Let’s first look at the rendering of the layer to be dragged (simulation window).
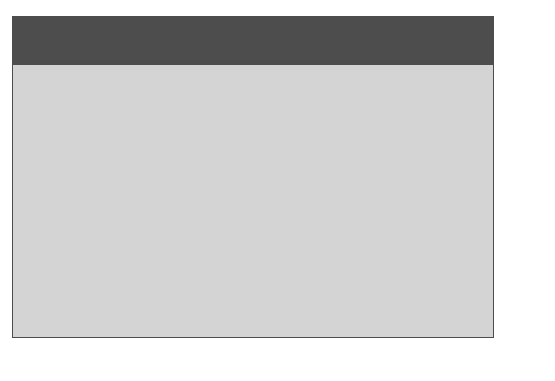
To achieve the dragging effect: press the left mouse button on the title bar above the window, move the mouse at the same time, and the window will move accordingly.
Window:
A little preparation:
Let the window be free Move, then the position of the window should be absolute;
Add a title bar to the window. Here, a layer placed on the top of the window is used, and the mouse cursor of the title bar is set to drag (move). ) shape (when dragging in chrome, the cursor will turn into a text cursor, and will recover after releasing the mouse button).
#win {
position:absolute;
width:480px;
height:320px;
background-color:#d4d4d4;
border: 1px solid #4d4d4d;
}
#win_header {
width:480px;
height:48px;
background-color:#4d4d4d;
cursor:move;
}
Define a utility function to get the element with the specified ID attribute:
function $id(id) {
return document .getElementById(id);
}
Define a browser core identity isIE:
var isIE = (window.navigator.userAgent.indexOf("IE") == -1 ) ? false : true;
Get the window element and its title bar:
var win = $id("win");
var header = $id("win_header");
In order to conveniently record the position information of the mouse and window, Create a position:
var pos =function(x, y ) {
this.x = x;
this.y = y;
};
Set an initial position for the window (left value and top value of css).
I don’t know why here. If you don’t use js to set these two attributes, you can’t get the value, and it won’t work if you specify them in CSS.
var originalpos = new pos(20, 20);
During the process of dragging the window, the values that need to be recorded are:
The position of the mouse cursor when the mouse is pressed
var oldmouse =new pos(0, 0);
The position of the window when the mouse is pressed
var oldpos = new pos(0, 0);
The new position of the window when the mouse moves
var newpos = new pos(0, 0);
Set the initial position of the window
win.style.left = originalpos.x "px";
win .style.top = originalpos.y "px";
Because of the differences in browsers (IE and non-IE), the methods of binding event handlers to elements are different (IE uses attachEvent, non-IE IE uses addEventListener), in order to simplify the event binding operation, define an event binding function:
function bind(ev, func) {
if(isIE) {
header.attachEvent("on" ev, func);
} else {
header. addEventListener(ev, func, false);
}
}
After doing these things, you can start processing mouse events.
In this program, you only want the left mouse button to drag the window, and no other keys can, so you need to determine whether the left mouse button is pressed. This judgment will be used in several functions, so it is extracted into a function and judged by the passed in parameters (mouse key value, that is, which key was pressed). Here, you need to pay attention to the differences between browsers: the value of the left mouse button in IE is 1, while the value in non-IE is 0.
function isLeftButton(btn) {
if(isIE) {
if(btn == 1)
return true;
else
return false;
} else {
if(btn == 0)
return true;
else
return false;
}
}
Drag action is done by pressing and moving the left mouse button. To share this action, the mouse first triggers the press action (mousedown), and then triggers the move action (mousemove). In order to determine whether it is really dragging or just the mouse passing over the window, set a variable to record the mouse down state:
var mousedown = false;
Due to compatibility issues in CSS, use here js to control the color change when the mouse is hovering over the window title bar.
Hovering
function over(e){
Header.style.backgroundColor = "#5d5d5d";
}
Leave
function out(e) {
header.style.backgroundColor = "#4d4d4d";
// Sometimes the mouse will not be released Leave the window,
// At this time, the window is released from the control of the mouse by triggering the mouse release event
up(e);
}
Press
In the press event, you need to first determine whether the left button of the mouse is pressed;
If so, the position of the mouse and window at this time will be recorded, otherwise it will not be recorded.
function down(e) {
e = e || event;
if(!isLeftButton(e.button))
return;
mousedown = true;
oldmouse.x = e.clientX;
oldmouse.y = e.clientY ;
oldpos.x = parseInt(win.style.left.replace("px", ""));
oldpos.y = parseInt(win.style.top.replace("px", "" ));
}
Release
function up(e) {
if(!isLeftButton(e.button))
return;
mousedown = false;
}
Moving
involves two mouse events:
press and move. The movement action is valid only when the left mouse button is pressed.
The new position of the window is determined by the movement distance of the mouse in the dragging state (the distance between X and Y). That is:
The new mouse position is sent to the position recorded when the left button is pressed, and a distance is obtained. Then the position of the window is added to the distance moved by the mouse to obtain the new position of the window.
function move(e) {
if(! isLeftButton(e.button))
return;
if(mousedown) {
e =e || event;
newpos.x = e.clientX - oldmouse.x;
newpos. y = e.clientY - oldmouse.y
win.style.left = (oldpos.x newpos.x) "px";
win.style.top = (oldpos.y newpos.y) "px" ;
}
}
Event processing has been written. Finally, let’s bind the elements. Amen!
bind("mouseover", over);
bind("mouseenter", over);
bind("mouseout", out);
bind("mouseleave", out);
bind("blur", out);
bind( "mousedown", down);
bind("mouseup", up);
bind("mousemove", move);
But there is a problem with dragging in FF. I can only drag it normally for the first time, but it gets a little messy after that!