Using requestAnimationFrame with React Hooks
Animating with requestAnimationFrame
should be simple, but if you don't read React's documentation carefully, you may have some headaches. Here are three "trap" moments I have experienced personally.
In short: use the empty array as the second parameter of useEffect
to avoid it running multiple times; pass the function to the setter function of the state to ensure that the correct state is always available; useRef
to store things like timestamps and request IDs.
useRef
is not only used for DOM references
There are three ways to store variables in a function component:
- We can define a simple
const
orlet
whose value will always be reinitialized every time the component is re-rendered. - We can use
useState
, whose value remains unchanged in re-rendering, and if we change it, it also triggers re-rendering. - We can use
useRef
.
useRef
hook is mainly used to access the DOM, but it's more than just this one. It is a mutable object that holds a value in multiple re-renders. It's very similar to useState
hook, except that you can read and write its value through its .current
property, and changing its value won't re-render the component .
For example, the following example always displays <samp>5</samp>
even if the component is re-rendered by its parent component.
function Component() { let variable = 5; setTimeout(() => { variable = variable 3; }, 100) Return<div> {variable}</div> }
...and this example will increase the number by three and continue to re-render even if the parent component has not changed.
function Component() { const [variable, setVariable] = React.useState(5); setTimeout(() => { setVariable(variable 3); }, 100) Return<div> {variable}</div> }
Finally, this example returns five and will not be re-rendered. However, if the parent component triggers re-rendering, it will have an increased value each time (assuming re-rendering happens after 100 milliseconds).
function Component() { const variable = React.useRef(5); setTimeout(() => { variable.current = variable.current 3; }, 100) Return<div> {variable.current}</div> }
If we have variable values that we want to remember in the next or later rendering, and we don't want them to trigger re-rendering when they change, then we should use useRef
. In our example, we need to constantly change request animation frame IDs during cleaning, and if we animate based on the elapsed time between cycles, then we need to remember the timestamp of the previous animation. These two variables should be stored as references.
Side effects of useEffect
We can use the useEffect
hook to initialize and clean our request, although we want to make sure it runs only once; otherwise, it will eventually create, cancel and recreate the animation frame request every time it renders. Here is a valid, but bad example:
function App() { const [state, setState] = React.useState(0) const requestRef = React.useRef() const animate = time => { // Change the status according to the animation requestRef.current = requestAnimationFrame(animate); } // Don't do this React.useEffect(() => { requestRef.current = requestAnimationFrame(animate); return () => cancelAnimationFrame(requestRef.current); }); Return<div> {state}</div> ; }
Why not? If you run this code, useEffect
will trigger the animate
function, which will change the state and request a new animation frame. Sounds good, except that the state change will re-render the component by running the entire function again (including canceling the request made by the animate
function in the previous cycle as a cleanup and then starting the new requested useEffect
hook). This eventually replaces the request made by animate
function, which is completely unnecessary. We can avoid this by not starting a new request in the animate
function, but this is still not very good. It still leaves unnecessary cleanups in each round, and if the component re-renders for other reasons—such as the parent component re-renders it or other state has been changed—the unnecessary cancellation and request re-creation will still happen. A better pattern is to initialize the requests only once, keep them rotated through animate
function, and then clean up once when the component is unloaded.
To ensure that useEffect
hook is run only once, we can pass an empty array to it as a second parameter. However, passing an empty array has a side effect, which prevents us from getting the correct state during the animation process. The second parameter is a list of the changed values that the effect needs to respond to. We don't want to react to anything - we just want to initialize the animation - so we have an empty array. But React will interpret it as this means that this effect doesn't have to be up to date with the status. This includes the animate
function, as it is initially called from the effect. As a result, if we try to get the value of the state in the animate
function, it will always be the initial value. If we want to change the state based on its previous value and elapsed time, then it may not work.
