Using Composer: Simplifying Package Management in PHP
Composer is a PHP dependency management tool that manages project dependencies through composer.json file. 1. Install Composer: Run several commands and move them to the global path. 2. Configure Composer: Create a composer.json file in the project root directory and run composer install. 3. Dependency management: Specify the library and its version through composer.json, and use semantic version number control. 4. Use Autoloading: Define the automatic loading rules of the class through the autoload field to simplify development. 5. Package management: supports private library management, and defines the private library address through the repositories field. 6. Performance optimization: Use the --no-dev flag to optimize the composer.json file, use composer update carefully, and set the cache size.
introduction
In the world of PHP development, Composer is like a magic wand that allows us to easily manage dependencies in our projects. You may have heard of Composer, but do you really understand how it simplifies our development process? This article will take you into the deep understanding of the mysteries of Composer. It will not only explain its basic usage, but also share some practical experience and optimization techniques to help you better utilize this powerful tool.
What is Composer?
Composer is a PHP dependency management tool that defines the libraries and versions required by the project through the composer.json
file, and then automatically downloads and manages these dependencies. It not only simplifies dependency management, but also resolves version conflicts and ensures compatibility of all dependencies in the project.
I remember when I first started using Composer, I was often overwhelmed by various version issues, but since I mastered its usage, my development efficiency has been a leap forward. Let's take a look at the specific usage of Composer and some practical tips.
Install and configure Composer
Installing Composer is very simple, just run a few commands in the terminal:
php -r "copy('https://getcomposer.org/installer', 'composer-setup.php');" php -r "if (hash_file('sha384', 'composer-setup.php') === 'e0ad994dedd97e12e0e91f4b3f084d460c7b712e018e34b442e15c5894e5bced') { echo 'Installer verified'; } else { echo 'Installer corrupt'; unlink('composer-setup.php'); } echo PHP_EOL;" php composer-setup.php php -r "unlink('composer-setup.php');"
After the installation is complete, you can move Composer to the global path for easy use:
sudo mv composer.phar /usr/local/bin/composer
When configuring Composer, you need to create a composer.json
file in the project root directory to define the project's dependencies:
{ "require": { "monolog/monolog": "1.0.*" } }
Then run the composer install
command, and Composer will automatically download and install the specified dependency package.
Dependency management and version control
One of the core functions of Composer is dependency management. Through the composer.json
file, you can specify the library and its version required for the project:
{ "require": { "php": "^7.2", "symfony/symfony": "3.4.*", "doctrine/orm": "^2.5", "twig/twig": "^2.0" } }
Version control here is implemented by semanticizing the version number. For example, ^7.2
means that the PHP version is 7.2 and above, but is lower than 8.0. This version control not only ensures the stability of the project's operation, but also automatically upgrades to the latest compatible version when the library is updated.
But it should be noted that version control may also bring some problems. For example, if you use the beta version of a certain library, subsequent stable versions may be incompatible with your code. Therefore, when selecting a version, careful evaluation is required to ensure that no unnecessary risks are introduced.
Simplify development with Autoloading
Another powerful feature of Composer is Autoloading. Through the autoload
field in the composer.json
file, you can define the automatic loading rules for the class:
{ "autoload": { "psr-4": { "App\\": "src/" } } }
In this way, when you need to use a certain class, just include the vendor/autoload.php
file, and Composer will automatically load the required classes for you, saving you the hassle of manually including the files.
In actual projects, I found that automatic loading not only improves development efficiency, but also greatly reduces redundancy in the code. Especially in large projects, automatic loading can significantly simplify the code structure and improve maintainability.
Package management and private libraries
Composer can not only manage public libraries, but also support the management of private libraries. You can define the address of a private library through repositories
field:
{ "repositories": [ { "type": "vcs", "url": "git@github.com:your-username/your-private-repo.git" } ], "require": { "your-username/your-private-repo": "dev-master" } }
The management of private libraries is especially important for enterprise-level projects because it protects sensitive code while taking advantage of Composer's dependency management capabilities. However, maintenance of private libraries requires more attention, because once problems arise, it may affect the stability of the entire project.
Performance optimization and best practices
When using Composer, there are some tips to help you optimize your project's performance and development process:
Use the
--no-dev
flag : In a production environment, you can usecomposer install --no-dev
command to avoid installing dependencies in the development environment, thereby reducing project size and improving loading speed.Optimize
composer.json
file : minimize unnecessary dependencies and avoid using too many wildcard version numbers, which can reduce the risk of version conflicts.Careful when using
composer update
: When updating dependencies, incompatible versions may be introduced. Therefore,composer update
should be used with caution in production environments. It is recommended to test first in development environments.Cache optimization : Composer supports caching, and the cache size can be set through
composer config -g -- cache-files-maxsize 1G
command to improve download speed.
In actual projects, I have encountered the problem of slow project startup due to too many dependency packages. Through the above optimization measures, the project startup time has been significantly reduced and the development efficiency has been improved.
