


Javascript image processing—threshold function example application_javascript skills
In the previous article , we explained the changes in brightness and contrast in image processing. In this article we will make a threshold function.
The simplest image segmentation method
Thresholding is the simplest image segmentation method.
For example, in order to segment an apple from the picture below, we use the grayscale difference between the foreground and the background to set a threshold. If the pixel is greater than this threshold, it will be represented in black, and if it is less than the threshold, it will be represented in gray.
Five threshold types
Like OpenCV, we will provide five threshold types for easy use.
The following is the waveform representation of the original image. The ordinate represents the gray value of the pixel, and the blue line is the threshold size.
The formula is:
The image representation is:
It can be seen that if the threshold is exceeded, it becomes the maximum value (that is, 255), otherwise it becomes the minimum value (that is, 0). We need a function to implement this function:
var CV_THRESH_BINARY = function(__value, __thresh, __maxVal){
return __value > __thresh ? __maxVal : 0;
};
Inverse binary thresholding
The formula is:
The image representation is:
This is the opposite, if it exceeds the threshold, it becomes the minimum value, otherwise it becomes the maximum value. The function implementation is:
var CV_THRESH_BINARY_INV = function(__value, __thresh, __maxVal){
return __value > __thresh ? 0 : __maxVal;
};
Truncate thresholding
The formula is:
The image representation is:
It can be seen that this is truncated if it exceeds the threshold. The function implementation is:
var CV_THRESH_TRUNC = function(__value, __thresh, __maxVal){
return __value > __thresh ? __thresh : 0;
};
Threshold to 0
The formula is:
The image representation is:
In this case, all values smaller than the threshold are set to 0. Function implementation:
var CV_THRESH_TOZERO = function(__value, __thresh, __maxVal){
return __value >
The formula is: texttt{thresh}$}{texttt{src}(x,y)}{otherwise}" src="http://files.jb51.net/file_images/article/201301/2013010314344061.png" >
The image representation is:
Copy code
The code is as follows:
Copy code
The code is as follows:
dData = dst.data,
maxVal = __maxVal || 255,
threshouldType = __thresholdType || CV_THRESH_BINARY;
var i, j, offset;
for(i = height; i --;){
for(j = width; j--;){
offset = i * width j;
dData[offset] = threshouldType(sData[offset], __thresh, maxVal);
}
}
}else{
error(arguments.callee, UNSPPORT_DATA_TYPE/* {line} */);
}
return dst;
};
This function is relatively simple, that is, assigning a value to each pixel as
Copy code
The code is as follows:

