


What are the advantages of using a database to store sessions?
The main advantages of using database storage sessions include persistence, scalability, and security. 1. Persistence: Even if the server restarts, the session data can remain unchanged. 2. Scalability: Applicable to distributed systems, ensuring session data is synchronized between multiple servers. 3. Security: The database provides encrypted storage to protect sensitive information.
introduction
In modern web development, how to manage user sessions is a key issue. Using a database to store session data is a common practice, and this article will dig into the advantages of this approach. By reading this article, you will learn about the specific benefits of database storage sessions and how to use these advantages to improve system performance and security in practical applications.
Review of basic knowledge
Before discussing the advantages of storing sessions in databases, let’s review the basic concepts of session management. A session is the status information maintained for a period of time when a user interacts with an application. Traditionally, session data can be stored in memory (such as the HttpSession of a Servlet container) or in a file system or database. As a persistent storage means, databases provide more powerful functions and flexibility than memory or file systems.
Core concept or function analysis
Definition and function of database storage sessions
A database storage session refers to saving user session data into a database rather than relying on memory or file system. The main function of this approach is to provide a persistent, scalable and secure session management mechanism.
For example, suppose we use a MySQL database to store session data:
CREATE TABLE sessions ( session_id VARCHAR(255) PRIMARY KEY, user_id INT, data TEXT, last_activity TIMESTAMP );
This simple table structure can help us understand how a database stores session data.
How it works
When the user logs in, the application generates a unique session ID and stores relevant data (such as user ID, session data, etc.) into the database. Whenever the user performs an action, the application updates the session data and refreshes the last active time. This method ensures the persistence of session data, and the user's session state remains unchanged even if the server is restarted or load balancing.
Example of usage
Basic usage
Let's look at a simple Java example showing how to store sessions using a database:
import java.sql.*; import java.util.HashMap; import java.util.Map; public class SessionManager { private Connection conn; public SessionManager() throws SQLException { conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password"); } public void saveSession(String sessionId, int userId, String data) throws SQLException { String sql = "INSERT INTO sessions (session_id, user_id, data, last_activity) VALUES (?, ?, ?, NOW()) " "ON DUPLICATE KEY UPDATE user_id = ?, data = ?, last_activity = NOW()"; try (PreparedStatement pstmt = conn.prepareStatement(sql)) { pstmt.setString(1, sessionId); pstmt.setInt(2, userId); pstmt.setString(3, data); pstmt.setInt(4, userId); pstmt.setString(5, data); pstmt.executeUpdate(); } } public Map<String, Object> getSession(String sessionId) throws SQLException { String sql = "SELECT user_id, data FROM sessions WHERE session_id = ? AND last_activity > DATE_SUB(NOW(), INTERVAL 30 MINUTE)"; try (PreparedStatement pstmt = conn.prepareStatement(sql)) { pstmt.setString(1, sessionId); try (ResultSet rs = pstmt.executeQuery()) { if (rs.next()) { Map<String, Object> session = new HashMap<>(); session.put("userId", rs.getInt("user_id")); session.put("data", rs.getString("data")); return session; } } } return null; } }
This example shows how to save and retrieve session data in a database. The saveSession
method is used to save or update session data, while the getSession
method is used to get session data and check whether the session has been active for the past 30 minutes.
Advanced Usage
In more complex scenarios, we may need to implement session replication and load balancing. Suppose we have a distributed system, and user requests may be routed to different servers. To ensure consistency of session data, we can use the database as the central repository for session storage.
public class DistributedSessionManager extends SessionManager { public DistributedSessionManager() throws SQLException { super(); } public void replicateSession(String sessionId, int userId, String data) throws SQLException { saveSession(sessionId, userId, data); // Suppose we have a message queue system that notifies other servers to update session data// Here you can add code to send messages to the message queue} }
This approach ensures that in a distributed environment, session data can be kept synchronized across all servers.
Common Errors and Debugging Tips
Common errors when using database storage sessions include:
- Loss of session data : It may be caused by database connection problems or improper transaction management. Make sure to use transactions to ensure data consistency and check database connection status regularly.
- Performance bottlenecks : Frequent database read and write operations may cause performance problems. The cache mechanism can be used to reduce the number of database accesses or optimize database queries.
Debugging skills include:
- Logging : Records the logs of session operations in detail to help track problems.
- Monitoring Tools : Use database monitoring tools to analyze query performance and connection status.
Performance optimization and best practices
In practical applications, it is very important to optimize the performance of database storage sessions. Here are some optimization strategies:
- Using Index : Create indexes on
session_id
andlast_activity
fields can significantly improve query performance. - Caching mechanism : Use in-memory caches (such as Redis) to store commonly used session data and reduce direct access to the database.
- Session Expiration Policy : Regularly clean out expired session data to avoid database bloating.
Best practices include:
- Security : Ensure the security of session data in the database and use encryption to store sensitive information.
- Scalability : Design database structures with future scalability requirements in mind to ensure that the system can handle more session data.
- Code readability : Keep the code clear and maintainable, ensuring that team members can easily understand and modify session management logic.
In-depth insights and thoughts
The advantages of using a database to store sessions are obvious, but there are also some potential challenges and tradeoffs to consider:
- Persistence and Performance : Although databases provide persistence, frequent database operations may affect performance. A balance between persistence and performance needs to be found.
- Complexity : Database storage sessions increase system complexity compared to in-memory storage and require more maintenance and management efforts.
