Home
Web Front-end
JS Tutorial
Javascript Inheritance (Part 1) - Introduction to Object Construction_JavaScript



Javascript Inheritance (Part 1) - Introduction to Object Construction_JavaScript
May 16, 2016 pm 05:48 PM
create
object
Does "class" exist in Javascript?
Everything is an object
Except for basic data (Undefined, Null, Boolean, Number, String), everything else in Javascript is Object.
In fact, objects in Javascript are collections of data and functions. For example, we know:
Copy code The code is as follows:
var foo = new Function("alert ('hello world!')");
foo();
It can be seen that foo is a function and an object. Another example:
Copy code The code is as follows:
function foo(){
//do something
}
foo.data = 123;
foo["data2"] = "hello";
alert(foo.data);
alert(foo.data2);
Functions can also add properties like objects.
Construction of objects
Generally we use constructors to construct objects, but if there is no constructor, we also have ways to construct the objects we want:
Copy code The code is as follows:
function creatPerson(__name, __sex, __age){
return {
name: __name,
sex: __sex,
age: __age,
get: function(__key){
alert(this[__key]);
}
};
}
var Bob = creatPerson("Bob", "male", 18);
Bob.get("name"); //Bob
Bob.get("sex"); //male
Bob.get("age"); //18
But this is not enough, I hope the method can be shared. For example, if I use this function to create a Tom object, the get function will be created again, which obviously wastes my memory.
Import shared resources
Because we know that functions are also objects, we can put the methods or properties that need to be shared on him:
Copy code The code is as follows:
function creatPerson(__name, __sex, __age){
var common = arguments.callee.common ;
return {
//Their own attributes
name: __name,
sex: __sex,
age: __age,
//Their own methods
sayhi: function( ){alert("hi");},
//Shared methods
get: common.get,
getType: common.getType,
//Shared attributes
type: common.type
};
}
creatPerson.common = {
get:function(__key){
alert(this[__key]);
},
getType : function(){
alert(this.type);
},
type: "Person"
};
var Bob = creatPerson("Bob", "male ", 18);
Bob.get("name"); //Bob
Bob.get("sex"); //male
Bob.getType(); //Person
So we used a crappy method to successfully create an object with its own property methods and shared property methods. But in fact, this is how Javascript creates objects poorly.
In fact, the shared attribute is not really implemented, because this shared attribute is still just a copy. This is not a shared property we really want.
new keyword
Same as the "Construction of Objects" above, the purpose of new is to create the object's own properties and methods. For example:
Copy code The code is as follows:
function Person(__name, __sex, __age){
this.name = __name;
this.sex = __sex;
this.age = __age;
this.get = function(__key){
alert(this[__key]);
};
}
var Bob = new Person("Bob", "male", 18);
Bob.get("name"); //Bob
Bob.get("sex"); //male
Bob.get("age"); //18
Prototype (Prototype)
Javascript The author used a method similar to the "import shared resources" above. Since functions are also objects, then put the "things" that need to be shared on him:
Copy code The code is as follows :
function Person(__name, __sex, __age){
this.name = __name;
this.sex = __sex;
this.age = __age;
this.sayhi = function(__key){
alert("hi");
};
}
Person.prototype = {
constructor: Person,
get: function( __key){
alert(this[__key]);
}
};
var Bob = new Person("Bob", "male", 18);
Bob .get("name"); //Bob
Bob.get("sex"); //male
alert(Bob.constructor); //function Person
Javascript’s model for creating objects is simple, new handles its own issues, and prototype handles sharing issues.
If Java’s object (instance) generation method is to throw raw materials into a mold (class) and smelt them; then Javascript’s object generation method is to give materials to a builder (constructor) and let him press the drawing Built.
Actual process
Of course the actual process is not like this. The first thing to do when creating a new object is to process shared resources, for example:
Copy code The code is as follows:
function A(){
console.dir(this);
alert(this .type); //A
}
A.prototype.type = "A";
var a = new A();
By printing a through console.dir we can see:
type | "A" |
__proto__ | A {type = "A"} |
type | "A" |
constructor | A() |
After the constructor creates an object, it immediately assigns its prototype reference to the internal attribute __proto__ of the new object, and then runs the construction statement in the constructor.
does not override
Copy code The code is as follows:
function A( ){
this.type = "B"
}
A.prototype.type = "A";
var a = new A();
alert(a. type); //B
When we want to get a.type, the engine will first check whether there is an attribute type in the a object. If there is, it will return the attribute. If not, it will try to use __proto_ Find whether there is a type attribute in _, and if so, return the attribute.
__proto__ is not standard. For example, it is not available on IE, but there are similar internal properties on IE, but we cannot use it.
For this reason, we can still return a.type when we delete a.type:
Copy code The code is as follows:
function A(){
this.type = "B"
}
A.prototype.type = "A";
var a = new A();
alert(a.type); //B
delete a.type;
alert(a.type); //A
Are there any classes?
Strictly speaking, Javascript does not have such a thing as a class.
But sometimes we use the name of the constructor as the "type (type not class) name" of the objects created by the constructor to facilitate communication when we use Javascript for object-oriented programming.
The name is just a code name, a tool for easy understanding.
References
Design ideas of Javascript inheritance mechanism
Statement of this Website
The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn

Hot Article
Repo: How To Revive Teammates
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
R.E.P.O. Energy Crystals Explained and What They Do (Yellow Crystal)
1 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
Hello Kitty Island Adventure: How To Get Giant Seeds
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
How Long Does It Take To Beat Split Fiction?
3 weeks ago
By DDD

Hot tools Tags

Hot Article
Repo: How To Revive Teammates
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
R.E.P.O. Energy Crystals Explained and What They Do (Yellow Crystal)
1 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
Hello Kitty Island Adventure: How To Get Giant Seeds
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
How Long Does It Take To Beat Split Fiction?
3 weeks ago
By DDD

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
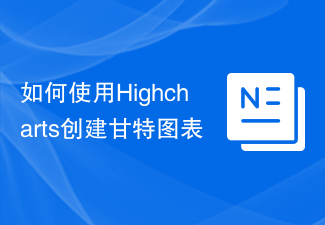
How to create a Gantt chart using Highcharts
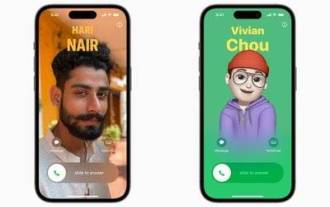
How to Create a Contact Poster for Your iPhone
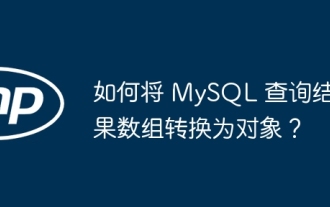
How to convert MySQL query result array to object?
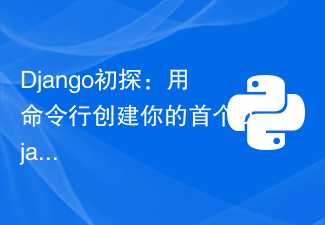
A first look at Django: Create your first Django project using the command line
