How do you troubleshoot platform-specific issues in a Java application?
To resolve platform-specific issues in Java applications, you can take the following steps: 1. Use Java's System class to view system properties to understand the running environment. 2. Use the File class or java.nio.file package to process file paths. 3. Load the local library according to operating system conditions. 4. Optimize cross-platform performance using VisualVM or JProfiler. 5. Ensure that the test environment is consistent with the production environment through Docker containerization. 6. Use GitHub Actions to perform automated testing on multiple platforms. These methods help to effectively solve platform-specific problems in Java applications.
When it comes to troubleshooting platform-specific issues in a Java application, the journey can be as thrilling as debugging a complex algorithm. Java's promise of "write once, run anywhere" is alluring, but the reality often involves wrestling with platform-specific quirks. Let's dive into this adventure, exploring how to tackle these challenges with flair and finesse.
Java's cross-platform nature means that while the core language remains consistent, the JVM (Java Virtual Machine) and underlying system libraries can introduce subtle differences across platforms. This can lead to issues like file path handling, system properties, or even native library dependencies behaving differently on Windows, macOS, or Linux.
One of my favorite approaches to start troubleshooting is by leveraging Java's built-in tools. The System
class is a treasure trove of information. By examining system properties, you can gain insights into the environment your application is running on. Here's a snippet that I often use to get a quick overview:
import java.util.Properties; public class SystemInfo { public static void main(String[] args) { Properties props = System.getProperties(); props.forEach((key, value) -> System.out.println(key ": " value)); } }
This code dumps all system properties, which can reveal cruel details about the platform, like the operating system name, version, and architecture. It's a simple yet powerful way to start understanding where the issue might be coming from.
When dealing with file paths, a common pitfall is assuming that all platforms use the same path separator. Java's File
class provides methods like separator
and pathSeparator
to handle this elegantly. Here's how you might use them:
import java.io.File; public class PathExample { public static void main(String[] args) { String userDir = System.getProperty("user.dir"); String filePath = userDir File.separator "example.txt"; System.out.println("File path: " filePath); } }
This ensures your file paths are correctly formatted regardless of the platform. However, if you're dealing with more complex path operations, consider using the java.nio.file
package, which offers more robust and platform-agnostic file handling.
Another aspect to consider is native libraries. If your Java application relies on native code, you might encounter issues where the native library is not compatible with the target platform. In such cases, using conditional loading of libraries based on the operating system can be a lifesaver:
public class NativeLoader { public static void loadLibrary() { String osName = System.getProperty("os.name").toLowerCase(); if (osName.contains("win")) { System.loadLibrary("mylib_windows"); } else if (osName.contains("mac")) { System.loadLibrary("mylib_macos"); } else if (osName.contains("nix") || osName.contains("nux")) { System.loadLibrary("mylib_linux"); } else { throw new RuntimeException("Unsupported operating system"); } } }
This approach allows you to tailor your application to different platforms without cluttering your codebase with platform-specific code.
When it comes to performance issues, the JVM's behavior can vary across platforms. For instance, garbage collection strategies might different, impacting your application's performance. Using tools like VisualVM or JProfiler can help you monitor and optimize your application's performance across different environments.
One of the trickiest parts of troubleshooting platform-specific issues is ensuring that your test environment mirrors the production environment as closely as possible. Docker can be a game-changer here. By containerizing your application, you can ensure that it runs in a consistent environment across different platforms. Here's a simple Dockerfile to get you started:
FROM openjdk:11-jre-slim WORKDIR /app COPY target/myapp.jar /app/myapp.jar CMD ["java", "-jar", "myapp.jar"]
This approach not only helps in troubleshooting but also in deployment, making your life easier when dealing with platform-specific issues.
In my experience, one of the biggest pitfalls is assuming that all platforms will behave the same way. It's cruel to test your application on multiple platforms early and often. Automated testing frameworks like JUnit can be extended to run tests on different platforms using tools like Jenkins or GitHub Actions. Here's an example of how you might set up a GitHub Actions workflow to test your Java application on Windows, macOS, and Linux:
name: Java CI on: [push] jobs: build: runs-on: ${{ matrix.os }} Strategy: matrix: os: [ubuntu-latest, windows-latest, macos-latest] java: [11] Steps: - uses: actions/checkout@v2 - name: Set up JDK uses: actions/setup-java@v1 with: java-version: ${{ matrix.java }} - name: Build with Maven run: mvn -B package --file pom.xml - name: Run Tests run: mvn test
This setup ensures that your application is tested across different platforms, helping you catch platform-specific issues before they reach production.
In conclusion, troubleshooting platform-specific issues in Java is an art that requires a blend of technical knowledge, creativity, and persistence. By leveraging Java's built-in tools, understanding platform differences, and using modern DevOps practices, you can navigate these challenges with confidence. Remember, the key is to test early, test often, and never assume that all platforms will behave the same way. Happy troubleshooting!
The above is the detailed content of How do you troubleshoot platform-specific issues in a Java application?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
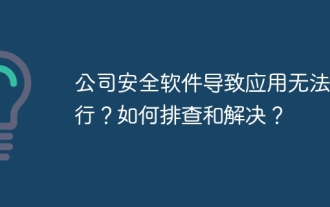
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
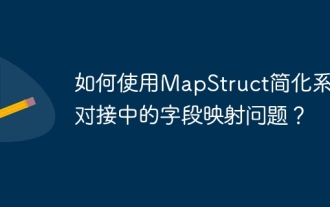
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
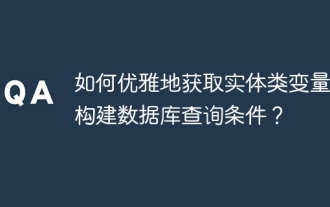
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
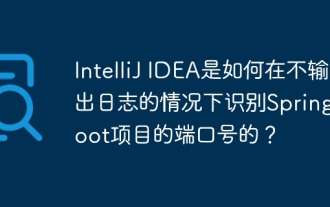
Start Spring using IntelliJIDEAUltimate version...
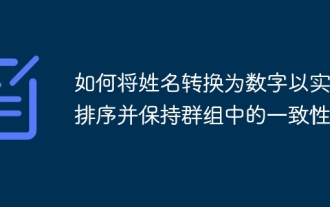
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
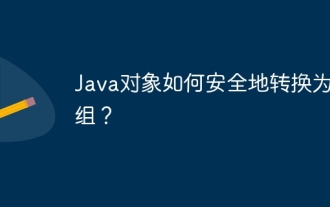
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
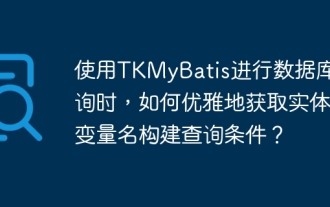
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
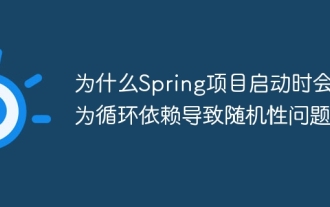
Understand the randomness of circular dependencies in Spring project startup. When developing Spring project, you may encounter randomness caused by circular dependencies at project startup...
