PHP is A-OK for Templating
PHP template engines are often criticized for their ease of producing poor-quality code, but this is not necessarily true. Let's see how the PHP project enforces a basic model-view-controller (MVC) structure without relying on a dedicated template engine.
First, a brief review of the history of PHP
PHP's history as an HTML template tool is full of twists and turns.
One of the earliest programming languages used for HTML templates was C, but it quickly found that it was cumbersome to use and was often not suitable for this task.
Rasmus Lerdorf created PHP with this in mind. He is not against using C to handle backend business logic, but wants a better way to generate frontend dynamic HTML. PHP was originally designed as a template language, but over time it adopted more features and eventually became a standalone, complete programming language.
PHP's unique ability to switch between programming mode and HTML mode is considered very convenient, but it also makes it easy for programmers to write hard-to-maintain code—code that mixes business logic and template logic together. A PHP file may start with some HTML templates and then suddenly penetrate into an advanced SQL query without warning. This structure is difficult to read and reuse HTML templates.
Over time, the Web development community has found that enforcing a strict MVC structure for PHP projects is becoming increasingly valuable. The template engine is created as a way to effectively separate the view from its controller.
To accomplish this task, template engines usually have the following features:
- The engine deliberately weakens the capabilities of business logic. For example, if a developer wants to execute a database query, they need to do that query in the controller and then pass the result to the template. It is impossible to query the HTML in the template.
- The engine handles common security risks behind the scenes. The template usually automatically escapes any dangerous HTML even if the developer fails to verify user input and pass it directly into the template.
Template engines are now a major feature in many web technology stacks. They create more maintainable and safer code bases, so it's no surprise.
However, pure PHP can also be used to handle HTML templates . You may want to do this for a number of reasons. Maybe you are working on a legacy project and don't want to introduce any extra dependencies, or you are working on a very small project and prefer to keep it lightweight. Or, this decision isn't up to you at all.
Use cases for PHP templates
One of the things I often implement pure PHP templates is WordPress, which does not enforce strict MVC structures by default. I find it a bit too tedious to introduce a full template engine, but I still want to separate my business logic from my templates and want my views to be reusable.
Whatever your reasoning is, using pure PHP to define your HTML templates is sometimes preferred. This article explores how to do this in a more professional way. This approach represents a practical tradeoff between the infamous spaghetti code style of PHP templates and the "logic-not allowed" approach provided by the formal template engine.
Let's take a deep dive into examples of how to put a basic template system into practice. Again, we use WordPress as an example, but this can be replaced with a pure PHP environment or many other environments. You don't need to be familiar with WordPress to continue reading.
The goal is to break our views into components and create a clear distinction between business logic and HTML templates. Specifically, we will create a view that shows the card grid. Each card will display the title, summary and author of the recent article.
Step 1: Get the data to be presented
The first step is to get the data we want to display in the view. This may involve executing SQL queries or indirect access to the database using framework/CMS's ORM or helper functions. It can also involve making HTTP requests to external APIs or collecting user input from forms or query strings.
In this example, we will use WordPress's get_posts
helper function to get some posts to be displayed on our homepage.
<?php // index.php $wp_posts = get_posts([ 'numberposts' => 3 ]);
We now have access to the data we want to display in the card grid, but we need to do some extra work before passing it to our view.
Step 2: Prepare the template data
The get_posts
function returns an array of WP_Post objects. Each object contains the article title, summary, and author information we need, but we don't want to couple our views to the WP_Post object type because we may want to display other types of data on our cards elsewhere in the project.
Instead, it makes sense to actively convert each article object to a neutral data type (such as an associative array):
<?php // index.php $wp_posts = get_posts([ 'numberposts' => 3 ]); $cards = array_map(function ($wp_post) { Return [ 'heading' => $wp_post->post_title, 'body' => $wp_post->post_excerpt, 'footing' => get_author_name($wp_post->post_author) ]; }, $wp_posts);
In this case, each WP_Post object is converted to an associative array using the array_map
function. Note that the keys for each value are not title, excerpt, and author, but are given more general names: heading, body, and footing. We do this because the Card Mesh component is designed to support any type of data, not just articles. For example, it can be easily used to display recommendation grids containing references and customer names.
Once the data is ready, it can now be passed into our render_view
function:
<?php // index.php // Data fetching and formatting are the same as before render_view('cards_grid', [ 'cards' => $cards ]);
Of course, render_view
function does not exist yet. Let's define it.
