RegExp object object in JS regular_javascript skills
There are two ways to create instances of RegExp objects.
Use the explicit constructor of RegExp, the syntax is: new RegExp("pattern"[,"flags"]).
Use the implicit constructor of RegExp, in plain text format: /pattern/[flags]. The
pattern part is the regular expression pattern text to be used, which is required. In the first method, the pattern part exists in the form of a JavaScript string and needs to be enclosed in double quotes or single quotes; in the second method, the pattern part is nested between two "/" and quotation marks cannot be used. . The
flags part sets the flag information of the regular expression, which is optional. If the flags part is set, in the first way, it exists in the form of a string; in the second way, it is in the form of text immediately after the last "/" character. flags can be a combination of the following flag characters.
g is a global flag. If this flag is set, a search and replace operation on a text will affect all matching parts of the text. If this flag is not set, only the earliest match is searched and replaced.
i is a case-ignoring flag. If this flag is set, case will be ignored when doing match comparisons.
m is a multi-line flag. If this flag is not set, the metacharacter "^" will only match the beginning of the entire searched string, and the metacharacter "$" will only match the end of the searched string. If this flag is set, "^" can also be combined with "
"or"
" matches the position after (i.e. the beginning of the next line), and "$" can also match "
"or"
" matches the position after "(that is, the end of the next line).
Code 1.1 is an example of creating a regular expression.
Code 1.1 Create a regular expression: 1.1.htm
The running result of the above code is shown in Figure 8.1.
Since "" in the JavaScript string is an escape character, use explicit. When the constructor creates a RegExp instance object, the "" in the original regular expression should be replaced with "". For example, the two statements in Code 1.2 are equivalent.
The "" in Code 1.2 escapes. :1.2.htm
Since the escape character in the regular expression pattern text is also "", if the literal character "" is to be matched in the regular expression, it must be represented by "" in the regular expression pattern text. When using explicit construction When creating a RegExp instance object using a function, you need to use "\" to represent the literal character "".
var re = new RegExp(\\).
1 Properties of RegExp object
The properties of RegExp object are divided into static properties and instance properties. They are introduced separately below.
1.1 Static attributes
(1) index attribute. It is the starting position of the first matching content of the current expression pattern, counting from 0. Its initial value is -1, and the index attribute will change every time there is a successful match.
(2) input attribute. Returns the current string being acted on, which can be abbreviated as $_, and the initial value is the empty string "".
(3)lastIndex attribute. It is the next position of the last character in the content that the current expression pattern first matches. Counting starts from 0. It is often used as the starting position when continuing the search. The initial value is -1, which means that the search starts from the starting position, and every time it succeeds When matching, the lastIndex attribute value will change accordingly.
(4)lastMatch attribute. Is the last matching string of the current expression pattern, which can be abbreviated as $&. Its initial value is the empty string "". The value of the lastMatch attribute changes with each successful match.
(5)lastParen attribute. If there is an enclosed submatch in the expression pattern, it is the substring matched by the last submatch in the current expression pattern, which can be abbreviated as $. Its initial value is the empty string "". The value of the lastParen attribute changes with each successful match.
(6) leftContext attribute. It is everything to the left of the last matching string in the current expression pattern, which can be abbreviated as $` (where "'" is the backquote mark under "Esc" on the keyboard). The initial value is the empty string "". Each time there is a successful match, its property value changes.
(7) rightContext attribute. It is everything on the right side of the last matching string in the current expression pattern, which can be abbreviated as $'. The initial value is the empty string "". Each time there is a successful match, its property value changes.
(8) $1…$9 attributes. These properties are read-only. If there are enclosed submatches in the expression pattern, the $1...$9 attribute values are the contents captured by the first to ninth submatches respectively. If there are more than 9 submatches, the $1...$9 attributes correspond to the last 9 submatches respectively. In an expression pattern, you can specify any number of parenthesized submatches, but the RegExp object can only store the results of the last nine submatches. In the result array returned by some methods of the RegExp instance object, all submatch results within parentheses can be obtained.