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
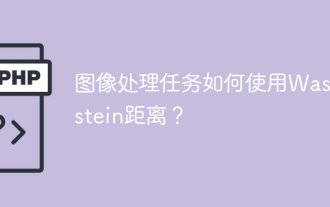
Wasserstein distance, also known as EarthMover's Distance (EMD), is a metric used to measure the difference between two probability distributions. Compared with traditional KL divergence or JS divergence, Wasserstein distance takes into account the structural information between distributions and therefore exhibits better performance in many image processing tasks. By calculating the minimum transportation cost between two distributions, Wasserstein distance is able to measure the minimum amount of work required to transform one distribution into another. This metric is able to capture the geometric differences between distributions, thereby playing an important role in tasks such as image generation and style transfer. Therefore, the Wasserstein distance becomes the concept
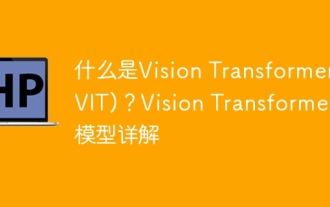
VisionTransformer (VIT) is a Transformer-based image classification model proposed by Google. Different from traditional CNN models, VIT represents images as sequences and learns the image structure by predicting the class label of the image. To achieve this, VIT divides the input image into multiple patches and concatenates the pixels in each patch through channels and then performs linear projection to achieve the desired input dimensions. Finally, each patch is flattened into a single vector, forming the input sequence. Through Transformer's self-attention mechanism, VIT is able to capture the relationship between different patches and perform effective feature extraction and classification prediction. This serialized image representation is
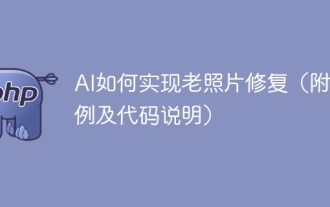
Old photo restoration is a method of using artificial intelligence technology to repair, enhance and improve old photos. Using computer vision and machine learning algorithms, the technology can automatically identify and repair damage and flaws in old photos, making them look clearer, more natural and more realistic. The technical principles of old photo restoration mainly include the following aspects: 1. Image denoising and enhancement. When restoring old photos, they need to be denoised and enhanced first. Image processing algorithms and filters, such as mean filtering, Gaussian filtering, bilateral filtering, etc., can be used to solve noise and color spots problems, thereby improving the quality of photos. 2. Image restoration and repair In old photos, there may be some defects and damage, such as scratches, cracks, fading, etc. These problems can be solved by image restoration and repair algorithms
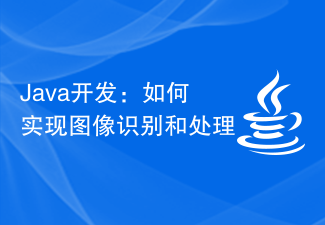
Java Development: A Practical Guide to Image Recognition and Processing Abstract: With the rapid development of computer vision and artificial intelligence, image recognition and processing play an important role in various fields. This article will introduce how to use Java language to implement image recognition and processing, and provide specific code examples. 1. Basic principles of image recognition Image recognition refers to the use of computer technology to analyze and understand images to identify objects, features or content in the image. Before performing image recognition, we need to understand some basic image processing techniques, as shown in the figure
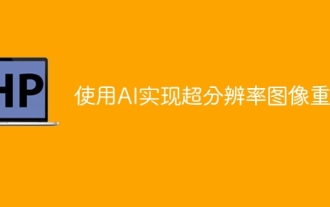
Super-resolution image reconstruction is the process of generating high-resolution images from low-resolution images using deep learning techniques, such as convolutional neural networks (CNN) and generative adversarial networks (GAN). The goal of this method is to improve the quality and detail of images by converting low-resolution images into high-resolution images. This technology has wide applications in many fields, such as medical imaging, surveillance cameras, satellite images, etc. Through super-resolution image reconstruction, we can obtain clearer and more detailed images, which helps to more accurately analyze and identify targets and features in images. Reconstruction methods Super-resolution image reconstruction methods can generally be divided into two categories: interpolation-based methods and deep learning-based methods. 1) Interpolation-based method Super-resolution image reconstruction based on interpolation

PHP study notes: Face recognition and image processing Preface: With the development of artificial intelligence technology, face recognition and image processing have become hot topics. In practical applications, face recognition and image processing are mostly used in security monitoring, face unlocking, card comparison, etc. As a commonly used server-side scripting language, PHP can also be used to implement functions related to face recognition and image processing. This article will take you through face recognition and image processing in PHP, with specific code examples. 1. Face recognition in PHP Face recognition is a
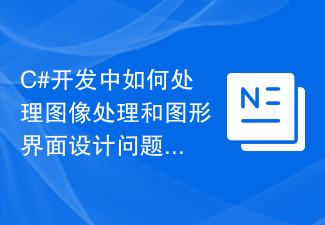
How to deal with image processing and graphical interface design issues in C# development requires specific code examples. Introduction: In modern software development, image processing and graphical interface design are common requirements. As a general-purpose high-level programming language, C# has powerful image processing and graphical interface design capabilities. This article will be based on C#, discuss how to deal with image processing and graphical interface design issues, and give detailed code examples. 1. Image processing issues: Image reading and display: In C#, image reading and display are basic operations. Can be used.N

The Scale Invariant Feature Transform (SIFT) algorithm is a feature extraction algorithm used in the fields of image processing and computer vision. This algorithm was proposed in 1999 to improve object recognition and matching performance in computer vision systems. The SIFT algorithm is robust and accurate and is widely used in image recognition, three-dimensional reconstruction, target detection, video tracking and other fields. It achieves scale invariance by detecting key points in multiple scale spaces and extracting local feature descriptors around the key points. The main steps of the SIFT algorithm include scale space construction, key point detection, key point positioning, direction assignment and feature descriptor generation. Through these steps, the SIFT algorithm can extract robust and unique features, thereby achieving efficient image processing.
