Demystifying C# .NET: An Overview for Beginners
C# is a modern, object-oriented programming language developed by Microsoft, and .NET is a development framework provided by Microsoft. C# combines the performance of C and the simplicity of Java, and is suitable for building various applications. The .NET framework supports multiple languages, provides garbage collection mechanisms, and simplifies memory management.
introduction
Explore the world of C# .NET, an overview designed for beginners. C# (pronounced as "C sharp") is a modern, object-oriented programming language developed by Microsoft, while .NET is a development framework provided by Microsoft. With this article, you will gain the basics of C# and .NET, understand their importance in modern software development, and how to start your programming journey.
Review of basic knowledge
C# language originated from Microsoft's .NET initiative to create a powerful language that can compete with Java. C# combines the performance of C and the simplicity of Java, providing rich libraries and frameworks, allowing developers to easily build various types of applications. The .NET framework is a platform for building and running applications. It supports a variety of programming languages, including C#, VB.NET, etc.
The core concepts of C# and .NET include classes, objects, inheritance, encapsulation, and polymorphism, which are the basic elements of object-oriented programming. In addition, .NET provides a garbage collection mechanism to help developers manage memory and reduce the burden of manual memory management.
Core concept or function analysis
Definition and function of C# language
C# is a statically typed, object-oriented language that provides powerful type security and object-oriented features. Its syntax is concise and easy to learn and use, and is especially suitable for building large enterprise-level applications. The role of C# is that it enables developers to write robust and maintainable code in an efficient way.
// Simple C# class definition public class HelloWorld { public static void Main(string[] args) { Console.WriteLine("Hello, World!"); } }
This simple example shows the basic syntax and structure of C#. Main
method is the entry point of the program, Console.WriteLine
is used to output text to the console.
How .NET frameworks work
The .NET framework is a collection of runtime environments and class libraries that allow developers to develop applications in multiple programming languages. At the heart of .NET is the Common Language Runtime (CLR), which manages code execution, memory allocation, and garbage collection. .NET also includes a huge class library called the base class library (BCL), which provides rich functions such as file I/O, network communication, database access, etc.
Another important component of the .NET framework is ASP.NET, which is used to build dynamic web applications. ASP.NET combines the powerful functions of C# and .NET frameworks, making web development more efficient and flexible.
Example of usage
Basic usage
Let's look at a simple C# program that shows how objects are created and used.
// Define a simple class public class Person { public string Name { get; set; } public int Age { get; set; } public void Introduction() { Console.WriteLine($"My name is {Name} and I am {Age} years old."); } } // Use this class public class Program { public static void Main() { Person person = new Person { Name = "Alice", Age = 30 }; person.Introduce(); // Output: My name is Alice and I am 30 years old. } }
This example shows how to define a class, create an object, and call a method. C#'s syntax is clear and easy to understand and use.
Advanced Usage
C# also supports many advanced features such as asynchronous programming, LINQ (language integration query), and lambda expressions. Let's look at an example using LINQ:
// Use LINQ to filter list using System; using System.Collections.Generic; using System.Linq; public class Program { public static void Main() { List<int> numbers = new List<int> { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; var evenNumbers = numbers.Where(n => n % 2 == 0); foreach (var number in evenNumbers) { Console.WriteLine(number); // Output: 2, 4, 6, 8, 10 } } }
LINQ allows developers to manipulate data collections in a declarative manner, greatly improving the readability and efficiency of the code.
Common Errors and Debugging Tips
One of the common mistakes that beginners make is forgetting access modifiers (such as public
, private
, etc.) to define methods in a class, which can lead to compilation errors. Another common problem is forgetting the properties of the initialization object, resulting in an empty reference exception.
When debugging C# programs, you can use Visual Studio's built-in debugger, which provides breakpoint settings, variable monitoring, and call stack viewing. These tools can help you quickly locate and resolve problems.
