


JavaScript Advanced Programming (3rd Edition) Study Notes 2 js basic syntax_basic knowledge
This article reviews the basic syntax in the ECMAScript specification. Friends who are good at English can directly read the official document. JavaScript is essentially a C-like language. Friends who are familiar with C language can easily read this article or even skip it. However, it is recommended that you take a look. During the introduction, I may quote some It is a usage that I think is not easy to understand and is relatively popular.
Basic Grammar
1. Identifier: The so-called identifier actually refers to a name that meets certain specifications and can be recognized by the engine. It can be used to represent all nameable constants, variables, function names, function parameters, objects, object properties, etc. The name of the object.
(1) Case sensitive.
(2) Starts with a letter, underscore (_) or dollar sign ($), and other characters can be letters, underscores, dollar signs or numbers. The letters here contain extended ASCII or Unicode characters.
(3) Identifiers cannot be keywords, reserved words, true, false, or null. (Some browsers allow undefined, some don't).
(4) If the object attributes contain spaces or other special characters, they can be enclosed in parentheses as a whole.
2. Keywords: have specific uses in the language itself.
🎜> 3. Reserved words: reserved by the language itself and may be used as keywords in the future.
abstract boolean byte char class const debugger double enum export extends final float goto implementations import int interface long native package private protected public short static super syn chronized throws transient volatile
Reserved words in non-strict mode in ES5:
class const enum export extends import super
ES5 reserved words in strict mode:
implements interface let (new in ES5) package private protected public static yield (new in ES5)
4. Strict mode: Strict mode is introduced in ES5. Strict mode can be turned on by using "use strict". Global strict mode can be turned on at the top, or local strict mode can be turned on within the function scope.
5. Note: In ECMAScript, two types are supported Format comments, single-line comments and block-level comments:
Explanation: As JS code becomes more and more complex, comments become more and more important, and document automation becomes more and more important. Currently There are already many open source JS libraries used to automatically generate JS documents similar to Javadoc, such as JSDoc, YUIDoc, etc. At this time, there will also be corresponding format requirements for comments. Interested friends can find relevant information to study.
(1) Dynamic type: In ECMAScript, variables are dynamically typed. You can initialize them to a Number type when defining them. Then, you can assign a string value to it:
(2) var operator: Variables are declared using var. For uninitialized variables, they will default to undefined. You can also use variables directly without declaring them (in my opinion, this is also a feature that has no reason to exist. ), the most important difference between them is that when declared using var, the declared variable is only valid in the current scope, while when var is not used, the variable will be defined in the global scope. You can appreciate the difference through the following example:
var name = 'linjisong'; //Define global variables and assign values
age = 29; //Use variables directly, which is equivalent to defining global variables and assign values
//sal; //Error
var salary; / /Define global variables, not initialized
//This is just a function declaration, no actual call, so internally defined variables will not take effect
function fn(){
var name = 'oulinhai';//Definition Local variables and assign values
age = 23; //Assign values to global variables
work = 'it'; // Without using var, even variables local to the function will become global variables
}
//Before the function is actually called
console.info(salary); //undefined
console.info(name); // linjisong
console.info(age); // 29
try{
console.info(work);//Since work is not defined in the global environment, an exception will be thrown here
}catch(e){}
fn();//Actual call, Changes to variables in the code will appear
console.info(name); // linjisong, because var is used inside the function, the global name value will not be changed
console.info(age); // 23
console.info(work); // it
(3) Statement promotion: This issue will be discussed again when talking about function declarations and function expressions. Let’s mention it here first. , look at the code:
console.info(name);/ /undefined
console.info(getName);//getName() function
console.info(getName());//undefined
try{
console.info(age);// Exception
}catch(e){
console.info(e);//ReferenceError
}
console.info(getAge);//undefined
try{
console. info(getAge());//Exception
}catch(e){
console.info(e);//TypeError
}
var name = 'linjisong';//Variable declaration , raise
age = 29;//Use global variables directly, without raising
function getName(){//Function declaration, raise
return name;
}
var getAge = function( ){//Declaration of variable getAge, promoted; anonymous function expression to obtain age, not promoted
return age;
}
console.info(name);//linjisong
console.info (getName);//getName() function
console.info(getName());//linjisong
console.info(age);//29
console.info(getAge);// Anonymous function to get age
console.info(getAge());//29
Have you ever inferred the above output yourself? If you have already inferred it, you can skip it. If you still have questions, first take a look at the description of statement promotion below, and then go back and verify the above output results:
A. When the engine is parsing, it will first Parse the function declaration, then parse the variable declaration (the type will not be overwritten during parsing), and finally execute the code;
B. When parsing the function declaration, the type (function) will be parsed at the same time, but it will not be executed. When parsing the variable declaration, Only variables are parsed, not initialized.
