What are Weak References in PHP and when are they useful?
In PHP, weak references are implemented through the WeakReference class and do not prevent the garbage collector from reclaiming objects. Weak references are suitable for scenarios such as caching systems and event listeners. It should be noted that it cannot guarantee the survival of objects and that garbage collection may be delayed.
introduction
In PHP, Weak References is a often overlooked but very useful feature. Today we will dig deeper into this topic and explore what weak quotes are and what scenarios they are particularly useful in. Through this article, you will not only understand the basic concepts of weak citations, but also master their practical application scenarios and some possible pitfalls.
What exactly are weak references in PHP? Simply put, a weak reference is a reference to an object that does not prevent the garbage collector from reclaiming the object. When an object has only weak references pointing to it, the garbage collector is free to recycle the object's memory. Weak references are useful in some cases, especially if you need to monitor the lifecycle of an object but don't want to block garbage collection.
Weak references are implemented in PHP through WeakReference
class. This class allows you to create weak references to objects without affecting garbage collection of objects. Let's take a look at the specific usage scenarios of weak references and some details that need to be paid attention to.
When using weak references in PHP, it should be noted that weak references do not guarantee the survival of objects. If you need to make sure that objects are not recycled for a certain period of time, weak references are not a suitable option. On the contrary, if your purpose is to perform some operations after the object is recycled, or if you need to monitor the life cycle of the object without affecting garbage collection, weak references are very suitable.
Here is a simple example using weak references:
<?php $object = new stdClass(); $weakRef = WeakReference::create($object); // The object still exists var_dump($weakRef->get()); // Output object// Remove strong reference $object = null; // After garbage collection, weak reference returns null gc_collect_cycles(); var_dump($weakRef->get()); // Output null
This example shows how a weak reference returns null
after an object is garbage collected. In practical applications, weak references can be used in caching systems, event listeners and other scenarios.
The working principle of weak references mainly depends on PHP's garbage collection mechanism. When an object has no strong reference pointing to it, the garbage collector marks it as recyclable. If there is a weak reference, the garbage collector recycles the object first and then sets the weak reference to null
. This process ensures that weak references do not prevent garbage collection, but also allows you to perform some operations after the object is recycled.
When using weak references, it is important to note that PHP's garbage collection mechanism may not recycle objects immediately. This means that even if the object has no strong reference, it may still exist for a while. Therefore, in codes that rely on weak references, the possibility of this delayed recycling needs to be considered.
Let's look at some practical usage scenarios:
Cache system
In a cache system, weak references can be used to store references to objects without preventing them from being garbage collected. For example, you can use weak references to cache some temporary data, which will be automatically recycled when it is no longer referenced by other parts.
<?php class Cache { private $cache = []; public function set($key, $value) { $this->cache[$key] = WeakReference::create($value); } public function get($key) { $weakRef = $this->cache[$key] ?? null; return $weakRef ? $weakRef->get() : null; } } $cache = new Cache(); $object = new stdClass(); $cache->set('key', $object); // Remove strong reference $object = null; // After garbage collection, weak references in the cache return null gc_collect_cycles(); var_dump($cache->get('key')); // Output null
This example shows how to use weak references in a cache system. It should be noted that weak references cannot guarantee the survival of objects, so when using weak reference cache, you need to consider the situation that objects may be recycled.
Event Listener
In event listeners, weak references can be used to store references to listener objects without preventing them from being garbage collected. For example, you can use weak references to store references to event listeners, which will be automatically recycled when they are no longer referenced by other parts.
<?php class EventDispatcher { private $listeners = []; public function addListener($event, $listener) { $this->listeners[$event][] = WeakReference::create($listener); } public function dispatch($event, $data) { if (isset($this->listeners[$event])) { foreach ($this->listeners[$event] as $weakRef) { $listener = $weakRef->get(); if ($listener) { $listener($data); } } } } } class MyListener { public function __invoke($data) { echo "Received data: " . $data . "\n"; } } $dispatcher = new EventDispatcher(); $listener = new MyListener(); $dispatcher->addListener('my_event', $listener); // Trigger event $dispatcher->dispatch('my_event', 'Hello, World!'); // Output Received data: Hello, World! // Remove strong reference $listener = null; // After garbage collection, weak reference returns null gc_collect_cycles(); $dispatcher->dispatch('my_event', 'Hello, World!'); // No output
This example shows how to use weak references in event listeners. It should be noted that weak references cannot guarantee the survival of listener objects, so when storing listeners with weak references, you need to consider the situation that the listener may be recycled.
