Summary of JQuery code I have collected over the years_jquery
1. How to create nested filters
//Allow You filter by reducing the matching elements in the collection,
// leaving only those that match the given selector. In this case,
//The query deletes any child nodes that do not (:not) have (:has)
//contain the class "selected" (.selected).
.filter(":not(:has(.selected))")
2. How to reuse element search
var allItems = $("div.item");
var keepList = $("div#container1 div.item" );
//Now you can continue working with these jQuery objects. For example,
//Crop the "keep list" based on the check box, the name of the check box
//Conforms to
$(formToLookAt " input:checked").each( function () {
keepList = keepList.filter("." $(this).attr("name"));
});
3 . Any use of has() to check whether an element contains a certain class or element
//jQuery 1.4.* includes support for this has method. This method finds out
//whether an element contains another element class or anything else
//what you are looking for and want to operate on.
$("input").has(".email").addClass("email_icon");
4. How to use jQuery to switch style sheets
//Find out the media type (media-type) you want to switch, Then set the href to the new style sheet.
$('link[media="screen"]').attr('href', 'Alternative.css');
5. How to limit the selection range (based on Optimization purpose)
//Use tag names as much as possible Prefix the class name with
// so that jQuery doesn’t have to spend more time searching for the
// element you want. Another thing to remember is that the more specific the
//operations are for elements on your page, the more
//you can reduce execution and search time. var in_stock = $('#shopping_cart_items input.is_in_stock');
- Item X
- Item Y
- Item Z
6. How to use ToggleClass correctly
//The toggle class allows you to add or delete a class based on whether the class's
//exists.
//In this case some developers use: a.hasClass('blueButton') ? a.removeClass('blueButton') : a.addClass('blueButton');
//toggleClass allows you to use The following statement can do this easily
a.toggleClass('blueButton');
7. How to set IE-specific functions
if ($.browser.msie) {
// Internet Explorer is actually not that Easy to use
}
8. How to use jQuery to replace an element
$('#thatdiv').replaceWith('fnuh');
9. How to verify whether an element is empty
if ($('#keks').html().trim()) {
//Nothing found;
}
10. How to find the index number of an element from an unsorted set
$("ul > li").click(function () {
var index = $(this).prevAll().length;
});11. How to put The function is bound to the event
$('#foo').bind('click', function () {
alert('User clicked on "foo."');
});12 . How to append or add html to an element
$('#lal').append('sometext');
13. How to use object literals when creating elements ( literal) to define attributes
var e = $("" , { href: "#", class: "a-class another-class", title: "..." });
14. How to use multiple attributes for filtering
//When using many similar input elements with different types
//This precision-based method is useful var elements = $('#someid input[type=sometype][value=somevalue]').get();15. How to use jQuery to preload Image
jQuery.preloadImages = function () {
for (var i = 0; i < arguments.length; i ) {
$("
}
}; //Usage$.preloadImages('image1.gif', '/path/to/image2.png', 'some/image3. jpg');
16. How to set event handlers for any element that matches the selector
$('button.someClass').live('click', someFunction);
//Note that in jQuery 1.4.2, delegate and unelegate options
// were introduced instead of live as they provide better context support
// For example, in the case of tables, previously you would use
// .live()
$("table").each(function () {
$("td", this).live("hover", function () {
$(this).toggleClass("hover");
});
});
//Now use
$("table").delegate("td", "hover", function () {
$(this) .toggleClass("hover");
});
17. How to find an option element that has been selected
$('#someElement').find('option:selected');
18. How to hide an element that contains a certain value text
$ ("p.value:contains('thetextvalue')").hide();
19. If you automatically scroll to a certain area on the page
jQuery.fn.autoscroll = function (selector) {
$('html,body'). animate( { scrollTop: $(this ).offset().top },
500
);
}
//Then scroll to the class/area you want to go to like this .
