


THREE.JS Getting Started Tutorial (5) Ten Things You Should Know_Basic Knowledge
Three.js is a great open source WebGL library. WebGL allows JavaScript to operate the GPU and achieve true 3D on the browser side. However, this technology is still in the development stage, and the information is extremely scarce. Enthusiasts basically have to learn through the Demo source code and the source code of Three.js itself.
0. Introduction
Hi, this is my first article about how to write good code. Like many developers, I learned by doing, but I also learned from other, more experienced developers. I've been spending a lot of time with the canvas tag over the past few months, and I thought it would be interesting to write down all the little tricks I've learned about WebGL and JavaScript during this time. Some are very specific and some are very general. I hope you like them!
1. Write a prototype as soon as possible
Let’s start simple. Now that you have a brilliant idea, you should quickly write a prototype of the most complex part of the program to see if the technology can implement your idea. WebGL is very powerful because it can directly manipulate the GPU in the graphics card, but don't forget that you need to access the graphics card through JavaScript, which is much less efficient than the internal computing of the graphics card. In fact, your genius idea is likely to be defeated by something as simple as this.
2. Use THREE.JS for 3D processing
Like my friend Hakim, I also fully understand the low-level details of the technology we are using. It's important to understand what's underneath the surface, but if you use three.js, it saves you so much trouble. You can use it with Canvas, WebGL, and SVG, and you should find which method suits your needs.
3. Avoid SetInterval
This is an important point for anyone who uses JavaScript to create animations. Why? Suppose you set a function to be executed every 20 milliseconds, and this function takes more than 20 milliseconds to execute, then after 20ms, the browser will not care and will directly start the next execution. At least you can use SetTimeout to set, after a function is executed, execute it again.
In fact, there is a more modern but still half-finished function called requestAnimationFrame, and it is great. It is very similar to the setTimeOut function, except in two respects: when the tab loses focus, it no longer runs; now this function is still browser-dependent, and the standard may change in the future. If you want more information, you can visit Paul Irish's blog.
4. Use reverse order loop
This is a nice little trick that can make your loop faster. Use reverse order and use a while loop. For example, this loop:
for(var a = 0; a < arr.length; a ) {
// Do something
}
It is not as efficient as the following loop:
// Assume the array arr exists
var aLength = arr.length;
while(aLength --) {
// Do something
}
This may not save you much overhead, because the efficiency of execution mainly depends on what you do in the loop body . But if you want to reduce the unnecessary overhead of the program to the last byte, the latter loop will definitely win.
To be honest, the main factor that affects program execution efficiency is the length of the array cache. You can (and indeed should) check out JSPerf to learn about this, and other factors that affect JavaScript performance.
5. Use textures
It may seem tempting to draw every detail of an object in WebGL, but if possible, you should pay attention to whether you can use textures , as it can greatly improve performance. In some specific cases, such as shadows or blur effects, you may have to use textures, but at other times, you should always pay attention to whether you can use textures.
6. Use caching
I have tried this a lot in my own experiments. In the frame loop, you should avoid referencing variables, objects or anything else. For this reason, it is worthwhile to cache all your models and vertices so that you can quickly access them when rendering animations.
7. Disable check
I love this little piece of code and I put it in any page that contains Canvas or WebGL.
// Disable mouse selection of DOM element
document. onselectstart = function() {
return false;
};
You may also want to disable selection only in the Canvas control. This is the code I use in projects where the Canvas takes up the entire screen.
8. Avoid defining CSS in JavaScript
Nowadays, it is so convenient to define CSS in JavaScript, especially when you use JQuery
// Try not to do this
$("#someid").css({
position: 'relative',
height: '30px',
width: '300px',
backgroundColor: '#A020F0'
});
The problem is After doing this, your JavaScript code will soon be filled with various types of CSS definitions, and you also use *.css files to define CSS, making potential problems difficult to detect. A better approach is to use classes to modularize CSS and only define unpredictable CSS classes in JavaScript.
9. Define the callback function in the object
I love the following code. This is by no means something I came up with, but it is so neat and beautiful. If you have a lot of callback functions to use, you might use them like this:
$("#someid").click(function() {
// Callback function
// Returning false in JQuery will prevent the delivery of messages and the release of default behavior
return false;
});
Alternatively, you would call back a loose function defined elsewhere in the code, like this
$("#someid").click(mySuperFunction);
function mySuperFunction(event) {
// Doing a lot of things here
return false;
}
There are some issues with doing this. In the first piece of code, you bound an anonymous function to an event, and it is difficult to unbind the function from the event. You can of course unload all functions on an event, but you may have multiple functions bound to it and you only want to unload one. In the second case, your function name pollutes the global variable space and the maintainability of the code is reduced. So, consider doing this:
$("#someid" ).click(callbacks.mySuperFunction);
// All callback functions are in the callbacks object
var callbacks = {
mySuperFunction:function(event) {
// More work
return false;
}
}
// Unbind a function
$("#someid").unbind('click', callbacks.mySuperFunction);
This is neat and clean, and avoids the two problems mentioned above.
10. Chained ternary operator
I learned this entirely from Paul Irish's "JQuery, 11 Things You Should Know". This is very useful and you should like it too. We often do this:
// According to the value of a, it is numberBasedOnA assignment
// If a is greater than 5, assign 200, otherwise assign 38
var numberBasedOnA = a > 5 ? 200 : 38;
But if you want to do this, For example, what if the value is a certain value, what if the value is greater than a certain value, what if the value is larger, you know? In this case, the chained ternary operator is very useful:
var numberBasedOnA =
a < 5 ? 200 :
a < 7 ? 38 :
a < 11 ? 15 :
a < 15 ? 49 :
64;
// More efficient than doing this
// when a >=15

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
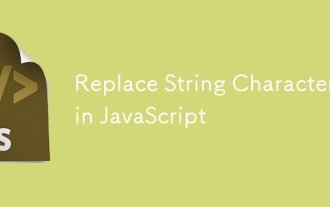
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
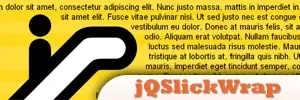
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
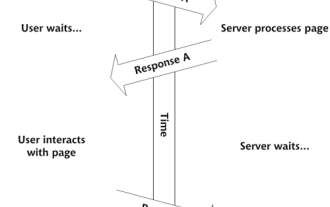
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
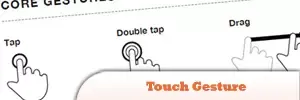
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig
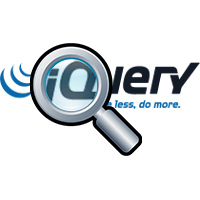
jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
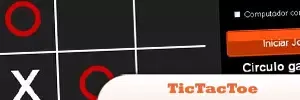
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
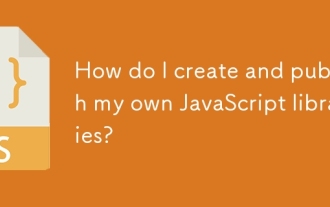
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
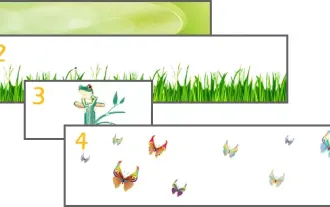
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
