


When the front-end passes data to the back-end, the back-end displays that the obtained data is NULL. How to solve it?
Problem Description: During the development process of using the Ruoyi separate framework, the front-end uses POST request to pass data to the back-end, but the back-end receives a NULL value.
Front-end code:
//Modify the order status export function updateorderstatus(id,status){ const data={ id, status } return request({ url:"/business/orderinfo/updatestate", method:"post", data:data }) }
Screenshot of front-end request data: (Screenshot of front-end request data should be included here)
Backend code (original code):
@ApiOperation("Order Management-Modify Order Status") @ApiImplicitParams({ @ApiImplicitParam(name="id", value = "primary key id", required = true, dataType = "integer"), @ApiImplicitParam(name="status", value = "Status 0 ends with 1", required = true, dataType = "integer") }) @PostMapping("/updatestate") public AjaxResult updateState(Integer id, Integer status) { System.out.println("Change Order Number: "id "\n"); System.out.println("Change order status: " status "\n"); // ... (Other code) }
Screenshot of backend printing results: (This should include screenshots of backend printing results, showing id and status as null)
Problem analysis: The backend uses Integer id, Integer status
to receive parameters. When processing POST requests, Spring Boot cannot correctly parse JSON data in the request body to these parameters by default.
Solution: Modify the backend code and bind the JSON data in the request body to an object using the @RequestBody
annotation.
Modified backend code:
@ApiOperation("Order Management-Modify Order Status") @PostMapping("/updatestate") public AjaxResult updateState(@RequestBody OrderStatusDTO orderStatusDTO) { System.out.println("Change Order Number:" orderStatusDTO.getId() "\n"); System.out.println("Change order status:" orderStatusDTO.getStatus() "\n"); if (orderStatusDTO.getId() == null) { return AjaxResult.error("enter primary key id"); } if (orderStatusDTO.getStatus() == null) { return AjaxResult.error("Input Status"); } BorderInfo borderInfo = new BorderInfo(); borderInfo.setId(orderStatusDTO.getId()); borderInfo.setStatus(orderStatusDTO.getStatus()); System.out.println("Change order status:" borderInfo.toString() "\n"); orderInfoService.update(borderInfo); return AjaxResult.success(); }
Added OrderStatusDTO class:
public class OrderStatusDTO { private Integer id; private Integer status; // getters and setters }
By using @RequestBody
annotation and creating an OrderStatusDTO
class to receive the JSON data passed by the front end, the backend can correctly parse the data to avoid the problem of NULL
values. Please make sure that the JSON data sent by the front end is consistent with the field name of OrderStatusDTO
. At the same time, check whether the front-end request
function has correctly set headers
, such as Content-Type: application/json
.
This solution is more in line with the design specifications of the RESTful API than the original @RequestParam
solution, and also processes the body data of POST requests more clearly.
The above is the detailed content of When the front-end passes data to the back-end, the back-end displays that the obtained data is NULL. How to solve it?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
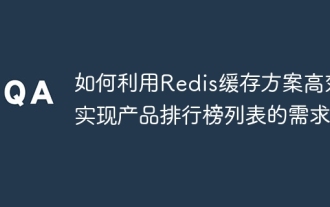
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
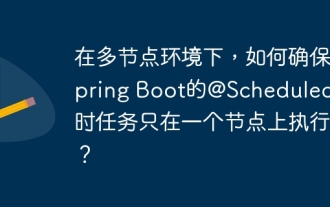
The optimization solution for SpringBoot timing tasks in a multi-node environment is developing Spring...
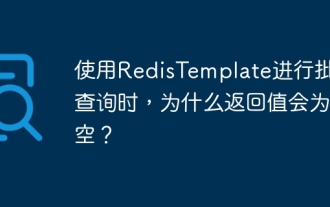
Why is the return value empty when using RedisTemplate for batch query? When using RedisTemplate for batch query operations, you may encounter the returned results...
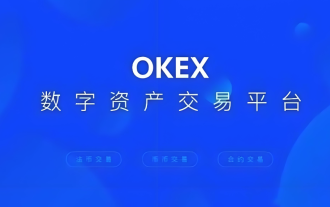
Ouyi OKX is the world's leading digital asset trading platform. 1) Its development history includes: it will be launched in 2017, the Chinese name "Ouyi" will be launched in 2021, and it will be renamed Ouyi OKX in 2022. 2) Core services include: trading services (coin, leverage, contracts, DEX, fiat currency trading) and financial services (Yubibao, DeFi mining, lending). 3) The platform's special functions include: market data services and risk control system. 4) Core advantages include: technical strength, security system, service support and market coverage.
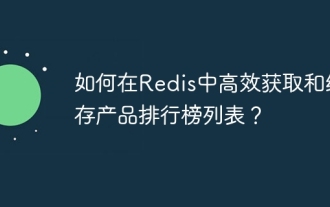
Redis Caching Solution: How to efficiently obtain product ranking list? During the development process, how to efficiently obtain product ranking lists is a common question...
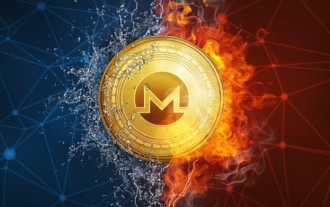
The time for recharge of digital currency varies depending on the method: 1. Bank transfer usually takes 1-3 working days; 2. Recharge of credit cards or third-party payment platforms within a few minutes to a few hours; 3. The time for recharge of digital currency transfer is usually 10 minutes to 1 hour based on the blockchain confirmation time, but it may be delayed due to factors such as network congestion.
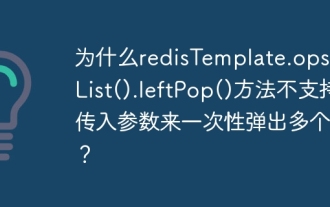
Regarding the reason why RedisTemplate.opsForList().leftPop() does not support passing numbers. When using Redis, many developers will encounter a problem: Why redisTempl...

The quantum chain (Qtum) transaction process includes three stages: preliminary preparation, purchase and sale. 1. Preparation: Select a compliant exchange, register an account, perform identity verification, and set up a wallet. 2. Purchase quantum chains: recharge funds, find trading pairs, place orders (market orders or limit orders), and confirm transactions. 3. Sell quantum chains: Enter the trading page, select the trading pair and order type (market order or limit order), confirm the transaction and withdraw cash.
