


How does PHP handle object cloning (clone keyword) and the __clone magic method?
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance issues in cloning, and optimize cloning operations to improve efficiency.
introduction
In PHP, object cloning is a very powerful feature that allows us to create a copy of an object, not just referencing the original object. This is useful for situations where an independent operation of object instances is required, such as copying characters in game development, or backing up data states in data processing. Today we will discuss the clone
keywords and __clone
magic methods in PHP. Through actual code examples and sharing of experience, we will help everyone better understand and use these functions.
After reading this article, you will learn how to use the clone
keyword to create copy of objects, how to customize cloning behavior through the __clone
magic method, and how to avoid common cloning traps in actual projects.
Review of basic knowledge
In PHP, objects are instances of classes, and each object has its own properties and methods. Typically, when we assign an object to another variable, we are actually just passing a reference instead of creating a new copy of the object. This is where the clone
keyword comes into play, it is able to really copy an object.
Before understanding the clone
keyword, we need to know the relationship between references and objects in PHP. A reference is similar to a pointer that points to an object in memory. If you do not use clone
, two variables may point to the same object, and modifying one of them will affect the other.
Core concept or function analysis
Definition and function of clone
keyword
The clone
keyword is used to create a shallow copy of an object. A shallow copy means that the cloned object will copy all properties of the original object, but if the properties themselves are object types, then those object properties are still references, not new objects.
for example:
class Person { public $name; public function __construct($name) { $this->name = $name; } } $original = new Person('Alice'); $cloned = clone $original; $cloned->name = 'Bob'; echo $original->name; // Output Alice echo $cloned->name; // Output Bob
In this example, $cloned
is a clone of $original
, modifying name
attribute of $cloned
will not affect $original
.
How __clone
magic method works
When you use the clone
keyword, PHP will automatically call the __clone
magic method (if the method is defined in the class). This method allows you to customize cloning behavior, especially when you need to do some extra processing on the cloned objects.
The __clone
method will be called after the cloning is complete, which means that you can modify the properties of the cloned object in this method, or set new object properties for the cloned object.
For example:
class Person { public $name; public $friend; public function __construct($name) { $this->name = $name; $this->friend = new Person('Friend'); } public function __clone() { // Make sure that the friend property is also cloned $this->friend = clone $this->friend; } } $original = new Person('Alice'); $cloned = clone $original; $cloned->friend->name = 'New Friend'; echo $original->friend->name; // Output Friend echo $cloned->friend->name; // Output New Friend
In this example, we ensure that friend
attribute is also cloned through the __clone
method, thus avoiding the problem of shallow copy.
Example of usage
Basic usage
The easiest way to use the clone
keyword directly to create a copy of an object:
$original = new stdClass(); $original->value = 42; $cloned = clone $original; $cloned->value = 100; echo $original->value; // Output 42 echo $cloned->value; // Output 100
Advanced Usage
In more complex scenarios, you may need to use the __clone
method to customize cloning behavior. For example, in a class with multiple object properties, you might want to make sure that all nested objects are cloned correctly:
class Address { public $street; public $city; public function __construct($street, $city) { $this->street = $street; $this->city = $city; } } class Person { public $name; public $address; public function __construct($name, $street, $city) { $this->name = $name; $this->address = new Address($street, $city); } public function __clone() { $this->address = clone $this->address; } } $original = new Person('Alice', '123 Main St', 'Wonderland'); $cloned = clone $original; $cloned->address->street = '456 Elm St'; echo $original->address->street; // Output 123 Main St echo $cloned->address->street; // Output 456 Elm St
Common Errors and Debugging Tips
There are some common pitfalls to be aware of when using clone
and __clone
:
Shallow copy problem : If your object contains other objects as properties, these properties will not be automatically cloned when cloned, but will still refer to the original object. You need to clone these properties manually in the
__clone
method.Circular reference : In complex object structures, circular references may occur (for example, two objects refer to each other) . This can lead to infinite recursion when cloning. You need to handle this situation carefully in the
__clone
method, which can usually be avoided by tagging the cloned object.Performance issues : Frequent use of
clone
can affect performance, especially when dealing with large objects or complex object structures. You need to evaluate whether the object is really needed, or if there are other more efficient alternatives.
Performance optimization and best practices
In practical applications, optimizing cloning operations can bring significant performance improvements. Here are some suggestions:
Avoid unnecessary cloning : Evaluate whether objects are really needed to be cloned, especially when dealing with large amounts of data. In some cases, the same functionality can be achieved in other ways without cloning.
Use shallow copy : If the object's properties do not require deep copy, using shallow copy can improve performance. Make sure you understand which attributes require deep copy and which do not.
Batch cloning : If you need to clone multiple objects, consider batch processing instead of cloning one by one, which can reduce the overhead of cloning operations.
Best practice : Keep the code concise and clear when writing the
__clone
method to make sure the cloning behavior is predictable. Also, add appropriate comments and documentation so that other developers can understand your cloning logic.
With these suggestions and practices, you can use clone
and __clone
more efficiently in your PHP project, avoiding common pitfalls and improving code maintainability and performance.
The above is the detailed content of How does PHP handle object cloning (clone keyword) and the __clone magic method?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
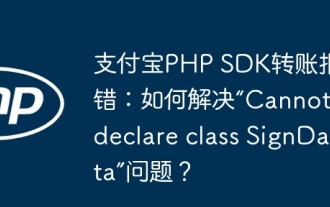
Alipay PHP...
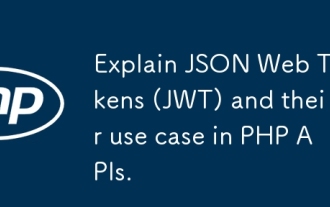
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
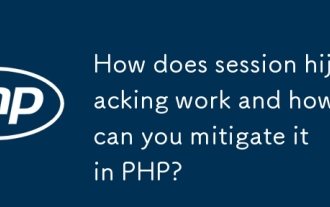
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
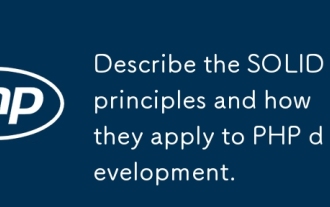
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
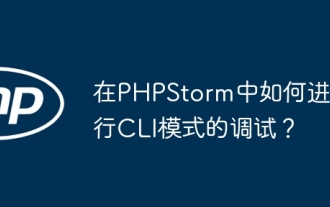
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...

The enumeration function in PHP8.1 enhances the clarity and type safety of the code by defining named constants. 1) Enumerations can be integers, strings or objects, improving code readability and type safety. 2) Enumeration is based on class and supports object-oriented features such as traversal and reflection. 3) Enumeration can be used for comparison and assignment to ensure type safety. 4) Enumeration supports adding methods to implement complex logic. 5) Strict type checking and error handling can avoid common errors. 6) Enumeration reduces magic value and improves maintainability, but pay attention to performance optimization.
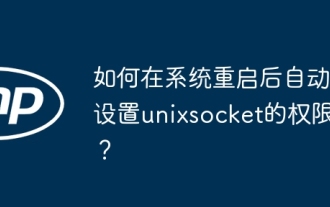
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
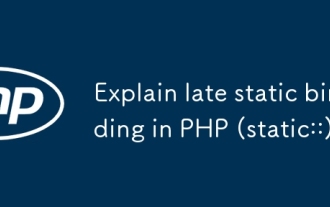
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
