


THREE.JS introductory tutorial (4) Creating a particle system_Basic knowledge
Three.js is a great open source WebGL library. WebGL allows JavaScript to operate the GPU and achieve true 3D on the browser side. However, this technology is still in the development stage, and the information is extremely scarce. Enthusiasts basically have to learn through the Demo source code and the source code of Three.js itself.
0. Introduction
Hi, we meet again. So we have started to learn Three.js. If you have not read the previous three tutorials, I suggest you read them first. If you've read the previous tutorials, you might want to do something with particles. Let’s face it, everyone loves particle effects. Whether you know it or not, you can create them easily.
1. Create a particle system
Three.js treats the particle system as a basic geometry, because it is like a basic geometry, with a shape, position, scaling factor, Rotation attribute. The particle system treats each point in the geometry object as a separate particle. Why do this? I think it's for the following reasons: first, to draw the entire particle system, you only need to call a certain drawing function once, instead of calling it thousands of times; second, this allows you to set some global parameters to affect all the components in your particle system. particle.
Even if the particle system is treated as a whole object, we can still color each particle individually, because during the process of drawing the particle system, Three.js passes it to the shader through the attribute variable color The color of each vertex. I'm not going to do that in this tutorial. If you want to know how this is done, you can go to GitHub to see the Three.js example.
The particle system may have a special effect that needs your attention: Three.js will cache the data of the particle system when it is rendered for the first time. You cannot increase or decrease the data in the system afterwards. particle. If you don't want to see a particle, you can set the alpha value in its color to 0, but you can't delete it. So when you create a particle system, you should take into account all the particles that may need to be displayed.
To start creating a particle system, you only need this much:
// Create particle geometry
var particleCount = 1800,
particles = new THREE.Geometry(),
pMaterial =
new THREE.ParticleBasicMaterial({
color: 0xFFFFFF,
size: 20
});
// Create individual particles in sequence
for(var p = 0; p < particleCount; p ) {
// Particle range Between -250 and 250
var pX = Math.random() * 500 - 250,
pY = Math.random() * 500 - 250,
pZ = Math.random() * 500 - 250,
particle = new THREE.Vertex(
new THREE.Vector3(pX, pY, pZ)
);
// Add particles to particle geometry
particles.vertices.push (particle);
}
// Create particle system
var particleSystem =
new THREE.ParticleSystem(
particles,
pMaterial);
// Add particle system Scene
scene.addChild(particleSystem);
If you run :
1. You will find that the particles are all square
2. The particles are all square Stay still
We will fix it one by one.
2. Style
We passed in the color and size when creating a particle basic material. What we might want to do is pass in a texture image to display the particles, so that we can have good control over how the particles look.
You can also see that the particles are drawn in a square shape, so we should also use a square texture image. To make it look better, I would also use additive blending, but this would make sure that the background of the texture image is black and not transparent. The reason I understand is: there is now incompatibility between additive blending and transparent materials. But it doesn't matter, it will look great in the end.
Let’s update the particle basic material and particle system, and add some additively mixed transparent particles. You can also use my particle pictures if you like.
// Create particle basic material
var pMaterial =
new THREE.ParticleBasicMaterial({
color: 0xFFFFFF,
size: 20,
map: THREE.ImageUtils.loadTexture(
"images/particle.png"
),
blending: THREE.AdditiveBlending,
transparent: true
});
// Allow the particle system to sort particles to achieve the effect we want
particleSystem.sortParticles = true;
This is looking a lot better already. Now let’s introduce a little physics and let the particles move.
3. Introducing physics
By default, the particle system does not move in three-dimensional space, which is good. But I want them to move, and I want the particle system to move like this: let the particles rotate around the y-axis. And the range of the particles on each axis is between -250 and 250, so rotating around the y-axis means they are rotating around the center of the system.