1.2 Instance attributes
(1) global attribute. Returns the status of the global flag (g) specified when creating the RegExp object instance. If the g flag is set when creating a RegExp object instance, this property returns True, otherwise it returns False. The default value is False.
(2)ignoreCase attribute. Returns the status of the ignoreCase flag (i) specified when creating the RegExp object instance. If the i flag is set when creating a RegExp object instance, this property returns True, otherwise it returns False. The default value is False.
(3) multiLine attribute. Returns the status of the multiLine flag (m) specified when creating the RegExp object instance. If the m flag is set when creating a RegExp object instance, this property returns True, otherwise it returns False. The default value is False.
(4) source attribute. Returns the expression text string specified when creating the RegExp object instance.
RegExp object in JS (2)
2 Methods of RegExp object
Common methods of RegExp object include test, exec and compile, this section introduces the functions and usage of these methods. Finally, a comprehensive example is given of the properties and methods of the RegExp object.
2.1 test method
The syntax format is test(str). This method checks whether the expression pattern specified when creating a RegExp object instance exists in a string, and returns True if it exists, otherwise it returns False. If a match is found, the relevant static properties in the RegExp object are updated to reflect the match.
2.2 exec method
The syntax format is exec(str). This method searches a string using the expression pattern specified when creating the RegExp object instance and returns an array containing the search results.
If the global flag (g) is set for a regular expression, you can perform a continuous search in the string by calling the exec and test methods multiple times, each time starting the search for characters from the position specified by the lastIndex attribute value of the RegExp object string.
If the global flag (g) is not set, the exec and test methods ignore the lastIndex attribute value of the RegExp object and start searching from the beginning of the string.
If the exec method does not find a match, the return value is null; if a match is found, an array is returned, and the relevant static properties in the RegExp object are updated to reflect the match. Element 0 in the returned array contains the complete matching result, while elements 1 to n are the results of each submatch defined in the expression pattern.
The array returned by the exec method has three attributes, namely input, index and lastIndex.
The input attribute is the entire string being searched.
The index attribute refers to the position of the match in the entire searched string.
The lastIndex attribute refers to the character position next to the last character of the matched substring.
Code 2.1 is an application example of this method.
Code 2.1 exec() method application: 2.1.htm
2.3 compile method
The syntax format is compile("pattern"[,"flags"]). This method replaces the expression pattern used by the RegExp object instance and compiles the new expression pattern into an internal format, making subsequent matching processes faster. If an expression is to be reused in a loop, compiling it will speed up execution. However, there is no benefit from such compilation if the originally compiled expression pattern is used after any other expression pattern has been used in the program.
2.4 Comprehensive example
Code 2.2 is a comprehensive example of the RegExp object. Carefully analyzing the code and its operation results can help you better understand the RegExp object.
Code 2.2 Usage of RegExp object: 2.2.htm

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


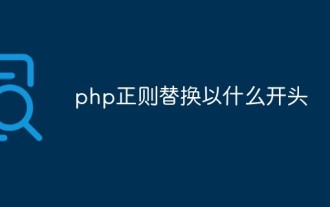
PHP regular expressions are a powerful tool for text processing and conversion. It can effectively manage text information by parsing text content and replacing or intercepting it according to specific patterns. Among them, a common application of regular expressions is to replace strings starting with specific characters. We will explain this as follows
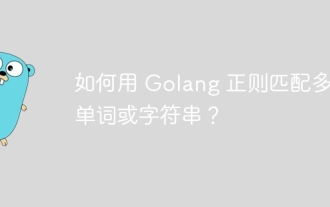
Golang regular expressions use the pipe character | to match multiple words or strings, separating each option as a logical OR expression. For example: matches "fox" or "dog": fox|dog matches "quick", "brown" or "lazy": (quick|brown|lazy) matches "Go", "Python" or "Java": Go|Python |Java matches words or 4-digit zip codes: ([a-zA
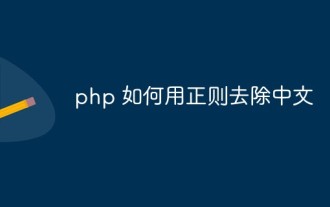
How to remove Chinese in PHP using regular expressions: 1. Create a PHP sample file; 2. Define a string containing Chinese and English; 3. Use "preg_replace('/([\x80-\xff]*)/i', '',$a);" The regular method can remove Chinese characters from the query results.
