


A brief discussion on Javascript mouse and wheel events_javascript skills
May 16, 2016 pm 05:52 PMMouse event may be the most commonly used event in web pages, because the mouse is the most commonly used navigation device. There are 9 mouse events defined on DOM3 level events. They are:
Click: Triggered when the user clicks the primary mouse button, usually the left mouse button or presses the Enter key.
dbclick: Triggered when the user double-clicks the primary mouse button. This event is not defined in DOM2 level events but is generally supported, and was later standardized in DOM3 level.
Mousedown: It will be triggered when the user presses any mouse button. This event cannot be triggered through the keyboard.
Mouseenter: Triggered when the mouse icon moves from outside the element to within the element boundary. This event does not support bubbling and is triggered when the mouse moves on the upper surface of the element. This event does not belong to DOM2 level event, but is an event added after DOM level 3. IE, FF9, and opera support this event.
mouseleave: This event is triggered when the mouse is over the element and then moves out of the element boundary. It is the same as the mouseenter event. It does not support bubbling. It is not triggered above the element. This event does not belong to DOM2 level events, but belongs to DOM3. An event added later, IE, FF9, and opera support this event.
Mousemove: Triggered repeatedly when the mouse moves around an element. This event cannot be triggered through keyboard events.
Mouseout: Triggered when the mouse is over an element and then moves over other elements. The element moves outside the bounds of the original element, or onto a child element of the original element. This event cannot be triggered by the keyboard.
Mouseover: Triggered when the user moves the mouse from outside the boundary of an element to within the boundary of the element for the first time. This event cannot be triggered through the keyboard.
Mouseup: Triggered when the user releases the mouse button. This event cannot be triggered through the keyboard.
All elements on the page support mouse events. Except for mouseenter and mouseleave, all events bubble up, and their default behaviors can be canceled. But canceling the default behavior of mouse events may affect other events, because some mouse events are dependent on each other.
Only when a mousedown event and a mouseup event are triggered on the same element can the mouse click event be triggered; assuming either event is canceled, the click event will never be triggered. A similar principle is that the dbclick event depends on the click event. If either of the two consecutive click events is canceled, dbclick will not be triggered. Commonly used mouse events are as follows:
1.mousedown, 2.mouseup, 3.click, 4.mousedown, 5.mouseup, 6.click, 7.dbclick.
Whether it is click or dbclick event, both depend on the triggering of other events.
You can use the following code to determine whether the browser supports mouse events on dom2 events,
var isSupport = document.implementation.hasFeature('MouseEvents', '2.0');
However, it is worth noting that the detection on dom3 level events is somewhat different:
var isSupport = document.implementation.hasFeature('MouseEvent','3.0');
The mouse event also contains a subclass event, the wheel event (wheel event). The wheel event contains only one event, the mousewheel event, which reflects the interaction of the mouse wheel or other devices, such as the Mac's touchpad.
b) Associated elements
For mouseover and mouseout events, there are also event-related elements. The actions performed by these events include, move The mouse moves from inside the bounds of one element to inside the bounds of another element. Taking the mouseover event as an example, its main target element is the element that the mouse will move to, and the associated element is the element that loses the mouse. Similarly for the mouseout event, the main target is the element that the mouse moves out, and the related element is the element that gets the mouse. The DOM provides information about the related element through the relatedTarget attribute on the event object. IE8 or earlier versions of IE do not support the relatedTarge attribute. , but provides the fromElement attribute and toElement attribute with similar functions. Under IE, when the mousemove event is triggered, the fromElement of the event object contains the associated element. When the mouseout event is triggered, the toElement attribute of the event contains the associated element. All attributes are supported in IE9. A cross-browser getRelatedTarget method can be written like this:
var eventUtil = {
getRelateTarget:function(event){
if (event.relatedTarget) {
return event.relatedTarget;
}else if(event.fromElement) {
return event.fromElement;
}else if(event.toElement){
return event.toElement;
}else {
return null;
}
}
};
c)buttons
The click event will only be triggered when the mouse primary button clicks on an element (or when an element gets focus and presses Enter key), for mousedown and mouseup, there is an attribute button on the event object event, which can determine which key is pressed or released. There are usually three possible button attribute values implemented in the DOM:
0: represents the primary key,
1: represents the scroll wheel,
2: represents the secondary key.