function App() { const [state, setState] = React.useState(0) const requestRef = React.useRef() const animate = time => { // The "state" here will always be the initial value requestRef.current = requestAnimationFrame(animate); } React.useEffect(() => { requestRef.current = requestAnimationFrame(animate); return () => cancelAnimationFrame(requestRef.current); }, []); // Make sure the effect is only run once.<div> {state}</div> ; }
The setter function of the state also accepts the function
Even if the useEffect
hook locks our state to its initial value, there is a way to use our latest state. The setter function of useState
hook can also accept a function. So instead of passing values based on the current state like you do most of the time:
setState(state delta)
…You can also pass a function that receives the previous value as a parameter. And, yes, this returns the correct value even in our case:
setState(prevState => prevState delta)
Put everything together
Here is a simple example to summarize everything. We'll put all the above together and create a counter that counts to 100 and start over from the beginning. We want to persist and mutate without rerendering the entire component's technical variables using useRef
storage. We ensure that useEffect
only runs once by taking the empty array as its second parameter. We mutate the state by passing a function to the setter of useState
to ensure we always have the correct state.
Update: Go further with custom hooks
Once the basics are clear, we can also use hooks for metaprogramming by extracting most of the logic into a custom hook. This will have two benefits:
- It greatly simplifies our components, hiding technical variables that are related to animation but not related to our main logic.
- Custom hooks are reusable. If you need animation in another component, you can also simply use it there.
Custom hooks may sound like a high-level topic at first, but in the end we just move a part of the code from the component to the function and then call that function in the component like any other function. By convention, the name of a custom hook should start with use
keyword, and the rules of the hook apply, but other than that, they are just simple functions that we can customize with input and may return something.
In our example, in order to create a common hook for requestAnimationFrame
, we can pass a callback function that our custom hook will call on each animation cycle. In this way, our main animation logic will remain in our components, but the components themselves will be more focused.
The above is the detailed content of Using requestAnimationFrame with React Hooks. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
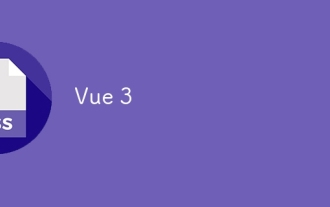
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
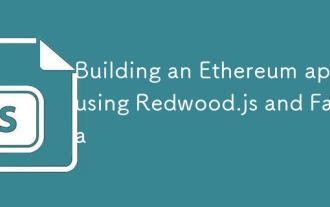
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
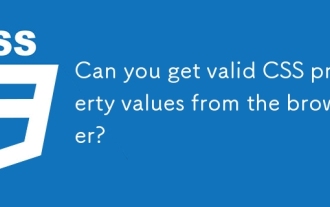
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
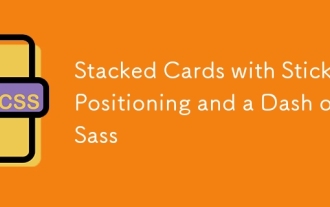
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
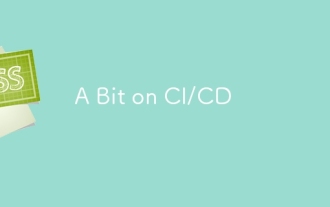
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
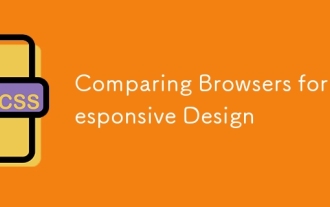
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
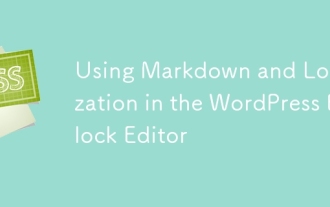
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
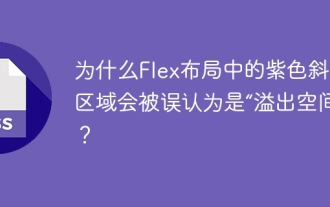
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