$('.area_name').autoscroll();
20. How to detect various browsers
if( $.browser.safari) //Detect Safari
if ($.browser.msie && $.browser.version > 6) //Detect IE6 and later versions
if ($.browser.msie && $.browser.version <= 6) //Detect IE6 and earlier versions
if ($.browser.mozilla && $. browser.version >= '1.8' ) //Detect FireFox 2 and later versions
21. How to replace words in a string
var el = $('#id'); el.html(el.html().replace(/word/ig , ''));
22. How to disable right-click context menu
$(document).bind('contextmenu', function (e) {
return false ;
});
23. How to define a custom selector
$.expr[':'].mycustomselector = function(element, index , meta, stack){
// element- a DOM element
// index – the current loop index in the stack
// meta – metadata about the selector
// stack – to Stack of all elements in the loop
// Returns true if it contains the current element
// Returns false if it does not contain the current element };
// Usage of custom selector:
$( '.someClasses:test').doSomething(); 24. How to check whether an element exists
if ($('#someDiv' ).length) {
//Your sister, finally found it
}
25. How to use jQuery to detect right and left mouse clicks
$("#someelement").live('click', function (e) {
if ((!$.browser.msie && e .button == 0) || ($.browser.msie && e.button == 1)) {
alert("Left Mouse Button Clicked");
} else if (e.button == 2 ) {
alert("Right Mouse Button Clicked");
}
});
26. How to display or delete the default value in the input field
//This code shows that when the user does not enter a value,
//How to retain a default value in a text input field
//A default value
$(".swap").each(function (i) {
wap_val[i] = $(this ).val();
$(this).focusin(function () {
if ($(this).val() == swap_val[i]) {
$(this).val ("");
}
}).focusout(function () {
if ($.trim($(this).val()) == "") {
$( this).val(swap_val[i]);
}
});
});
27. How to automatically hide or close an element after a period of time (supported Version 1.4)
//This is what we have in 1.3.2 Use setTimeout to implement
setTimeout(function () {
$('.mydiv').hide('blind', {}, 500)
}, 5000);
// This is how you can use the delay() function in 1.4 (this is very similar to hibernation)
$(".mydiv").delay(5000).hide('blind', {}, 500);28. How to dynamically add created elements to DOM
var newDiv = $('');
newDiv.attr('id', 'myNewDiv').appendTo('body' );
29. How to limit the number of characters in the "Text-Area" field
jQuery.fn.maxLength = function (max) {
this.each(function () {
var type = this.tagName.toLowerCase() ;
var inputType = this.type ? this.type.toLowerCase() : null;
if (type == "input" && inputType == "text" || inputType == "password") {
this.maxLength = max;
}
else if (type == "textarea") {
this.onkeypress = function (e) {
var ob = e || event;
var keyCode = ob.keyCode;
var hasSelection = document.selection
? document.selection.createRange().text.length > 0
: this.selectionStart != this.selectionEnd;
return !(this.value.length >= max
&& (keyCode > 50 || keyCode == 32 || keyCode == 0 || keyCode == 13)
&& !ob.ctrlKey && !ob.altKey && !hasSelection);
};
this.onkeyup = function () {
if (this.value.length > max) {
this.value = this. value.substring(0, max);
}
};
}
});
}; //Usage$('#mytextarea').maxLength(500);
30. How to create a basic test for a function
//Put the test separately in the module
module("Module B");
test("some other test", function () {
//Indicate the internal expectations of the test How many assertions to run
expect(2);
//A comparison assertion, equivalent to JUnit’s assertEquals
equals(true, false, "failing test");
equals(true, true, "passing test");
}); 31. How to clone an element in jQuery
var cloned = $('#somediv').clone();
32. How to test whether an element is visible in jQuery
if ($(element).is(':visible') ) {
//The element is visible
}
33. How to put an element in Center position of the screen
jQuery.fn.center = function ( ) {
this.css('position', 'absolute');
this.css('top', ($(window).height() - this.height()) / $(window) .scrollTop() 'px');
this.css('left', ($(window).width() - this.width()) / 2 $(window).scrollLeft() 'px') ;
return this;
} //Use the above function like this: $(element).center();
34. How to return all elements with a specific name The values are placed in an array
var arrInputValues = new Array();
$("input[name='table[]']").each(function () {
arrInputValues.push($(this ).val());
} ; >
$.fn.stripHtml = function () {
var regexp = /<("[^"]*"|'[^']* '|[^'">])*>/gi;
Copy code
The code is as follows:
$('#searchBox').closest('div');
37. How to log jQuery events using Firebug and Firefox
// Usage:
$('#someDiv').hide() .log('div hidden').addClass('someClass');
Copy code
The code is as follows:
newwindow = window.open($(this).attr('href'), '', 'height=200,width=150');
if (window.focus) {
Copy code
The code is as follows:
newwindow = window.open($(this).href);
jQuery(this).target = "_blank";
Copy code
The code is as follows:
$('#nav li').click(function () {
$('#nav li').removeClass('active');
41. How to toggle all checkboxes on the page
var tog = false ;
// Or true if they are selected when loading
$('a').click(function () {
$( "input[type=checkbox]").attr("checked", !tog);
tog = !tog;
});
42. How to based on some input text To filter a list of elements
//If the value of the element and If the input text matches
//The element will be returned $('.someClass').filter(function () {
return $(this).attr('value') == $(' input#someId').val();
})
43. How to get the mouse pad cursor position x and y
$(document).ready(function () {
$(document).mousemove(function (e) {
$('#XY').html("X Axis : " e.pageX " | Y Axis " e.pageY);
});
});
44. How to make the entire List Element (LI) clickable
$("ul li").click(function () {
window.location = $(this).find("a").attr("href");
return false;
});
45. How to use jQuery to parse XML (basic example)
function parseXml(xml) {
//Find each Tutorial and print out the author
$(xml).find("Tutorial").each(function () {
$("#output").append($(this ).attr("author") "");
});
}
46. How to check if the image has been fully loaded
$('#theImage').attr('src', 'image.jpg') .load(function () {
alert('This Image Has Been Loaded');
});
47. How to use jQuery to specify a namespace for events
//The event can be bound to the namespace like this
$(' input').bind('blur.validation', function (e) {
// ...