What is involved here is only the global scope. There are also function parameters in the function scope that are also related to declaration promotion. We will discuss it later when we talk about functions.
The above code will first promote the function declaration on line 18 and the variable declaration on lines 16 and 21 to the beginning for parsing, and then execute it. Therefore, lines 1 and 9 output undefined because the variable declaration is promoted but not initialized, so line 11 throws a type exception because the function type cannot be determined; lines 2 and 3 are because the function declaration is promoted and the function type is parsed, so line 11 Line 2 outputs the function, line 3 can call the function, but the return value is not initialized and outputs undefined; line 5 will throw a reference exception because the variable has not been declared.
(4) You can use one statement to define multiple variables, just separate them with commas. For example:
var name='linjisong',
age=29,
work='it';
(5) In the strict mode of ES5, variables named eval or arguments cannot be defined.
7. Statement
(1) Statement: ends with a semicolon ";". If the semicolon is omitted, the parser determines the end of the statement.
I can’t think of any reason why statements in JS can omit semicolons. It is strongly recommended that each statement be clearly ended with a semicolon. Don’t let the parser spend time “guessing” your program. Moreover, More importantly, in many compression tools, guessing is not guaranteed to be 100% correct.
(2) Code block: Start with a left curly brace ({) and end with a right curly brace (}).
Although there is the concept of code block in JS, there is no corresponding block-level scope, which is different from general C-like languages. For control statements (such as if), don't avoid using code blocks just because there is only one statement. This will sow the seeds of mistakes for the people who maintain your program.
for(var i=0; i<10; i )
{
}
console.info(i);//Output 10, i can still be accessed after the code block, indicating that JS has no block-level scope
if(i < 10)
//console.info(i); Without using code blocks, it is easy to make mistakes during maintenance (such as adding a statement)
{
console.info(i);
}
In addition to being used as code blocks, curly braces ({}) also have a very important use in defining object literals, which will be discussed later.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


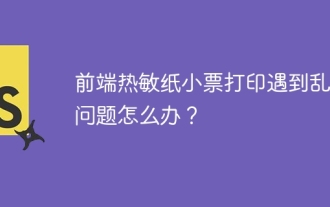
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
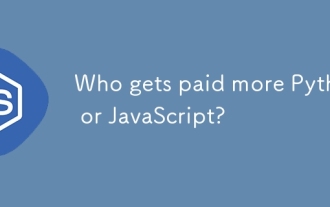
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
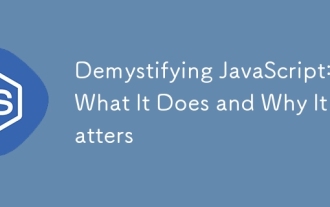
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
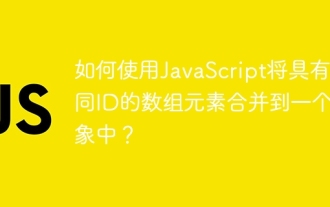
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
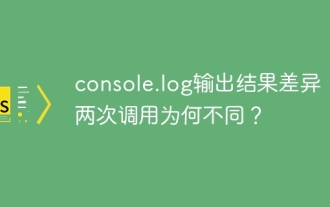
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
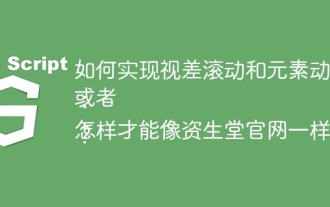
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
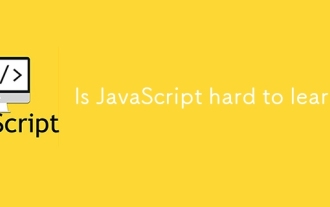
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
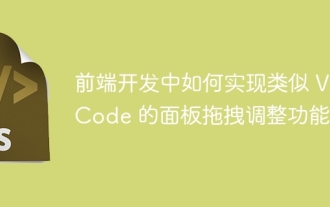
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
