How does the JVM handle multithreading on different operating systems?
Multithreading is important in modern programming because it can improve program responsiveness and resource utilization and handle complex concurrent tasks. JVM ensures the consistency and efficiency of multithreads on different operating systems through thread mapping, scheduling mechanism and synchronization lock mechanism.
introduction
Before exploring how JVM handles multithreading on different operating systems, let's first think about one question: Why is multithreading so important in modern programming? Multithreading can not only improve program responsiveness and resource utilization, but also handle complex concurrent tasks. However, while achieving these advantages, how can we ensure consistency and efficiency across different operating systems? This article will explore the cross-platform implementation of JVM in multi-threaded processing in depth, and will take you to fully understand this key technology from basic knowledge to practical applications.
Review of basic knowledge
Multithreaded programming is a key concept in modern software development, which allows programs to perform multiple tasks simultaneously, thereby improving performance and responsiveness. JVM (Java Virtual Machine) provides powerful multi-threading support as the running environment of Java programs. To understand how JVM handles multithreading, you need to first understand the threading model in Java and the thread management mechanism of the operating system.
Threads in Java are created through Thread
class or the implementation of the Runnable
interface. These threads run inside the JVM but ultimately rely on the thread implementation of the operating system. Different operating systems (such as Windows, Linux, and macOS) have different thread implementation methods, and the JVM needs to maintain consistency and efficiency in these differences.
Core concept or function analysis
Multithreaded model in JVM
The JVM's multi-threading model is based on the threading API of the Java language, and manages and controls threads through Thread
class and Runnable
interface. The JVM maintains a thread pool internally to manage and schedule these threads. No matter which operating system is on, the JVM is responsible for mapping Java threads to threads on the operating system.
How it works
On different operating systems, the JVM handles multithreading in the following ways:
- Thread mapping : The JVM maps Java threads to threads in the operating system. For example, on Linux, the JVM uses the pthread library to create and manage threads, while on Windows, it uses threading functions from the Windows API.
- Scheduling mechanism : There is a thread scheduler inside the JVM, which is responsible for managing the execution order and priority of threads. Although the operating system also has its own scheduling mechanism, the JVM scheduler will affect the actual execution order of threads to a certain extent.
- Synchronization and locking mechanism : The JVM provides
synchronized
keywords and various locking and synchronization tools in thejava.util.concurrent
package. The consistency of these tools on different operating systems is guaranteed by the JVM.
Example
Let's look at a simple multithreaded example, which works fine on different operating systems:
public class MultiThreadExample { public static void main(String[] args) { Thread thread1 = new Thread(() -> { for (int i = 0; i Common Errors and Debugging Tips<p> Problems that are easy to encounter in multithreaded programming include deadlock, race conditions and thread safety issues. Here are some common errors and debugging tips:</p>
- Deadlock : A deadlock occurs when two or more threads are waiting for each other to release resources. Using
jstack
tools can help diagnose deadlock problems. - Race condition : Data inconsistency may occur when multiple threads access shared resources at the same time. Using
synchronized
orLock
objects can solve this problem. - Thread safety : Ensure that access to shared data is thread-safe, using atomic operations or concurrent collections if necessary.
Performance optimization and best practices
In multithreaded programming, performance optimization and best practices are crucial. Here are some suggestions:
- Thread pool : Using thread pools can reduce the overhead of thread creation and destruction and improve program performance. Java provides the
ExecutorService
interface andExecutors
class to create and manage thread pools. - Concurrent collection : Use concurrent collections in the
java.util.concurrent
package (such asConcurrentHashMap
,CopyOnWriteArrayList
) to improve thread safety and performance. - Avoid oversync : Too much synchronization can lead to performance degradation and minimize the range of synchronized code blocks.
- Code readability and maintenance : Write clear and readable multi-threaded code and add appropriate comments and documents to facilitate subsequent maintenance and debugging.
In-depth thinking and suggestions
Cross-platform consistency of JVM is one of its major advantages when dealing with multithreading, but it also brings some challenges. Different thread implementation differences in different operating systems may lead to performance differences, and developers need to conduct adequate testing in different environments. At the same time, the JVM garbage collection mechanism also needs special attention in a multi-threaded environment, because frequent GC may affect the performance of multi-threaded.
In a practical project, I encountered an interesting case: In a large-scale distributed system, we use a large number of multithreading to handle concurrent requests. Since the system runs on different operating systems, we find that some threads perform much better on Linux than on Windows. After in-depth analysis, we found that this is because Linux's thread scheduling mechanism is more suitable for the JVM's thread scheduler. Finally, we successfully achieved performance consistency on different operating systems by adjusting JVM parameters and optimizing code logic.
In short, understanding the way JVM handles multithreading on different operating systems can not only help us write more efficient multithreading programs, but also allow us to better cope with challenges in cross-platform development. Hope this article provides you with valuable insights and practical advice.