});
//data method also accepts namespace
$( 'input').data('validation.isValid', true);
48. How to check whether cookies are enabled
var dt = new Date();
dt.setSeconds(dt.getSeconds() 60);
document.cookie = "cookietest=1; expires=" dt.toGMTString();
var cookiesEnabled = document.cookie.indexOf("cookietest=") != -1;
if (!cookiesEnabled) {
// Cookies are not enabled
}
49. How to expire cookies
var date = new Date();
date.setTime(date.getTime() (x * 60 * 1000));
$.cookie('example', 'foo', { expires: date });50. How to use a clickable link to replace any URL in the page
$.fn.replaceUrl = function () {
var regexp =
/( (ftp|http|https)://(w :{0,1}w*@)?(S )(:[0-9] )?(/|/([w#!:.? =&% @!-/]))?)/gi;
this.each(function () {
$(this).html(
$(this).html().replace(regexp, ' $1')
);
});
return $(this);
} //Usage $('p' ).replaceUrl();
It’s finally finished. Typesetting is also a laborious task. The resources are compiled from the Internet and are given to those children’s shoes who have not collected them. If you have already collected them, please do not throw bricks.
(Thank you for your feedback, the errors have been corrected, I hope I didn’t mislead you)
PS: Due to the correction of errors, the layout is messed up, so I am re-posting it now.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


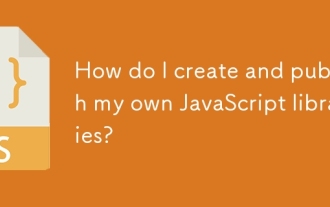
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
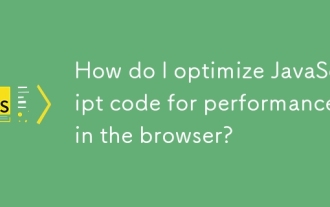
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
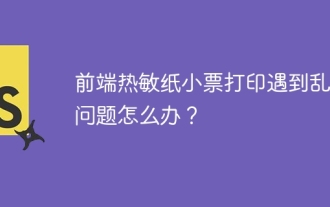
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
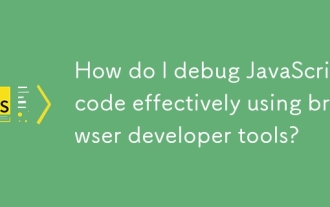
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
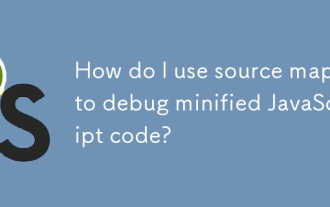
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
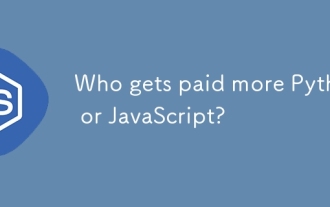
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
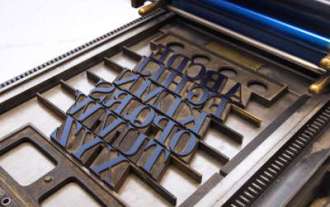
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
