Using React with HTML: Rendering Components and Data
Using HTML to render components and data in React can be achieved through the following steps: Using JSX syntax: React uses JSX syntax to embed HTML structures into JavaScript code, and operates the DOM after compilation. Components are combined with HTML: React components pass data through props and dynamically generate HTML content, such as
introduction
In modern front-end development, React has become an indispensable tool. It not only simplifies the construction of UI, but also greatly improves development efficiency. What we are going to explore today is how to use HTML in React to render components and data. Through this article, you will learn how to seamlessly combine React components with HTML, understand data flow management, and how to apply this knowledge in real-world projects.
Review of basic knowledge
React is a JavaScript library for building user interfaces, which manages the UI in a componentized way. HTML is the skeleton of a web page, defining the structure and content of a web page. Using React with HTML allows us to take advantage of the power of React while keeping HTML intuitive and readable.
In React, we usually use JSX syntax, which is an extension of JavaScript that allows us to write HTML structures directly in JavaScript code. JSX will eventually be compiled into plain JavaScript, allowing React to operate the DOM efficiently.
Core concept or function analysis
The combination of React components and HTML
React components can be regarded as extensions of HTML elements, which can contain HTML tags and pass data through props. In this way, we can generate HTML content dynamically.
function Greeting(props) { return <h1 id="Hello-props-name">Hello, {props.name}!</h1>; } ReactDOM.render( <Greeting name="World" />, document.getElementById('root') );
In this example, the Greeting
component takes a name
attribute and inserts it into the <h1>
tag of the HTML. This method is not only concise, but also easy to understand and maintain.
React data flow
React's data flow is unidirectional, flowing from the parent component to the child component. Passing data through props ensures that the flow of data between components is predictable and controllable.
function App() { const name = "React User"; return <Greeting name={name} />; }
In this example, App
component passes name
as props to the Greeting
component. This one-way data stream design makes debugging and maintenance easier.
Example of usage
Basic usage
Let's look at a simple example of how to use HTML in React to render a list.
function List(props) { const items = props.items; Return ( <ul> {items.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> ); } const items = ['Apple', 'Banana', 'Cherry']; ReactDOM.render( <List items={items} />, document.getElementById('root') );
In this example, we use map
function to iterate over the items
array and generate a <li>
element for each item. key
attribute is used to help React identify each element in the list and improve rendering efficiency.
Advanced Usage
Now let's look at a more complex example showing how conditional rendering and event handling are used in React.
function TodoList(props) { const [todos, setTodos] = useState(props.todos); const [newTodo, setNewTodo] = useState(''); const addTodo = () => { if (newTodo.trim()) { setTodos([...todos, newTodo]); setNewTodo(''); } }; Return ( <div> <input type="text" value={newTodo} onChange={(e) => setNewTodo(e.target.value)} /> <button onClick={addTodo}>Add Todo</button> <ul> {todos.map((todo, index) => ( <li key={index}>{todo}</li> ))} </ul> </div> ); } const initialTodos = ['Learn React', 'Build a Todo App']; ReactDOM.render( <TodoList todos={initialTodos} />, document.getElementById('root') );
In this example, we use the useState
hook to manage state, implementing the function of dynamically adding Todo items. Conditional rendering and event processing make components more flexible and interactive.
Common Errors and Debugging Tips
Common errors when using React and HTML include:
- <li> Forgot to add key attributes : React may experience performance issues when updating the list if no unique key attribute is added to each element when rendering the list.<li> Incorrect props pass : Make sure that the props passed to the child component are of the correct type and format, otherwise it may cause rendering errors.<li> Error usage of event handler function : Ensure that event handler function handles event objects and state updates correctly.
Methods to debug these problems include:
- <li> Use React DevTools to check the props and status of the component.<li> Add logs in the console to help track data flow and event processing.<li> Use React's strict pattern to capture potential problems.
Performance optimization and best practices
In real projects, it is very important to optimize the performance of React applications and follow best practices. Here are some suggestions:
- <li> Use
React.memo
to optimize components : For pure function components, use React.memo
to avoid unnecessary re-rendering.const MyComponent = React.memo(function MyComponent(props) { // Component logic});
- <li> Avoid unnecessary re-rendering : Control component updates by
shouldComponentUpdate
or React.PureComponent
.class MyComponent extends React.PureComponent { // Component Logic}
-
<li>
Using Virtualization Technology : For long lists, virtualization technology (such as react-window
) can be used to improve performance.
Code readability and maintenance : Keep the component's single responsibility, avoid over-necking, and ensure the code readability and maintenance.
Through these methods and practices, we can build efficient and maintainable React applications. Hopefully this article helps you better understand how to use HTML in React to render components and data and apply this knowledge in real projects.
The above is the detailed content of Using React with HTML: Rendering Components and Data. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
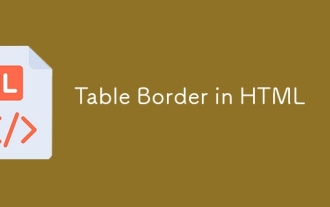
Guide to Table Border in HTML. Here we discuss multiple ways for defining table-border with examples of the Table Border in HTML.
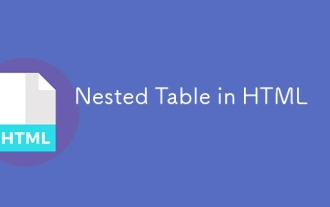
This is a guide to Nested Table in HTML. Here we discuss how to create a table within the table along with the respective examples.
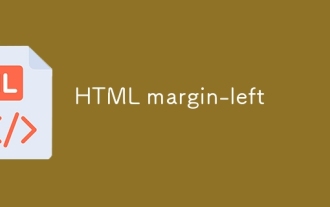
Guide to HTML margin-left. Here we discuss a brief overview on HTML margin-left and its Examples along with its Code Implementation.
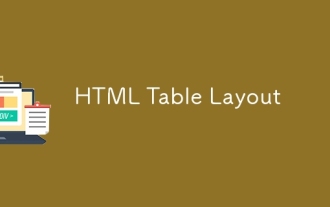
Guide to HTML Table Layout. Here we discuss the Values of HTML Table Layout along with the examples and outputs n detail.
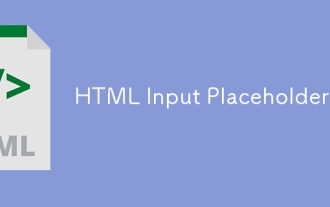
Guide to HTML Input Placeholder. Here we discuss the Examples of HTML Input Placeholder along with the codes and outputs.
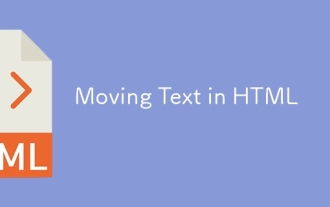
Guide to Moving Text in HTML. Here we discuss an introduction, how marquee tag work with syntax and examples to implement.
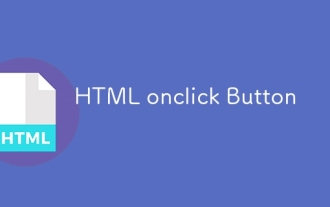
Guide to HTML onclick Button. Here we discuss their introduction, working, examples and onclick Event in various events respectively.
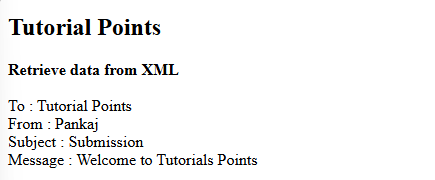
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