Step 3: Create a rendering function
// Define in functions.php or other locations that make it globally available. // If you are worried about possible conflicts in the global namespace, // You can define this function as a static method of the namespace class function render_view($view, $data) { extract($data); require('views/' . $view . '.php'); }
This function accepts the name of the view to be rendered and an associative array representing any data to be displayed. The extract
function takes each item in the associative array and creates a variable for it. In this example, we now have a variable called $cards
that contains the items we prepared in index.php.
Since the view executes in its own functions, it has its own scope. This is good because it allows us to use simple variable names without worrying about conflicts.
The second line of our function prints the view that matches the passed name. In this case, it looks for the view in views/cards_grid.php
. Let's go ahead and create the file.
Step 4: Create a template
<?php /* views/cards_grid.php */ ?>
This template uses the $cards
variable that you just extracted and renders it as an unordered list. For each card in the array, the template renders a subview: a single card view.
A template with a single card is useful because it gives us the flexibility to render a single card directly or use it in another view elsewhere in the project.
Let's define the basic card view:
<?php /* views/card.php */ ?> <div> <?php if (!empty($heading)) : ?> <h4><?php echo htmlspecialchars($heading) ?></h4> <?php endif; ?> <?php if (!empty($body)) : ?> <p><?php echo htmlspecialchars($body) ?></p> <?php endif; ?> <?php if (!empty($footing)) : ?> <?php echo htmlspecialchars($footing) ?> <?php endif; ?> </div>
Since $card
passed to the render function contains keys for heading, body, and footing, variables with the same name can now be used in the template.
In this example, we can be fairly sure that our data is not XSS dangerous, but it is possible that this view may later be used with user input, so it is prudent to pass each value to htmlspecialchars
. If a script tag exists in our data, it will be safely escaped.
It is generally helpful to check if each variable contains non-null values before rendering. This allows variables to be omitted without leaving empty HTML tags in our tags.
The PHP template engine is great, but it is sometimes appropriate to use PHP to accomplish its original design purpose: generate dynamic HTML .
Template in PHP does not have to cause spaghetti-style code that is difficult to maintain. With a little foreseeable viewing, we can implement a basic MVC system that separates the views and controllers from each other, and this can be done with a surprisingly small amount of code.
The above is the detailed content of PHP is A-OK for Templating. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




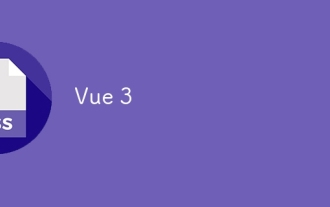
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
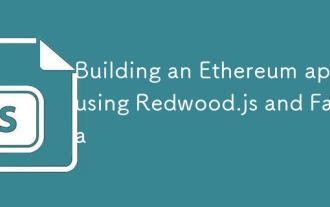
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
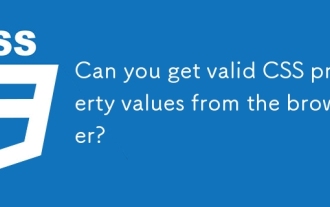
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
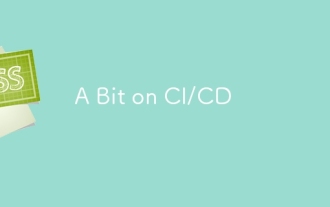
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
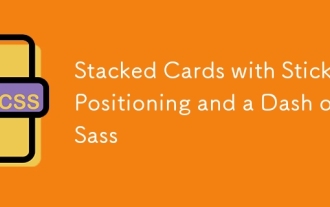
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
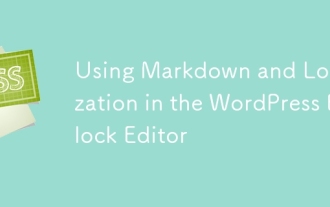
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
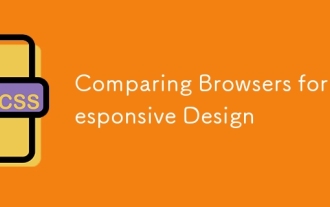
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
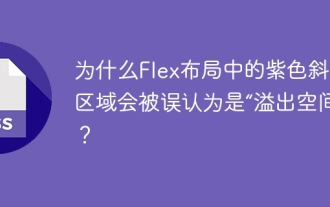
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
