Laravel (PHP) vs. Python: Weighing the Pros and Cons
Laravel is suitable for building web applications quickly, while Python is suitable for a wider range of application scenarios. 1. Laravel provides Eloquent ORM, Blade template engine and Artisan tools to simplify web development. 2. Python is known for its dynamic types, rich standard library and third-party ecosystem, and is suitable for web development, data science and other fields.
introduction
Laravel and Python often appear in our field of vision when we choose programming languages and frameworks. These two options have their own advantages and are suitable for different application scenarios and development needs. In this article, I will dig into the pros and cons of Laravel (PHP) and Python, hoping to help you make smarter choices. By reading this article, you will learn about the basics of the two, core features, practical application examples, and performance optimization strategies.
Review of basic knowledge
Laravel is a PHP-based web application framework designed to simplify the web development process. It provides rich functions, such as ORM (object relational mapping), routing, authentication systems, etc., allowing developers to quickly build complex web applications. In contrast, Python is a general programming language that is widely used in the fields of Web development, data science, artificial intelligence, etc. Python's concise syntax and powerful library ecosystem make it the first choice for many developers.
When choosing Laravel or Python, we need to consider the specific needs of the project. Laravel is more suitable for projects that focus on web development, while Python is suitable for a wider range of application scenarios.
Core concept or function analysis
Core features of Laravel
Laravel is known for its elegant syntax and rich feature library. Its core functions include:
Eloquent ORM : Laravel's Eloquent ORM provides a simple and intuitive way to interact with a database. It supports relational mapping, making it easy to handle complex data relationships.
Blade Template Engine : Blade is a powerful template engine that allows developers to embed PHP code into HTML, improving the readability and maintenance of the code.
Artisan Command Line Tools : Artisan provides a series of command line tools to help developers quickly generate code, manage database migration, etc.
// Use Eloquent ORM to define the model class User extends Model { protected $fillable = ['name', 'email', 'password']; }
Core features of Python
Python is known for its concise syntax and a powerful library ecosystem. Its core functions include:
Dynamic Type : Python is a dynamic typed language, which means that the type of a variable is determined only at runtime. This feature makes Python's code more flexible and easy to maintain.
Rich standard library : Python's standard library provides a wide range of functions, from file I/O to network programming.
Third-party library ecosystem : Python's third-party library ecosystem is very rich, such as web frameworks such as Django and Flask, as well as data science libraries such as NumPy and Pandas.
# Use Python's dynamic types and standard library def greet(name): return f"Hello, {name}!" print(greet("World"))
Example of usage
Basic usage of Laravel
Let's look at a simple Laravel example showing how to create a basic web application:
// Define route Route::get('/', function () { return view('welcome'); }); // Define the controller class UserController extends Controller { public function index() { $users = User::all(); return view('users.index', ['users' => $users]); } }
This example shows how to use Laravel's routing system and Eloquent ORM to create a simple web application.
Basic usage of Python
Here is a simple Python example showing how to create a web application using Flask:
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def home(): return render_template('home.html') if __name__ == '__main__': app.run(debug=True)
This example shows how to create a simple web application using the Flask framework.
Advanced Usage
Advanced usage of Laravel
Laravel's advanced features include queueing systems and event broadcasting. Let's look at an example using a queue:
// Define a task class ProcessPodcast implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; public function handle() { // Handle the logic of podcast} } // Send task ProcessPodcast::dispatch();
This example shows how to use Laravel's queue system to handle asynchronous tasks.
Advanced usage of Python
Advanced features of Python include asynchronous programming and decorator. Let's look at an example using asynchronous programming:
import asyncio async def fetch_data(): # Simulate a time-consuming operation await asyncio.sleep(2) return "Data fetched" async def main(): data = await fetch_data() print(data) asyncio.run(main())
This example shows how to use Python's asynchronous programming to handle concurrent tasks.
Common Errors and Debugging Tips
Common Errors in Laravel
Migration Error : An error may be encountered while performing a database migration. This is usually caused by syntax errors in the migration file or database connection issues. This can be solved by checking the migration file and database configuration.
Routing error : If the route is not defined correctly, it may result in a 404 error. This can be solved by checking routing files and controller methods.
Common Errors in Python
Indentation error : Python is very sensitive to indentation, indentation errors are a common problem. This can be solved by carefully checking the indentation of the code.
Type Error : Since Python is dynamically typed, you may encounter type errors. You can reduce such errors by adding type prompts and using type checking tools.
