Netflix's Frontend: Examples and Applications of React (or Vue)
Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) By componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.
introduction
Netflix's user interface has always been an object that front-end developers have relished. It not only provides a smooth user experience, but also demonstrates the powerful capabilities of modern front-end technology. Today we will dive into how the Netflix front-end uses React (or Vue) to build its complex and efficient user interface. Through this article, you will learn how Netflix applies these frameworks to real-world projects, while also learning some practical front-end development tips and best practices.
Review of basic knowledge
Before diving into the front-end implementation of Netflix, let's review the basic concepts of React and Vue. React is a JavaScript library developed by Facebook that focuses on building user interfaces. It makes it easier for developers to manage and reuse UI elements through a componentized approach. Vue is a progressive JavaScript framework that also supports component development, but it is known for its flexibility and easy-to-use features.
Whether it is React or Vue, they support virtual DOM, a technology that optimizes rendering performance. By comparing the differences between virtual DOM and actual DOM, only the necessary parts are updated, thereby improving the performance of the application.
Core concept or function analysis
Application of React/Vue in Netflix
The main reason why Netflix chose React as its front-end framework is its efficient component development model and a strong ecosystem. React's componentization allows Netflix to split complex user interfaces into manageable chunks, each component responsible for its own state and logic, which greatly simplifies development and maintenance.
For example, Netflix's playback page can be broken down into multiple components, such as video players, recommendation lists, user comments, etc. Each component can be developed and tested independently and then combined to form a complete page.
// Example: Netflix play page component import React from 'react'; const VideoPlayer = () => { return <div>Video Player Component</div>; }; const RecommendationList = () => { return <div>Recommendation List Component</div>; }; const UserReviews = () => { return <div>User Reviews Component</div>; }; const PlaybackPage = () => { Return ( <div> <VideoPlayer /> <RecommendationList /> <UserReviews /> </div> ); }; export default PlaybackPage;
How it works
How React works mainly depends on its virtual DOM and component lifecycle. Virtual DOM allows React to build a lightweight DOM tree in memory, and then compare the differences between the old and new virtual DOMs through the Diff algorithm, and only update the parts that need to be changed, thereby improving rendering efficiency.
The component life cycle provides the opportunity to perform specific operations at different stages of the component, such as getting data when the component is mounted, or cleaning up resources when the component is uninstalled. Netflix leverages these lifecycle approaches to manage complex user interactions and data flows.
Example of usage
Basic usage
In Netflix, basic React components are very common. For example, the user avatar component might be just a simple React component that accepts user data as props, and then renders the avatar image.
// Basic usage example: User avatar component import React from 'react'; const UserAvatar = ({ user }) => { return <img src={user.avatarUrl} alt={user.name} />; }; export default UserAvatar;
Advanced Usage
In the front-end development of Netflix, some complex needs are often encountered, such as dynamic loading of content, complex animation effects, etc. At this time, React's advanced features such as Hooks and Context APIs come in handy.
For example, Netflix might use useEffect Hook to handle asynchronous data loading, or use the Context API to manage global state, such as user login information.
// Advanced usage example: Using useEffect and Context API import React, { useEffect, useContext } from 'react'; import { UserContext } from './UserContext'; const UserProfile = () => { const { user, setUser } = useContext(UserContext); useEffect(() => { // Asynchronously load user data fetchUserData().then(data => setUser(data)); }, []); if (!user) return <div>Loading...</div>; Return ( <div> <h1 id="user-name">{user.name}</h1> <UserAvatar user={user} /> </div> ); }; export default UserProfile;
Common Errors and Debugging Tips
Common errors when developing the front-end of Netflix using React or Vue include improper component state management, performance bottlenecks, and debugging difficulties caused by complex component nesting. Here are some debugging tips:
- Use React DevTools or Vue DevTools to check component tree and state changes.
- Use performance analysis tools such as Chrome DevTools' Performance tab to identify performance bottlenecks.
- For complex component nesting, you can use React's Fragments or Vue's slots to simplify the structure.
Performance optimization and best practices
In Netflix's front-end development, performance optimization is the top priority. Here are some optimization strategies that Netflix may adopt:
- Lazy loading: Netflix will use React.lazy and Suspense to implement lazy loading of components, reducing the initial loading time.
- Code segmentation: Through tools such as Webpack, the code is divided into multiple small pieces and loaded as needed.
- Caching: Use browser cache and server cache to reduce unnecessary network requests.
In terms of best practice, Netflix's front-end team emphasizes the readability and maintainability of the code. Here are some suggestions:
- Componentization: Split the UI into small and independent components as much as possible to improve reusability and maintainability.
- State management: Use Redux or Context API reasonably to manage global state and avoid state confusion.
- Testing: Write unit tests and integration tests to ensure the reliability and stability of your code.
Through these strategies and practices, Netflix’s front-end team is able to efficiently develop and maintain its complex user interface while providing users with a smooth viewing experience.
In general, the front-end development of Netflix is a complex and interesting field. Through the application of React or Vue, Netflix not only realizes efficient user interface development, but also provides a model for front-end developers to learn and learn from. I hope this article can bring you some inspiration and practical skills to help you take a step further on the road of front-end development.
The above is the detailed content of Netflix's Frontend: Examples and Applications of React (or Vue). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
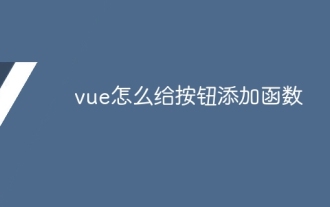
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

Function interception in Vue is a technique used to limit the number of times a function is called within a specified time period and prevent performance problems. The implementation method is: import the lodash library: import { debounce } from 'lodash'; Use the debounce function to create an intercept function: const debouncedFunction = debounce(() => { / Logical / }, 500); Call the intercept function, and the control function is called at most once in 500 milliseconds.
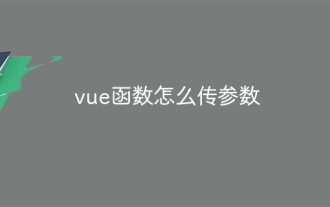
There are two main ways to pass parameters to Vue.js functions: pass data using slots or bind a function with bind, and provide parameters: pass parameters using slots: pass data in component templates, accessed within components and used as parameters of the function. Pass parameters using bind binding: bind function in Vue.js instance and provide function parameters.
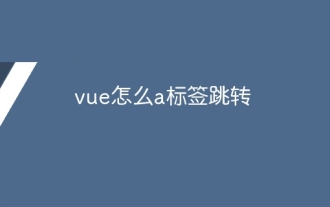
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
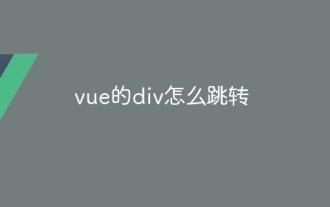
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.
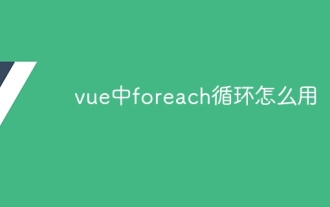
The foreach loop in Vue.js uses the v-for directive, which allows developers to iterate through each element in an array or object and perform specific operations on each element. The syntax is as follows: <template> <ul> <li v-for="item in items>>{{ item }}</li> </ul> </template>&am
