


How does PHP type hinting work, including scalar types, return types, union types, and nullable types?
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP 7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type tip: Since PHP 8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
introduction
PHP type hinting is a powerful tool to improve code quality and readability. In this challenging and opportunity programming world, mastering type tips not only allows you to write more robust code, but also allows you to stand out from the team. Today, I'll take you into the depth of various aspects of PHP type hints, including scalar types, return types, union types, and nullable types. Through this article, you will not only understand these concepts, but also flexibly apply them in actual projects to avoid common pitfalls.
Review of basic knowledge
Before diving into the type prompt, let's review the type system in PHP. PHP is a weakly typed language, which means that the type of a variable can be changed dynamically at runtime. Although this brings flexibility to developers, it can also lead to type-related errors. To solve this problem, PHP introduced the type prompt function.
Type prompts allow developers to specify the expected type in function parameters and return values, which can help catch type errors and improve code reliability. PHP supports a variety of types, including objects, arrays, callable types, and scalar types introduced since PHP 7.0 (such as int, float, string, bool).
Core concept or function analysis
Scalar type prompts
Scalar type hints are an important feature introduced by PHP 7.0, allowing developers to specify basic data types in function parameters. Let's look at a simple example:
function add(int $a, int $b): int { return $a $b; }
In this example, the add
function takes two integer parameters and returns an integer result. If the incoming parameter types do not match, PHP will throw a TypeError
exception.
The advantage of scalar type hint is that it can catch type errors at compile time (or in strict mode) to avoid runtime errors. However, it should be noted that PHP's type prompt is loose by default, which means that in some cases PHP will try to convert the passed in value to the expected type. For example:
function greet(string $name): string { return "Hello, " . $name; } echo greet(123); // Output "Hello, 123"
In this example, although an integer is passed in, PHP converts it to a string. This may be useful in some cases, but in others it may lead to unexpected behavior. Therefore, when using scalar type hints, you need to carefully consider whether to enable strict mode ( declare(strict_types=1);
).
Return type prompt
Return type prompts allow developers to specify the return value type of the function, which is very important to ensure consistency of function behavior. Let's look at an example:
function divide(int $a, int $b): float { if ($b === 0) { throw new DivisionByZeroError("cannot be divided by zero"); } return $a / $b; }
In this example, the divide
function returns a floating point number. If the type returned by the function does not match, PHP will throw a TypeError
exception.
Return type prompts can not only improve the readability of the code, but also help developers discover potential problems during the code writing stage. However, it is important to note that return type hints may limit the flexibility of the function. For example, if a function needs to return a different type, it may be necessary to use a union type or a nullable type.
Union type prompts
Union type prompts are a new feature introduced in PHP 8.0, allowing developers to specify multiple types in function parameters or return values. Let's look at an example:
function process(mixed $value): int|float|string { if (is_int($value)) { return $value * 2; } elseif (is_float($value)) { return $value * 2.0; } else { return (string)$value; } }
In this example, process
function can accept any type of value and return an integer, floating point number, or string. The advantage of the union type prompt is that it provides more flexibility, but also requires developers to be more careful when using it, as it may mask type errors.
Nullable type prompts
Nullable type prompts allow developers to include null
values in type prompts, which is useful when dealing with functions that may return null values. Let's look at an example:
function findUser(?string $username): ?User { if ($username === null) { return null; } // Find user logic return new User($username); }
In this example, the findUser
function can accept a string or null
value and return a User
object or null
. The advantage of nullable type prompts is that it clarifies the situation where the function may return null
, but it also requires developers to carefully handle null
values when using them to avoid null pointer exceptions.
Example of usage
Basic usage
Let's look at a simple example showing how to use type tips in daily development:
class UserService { public function getUser(int $id): ?User { // Find user logic return new User($id, 'John Doe'); } } $userService = new UserService(); $user = $userService->getUser(1); if ($user !== null) { echo $user->getName(); // Output "John Doe" }
In this example, we use scalar type prompts, return type prompts, and nullable type prompts to ensure the correctness and readability of the getUser
function.
Advanced Usage
In more complex scenarios, type prompts can be used in conjunction with other PHP features. For example, using union types and generics can create more flexible and type-safe code:
function processArray(array $items): array { return array_map(function($item): int|float|string { if (is_int($item)) { return $item * 2; } elseif (is_float($item)) { return $item * 2.0; } else { return (string)$item; } }, $items); } $result = processArray([1, 2.5, 'hello']); print_r($result); // Output Array ( [0] => 2 [1] => 5 [2] => hello )
In this example, we use union type hints to handle different types in the array and use array_map
function to handle each element in the array.
Common Errors and Debugging Tips
When using type prompts, developers may encounter some common mistakes and misunderstandings. For example:
- Type mismatch error : PHP will throw a
TypeError
exception when the incoming parameter types do not match. This error can be avoided by enabling strict mode. - Return value type error : PHP will also throw a
TypeError
exception when the value type returned by the function does not match. This error can be avoided by adding type checking in the function. - Union type misuse : If you are not careful when using union types, type errors may be masked. This problem can be avoided by adding more type checks to the code.
When debugging these errors, you can use PHP's error reporting function to view detailed error information. In addition, debugging tools such as Xdebug can be used to track the execution process of the code and find out the source of type errors.
Performance optimization and best practices
There are some performance optimizations and best practices to note when using type prompts:
- Enable Strict Mode : In Strict Mode, PHP strictly checks for type matching, which can help catch more type errors, but may have a slight impact on performance.
