What I Like About Writing Styles with Svelte
Svelte's rising popularity (over 24,000 GitHub stars) is well-deserved. Created by Rich Harris (also behind Rollup), it's arguably the simplest JavaScript framework available. While its performance, built-in state management, and clean markup are compelling, its CSS handling is a standout feature for me.
Single-File Components: Elegance in Simplicity
React's documentation famously states, "React does not have an opinion about how styles are defined." Svelte takes a different approach: "A UI framework that doesn’t have a built-in way to add styles to your components is unfinished," says Harris.
Svelte lets you write CSS directly within your components, mirroring standard project workflows. CSS-in-JS solutions remain an option, but Svelte's approach streamlines development by combining JavaScript, markup, and styling in a single .svelte
file – a familiar concept for Vue users.
// button.svelte button { border-radius: 0; background-color: aqua; } <slot></slot>
Scoped Styles: Conflict-Free Styling
Svelte's default scoped styling prevents style conflicts between components. Similar to CSS Modules or CSS-in-JS, it generates unique class names during compilation. This allows the use of simple selectors (like div
and button
) without complex class name management. Multiple buttons within a component still require classes for differentiation, and these classes are also scoped.
While the generated class names (e.g., svelte-433xyz
) might seem cryptic in DevTools, this approach is significantly simpler than BEM naming conventions. You can combine Svelte's scoped styles with external stylesheets for global styles, utility classes, or CSS frameworks.
Global Styles: Maintaining Control
Global styles can be defined in a linked stylesheet or, if necessary, within a Svelte component using :global
. This allows targeted overriding of scoping when needed. For example, a modal component might use:
:global(.noscroll) { overflow: hidden; }
Unused Style Detection: Efficiency Boost
Svelte's compiler flags unused styles, enhancing efficiency by identifying and eliminating redundant code.
Concise Conditional Classes: Streamlined Syntax
Svelte simplifies conditional class application. When the JavaScript variable and class name match, the syntax is exceptionally concise:
export let big = false; export let ghost = false; .big { font-size: 20px; display: block; width: 100%; } .ghost { background-color: transparent; border: solid currentColor 2px; } <slot></slot>
The ghost
and big
classes are applied based on the corresponding props. Even if names don't match, multiple classes can be passed efficiently via a single prop:
let class_name = ''; export { class_name as class }; <slot></slot>
Used like this:
<button class="my-class another-class">Click Me</button>
From BEM to Svelte: A Simplicity Revolution
Compare a BEM-styled card component:
.c-card { border-radius: 3px; border: solid 2px; } .c-card__title { text-transform: uppercase; } .c-card__text { color: gray; } .c-card--featured { border-color: gold; }
To its Svelte equivalent:
div { border-radius: 3px; border: solid 2px; } h2 { text-transform: uppercase; } p { color: gray; } .featured { border-color: gold; } <div class:featured=""> <h2 id="title">{title}</h2> <p><slot></slot></p> </div>
The Svelte version is significantly more concise and readable.
Preprocessor Compatibility: Expanding Options
While less crucial with Svelte, preprocessors are supported via svelte-preprocess
. This allows the use of Sass, Less, Stylus, and PostCSS. For Sass, install svelte-preprocess
and node-sass
, configure rollup.config.js
, and use type="text/scss"
or lang="scss"
in the style tag.
$pink: rgb(200, 0, 220); p { color: black; span { color: $pink; } }
Dynamic Values and CSS Variables: A Powerful Combination
While Svelte can't directly use JavaScript variables in CSS, CSS variables provide a workaround:
export let cols = 4; ul { display: grid; width: 100%; grid-column-gap: 16px; grid-row-gap: 16px; grid-template-columns: repeat(var(--columns), 1fr); } <slot></slot>
In conclusion, Svelte offers a remarkably intuitive and user-friendly styling API, surpassing other approaches I've used (Sass, Shadow DOM, CSS-in-JS, BEM, atomic CSS, and PostCSS). For a deeper dive, see Rich Harris's "The Zen of Just Writing CSS."
The above is the detailed content of What I Like About Writing Styles with Svelte. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
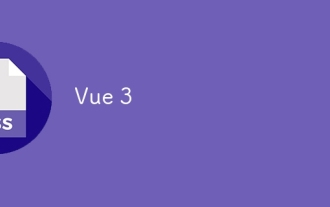
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
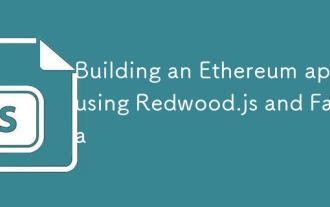
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
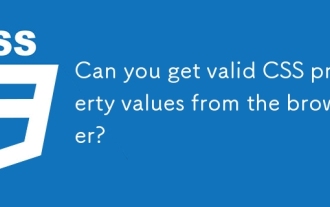
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
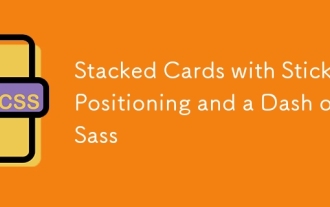
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
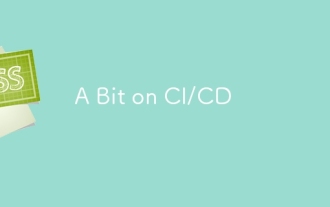
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
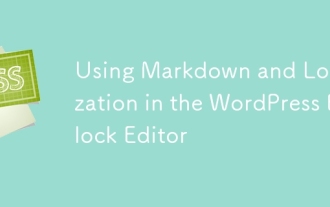
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
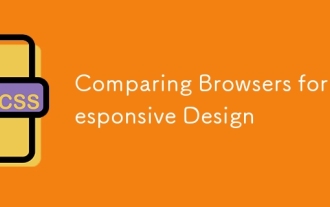
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
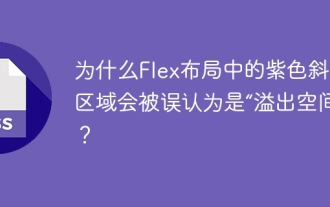
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