Generally speaking, the primary key refers to the left mouse button, and the secondary key represents the right mouse button.
Starting from IE8, button attributes are also provided, but they have completely different value ranges:
0: No button is pressed,
1: The primary key has been pressed down,
2: represents the secondary key has been pressed,
3: both the main key and secondary keys have been pressed,
4: represents the middle key has been pressed,
5: Indicates that the primary key and middleware are pressed,
6: Indicates that the secondary key and middle key are pressed,
7: Indicates that all three keys are pressed .
It can be seen that the value range of the button attribute under the DOM model is much simpler than the value range under the IE model, and I personally feel that the operation situation under IE is slightly abnormal.
d) Other event information
On DOM2 level events, the detail attribute is also provided for the event object event to provide more event information, such as click events For example, detail can record the number of clicks at the same pixel position. The value of detail is counted from 1, and one is added after each click. If the mouse moves between mousedown and mouseup, this value will be cleared.
I will write these about mouse events first, and will slowly complete them in the future.

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
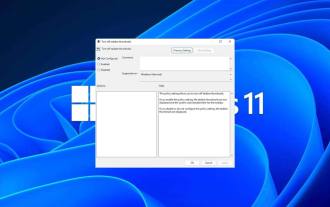
How to disable taskbar thumbnail preview in Win11? Turn off the taskbar icon display thumbnail technique by moving the mouse
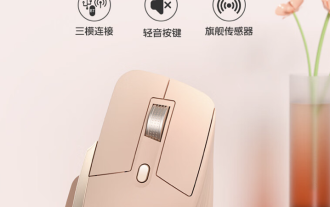
HP launches Professor 1 three-mode soft mouse: 4000DPI, Blue Shadow RAW3220, initial price 99 yuan
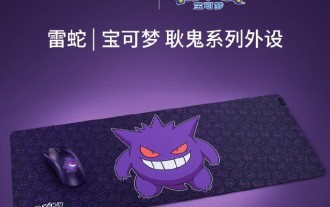
Razer | Pokémon Gengar wireless mouse and mouse pad are now available, with a set price of 1,549 yuan
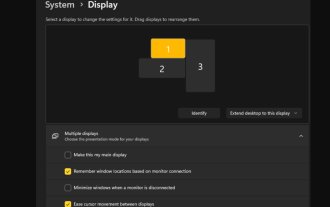
Turn Easy T cursor movement between monitors on or off on Windows 11
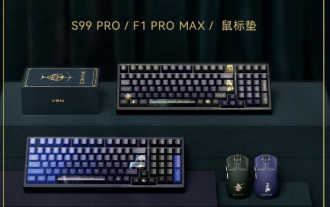
VGN co-branded 'Elden's Circle' keyboard and mouse series products are now on the shelves: Lani / Faded One custom theme, starting from 99 yuan
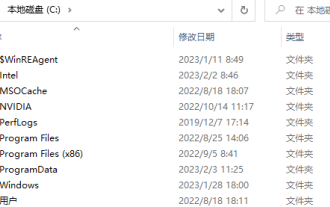
In which folder is the Razer mouse driver located?
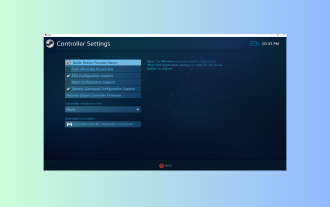
Windows 11 controller behaves like mouse? how to stop it
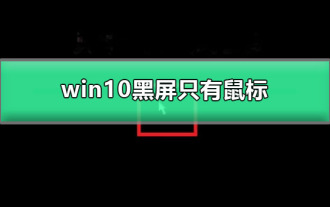
Windows 10 only shows black screen and mouse after logging in
