


The Continued Relevance of C# .NET: A Look at Current Usage
C# .NET remains important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
introduction
Since its debut, C# .NET has been a programming language and framework that developers are passionate about. Why does C# .NET still maintain its importance in today's technological environment? This article will explore the current usage of C# .NET and share some of my personal experiences and insights. By reading this article, you will learn about the application scenarios of C# .NET in modern software development, its advantages, and how to use it efficiently in your projects.
Review of basic knowledge
C# is a modern, object-oriented programming language developed by Microsoft, while .NET is a development framework provided by Microsoft that supports multiple programming languages, including C#. C# combines the .NET framework to provide developers with powerful tools and libraries, making it more efficient and convenient to develop desktop applications, web applications, mobile applications and games.
During my career, I have developed several enterprise-level applications using C# .NET, knowing their powerful capabilities in handling complex business logic and data operations. The syntax of C# is concise and clear, and combined with the rich libraries of .NET, makes the development process smoother.
Core concept or function analysis
The definition and function of C# .NET
C# .NET is a powerful combination that not only provides the flexibility and ease of use of the C# language, but also provides a rich library and service through the .NET framework. The main advantages of C# are its type safety, garbage collection mechanism and comprehensive support for object-oriented programming, while .NET provides a cross-platform running environment and rich APIs.
using System; <p>class Program { static void Main() { Console.WriteLine("Hello, C# .NET!"); } }</p>
This simple example shows the basic syntax of C# and the use of .NET. Through Console.WriteLine, we can see how convenient the class library in the System namespace provided by .NET is.
How it works
The working principle of C# .NET can be understood from two aspects: compilation and operation. C# code is first compiled into an intermediate language (IL) and then converted into machine code by the .NET runtime (CLR) when executed. This approach not only improves the portability of the code, but also enables .NET to provide features such as garbage collection and type safety.
In actual projects, I found this mechanism to be very efficient when handling large-scale applications because it can effectively manage memory and reduce the risk of memory leaks. At the same time, the .NET JIT compiler can optimize code based on the running environment to further improve performance.
Example of usage
Basic usage
The basic usage of C# .NET is very intuitive. Here is a simple class definition and instantiation example:
using System; <p>public class Person { public string Name { get; set; } public int Age { get; set; }</p><pre class='brush:php;toolbar:false;'> public void Introduction() { Console.WriteLine($"My name is {Name} and I am {Age} years old."); }
}
class Program { static void Main() { Person person = new Person { Name = "John", Age = 30 }; person.Introduce(); } }
This code shows the basic usage of C# class definitions, properties, and methods. In this way, we can easily create and manipulate objects.
Advanced Usage
C# .NET also supports many advanced features, such as asynchronous programming, LINQ query, etc. Here is an example using asynchronous programming and LINQ:
using System; using System.Linq; using System.Threading.Tasks; <p>class Program { static async Task Main() { var numbers = Enumerable.Range(1, 10); var evenNumbers = await Task.Run(() => numbers.Where(n => n % 2 == 0));</p><pre class='brush:php;toolbar:false;'> foreach (var number in evenNumbers) { Console.WriteLine(number); } }
}
This example shows how to use asynchronous programming and LINQ to process data. Asynchronous programming can improve application responsiveness, while LINQ provides powerful data query capabilities.
Common Errors and Debugging Tips
Common errors when using C# .NET include type conversion errors, empty reference exceptions, etc. Here are some debugging tips:
- Using Visual Studio's debugging tools, you can set breakpoints, view variable values, and help locate problems.
- Use the try-catch block to catch exceptions and record detailed error information in the catch block.
- Use logging tools such as Serilog or NLog to help track the operation of your application.
In my project, I found that using these tips can greatly reduce debugging time and improve development efficiency.
Performance optimization and best practices
In practical applications, how to optimize C# .NET code is a key issue. Here are some recommendations for performance optimization and best practices:
- Use asynchronous programming to improve application responsiveness, especially when handling I/O operations.
- Use the delayed execution characteristics of LINQ to avoid unnecessary data processing.
- Use the caching mechanism rationally to reduce the number of database queries.
using System; using System.Collections.Generic; using System.Linq; <p>class Program { static void Main() { List<int> numbers = Enumerable.Range(1, 1000000).ToList(); var evenNumbers = numbers.Where(n => n % 2 == 0).Take(10);</p><pre class='brush:php;toolbar:false;'> foreach (var number in evenNumbers) { Console.WriteLine(number); } }
}
This example shows how to use LINQ's latency execution characteristics to optimize performance. With Take(10), we only process the top 10 numbers that meet the criteria, avoiding traversal of the entire list.
In my experience, following these best practices not only improves the performance of your code, but also improves the readability and maintainability of your code. The power of C# .NET is that it provides a wealth of tools and libraries to help developers solve various problems efficiently.
In general, C# .NET still plays an important role in the current technology environment. Whether it is developing desktop applications, web applications or mobile applications, C# .NET provides powerful support and rich features. Through this discussion, I hope you can have a deeper understanding of the usage and advantages of C# .NET and better apply it in real projects.
The above is the detailed content of The Continued Relevance of C# .NET: A Look at Current Usage. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
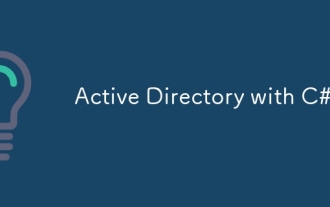
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
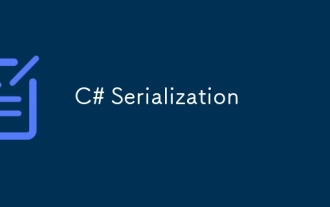
Guide to C# Serialization. Here we discuss the introduction, steps of C# serialization object, working, and example respectively.
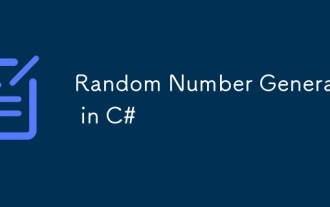
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
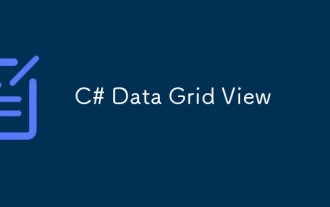
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
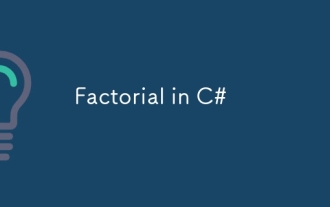
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
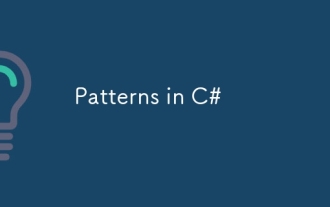
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
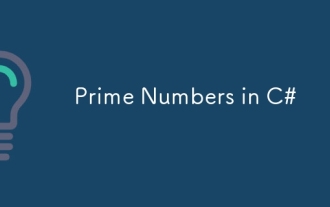
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
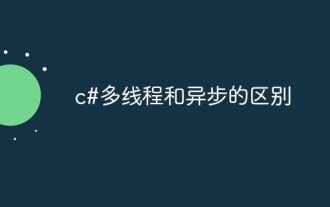
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
