How to Use the Web Share API
It seems to have been obscured since Chrome 61 for Android first launched its Web Sharing API. In fact, it provides a way to trigger a native sharing dialog for the device (the desktop if using Safari) when sharing content directly from a website or web application (such as a link or contact card).
While users can already share content from web pages in native ways, they must find the option in the browser menu, and even then, there is no control over the shared content. The introduction of this API allows developers to add sharing capabilities to applications or websites by leveraging native content sharing capabilities on user devices.
This method has many advantages over the traditional method:
- Users have a wider range of content sharing options than the limited number of options you may have in your DIY implementation.
- By canceling third-party scripts from various social platforms, you can reduce page loading time.
- You don't need to add a series of buttons to different social media sites and emails. The native sharing option of the device is triggered with just one button.
- Users can customize their preferred shared targets on their devices without being limited to predefined options.
Browser support instructions
Before we go into detail about how API works, let's solve the problem of browser support. To be honest, the browser support is not ideal at present. It only works with Chrome for Android and Safari (Desktop and iOS).
This browser supports data from Caniuse, which contains more details. The number indicates that the browser supports this feature in this version and later.
Desktop
Mobile/Tablet PC
But don't be discouraged by this, don't adopt this API on your website. As you will see, implementing a fallback to a browser that does not support it is very easy.
Some requirements for using API
Before you can adopt this API on your own web project, there are two things to note:
- Your website must be provided via HTTPS. For local development, the API works as well when your site runs on localhost.
- To prevent abuse, the API can only trigger in response to certain user actions, such as click events.
Example
To demonstrate how to use this API, I prepared a demo that works basically the same as my website. Here is how it looks:
Currently, after clicking the share button, a dialog box will pop up showing some options for sharing content. Here is the part of the code that helps us achieve this:
<code>shareButton.addEventListener('click', event => { shareDialog.classList.add('is-open'); });</code>
Let's move on to convert this example to using the Web Sharing API. The first thing to do is check whether the API is indeed supported by the user's browser, as shown below:
<code>if (navigator.share) { // 支持Web 共享API } else { // 回退}</code>
Using a Web Sharing API is as simple as calling navigator.share()
method and passing an object that contains at least one of the following fields:
-
url
: A string representing the URL to be shared. This is usually a document URL, but not necessarily. You can share any URL through the Web Sharing API. -
title
: A string representing the title to be shared, usuallydocument.title
. -
text
: Any text you want to include.
Here is what it looks like in actual application:
<code>shareButton.addEventListener('click', event => { if (navigator.share) { navigator.share({ title: 'WebShare API Demo', url: 'https://codepen.io/ayoisaiah/pen/YbNazJ' }).then(() => { console.log('Thanks for sharing!'); }) .catch(console.error); } else { // 回退} });</code>
At this point, once the share button is clicked in the supported browser, the native selector will pop up all possible targets that users can share data. The goal can be a social media app, email, instant messaging, text messages, or other registered shared target.
The API is based on Promise, so you can attach the .then()
method to display the shared successful message and use .catch()
to handle the error. In a real-life scenario, you may want to use the following code snippet to get the title and URL of the page:
<code>const title = document.title; const url = document.querySelector('link[rel=canonical]') ? document.querySelector('link[rel=canonical]').href : document.location.href;</code>
For URLs, we first check if the page has a canonical URL, and if so, use that URL. Otherwise, we get href
from document.location
.
It's a good idea to provide a fallback
In browsers that do not support the Web Sharing API, we need to provide a fallback mechanism so that users on these browsers can still get some sharing options.
In our case, we have a pop-up dialog with some options to share content, and the buttons in our demo aren't actually linked to anywhere, because, well, it's just a demo. However, if you want to know how to create your own link to share a webpage without a third-party script, Adam Coti’s article is a great place to start.
What we want to do is display a fallback dialog for users of browsers that do not support the Web Sharing API. It's as simple as moving the code that opens a shared dialog into else
block:
<code>shareButton.addEventListener('click', event => { if (navigator.share) { navigator.share({ title: 'WebShare API Demo', url: 'https://codepen.io/ayoisaiah/pen/YbNazJ' }).then(() => { console.log('Thanks for sharing!'); }) .catch(console.error); } else { shareDialog.classList.add('is-open'); } });</code>
Now, no matter what browser the user uses, all users are covered. Here is a comparison of how the share button behaves on two mobile browsers, one supports the Web Sharing API and the other does not:
Try it! Use browsers that support web sharing and unsupported browsers. It should be similar to the above demonstration.
Summarize
This covers almost all the basics you need to know about the Web Sharing API. By implementing it on your website, visitors can more easily share your content through various social networks, contacts, and other native applications.
It is also worth noting that if your web application meets the progressive web application installation criteria and is added to the user's home screen, you can add it as a shared target. This is a feature that you can read about the Web Share Target API on Google Developers.
Although browser support is jagged, fallbacks are easy to implement, so I don't think there is any reason more websites don't adopt it. If you want to learn more about this API, you can read the specification here.
Have you used the Web Sharing API? Please share your experience in the comments.
The above is the detailed content of How to Use the Web Share API. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




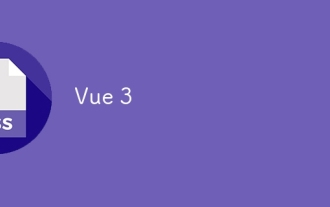
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
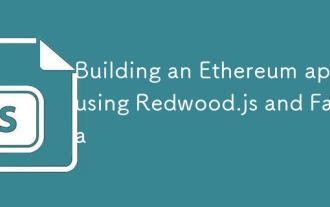
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
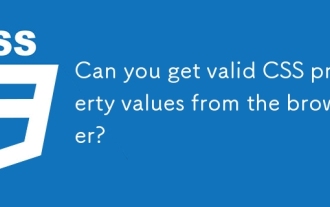
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
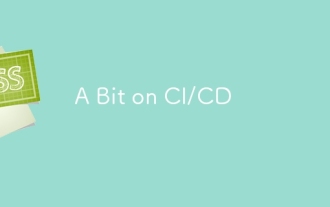
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
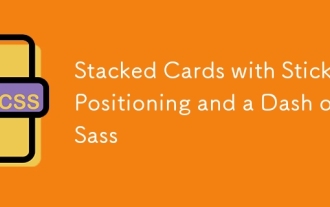
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
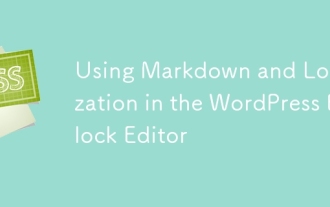
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
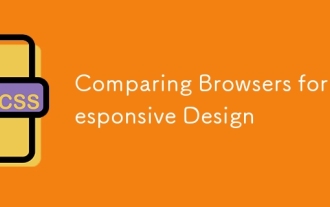
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
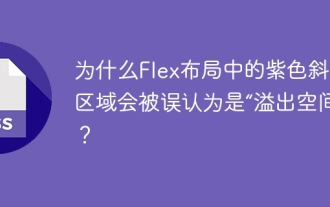
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
