible programming paradigm and a wide range of application areas. Despite the steep learning curve, the rewards of mastering C are huge. I hope this article can help you better understand the charm of C and flexibly apply it in actual programming.
The above is the detailed content of The Continued Use of C : Reasons for Its Endurance. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


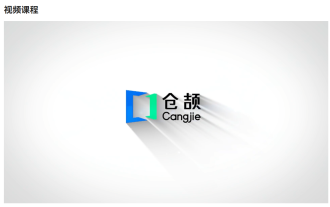
According to news from this site on June 24, at the keynote speech of the HDC2024 Huawei Developer Conference on June 21, Gong Ti, President of Huawei Terminal BG Software Department, officially announced Huawei’s self-developed Cangjie programming language. This language has been developed for 5 years and is now available for developer preview. Huawei's official developer website has now launched the official introductory tutorial video of Cangjie programming language to facilitate developers to get started and understand it. This tutorial will take users to experience Cangjie, learn Cangjie, and apply Cangjie, including using Cangjie language to estimate pi, calculate the stem and branch rules for each month of 2024, see N ways of expressing binary trees in Cangjie language, and use enumeration types to implement Algebraic calculations, signal system simulation using interfaces and extensions, and new syntax using Cangjie macros, etc. This site has tutorial access address: ht
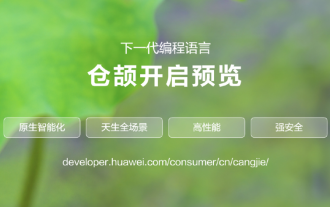
This site reported on June 21 that at the HDC2024 Huawei Developer Conference this afternoon, Gong Ti, President of Huawei Terminal BG Software Department, officially announced Huawei’s self-developed Cangjie programming language and released a developer preview version of HarmonyOSNEXT Cangjie language. This is the first time Huawei has publicly released the Cangjie programming language. Gong Ti said: "In 2019, the Cangjie programming language project was born at Huawei. After 5 years of R&D accumulation and heavy R&D investment, it finally meets global developers today. Cangjie programming language integrates modern language features, comprehensive compilation optimization and Runtime implementation and out-of-the-box IDE tool chain support create a friendly development experience and excellent program performance for developers. "According to reports, Cangjie programming language is an all-scenario intelligence tool.
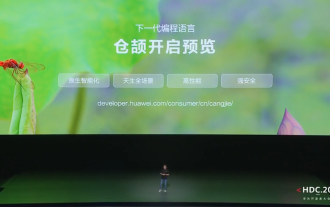
According to news from this site on June 21, Huawei’s self-developed Cangjie programming language was officially unveiled today, and the official announced the launch of HarmonyOSNEXT Cangjie language developer preview version Beta recruitment. This upgrade is an early adopter upgrade to the developer preview version, which provides Cangjie language SDK, developer guides and related DevEcoStudio plug-ins for developers to use Cangjie language to develop, debug and run HarmonyOSNext applications. Registration period: June 21, 2024 - October 21, 2024 Application requirements: This HarmonyOSNEXT Cangjie Language Developer Preview Beta recruitment event is only open to the following developers: 1) Real names have been completed in the Huawei Developer Alliance Certification; 2) Complete H
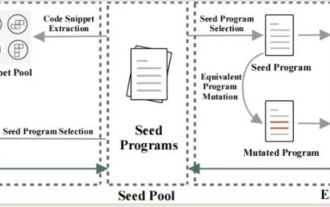
According to news from this site on June 22, Huawei yesterday introduced Huawei’s self-developed programming language-Cangjie to developers around the world. This is the first public appearance of Cangjie programming language. According to inquiries on this site, Tianjin University and Beijing University of Aeronautics and Astronautics were deeply involved in the research and development of Huawei’s “Cangjie”. Tianjin University: Cangjie Programming Language Compiler The software engineering team of the Department of Intelligence and Computing of Tianjin University joined hands with the Huawei Cangjie team to deeply participate in the quality assurance research of the Cangjie programming language compiler. According to reports, the Cangjie compiler is the basic software that is symbiotic with the Cangjie programming language. In the preparatory stage of the Cangjie programming language, a high-quality compiler that matches it became one of the core goals. As the Cangjie programming language evolves, the Cangjie compiler is constantly being upgraded and improved. In the past five years, Tianjin University
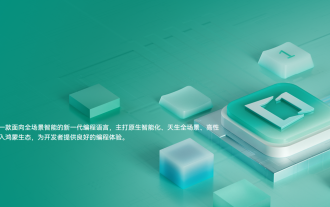
According to news from this site on June 21, before the HDC2024 Huawei Developer Conference, Huawei’s self-developed Cangjie programming language was officially unveiled, and the Cangjie official website is now online. The official website introduction shows that Cangjie programming language is a new generation programming language for all-scenario intelligence, focusing on "native intelligence, natural all-scenarios, high performance, and strong security." Integrate into the Hongmeng ecosystem to provide developers with a good programming experience. The official website attached to this site introduces as follows: Native intelligent programming framework embedded with AgentDSL, organic integration of natural language & programming language; multi-Agent collaboration, simplified symbolic expression, free combination of patterns, supporting the development of various intelligent applications. Innately lightweight and scalable runtime for all scenes, modular layered design, no matter how small the memory is, it can be accommodated; all-scenario domain expansion
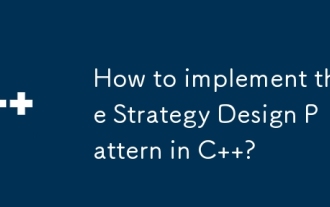
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
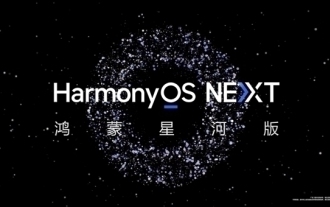
According to news on June 21, this afternoon, Huawei Developer Conference 2024 will be officially opened. "Pure-blood Hongmeng" Harmony OS NEXT is naturally a top priority. According to the plan previously revealed by Yu Chengdong, the public beta may be officially announced this afternoon, and ordinary consumers can also try out "pure-blood Harmony". According to reports, the first batch of supported mobile phones are the Mate60 series and Pura70 series. It is worth noting that as a "pure-blooded Hongmeng", HarmonyOSNEXT has removed the traditional Linux kernel and AOSP Android open source code and developed the entire stack in-house. According to the latest report from Sina Technology, Huawei will also complete the last link of Hongmeng Ecosystem and expand its presence in the world.
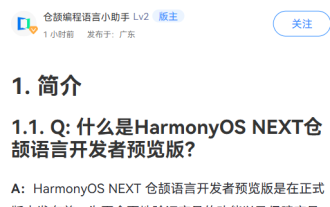
According to news from this site on June 21, Huawei’s self-developed Cangjie programming language was officially unveiled today, and the HarmonyOSNEXT Cangjie language developer preview version Beta recruitment was launched. The Cangjie Programming Language Q&A page on Huawei's official website shows that Cangjie Programming Language is a next-generation application programming language oriented to all-scenario intelligence. It focuses on native intelligence, natural all-scenarios, high performance and strong security. It is combined with Hongmeng system to provide good programming. experience. For different business scenarios, Hongmeng Ecosystem provides application developers with multi-language hybrid development capabilities such as Cangjie and ArkTS. Cangjie and ArkTS develop together and form complementary advantages in the Hongmeng ecosystem. Cangjie is more suitable for business scenarios with high performance and high concurrency requirements. The goal of Cangjie programming language is to create Hongmeng applications that can perform tasks concurrently.
