


C# and .NET: Understanding the Relationship Between the Two
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
introduction
In the world of programming, the two names C# and .NET are almost like shadows. When it comes to one, the other will always follow. As a programming master, today I will take you to deeply analyze the relationship between C# and .NET. Through this article, you will not only understand the close connection between them, but also master how to use this relationship to enhance your programming skills. Whether you are a beginner or an experienced developer, this article will provide you with unique perspectives and practical advice.
The relationship between C# and .NET can be described as "intrinsic", but they are not the same thing. C# is a programming language, while .NET is a development platform. The relationship between them is like the relationship between keys and locks. C# is the key to opening the door of .NET, but .NET functions are much more than that.
When I first came into contact with C# and .NET, I was deeply attracted by their strength and flexibility. C# has concise syntax and powerful functions, while .NET provides rich libraries and frameworks to help developers quickly build various types of applications. From desktop applications to web applications to mobile applications, .NET can do almost everything. Through this article, I hope to share my experience and experience over the years with you so that you can also feel the joy of programming.
Review of basic knowledge
C# is a modern programming language developed by Microsoft. It was designed to integrate seamlessly with the .NET framework. It inherits the syntax of C and C, and at the same time introduces many features of modern programming languages, such as garbage collection, type safety, etc.
.NET is a software framework developed by Microsoft. It provides a large number of class libraries and APIs to help developers build various types of applications. It is not only a runtime environment, but also a complete ecosystem, including a complete set of solutions from development tools to deployment platforms.
When I first started learning C#, I found that it has many similarities with Java, but the syntax of C# is more concise and more powerful. For example, the delegate and event system of C# allows me to handle asynchronous operations more flexibly, while the LINQ of .NET (Language Integrated Query) allows me to query and manipulate data in a more intuitive way.
Core concept or function analysis
Definition and function of C# and .NET
C# is an object-oriented programming language designed to be simple, modern, object-oriented and type-safe. It is one of the main programming languages in the .NET ecosystem for developing various types of applications.
.NET is a cross-platform development framework that provides rich class libraries and APIs to help developers build various types of applications. It not only supports C#, but also supports many other programming languages, such as F#, VB.NET, etc.
I remember in a project I developed a complex enterprise-level application using C# and .NET. The powerful type system of C# and the rich class library of .NET allow me to quickly build efficient and reliable applications. Especially the asynchronous programming model of .NET allows me to easily handle high concurrency scenarios.
How it works
When you write C# code, you are actually writing the intermediate language (IL) of .NET. When you compile C# code, the compiler converts your code to IL and then executes it by the .NET runtime (CLR). CLR is responsible for memory management, thread management, security checking and other tasks to ensure that your application can run efficiently and safely.
In actual development, I found that the garbage collection mechanism of .NET is a very powerful feature. It can automatically manage memory, avoiding the cumbersome and errors of manually managing memory. But at the same time, I also encountered some challenges, such as garbage collection may lead to temporary performance degradation in high concurrency scenarios. To solve this problem, I used the concurrent garbage collection feature provided by .NET, which significantly improved the performance of the application.
Example of usage
Basic usage
Let's look at a simple example of C# and .NET. This example shows how to write a simple console application using C# and use the .NET class library to read user input and output results.
using System; class Program { static void Main(string[] args) { Console.WriteLine("Please enter your name:"); string name = Console.ReadLine(); Console.WriteLine($"Hello, {name}!"); } }
This example shows the basic syntax of C# and the basic usage of .NET. Console.WriteLine
and Console.ReadLine
are .NET-provided class libraries for outputting and reading console input.
Advanced Usage
Now, let's look at a more complex example. This example shows how to use the asynchronous programming model of C# and .NET to handle high concurrency scenarios.
using System; using System.Threading.Tasks; class Program { static async Task Main(string[] args) { Console.WriteLine("Start asynchronous operation..."); await Task.Run(() => { for (int i = 0; i < 10; i ) { Console.WriteLine($"Async task is executing: {i}"); Task.Delay(1000).Wait(); } }); Console.WriteLine("Async operation is completed."); } }
This example shows the asynchronous programming model of C# and the task parallel library (TPL) of .NET. By using async
and await
keywords, we can easily write asynchronous code to improve the responsiveness and concurrency of our applications.