- Avoid overuse of union types : While union types provide more flexibility, in some cases it may affect the readability and type safety of your code. Therefore, joint types should be used with caution and add more type checks if necessary.
- Use nullable type tips : When dealing with functions that may return null values, using nullable type tips can clarify the behavior of the function and avoid null pointer exceptions.
In actual projects, type prompts not only improve the reliability and readability of the code, but also help team members better understand the intent and behavior of the code. By using type prompts reasonably, developers can write more robust and easier to maintain code, thereby improving the overall quality of the project.
In short, PHP type prompts are a powerful tool that can help developers write higher quality code. When using type prompts, you need to pay attention to their advantages and disadvantages and flexibly apply them in accordance with actual project requirements. I hope this article can provide you with valuable insights and practical guidance to help you go further on the road of PHP development.
The above is the detailed content of How does PHP type hinting work, including scalar types, return types, union types, and nullable types?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
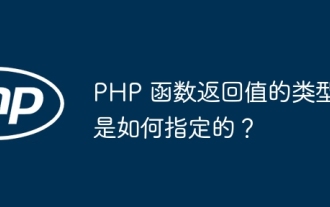
The type of function return value in PHP can be specified through type hints, including the following steps: Use a colon (:) after the function declaration. Specify the expected return type. PHP supports built-in types and custom types. Type hints improve code readability, maintainability, and testability.

Example of new features in PHP8: How to use type declarations and code to strengthen data validation? Introduction: With the release of PHP8, developers have welcomed a series of new features and improvements. One of the most exciting is the ability for type declarations and code to enforce data validation. This article will take some practical examples to introduce how to use these new features to strengthen data validation and improve code readability and maintainability. Advantages of type declaration: Before PHP7, the type of variables could be changed at will, which brought great difficulties to data verification.
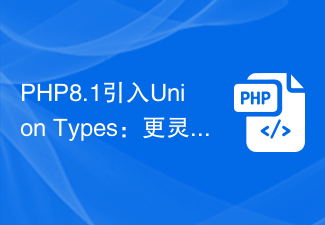
PHP8.1 introduces UnionTypes: more flexible type declarations Introduction: During the development process, type declarations are a very important feature that can help developers reduce errors and improve code readability. As a dynamically typed language, PHP had relatively weak support for type declarations in past versions. However, in PHP8.1 version, UnionTypes was introduced, bringing developers more flexible and powerful type declaration capabilities. 1. What are UnionTypes? in PHP
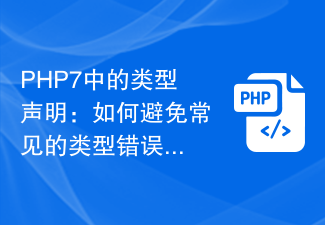
Strict type declarations were introduced in PHP7, which is an important improvement that can help developers catch type errors earlier in the development process and reduce bugs caused by type errors. This article will introduce type declarations in PHP7 and how to avoid common type errors. 1. Introduction to type declarations In PHP7, we can use type declarations to specify the types of function parameters and return values. Type declarations have the following forms: scalar type declaration a.int: integer type b.float: floating point type c.str
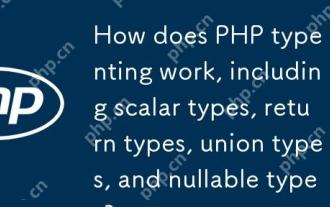
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
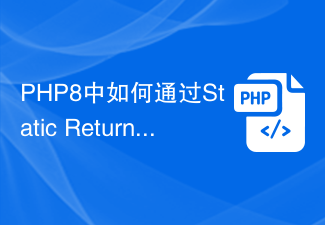
As an object-oriented scripting language, PHP8 provides many new features and improvements. One of the important changes is the enhancement of type declarations, especially the return type declaration of static methods. In this article, we will explore how to use the new feature of PHP8 - StaticReturnType (static return type) to better declare the return type of static methods and provide specific code examples. In past versions of PHP, we could specify the return value of a function or method using a return type declaration
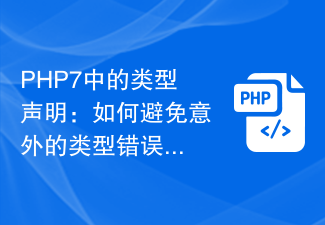
Type declarations in PHP7: how to avoid unexpected type errors? Introduction: During the development process, type errors are one of the common causes of program malfunctions and runtime errors. To solve this problem, PHP7 introduced the feature of type declaration. This article will provide an in-depth introduction to the use of type declarations in PHP7 and how to avoid unexpected type errors through type declarations. 1. Overview of type declaration The type declaration mechanism refers to declaring the expected data type on the parameters of a function or method. In PHP, type declaration
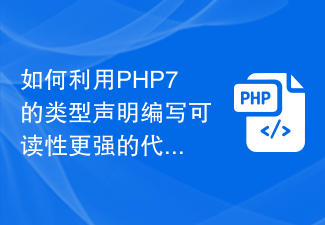
How to use PHP7's type declarations to write more readable code? With the release of PHP7, type declaration has become an important feature of PHP. Type declarations allow us to explicitly specify the data types of input parameters and return values in functions and methods. This can effectively improve the readability and robustness of your code. In this article, we’ll cover how to use PHP7’s type declarations to write more readable code, and provide concrete code examples. The parameter types of functions and methods are declared in PHP7. We can
