Integrating Bootstrap Styles in React: Methods and Techniques
Integrating Bootstrap in React projects can be done in two ways: 1) introduced using CDN, suitable for small projects or rapid prototyping; 2) installation using npm package manager, suitable for scenarios that require deep customization. With these methods, you can quickly build beautiful and responsive user interfaces in React.
introduction
As you step into the world of React, you may find yourself looking for a way to quickly and gracefully improve your user interface. In the process, Bootstrap is undoubtedly a powerful ally. Why choose Bootstrap? Because it provides a rich set of predefined styles and components that can greatly speed up your development process while ensuring your application has a modern look and responsive design. This article will take you into a deep dive into how to integrate Bootstrap into React projects, from basic to advanced tips, and we will explore this topic together.
After reading this article, you will learn how to introduce Bootstrap into your React project, how to use its components, and how to customize styles to meet your unique needs. Let's start this journey.
Review of basic knowledge
Bootstrap is a front-end framework based on HTML, CSS and JavaScript. It provides developers with a consistent design system, including raster systems, predefined components (such as buttons, forms, navigation bars, etc.), and responsive design tools. React is a JavaScript library for building user interfaces. It helps developers build reusable UI elements through componentized methods.
Using Bootstrap in a React project, you need to understand how React components work and how to introduce external CSS and JavaScript files in React. What's powerful about Bootstrap is that it not only provides styles, but also provides JavaScript plugins to enhance user interaction.
Core concept or function analysis
Bootstrap integration method in React
There are two main ways to integrate Bootstrap into React projects: use CDN to introduce and install using npm package manager.
Introduced using CDN
Using a CDN is the fastest way, especially for small projects or rapid prototyping. You just need to add the CSS and JavaScript links of Bootstrap in the index.html
file of the React project.
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/js/bootstrap.bundle.min.js"></script>
The advantage of this method is that it is simple and fast, but the disadvantage is that it cannot be deeply customized and locally developed.
Install using npm package manager
For larger projects or scenarios that require deep customization, installing Bootstrap using npm is a better option.
npm install bootstrap
After installation, you can import Bootstrap's CSS file in the React component:
import 'bootstrap/dist/css/bootstrap.min.css';
The advantage of this approach is that it allows more flexibility in managing dependencies and can be deeply customized. The disadvantage is that more configuration and management are required.
How it works
When you introduce Bootstrap into your React project, you actually integrate Bootstrap's CSS and JavaScript files into your application. Bootstrap's CSS file defines a series of style rules that are applied to your HTML elements to achieve predefined style effects. The JavaScript file contains Bootstrap's interactive components, such as modal boxes, drop-down menus, etc. These components enhance the user experience through JavaScript.
In React, components are the basic unit for building a UI. When you use Bootstrap's styles, you can directly apply these style class names in the React component to achieve a consistent design style.
Example of usage
Basic usage
Let's look at a simple example of how to use Bootstrap's button style in a React component.
import React from 'react'; import 'bootstrap/dist/css/bootstrap.min.css'; function ButtonExample() { Return ( <button type="button" className="btn btn-primary">Primary Button</button> ); } export default ButtonExample;
In this example, we applied btn
and btn-primary
class names of Bootstrap through className
property, thus creating a button with Bootstrap style.
Advanced Usage
In more complex scenarios, you may need to use Bootstrap's raster system to create responsive layouts. Here is an example of React components using Bootstrap raster system:
import React from 'react'; import 'bootstrap/dist/css/bootstrap.min.css'; function GridExample() { Return ( <div className="container"> <div className="row"> <div className="col-md-4"> <h2 id="Column">Column 1</h2> <p>This is the first column.</p> </div> <div className="col-md-4"> <h2 id="Column">Column 2</h2> <p>This is the second column.</p> </div> <div className="col-md-4"> <h2 id="Column">Column 3</h2> <p>This is the third column.</p> </div> </div> </div> ); } export default GridExample;
In this example, we used Bootstrap's container
, row
, and col-md-4
class names to create a three-column responsive layout.
Common Errors and Debugging Tips
Common errors when using Bootstrap include style conflicts and JavaScript plug-ins not loading correctly. Here are some debugging tips:
- Style conflict : If your custom style conflicts with Bootstrap's style, you can use a higher CSS priority (such as using
!important
) to override Bootstrap's styles, or use CSS modular technology to isolate the styles. - JavaScript plugin is not loaded : Make sure you have correctly introduced the JavaScript file of Bootstrap and are correctly using Bootstrap's JavaScript component in the React component.
