Analysis of doubts about the getAll method in jQuery 1.7.2_jquery
The getAll method is private and in the manipulation module. The code only has a few simple lines, as follows
function getAll( elem ) {
if ( typeof elem.getElementsByTagName !== "undefined" ) {
return elem.getElementsByTagName( "*" );
} else if ( typeof elem.querySelectorAll !== "undefined" ) {
return elem.querySelectorAll( "*" );
} else {
return [];
}
}
You can know this method from the function name Used to get all child elements of the passed in HTML element. There are three internal branches
1. First determine whether elem has a getElementsByTagName method. If so, use the getElementsByTagName method to obtain all child elements and return.
2. GetElementsByTagName is not supported. Then determine whether elem supports the querySelectorAll method. If it supports using the querySelectorAll method to obtain the element's sub-elements, it will return.
3, getElementsByTagName and querySelectorAll are not supported and return an empty array.
When I looked at this code, I was confused and felt that the second branch was a bit redundant.
1, getElementsByTagName is an API in DOM Level 2 (earlier). All current browsers should already support it. Since they are all supported, there will be no need to enter the second one. branched and returned directly. Isn't the following code redundant?
2. querySelectorAll is an API in DOM Level 3 (newer) and is not supported by IE6/7.
After seeing this, do you think the last two branches are redundant? Or can you find a reason why it is not redundant? That is, just find the element elem that meets the following conditions.
"elem does not have a getElementsByTagName method, but it has a querySelectorAll method"
After many searches, the answer was finally found through discussion (discovered by Xiaoniu). DocumentFragment meets this condition.
var frag = document.createDocumentFragment();
alert('getElementsByTagName' in frag);
alert('querySelectorAll' in frag);
The above code pops up false in IE9/Chrome/Safari/Firefox/Opera. , true.
No more explanation at this point.
Note: Several special points of the DocumentFragment object
1. IE6/7/8 has the createElement method, but other browsers (IE9/10/Safari/Chrome/Firefox/Opera) do not
2. There is no getElementsByTagName method in IE9/10/Firefox/Safari/Chrome/Opera, but there is a querySelectorAll method.
Related:
http://www.jb51.net/article/30352.htm
https://developer.mozilla.org/en/DOM/document .createDocumentFragment
https://developer.mozilla.org/En/DOM/DocumentFragment
http://www.w3.org/TR/DOM-Level -3-Core/core.html#ID-B63ED1A3

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
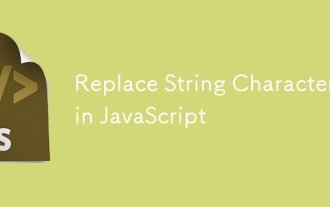
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
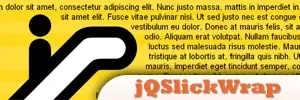
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
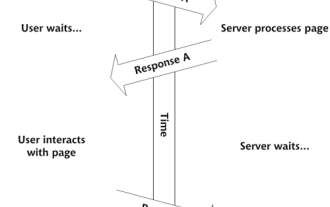
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
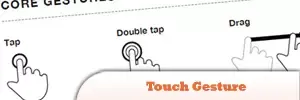
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig
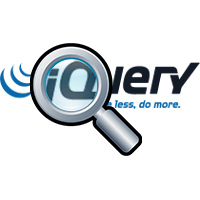
jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
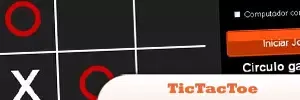
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
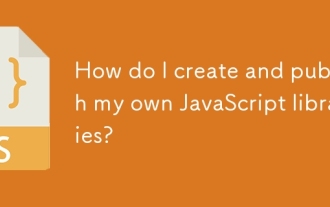
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
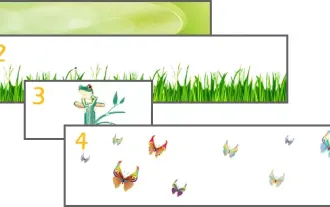
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