There are some common errors and debugging tips to be aware of when using weak references:
- Error 1: Relying on weak references to ensure the survival of the object : Weak references cannot guarantee the survival of the object. If you need to ensure that the object is not recycled for a certain period of time, you should use strong references.
- Error 2: Ignore the latency of garbage collection : PHP's garbage collection mechanism may not recycle objects immediately, so in code that depends on weak references, the possibility of this latency recycling needs to be considered.
- Debugging tips : When debugging weak references, you can use
gc_collect_cycles()
function to force trigger garbage collection to observe the behavior of weak references.
When it comes to performance optimization and best practices, using weak references can bring some benefits:
- Performance optimization : Weak references reduce memory usage because they do not prevent the garbage collector from reclaiming objects. In large-scale applications, this can significantly improve performance.
- Best practice : When using weak references, you should try to avoid relying on weak references to ensure the survival of objects, but instead regard them as a tool to monitor the life cycle of objects. In code, the use of weak references should be explicitly commented to improve the readability and maintainability of the code.
Overall, weak references are a powerful tool in PHP that monitor the life cycle of an object without preventing garbage collection. By rationally using weak references, memory usage can be optimized and application performance and maintainability can be improved.
The above is the detailed content of What are Weak References in PHP and when are they useful?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


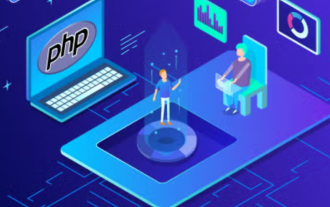
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
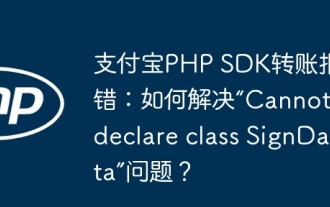
Alipay PHP...
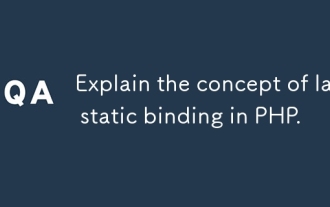
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
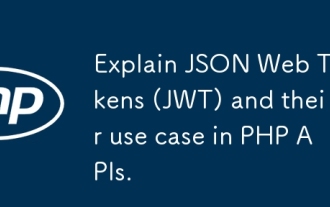
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
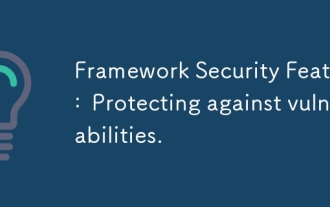
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
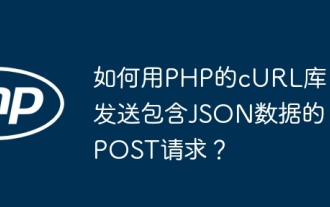
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
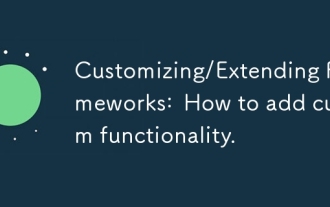
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
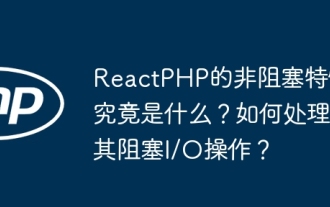
An official introduction to the non-blocking feature of ReactPHP in-depth interpretation of ReactPHP's non-blocking feature has aroused many developers' questions: "ReactPHPisnon-blockingbydefault...