Performance optimization and best practices
When using Bootstrap, there are several ways to optimize performance and improve development efficiency:
- Loading on demand : If you only use a portion of Bootstrap's features, consider using on demand loading packages like
bootstrap-icons
instead of introducing the entire Bootstrap library. - Custom theme : Customize themes using Bootstrap's Sass variables to reduce unnecessary style file sizes.
- Using React-Bootstrap : React-Bootstrap is a library of Bootstrap components designed specifically for React. It can help you use Bootstrap in React more easily while providing better performance and less DOM operations.
Here is an example using React-Bootstrap:
import React from 'react'; import { Button } from 'react-bootstrap'; function ReactBootstrapExample() { Return ( <Button variant="primary">Primary Button</Button> ); } export default ReactBootstrapExample;
In this example, we use React-Bootstrap's Button
component, which not only provides the style of Bootstrap, but also seamlessly integrates with React's component system.
Overall, Bootstrap's integration in React projects is a powerful and flexible tool that can help you quickly build a beautiful and responsive user interface. Through the introduction and examples of this article, you should have mastered the methods and techniques of using Bootstrap in React. Hope this knowledge works in your project and I wish you a happy development!
The above is the detailed content of Integrating Bootstrap Styles in React: Methods and Techniques. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics





How to build a real-time chat application using React and WebSocket Introduction: With the rapid development of the Internet, real-time communication has attracted more and more attention. Live chat apps have become an integral part of modern social and work life. This article will introduce how to build a simple real-time chat application using React and WebSocket, and provide specific code examples. 1. Technical preparation Before starting to build a real-time chat application, we need to prepare the following technologies and tools: React: one for building
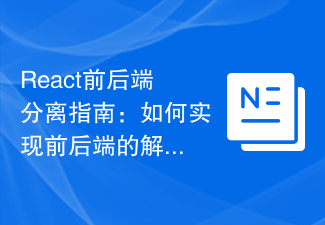
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
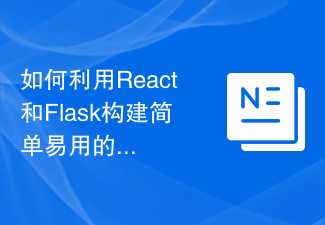
How to use React and Flask to build simple and easy-to-use web applications Introduction: With the development of the Internet, the needs of web applications are becoming more and more diverse and complex. In order to meet user requirements for ease of use and performance, it is becoming increasingly important to use modern technology stacks to build network applications. React and Flask are two very popular frameworks for front-end and back-end development, and they work well together to build simple and easy-to-use web applications. This article will detail how to leverage React and Flask
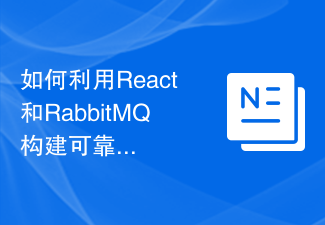
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
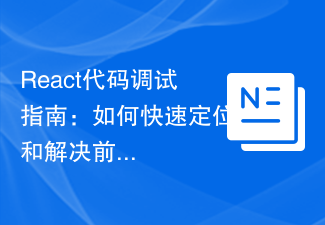
React code debugging guide: How to quickly locate and resolve front-end bugs Introduction: When developing React applications, you often encounter a variety of bugs that may crash the application or cause incorrect behavior. Therefore, mastering debugging skills is an essential ability for every React developer. This article will introduce some practical techniques for locating and solving front-end bugs, and provide specific code examples to help readers quickly locate and solve bugs in React applications. 1. Selection of debugging tools: In Re
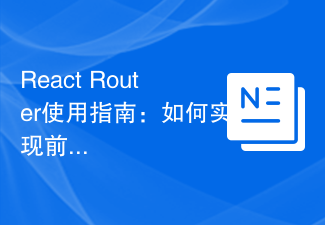
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
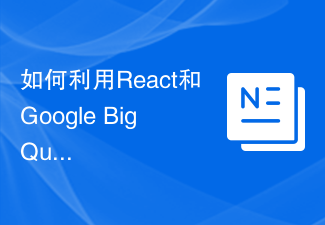
How to use React and Google BigQuery to build fast data analysis applications Introduction: In today's era of information explosion, data analysis has become an indispensable link in various industries. Among them, building fast and efficient data analysis applications has become the goal pursued by many companies and individuals. This article will introduce how to use React and Google BigQuery to build a fast data analysis application, and provide detailed code examples. 1. Overview React is a tool for building

How to use React and Apache Kafka to build real-time data processing applications Introduction: With the rise of big data and real-time data processing, building real-time data processing applications has become the pursuit of many developers. The combination of React, a popular front-end framework, and Apache Kafka, a high-performance distributed messaging system, can help us build real-time data processing applications. This article will introduce how to use React and Apache Kafka to build real-time data processing applications, and