Summarize
Composer is undoubtedly an indispensable tool for PHP developers. It not only simplifies dependency management, but also provides functions such as automatic loading and private library management. Through the introduction and shared practical experience in this article, I hope you can better master the skills of Composer and improve development efficiency and project quality. During use, remember to try more, summarize more, and find the best practices that suit you the most.
The above is the detailed content of Using Composer: Simplifying Package Management in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




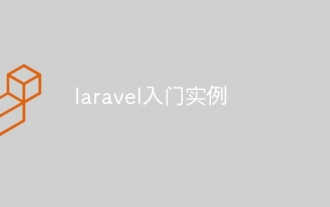
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
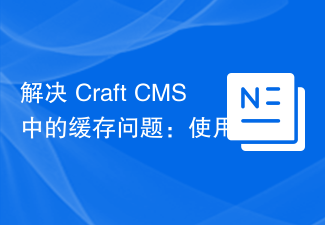
When developing websites using CraftCMS, you often encounter resource file caching problems, especially when you frequently update CSS and JavaScript files, old versions of files may still be cached by the browser, causing users to not see the latest changes in time. This problem not only affects the user experience, but also increases the difficulty of development and debugging. Recently, I encountered similar troubles in my project, and after some exploration, I found the plugin wiejeben/craft-laravel-mix, which perfectly solved my caching problem.
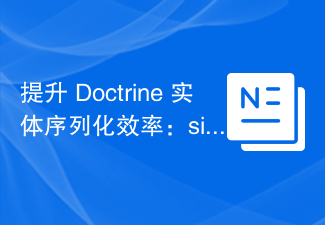
I had a tough problem when working on a project with a large number of Doctrine entities: Every time the entity is serialized and deserialized, the performance becomes very inefficient, resulting in a significant increase in system response time. I've tried multiple optimization methods, but it doesn't work well. Fortunately, by using sidus/doctrine-serializer-bundle, I successfully solved this problem, significantly improving the performance of the project.
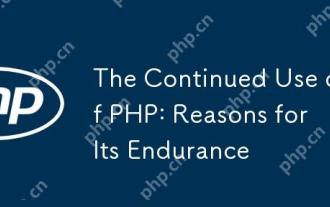
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
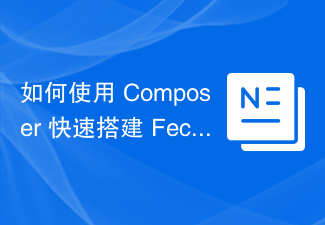
When developing an e-commerce platform, it is crucial to choose the right framework and tools. Recently, when I was trying to build a feature-rich e-commerce website, I encountered a difficult problem: how to quickly build a scalable and fully functional e-commerce platform. I tried multiple solutions and ended up choosing Fecmall's advanced project template (fecmall/fbbcbase-app-advanced). By using Composer, this process becomes very simple and efficient. Composer can be learned through the following address: Learning address
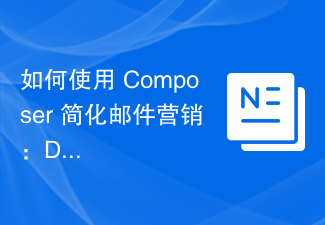
I'm having a tricky problem when doing a mail marketing campaign: how to efficiently create and send mail in HTML format. The traditional approach is to write code manually and send emails using an SMTP server, but this is not only time consuming, but also error-prone. After trying multiple solutions, I discovered DUWA.io, a simple and easy-to-use RESTAPI that helps me create and send HTML mail quickly. To further simplify the development process, I decided to use Composer to install and manage DUWA.io's PHP library - captaindoe/duwa.
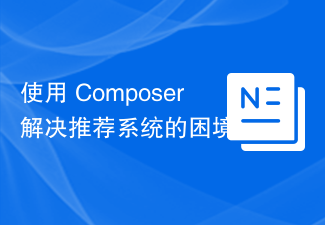
When developing an e-commerce website, I encountered a difficult problem: how to provide users with personalized product recommendations. Initially, I tried some simple recommendation algorithms, but the results were not ideal, and user satisfaction was also affected. In order to improve the accuracy and efficiency of the recommendation system, I decided to adopt a more professional solution. Finally, I installed andres-montanez/recommendations-bundle through Composer, which not only solved my problem, but also greatly improved the performance of the recommendation system. You can learn composer through the following address:
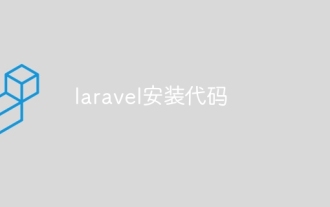
To install Laravel, follow these steps in sequence: Install Composer (for macOS/Linux and Windows) Install Laravel Installer Create a new project Start Service Access Application (URL: http://127.0.0.1:8000) Set up the database connection (if required)