Performance optimization and best practices
Performance optimization is an important topic when using C# and .NET. Here are some suggestions:
- Use
using
statements to ensure that resources are released correctly, especially when processing files or database connections. - Try to avoid creating unnecessary objects in loops, as this will increase the burden of garbage collection.
- Use asynchronous programming to improve application responsiveness, especially when performing I/O operations.
In terms of best practice, it is crucial to keep the code readable and maintainable. Use meaningful variable names and method names to write clear comments, following code style guides (such as Microsoft's C# coding conventions). In addition, using the various tools and libraries provided by .NET can greatly improve development efficiency and code quality.
In-depth insights and thoughts
The ecosystem of C# and .NET is huge and constantly evolving, which means you need to constantly learn and adapt to new technologies and tools. The version of C# is updated frequently, and each new version brings new features and improvements, which is both a challenge and an opportunity. For beginners, after mastering the basics, they can gradually gain insight into more advanced features and best practices.
When choosing C# and .NET for development, the following points need to be considered:
- Platform Compatibility : The .NET core version supports cross-platform development, which means you can run your applications on Windows, Linux, and macOS.
- Community and Resources : C# and .NET have a huge community and abundant learning resources, which is a huge advantage for beginners.
- Performance and Scalability : C# and .NET provide powerful performance and scalability for building applications of all sizes.
However, C# and .NET also have some potential challenges:
- Learning curve : Although the syntax of C# is relatively simple, the .NET framework is huge, and learning and mastering all functions takes time and effort.
- Depend on Microsoft : While the .NET core version supports cross-platform, some advanced features may still depend on Windows, which may limit certain application scenarios.
Overall, C# and .NET are a powerful combination suitable for all types of software development. Whether you are a beginner or experienced developer, you can benefit from it. I hope this article will help you better understand C# and .NET and stimulate your interest in further exploration.
The above is the detailed content of Demystifying C# .NET: An Overview for Beginners. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




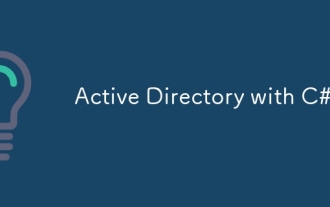
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
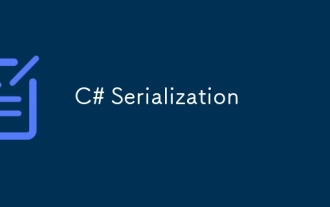
Guide to C# Serialization. Here we discuss the introduction, steps of C# serialization object, working, and example respectively.
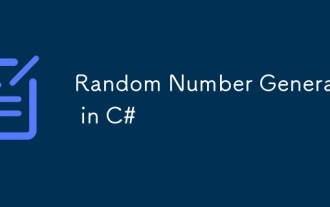
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
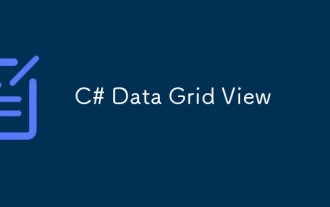
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
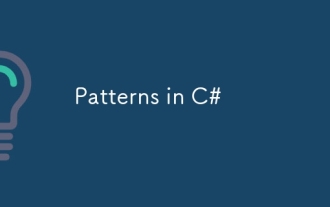
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
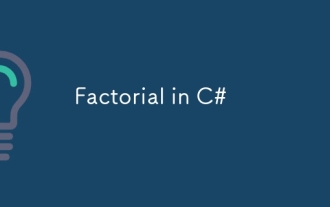
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
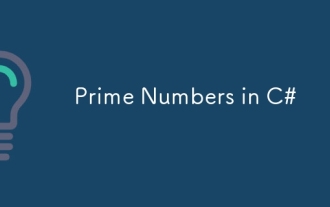
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
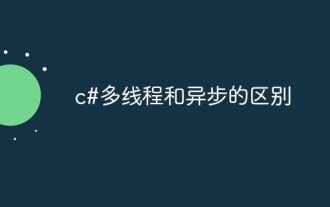
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