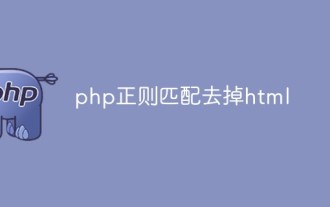
In this article, we will learn how to remove HTML tags and extract plain text content from HTML strings using PHP regular expressions. To demonstrate how to remove HTML tags, let's first define a string containing HTML tags.
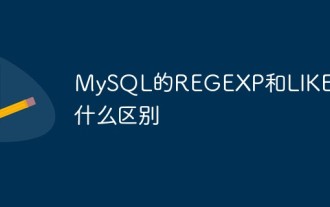
1. The difference in matching content. LIKE requires that the entire data must be matched. With Like, all the contents of this field must meet the conditions; REGEXP only requires partial matching, and only one fragment needs to be satisfied. 2. Differences in matching positions: LIKE matches the entire column. If the matched text appears in the column value, LIKE will not find it and the corresponding row will not be returned (unless wildcards are used); REGEXP is within the column value. A match is performed. If the matched text appears in the column value, REGEXP will find it, the corresponding row will be returned, and REGEXP can match the entire column value (same effect as LIKE). 3. The SQL statement returns data that differs from LIKE matching: the SQL statement
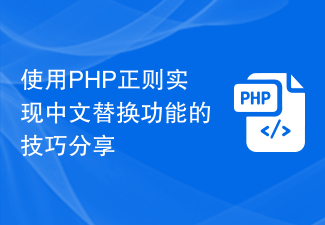
Sharing tips on using PHP regular expressions to implement the Chinese replacement function. In web development, we often encounter situations where Chinese content needs to be replaced. As a popular server-side scripting language, PHP provides powerful regular expression functions, which can easily realize Chinese replacement. This article will share some techniques for using regular expressions to implement Chinese substitution in PHP, and provide specific code examples. 1. Use the preg_replace function to implement Chinese replacement. The preg_replace function in PHP can be used
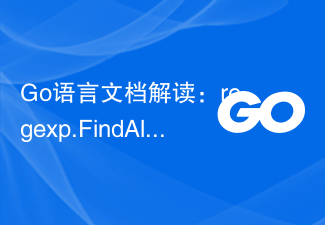
Go language document interpretation: Detailed explanation of regexp.FindAllString function Regular expressions play an important role in text processing. The Go language provides the regexp package to support regular expression operations. Among them, the regexp.FindAllString function has important functions. This article will explain the usage of this function and its corresponding code examples in detail. The function of regexp.FindAllString function is to search and return all strings with regular expressions in the provided string.
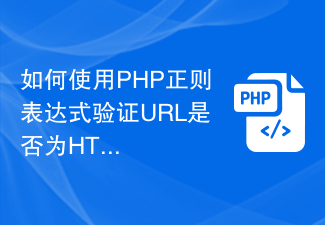
Website security has attracted more and more attention, and using the HTTPS protocol to ensure the security of data transmission has become an important part of current website development. In PHP development, how to use regular expressions to verify whether the URL is HTTPS protocol? here we come to find out. Regular expression Regular expression is an expression used to describe rules. It is a powerful tool for processing text and is widely used in text matching, search and replacement. In PHP development, we can use regular expressions to match http in the URL