- Cost : A database storage session may require more hardware resources and maintenance costs and needs to be evaluated for whether it is worth it.
In practical applications, when choosing a database storage session plan, it is necessary to comprehensively consider the specific needs and resource conditions of the system. Through reasonable design and optimization, the advantages of database storage sessions can be fully utilized while avoiding potential pitfalls.
In short, using a database to store sessions is a powerful and flexible method of session management. Through this discussion, I hope you can better understand its advantages and flexibly apply them in real projects.
The above is the detailed content of What are the advantages of using a database to store sessions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
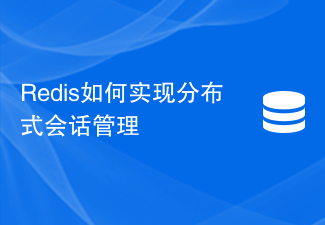
How Redis implements distributed session management requires specific code examples. Distributed session management is one of the hot topics on the Internet today. In the face of high concurrency and large data volumes, traditional session management methods are gradually becoming inadequate. As a high-performance key-value database, Redis provides a distributed session management solution. This article will introduce how to use Redis to implement distributed session management and give specific code examples. 1. Introduction to Redis as a distributed session storage. The traditional session management method is to store session information.
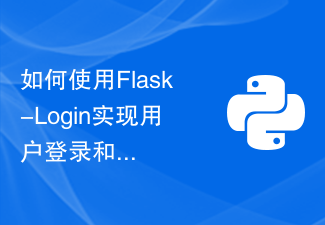
How to use Flask-Login to implement user login and session management Introduction: Flask-Login is a user authentication plug-in for the Flask framework, through which we can easily implement user login and session management functions. This article will introduce how to use Flask-Login for user login and session management, and provide corresponding code examples. 1. Preparation Before using Flask-Login, we need to install it in the Flask project. You can use pip with the following command
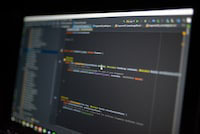
This article will explain in detail about starting a new or restoring an existing session in PHP. The editor thinks it is very practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP Session Management: Start a New Session or Resume an Existing Session Introduction Session management is crucial in PHP, it allows you to store and access user data during the user session. This article details how to start a new session or resume an existing session in PHP. Start a new session The function session_start() checks whether a session exists, if not, it creates a new session. It can also read session data and convert it

In-depth study of the underlying development principles of PHP: session management and state retention methods Preface In modern web development, session management and state retention are very important parts. Whether it is the maintenance of user login status or the maintenance of shopping cart and other status, session management and status maintenance technology are required. In the underlying development of PHP, we need to understand the principles and methods of session management and state retention in order to better design and tune our web applications. The basic session of session management refers to the client and server
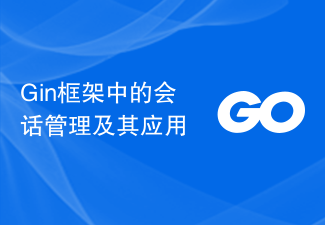
The Gin framework is a lightweight Web framework developed using the Go language and has the advantages of efficiency, ease of use, and flexibility. In web application development, session management is a very important topic. It can be used to save user information, verify user identity, prevent CSRF attacks, etc. This article will introduce the session management mechanism and its application in the Gin framework. 1. Session management mechanism In the Gin framework, session management is implemented through middleware. The Gin framework provides a ses
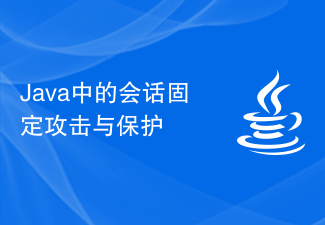
Session Fixation Attacks and Protection in Java In web applications, sessions are an important mechanism for tracking and managing user activities on a website. It does this by storing session data between the server and client. However, a session fixation attack is a security threat that exploits session identifiers to gain unauthorized access. In this article, we will discuss session fixation attacks in Java and provide some code examples of protection mechanisms. A session fixation attack occurs when an attacker injects malicious code or otherwise steals legitimate users
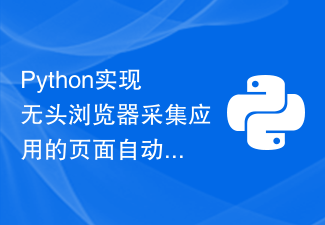
Analysis of the automatic page login and session management functions of Python to implement headless browser collection applications Introduction: With the rapid development of the Internet, our lives are increasingly inseparable from network applications. For many web-type applications, we need to log in manually to obtain more information or operate certain functions. In order to improve efficiency, we can implement automatic page login and session management functions through automated scripts. Headless browser: Before implementing automatic page login and session management functions, we first need to understand what a headless browser is.
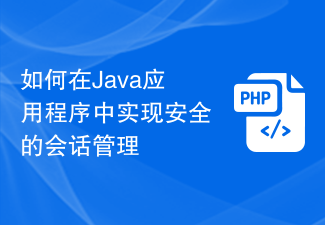
How to implement secure session management in Java applications With the popularity of the Internet and the rapid transmission of data, security issues have become more and more prominent. In a Java application, session management is a crucial security measure. It involves handling user authentication, rights management, session timeout, etc. This article will introduce how to implement secure session management in Java applications. User Authentication User authentication is the foundation of session management. In Java applications, it is common to use username and password to authenticate users.
