How to build a vscode extension
Building a VS Code extension requires understanding its architecture and extension API, which is essentially a Node.js application that interacts with VS Code through the API, and the core API covers command, language support, debugging, and status bar capabilities. Common pitfalls include inaccurate syntax rules, data synchronization issues, and debugging complexity. But VS Code extensions also have rich APIs and documentation that follow best practices, including leveraging debugging tools, code specifications, and version control, which can improve development efficiency and avoid pitfalls.
How to build a VS Code extension: From Getting Started to Advanced
With its powerful expansion capabilities, VS Code has become the editor of choice for many developers. Building your own VS Code extension can greatly improve development efficiency and even solve some difficult problems in specific scenarios. But this is not easy, and requires a deep understanding of the architecture and extension API of VS Code. This article will share my experience in building VS Code extensions and focus on some key points and common pitfalls.
Getting started: Understand the extension architecture
A VS Code extension is essentially a Node.js application that interacts with the VS Code main program through a series of APIs. Understanding this core concept is crucial. Instead of directly operating the UI elements of VS Code, you use the API to trigger events, modify editor status, register commands, etc. This makes the extensions have good scalability and stability, and also avoids the potential risks caused by direct operation of the UI.
Core API and functional modules
VS Code provides a rich API covering almost all the features you can think of:
- Commands: This is the main way to extend interaction with users. You can register custom commands to let users trigger your extended functions via shortcut keys or menus. For example, an extension to automatically format code will register a "Format Document" command.
<code class="typescript">// package.json { "contributes": { "commands": [ { "command": "myextension.formatDocument", "title": "Format Document" } ] } } // extension.ts import * as vscode from 'vscode'; export function activate(context: vscode.ExtensionContext) { let disposable = vscode.commands.registerCommand('myextension.formatDocument', () => { // 你的格式化代码逻辑const editor = vscode.window.activeTextEditor; if (editor) { // 使用合适的格式化工具,例如prettier // ... } }); context.subscriptions.push(disposable); }</code>
- Language Support: This is the core of building syntax highlighting, code completion, code inspection and other functions. You need to write a syntax definition file (usually a
.tmLanguage
file) to define the syntax rules of the language. This part is quite complex and requires a certain amount of regular expression and syntax analysis knowledge. A common pitfall is that the grammar rules are not written accurately enough, resulting in errors in highlighting or completion. - Debugging: VS Code's built-in debugger is very powerful, and you can easily debug your extension code. This is essential for troubleshooting bugs in extensions. Remember to configure the correct debug parameters in
launch.json
. - StatusBar: You can display some information to the user through the status bar, such as how the current file is encoded or the status of the task in progress.
Actual case: A simple code snippet management extension
I once developed a simple code snippet management extension that allows users to save and manage custom code snippets. This extension uses VS Code's storage API to save user data and manage fragments through commands. One of the problems I encountered during this process is how to handle the synchronization of user data between different VS Code instances. Finally, I used VS Code's workspace.getConfiguration()
API to read and save configurations, and achieved cross-instance synchronization through user configuration files.
Summary of advantages and disadvantages
The advantages of VS Code extensions are its strong API, active community and rich documentation. However, building extensions is not without challenges. You need to master Node.js and TypeScript, and have a deep understanding of the architecture of VS Code. The learning curve is relatively steep and debugging may be more complicated.
Best Practices
- Make full use of VS Code's debugging tools.
- Follow code specifications and write maintainable code.
- Use a version control system (such as Git) to manage your extension code.
- Perform adequate testing before releasing the extension.
All in all, building VS Code extensions is a challenging but also highly rewarding process. Through learning and practice, you can master this skill and create tools that can improve your development efficiency. Remember, practice to gain true knowledge, try more hands-on, and you can become a VS Code expansion development expert.
The above is the detailed content of How to build a vscode extension. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


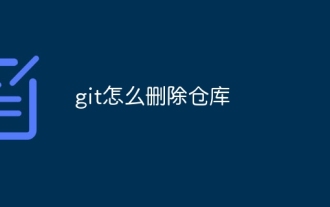
To delete a Git repository, follow these steps: Confirm the repository you want to delete. Local deletion of repository: Use the rm -rf command to delete its folder. Remotely delete a warehouse: Navigate to the warehouse settings, find the "Delete Warehouse" option, and confirm the operation.
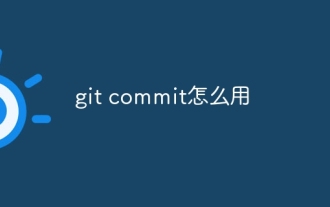
Git Commit is a command that records file changes to a Git repository to save a snapshot of the current state of the project. How to use it is as follows: Add changes to the temporary storage area Write a concise and informative submission message to save and exit the submission message to complete the submission optionally: Add a signature for the submission Use git log to view the submission content
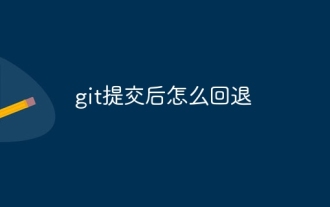
To fall back a Git commit, you can use the git reset --hard HEAD~N command, where N represents the number of commits to fallback. The detailed steps include: Determine the number of commits to be rolled back. Use the --hard option to force a fallback. Execute the command to fall back to the specified commit.

Connecting a Git server to the public network includes five steps: 1. Set up the public IP address; 2. Open the firewall port (22, 9418, 80/443); 3. Configure SSH access (generate key pairs, create users); 4. Configure HTTP/HTTPS access (install servers, configure permissions); 5. Test the connection (using SSH client or Git commands).
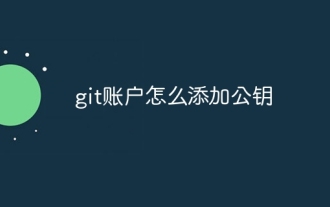
How to add a public key to a Git account? Step: Generate an SSH key pair. Copy the public key. Add a public key in GitLab or GitHub. Test the SSH connection.
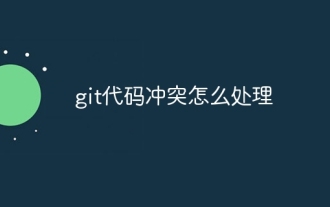
Code conflict refers to a conflict that occurs when multiple developers modify the same piece of code and cause Git to merge without automatically selecting changes. The resolution steps include: Open the conflicting file and find out the conflicting code. Merge the code manually and copy the changes you want to keep into the conflict marker. Delete the conflict mark. Save and submit changes.
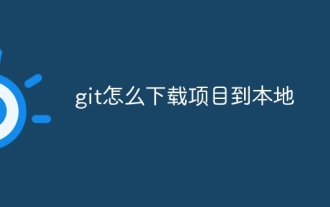
To download projects locally via Git, follow these steps: Install Git. Navigate to the project directory. cloning the remote repository using the following command: git clone https://github.com/username/repository-name.git
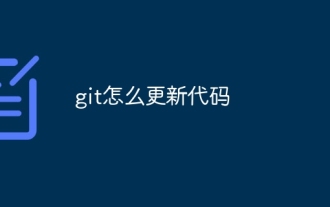
Steps to update git code: Check out code: git clone https://github.com/username/repo.git Get the latest changes: git fetch merge changes: git merge origin/master push changes (optional): git push origin master