I also assume that you already have the frame loop code somewhere, similar to what I did in my last tutorial on shaders. So here we only need to do this:
// Frame loop
function update() {
// Add a little rotation
particleSystem.rotation.y = 0.01;
// Draw the particle system
renderer.render(scene, camera);
//Set the call to update when the next frame is refreshed
requestAnimFrame(update);
}
Now we start to define the movement of a single particle (Translator's Note: The previous rotation is the motion of the entire particle system). Let’s make a simple raindrop effect. This includes the following steps:
1. Assign each particle an initial speed of 0
2. In each frame, for each A particle is assigned a random gravitational acceleration
3. In each frame, the velocity is updated through acceleration and the position is updated through velocity
4. When a particle moves out of sight, reset the initial position and velocity
Sounds like a lot, but actually it takes very little code to write. First, in the process of creating particles, we add a horizontal speed to each particle:
// Create a horizontal motion velocity for each particle
particle.velocity = new THREE.Vector3(
0, // x
-Math.random(), // y: random number
0); // z
Next, we pass each particle in the framebuffer, and reset it when the particle leaves the bottom of the screen and needs to be reset position and speed.
// Frame loop
function update() {
// Increase the amount of rotation
particleSystem.rotation.y = 0.01;
var pCount = particleCount;
while(pCount--) {
// Get a single particle
var particle = particles.vertices[pCount];
// Check if reset is needed
if(particle.position.y < -200) {
particle.position.y = 200;
particle. velocity.y = 0;
}
// Update the horizontal velocity component with a random number and update the position according to the velocity
particle.velocity.y -= Math.random() * .1;
particle.position.addSelf(
particle.velocity);
}
// Tell the particle system that we changed the particle position
particleSystem.geometry.__dirtyVertices = true;
// Draw
renderer.render(scene, camera);
//Set the next call
requestAnimFrame(update);
}
Although it is not shocking enough, this particle at least shows How to do it. You should totally create some awesome particle effects yourself and let me know.
Here’s a warning you should be aware of, with the frame loop, I went overboard: I looped through all the particles in one loop, which is actually a very rough approach. If you are doing too much work in your frame loop (Translator's Note: Note that the js code of the frame loop is run in the CPU, it is not like the GPU, which can issue thousands of simple processes at once), browse The browser will freeze. In fact, if you use requestAnimationFrame, its view will refresh 60 times per second. So it's better to optimize your code and do as little as possible in the frame loop.
4. Summary
The particle effect is great, everyone loves particle effects, and now you know how to add particle effects in Three.js. I hope you find it easy to use, just like before!
Again, here is the source code for download, and let me know how you like it!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
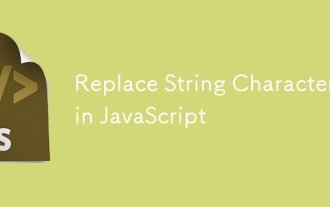
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
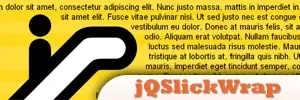
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
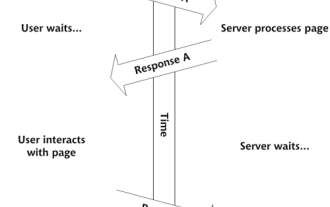
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
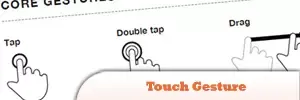
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig
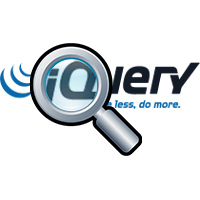
jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
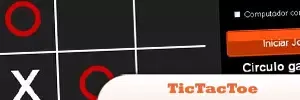
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
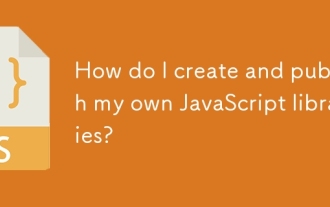
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
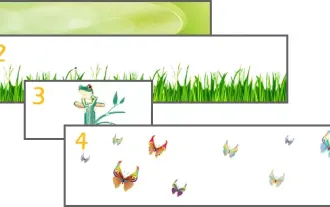
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
