Python's Main Uses: A Comprehensive Overview
Python is widely used in data science, web development and automation scripting fields. 1) In data science, Python simplifies data processing and analysis through libraries such as NumPy and Pandas. 2) In web development, the Django and Flask frameworks enable developers to quickly build applications. 3) Python's simplicity and standard library make it ideal in automated scripts.
introduction
In the programming world, Python is like a Swiss army knife, with varied functions and wide applications. Have you ever wondered why Python shines in every field? This article will take you into the deep understanding of the main uses of Python and reveal its charm. Whether you are a beginner or an experienced developer, after reading this article, you will have a comprehensive understanding of the application areas of Python and be able to better utilize its advantages.
Review of basic knowledge
Python is an interpreted, advanced universal programming language first released by Guido van Rossum in the late 1980s. It is known for its concise syntax and easy-to-learn features, which makes Python particularly popular in the field of education. Python's standard library is very rich, covering a variety of functions from file operations to network programming, which allows developers to quickly build various applications.
If you have a certain understanding of the basic syntax and concepts of Python, then you will find how widely it is used in the fields of data processing, network development, scientific computing, etc.
Core concept or function analysis
Python's application in data science and machine learning
Python's application in the fields of data science and machine learning can be said to be like a fish in water. Its ecosystem contains powerful libraries such as NumPy, Pandas, Matplotlib, etc., which greatly simplify the process of data processing and analysis. Meanwhile, machine learning frameworks such as Scikit-learn and TensorFlow allow developers to easily build and train models.
For example, use Pandas for data processing:
import pandas as pd # Read CSV file data = pd.read_csv('data.csv') # View the first few lines of data print(data.head()) # Conduct simple statistics on data print(data.describe())
This simple and powerful data processing capability makes Python the first tool of choice for data scientists.
Python application in web development
Python also occupies a place in the field of web development. Web frameworks such as Django and Flask allow developers to quickly build web applications. Django provides a "batteries included" philosophy that includes everything from ORM to management backend, while Flask is known for its lightweight and flexibility, suitable for building small to medium-sized web applications.
For example, a simple Flask application:
from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!' if __name__ == '__main__': app.run(debug=True)
This concise syntax and powerful functions make Python shine in web development.
Python application in automation and scripting
Python's simplicity and ease of use make it ideal for automation and scripting. Whether the system administrator needs to write automated scripts or the developers need to conduct rapid prototype development, Python is competent. Its standard library includes modules such as os and shutil, which facilitates file and directory operations.
For example, a simple automation script:
import os import shutil # Create a new directory os.mkdir('new_directory') # Copy the file to the new directory shutil.copy('source_file.txt', 'new_directory/')
This simple and powerful scripting ability makes Python popular in the field of automation.
Example of usage
Applications in data science
In data science, Python is widely used. For example, use Scikit-learn for machine learning modeling:
from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import accuracy_score # Suppose we already have feature X and tag y X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Initialize and train the model model = RandomForestClassifier(n_estimators=100, random_state=42) model.fit(X_train, y_train) # Make predictions y_pred = model.predict(X_test) # Calculate accuracy = accuracy_score(y_test, y_pred) print(f'model accuracy: {accuracy}')
This example shows how to use Python for data segmentation, model training and evaluation, reflecting Python's powerful capabilities in data science.
Applications in Web Development
In web development, Python is also widely used. For example, build a simple blog system using Django:
from django.db import models from django.utils import timezone class Post(models.Model): title = models.CharField(max_length=200) content = models.TextField() created_date = models.DateTimeField(default=timezone.now) def __str__(self): return self.title
This example shows how to define a model using Django's ORM, reflecting the simplicity and power of Python in web development.
Applications in automated scripts
Python is also excellent in automated scripts. For example, write a simple backup script in Python:
import os import shutil import datetime # Define source and target directory source_dir = '/path/to/source' backup_dir = '/path/to/backup' # Create backup directory backup_path = os.path.join(backup_dir, datetime.datetime.now().strftime('%Y%m%d_%H%M%S')) os.makedirs(backup_path, exist_ok=True) # traverse the source directory and copy the file for root, dirs, files in os.walk(source_dir): for file in files: source_file = os.path.join(root, file) relative_path = os.path.relpath(source_file, source_dir) target_file = os.path.join(backup_path, relative_path) os.makedirs(os.path.dirname(target_file), exist_ok=True) shutil.copy2(source_file, target_file) print(f'backup is completed, stored in {backup_path}')
This example shows how to use Python for file backup, reflecting the simplicity and power of Python in automated scripts.