Performance optimization and best practices
Laravel's performance optimization
Laravel's performance optimization strategies include:
Using caching : Laravel provides a powerful caching system that can cache database query results, API responses, etc., significantly improving application performance.
Optimize database queries : By using Eloquent's query builder and index, database queries can be optimized and response time can be reduced.
// Use cache $value = Cache::remember('key', 3600, function () { return DB::table('users')->count(); });
Performance optimization of Python
Python's performance optimization strategies include:
Using PyPy : PyPy is a Python JIT compiler that can significantly increase the execution speed of Python code.
Using Cython : Cython can compile Python code into C code to improve performance.
# Optimize import cython with Cython @cython.cfunc def fibonacci(n: cython.int) -> cython.int: if n <= 1: Return n return fibonacci(n-1) fibonacci(n-2)
Best Practices
Whether using Laravel or Python, there are some common best practices:
Code readability : Whether it is PHP or Python, it is very important to keep the code readable. Using meaningful variable names, adding comments, and following code style guides (such as PSR-2 for PHP, PEP 8 for Python) can improve the maintainability of your code.
Test-driven development (TDD) : Using TDD can ensure the quality and reliability of the code. Laravel and Python have powerful testing frameworks, namely PHPUnit and pytest.
Continuous Integration and Deployment (CI/CD) : Use CI/CD tools to automate the testing and deployment process, improving development efficiency and code quality.
When choosing Laravel or Python, you need to consider the specific needs of the project, the team's technology stack, and future scalability. Laravel is suitable for building web applications quickly, while Python is suitable for a wider range of application scenarios. I hope this article can help you better understand the advantages and disadvantages of both and make the choice that suits you best.
The above is the detailed content of Laravel (PHP) vs. Python: Weighing the Pros and Cons. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1.Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2.Laravel is based on PHP, emphasizing the developer experience, and is suitable for small to medium-sized projects.
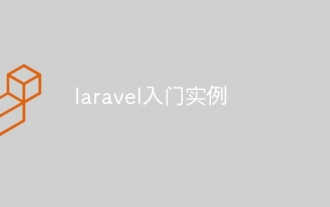
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.

LaravelisabackendframeworkbuiltonPHP,designedforwebapplicationdevelopment.Itfocusesonserver-sidelogic,databasemanagement,andapplicationstructure,andcanbeintegratedwithfrontendtechnologieslikeVue.jsorReactforfull-stackdevelopment.

PHP and Laravel are not directly comparable, because Laravel is a PHP-based framework. 1.PHP is suitable for small projects or rapid prototyping because it is simple and direct. 2. Laravel is suitable for large projects or efficient development because it provides rich functions and tools, but has a steep learning curve and may not be as good as pure PHP.
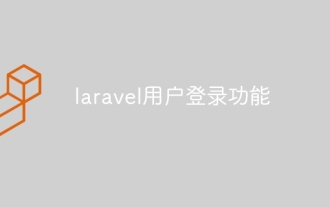
Laravel provides a comprehensive Auth framework for implementing user login functions, including: Defining user models (Eloquent model), creating login forms (Blade template engine), writing login controllers (inheriting Auth\LoginController), verifying login requests (Auth::attempt) Redirecting after login is successful (redirect) considering security factors: hash passwords, anti-CSRF protection, rate limiting and security headers. In addition, the Auth framework also provides functions such as resetting passwords, registering and verifying emails. For details, please refer to the Laravel documentation: https://laravel.com/doc
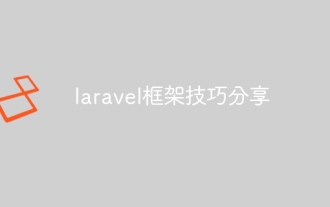
In this era of continuous technological advancement, mastering advanced frameworks is crucial for modern programmers. This article will help you improve your development skills by sharing little-known techniques in the Laravel framework. Known for its elegant syntax and a wide range of features, this article will dig into its powerful features and provide practical tips and tricks to help you create efficient and maintainable web applications.
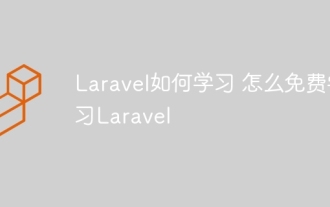
Want to learn the Laravel framework, but suffer from no resources or economic pressure? This article provides you with free learning of Laravel, teaching you how to use resources such as online platforms, documents and community forums to lay a solid foundation for your PHP development journey from getting started to master.