Common Errors and Debugging Tips
I've encountered some common mistakes and challenges when developing with C# and .NET. For example, type conversion errors, null reference exceptions, deadlocks in asynchronous programming, etc. To solve these problems, I have summarized some debugging tips:
- Debugging tools with Visual Studio: Visual Studio provides powerful debugging features that can help you quickly locate and resolve problems.
- Use logging: Adding logging in the code can help you track the execution process of the program and find out the problem.
- Using Unit Tests: Writing unit tests can help you discover and resolve potential problems in advance.
Performance optimization and best practices
Performance optimization and best practices are very important in actual development. Here are some performance optimization and best practices for C# and .NET that I summarized:
- Using asynchronous programming: Asynchronous programming can significantly improve application responsiveness and concurrency, especially in I/O-intensive operations.
- Using LINQ: LINQ can help you query and manipulate data in a more intuitive way, improving the readability and maintainability of your code.
- Using Cache: In data-intensive applications, using cache can significantly improve performance and reduce the number of database queries.
- Code refactoring: Regular refactoring of code can improve the readability and maintainability of the code and reduce potential errors.
On a large project, I used .NET's memory caching capability, which significantly improved the performance of the application. By caching frequently accessed data into memory, I reduce the number of database queries and improve the application's response speed.
In general, the relationship between C# and .NET is tight and complex. By gaining a deep understanding of their features and capabilities, you can better utilize them to build efficient and reliable applications. Hopefully this article provides you with some useful insights and suggestions to help you go further on the programming path of C# and .NET.
The above is the detailed content of C# and .NET: Understanding the Relationship Between the Two. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
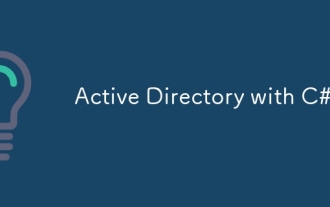
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
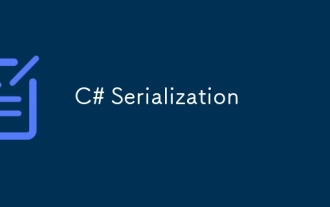
Guide to C# Serialization. Here we discuss the introduction, steps of C# serialization object, working, and example respectively.
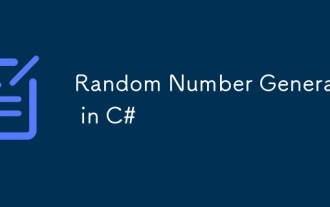
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
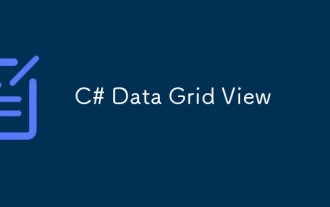
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
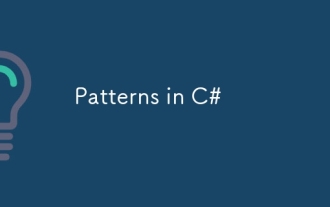
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
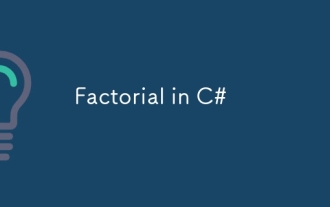
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
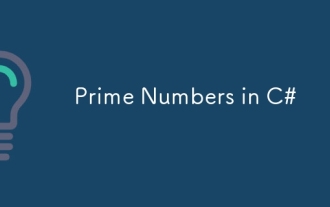
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
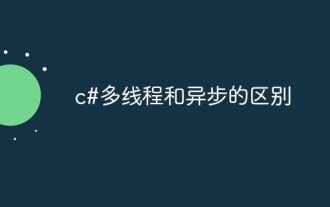
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