Performance optimization and best practices
Performance optimization
Performance optimization is a concern when using Python. Here are some optimization suggestions:
- Use list comprehensions instead of loops : list comprehensions are usually faster when working with small datasets. For example:
# Slow squares = [] for i in range(1000): squares.append(i**2) # Fast squares = [i**2 for i in range(1000)]
- Numerical calculations using NumPy : NumPy is much faster than pure Python when dealing with large arrays. For example:
import numpy as np # Slow a = range(1000000) b = range(1000000) c = [a[i] b[i] for i in range(len(a))] # Fast a = np.arange(1000000) b = np.arange(1000000) c = ab
Best Practices
In Python programming, following some best practices can improve the readability and maintenance of your code:
- Style Guide to Using PEP 8 : PEP 8 is the official style guide for Python, following it can make the code more readable. For example:
# Good practice def function_name(parameter): """Function description""" if parameter > 0: return parameter * 2 else: Return parameter # Bad practice def function_name(parameter):return parameter*2 if parameter>0 else parameter
- Using Virtual Environment : Virtual Environment can isolate project dependencies and avoid version conflicts. For example:
# Create a virtual environment python -m venv myenv # Activate the virtual environment source myenv/bin/activate # myenv\Scripts\activate on Unix systems # Install dependency pip install package_name
- Writing tests : Writing unit tests ensures the correctness of the code. For example:
import unittest def add(a, b): return ab class TestAddFunction(unittest.TestCase): def test_add_positive_numbers(self): self.assertEqual(add(2, 3), 5) def test_add_negative_numbers(self): self.assertEqual(add(-2, -3), -5) if __name__ == '__main__': unittest.main()
Through these optimizations and best practices, you can better leverage the advantages of Python, improve development efficiency and code quality.
In short, Python's diversity and powerful capabilities make it shine in fields such as data science, web development, and automated scripting. Whether you are a beginner or an experienced developer, mastering the main uses of Python will help you better deal with various programming challenges.
The above is the detailed content of Python's Main Uses: A Comprehensive Overview. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
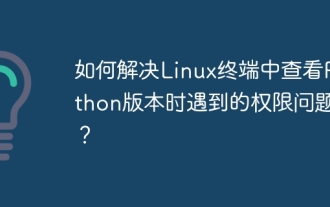
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
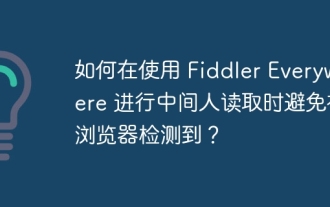
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
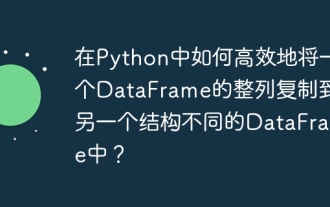
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
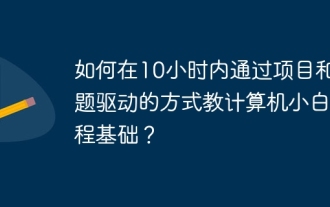
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
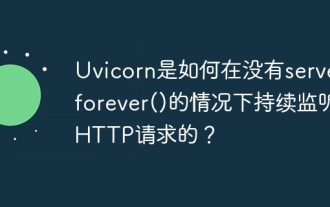
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
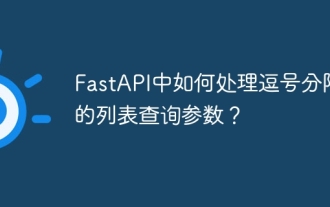
Fastapi ...
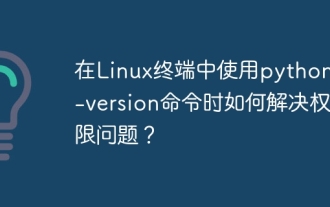
Using python in Linux terminal...
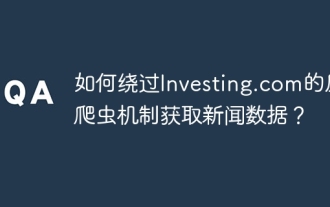
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