The above is the detailed content of How does the JVM handle multithreading on different operating systems?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




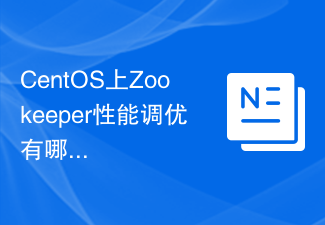
Zookeeper performance tuning on CentOS can start from multiple aspects, including hardware configuration, operating system optimization, configuration parameter adjustment, monitoring and maintenance, etc. Here are some specific tuning methods: SSD is recommended for hardware configuration: Since Zookeeper's data is written to disk, it is highly recommended to use SSD to improve I/O performance. Enough memory: Allocate enough memory resources to Zookeeper to avoid frequent disk read and write. Multi-core CPU: Use multi-core CPU to ensure that Zookeeper can process it in parallel.
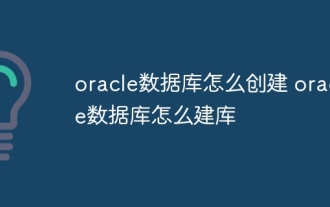
To create an Oracle database, the common method is to use the dbca graphical tool. The steps are as follows: 1. Use the dbca tool to set the dbName to specify the database name; 2. Set sysPassword and systemPassword to strong passwords; 3. Set characterSet and nationalCharacterSet to AL32UTF8; 4. Set memorySize and tablespaceSize to adjust according to actual needs; 5. Specify the logFile path. Advanced methods are created manually using SQL commands, but are more complex and prone to errors. Pay attention to password strength, character set selection, tablespace size and memory

This article discusses how to improve Hadoop data processing efficiency on Debian systems. Optimization strategies cover hardware upgrades, operating system parameter adjustments, Hadoop configuration modifications, and the use of efficient algorithms and tools. 1. Hardware resource strengthening ensures that all nodes have consistent hardware configurations, especially paying attention to CPU, memory and network equipment performance. Choosing high-performance hardware components is essential to improve overall processing speed. 2. Operating system tunes file descriptors and network connections: Modify the /etc/security/limits.conf file to increase the upper limit of file descriptors and network connections allowed to be opened at the same time by the system. JVM parameter adjustment: Adjust in hadoop-env.sh file
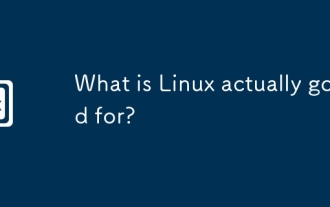
Linux is suitable for servers, development environments, and embedded systems. 1. As a server operating system, Linux is stable and efficient, and is often used to deploy high-concurrency applications. 2. As a development environment, Linux provides efficient command line tools and package management systems to improve development efficiency. 3. In embedded systems, Linux is lightweight and customizable, suitable for environments with limited resources.
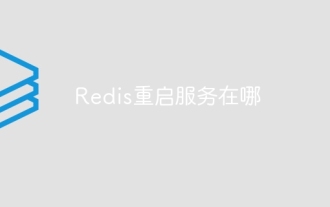
How to restart the Redis service in different operating systems: Linux/macOS: Use the systemctl command (systemctl restart redis-server) or the service command (service redis-server restart). Windows: Use the services.msc tool (enter "services.msc" in the Run dialog box and press Enter) and right-click the "Redis" service and select "Restart".
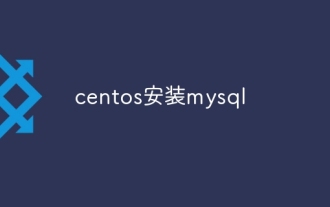
Installing MySQL on CentOS involves the following steps: Adding the appropriate MySQL yum source. Execute the yum install mysql-server command to install the MySQL server. Use the mysql_secure_installation command to make security settings, such as setting the root user password. Customize the MySQL configuration file as needed. Tune MySQL parameters and optimize databases for performance.
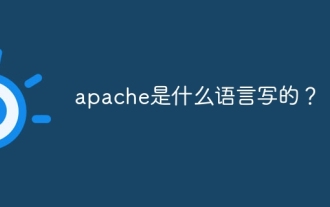
Apache is written in C. The language provides speed, stability, portability, and direct hardware access, making it ideal for web server development.
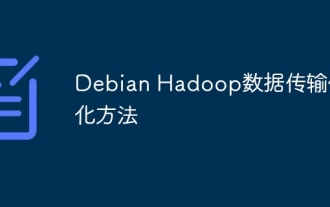
The key to improving the efficiency of data transmission in DebianHadoop cluster lies in the comprehensive application of multiple strategies. This article will elaborate on optimization methods to help you significantly improve cluster performance. 1. The data localization strategy maximizes the allocation of computing tasks to the data storage nodes, effectively reducing data transmission between nodes. Hadoop's data localization mechanism will automatically move data blocks to the node where the computing task is located, thereby avoiding performance bottlenecks caused by network transmission. 2. Data compression technology adopts data compression technology during data transmission to reduce the amount of data transmitted on the network and thereby improve transmission efficiency. Hadoop supports a variety of compression algorithms, such as Snappy, Gzip, LZO, etc. You can choose the optimal algorithm according to the actual situation. three,
