HTML and React: The Relationship Between Markup and Components
introduction
<p> In modern front-end development, the relationship between HTML and React is like the relationship between dancers and dance steps, which are closely connected but each performs its own duties. Today we will explore this relationship in depth and understand how they work together to build a colorful user interface. Through this article, you will learn how to use HTML effectively in React and how to make your components more expressive and maintainable.The basic concepts of HTML and React
<p> HTML is the skeleton of a web page, which defines the structure and semantics of content. React is a powerful JavaScript library that builds user interfaces through componentization. React components can be regarded as smart versions of HTML elements, which can not only contain HTML, but also carry state and logic. <p> When we talk about React, we are actually talking about a new way of thinking - moving from static HTML pages to dynamic, interactive component systems. In React, we write code that looks like HTML through JSX syntax, but in fact the code is executed in JavaScript.Fusion of React components and HTML
<p> In React, components are defined by JSX syntax, which is a kind of HTML-like syntax, but it is actually syntactic sugar for JavaScript objects. Let's look at a simple example:function Welcome(props) { return <h1 id="Hello-props-name">Hello, {props.name}</h1>; }
<h1>
tag is part of the HTML, but it is embedded in the React component. React will render this component into a real DOM element, but before that, it will convert it into a React element.Component life cycle and HTML rendering
<p> React components have a life cycle in which components go through stages such as mount, update and uninstall. At each stage, React decides how to render HTML based on the state and properties of the component.class LifecycleExample extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } componentDidMount() { console.log('Component mounted'); } render() { Return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.setState({ count: this.state.count 1 })}> Increment </button> </div> ); } }
<div>
, <p>
and <button>
are all HTML elements, but they are wrapped in a React component. React rerenders these HTML elements according to the state changes of the component.Optimize HTML structure using React components
<p> React components can not only contain HTML, but also other React components, so that complex user interfaces can be built. In this way, we can break down the HTML structure into smaller, reusable components, thereby improving the maintainability of the code.function Header() { return <header><h1 id="My-Website">My Website</h1></header>; } function Footer() { return <footer><p>© 2023 My Company</p></footer>; } function App() { Return ( <div> <Header /> <main> <h2 id="Welcome-to-my-site">Welcome to my site!</h2> <p>This is the main content.</p> </main> <Footer /> </div> ); }
Header
, Footer
and App
. Such decomposition not only makes the code easier to understand and maintain, but also allows us to manage state and logic independently in different components.Performance optimization and best practices
<p> There are some performance optimizations and best practices worth noting when using React and HTML. First, avoid unnecessary re-rendering. You can do this by usingshouldComponentUpdate
method or using React.memo
.class OptimizedComponent extends React.Component { shouldComponentUpdate(nextProps, nextState) { return nextProps.value !== this.props.value; } render() { return <div>{this.props.value}</div>; } }
key
attribute rationally to help React identify which elements have changed in the array, thereby improving rendering performance.function ListItem(props) { return <li>{props.value}</li>; } function NumberList(props) { const numbers = props.numbers; Return ( <ul> {numbers.map((number) => <ListItem key={number.toString()} value={number} /> )} </ul> ); }
FAQs and debugging tips
<p> There are some common problems you may encounter when using React and HTML. For example, the component is not rendering as expected, which may be due to improper state management or props delivery errors. You can debug these issues through React DevTools and view the component's props and state. <p> Another common problem is that the style is not in effect, which may be due to priority issues with CSS or the style is overwritten. You can find out what the problem is by checking the calculation style of the element.Summarize
<p> The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. By understanding this relationship, we can better utilize React's componentized features while maintaining the structure and semantics of HTML. I hope this article can help you better understand and apply React and HTML, and build more efficient and easier to maintain Web applications.The above is the detailed content of HTML and React: The Relationship Between Markup and Components. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


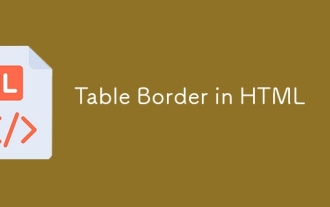
Guide to Table Border in HTML. Here we discuss multiple ways for defining table-border with examples of the Table Border in HTML.
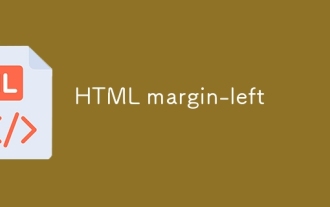
Guide to HTML margin-left. Here we discuss a brief overview on HTML margin-left and its Examples along with its Code Implementation.
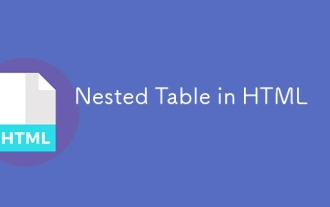
This is a guide to Nested Table in HTML. Here we discuss how to create a table within the table along with the respective examples.
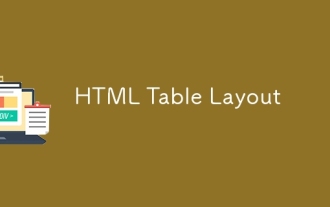
Guide to HTML Table Layout. Here we discuss the Values of HTML Table Layout along with the examples and outputs n detail.
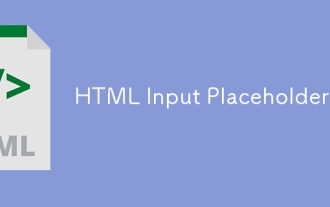
Guide to HTML Input Placeholder. Here we discuss the Examples of HTML Input Placeholder along with the codes and outputs.
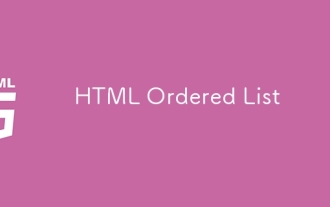
Guide to the HTML Ordered List. Here we also discuss introduction of HTML Ordered list and types along with their example respectively
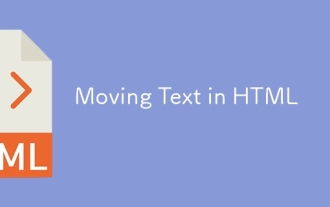
Guide to Moving Text in HTML. Here we discuss an introduction, how marquee tag work with syntax and examples to implement.
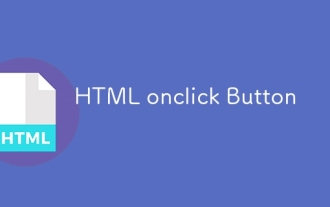
Guide to HTML onclick Button. Here we discuss their introduction, working, examples and onclick Event in various events respectively.
