First of all, the framework used is of course my framework mass Framework. Of course, you can also use other frameworks, such as jQuery. There is nothing complicated. As long as you understand the principle, you can do it in no time. Presumably, you will also encounter the task of building a search box in your future work.
Since I don’t have a backend, I use an object as a local database. What I want to do now is actually far more advanced than suggestion, something similar to the syntax prompts of an IDE. The current finished product has been put on github.
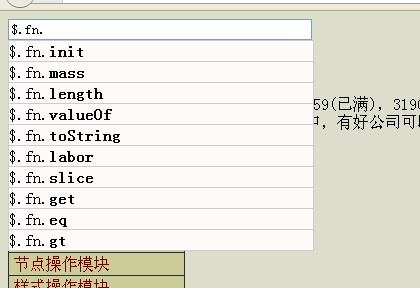
Okay, let’s do it. The first is the structural layer. Students who have installed FF can view the source code on the Baidu homepage. When a few letters are entered, the HTML will be dynamically generated. But no matter what, the result is that a DIV is placed below the search bar, with a table inside, and the table dynamically stores candidate words. And if the candidate words are not part of the user input, that is, the parts automatically added by JS, it will put them in a b tag and display them in bold. However, I felt that using table was too heavy-duty, so I used a ul list instead. In order to make IE6 also support the skimming color effect, I also put an a tag in it. In order to make it easier to pick words, I also added an attribute to it (a tag), which is specially used to store the vocabulary after the completion. It probably looks like this:
Look at the structure. It is actually two parts. div#search_wrapper is visible and div#suggest_wrapper is "invisible" (as long as there is no li element in it, it does not take up space and cannot be displayed). The input search box has an attribute autocomplete, which is used to turn off the prompt function that comes with the browser. Regarding data-value, this naming method is recommended by HTML5 and is used to define the data to be cached. Data-* will be placed in an object called dataset in cutting-edge browsers. For example:
data-drink="coffee"
data-meal-time="12:00">12:00
We can access it in the following ways:
var el= document.getElementById('Situ Zhengmei') ;
alert( el.dataset.drink );
alert( el.dataset.mealTime );
Of course, you can also directly take the innerText or textContext.
Note: Complete vocabulary = user input part auto-suggestion part. Therefore, you should not add so many things in the a tag to prevent spaces or other things from appearing, causing the retrieval to fail!
Then there is the style part, but I won’t go into details. Very simple:
#search_wrapper {
height:50px ;
}
#search{
width:300px;
}
#suggest_wrapper{
position:relative;
}
#suggest_list{
position: absolute;
z-index:100;
list-style: none;
margin:0;
padding:0;
background:#fffafa;
border:1px solid # ccc;
border-bottom:0 none;
}
#suggest_list li a{
display: block;
height:20px;
width:304px;
color: #000;
border-bottom:1px solid #ccc;
line-height:20px;
text-decoration: none;
}
#suggest_list li a:hover, .glow_suggest {
background:#ffff80;
}
Okay, let’s get to the point. Since I don't have a backend, I want to use a local object as a local database. This object is of course a JS object. The objects we traverse are usually obj.aaa.bbb.ccc. If we keep clicking in this way, in fact, every time we reach a dot number, we use a for in loop to traverse. Therefore, we monitor the input of text content, obtain the content of the input box once it changes, and then compare it in the for in loop. If it is an attribute that starts with this input value, take it out and put it into an array until you get ten. Then splice the contents of these arrays into the li element format depicted above and paste them into the ul element. Among them, we also need to pay attention to the dots. If we enter the dot number at the beginning, we will take the ten attributes of the window object. When we encounter the dot number in the future, we will switch this object.
Okay, let’s start writing code. Since my framework is used, you can go here. There is a README on the project homepage, which teaches you how to install the micro.Net server and view documents. At the beginning, you can think of it as jQuery with the module loading function added. The API is 90% similar. We need to use its event module and attribute module. It will load the relevant dependencies, add the ready parameter, and it will be executed after domReady. After we select the input box, we bind an input event to it. This is an event supported by standard browsers. My framework is already compatible under IE. Students who use jQuery and native please use the propertychange event to simulate it.
//by Situ Zhengmei
$.require( "ready,event,attr",function(){
var search = $("#search"), hash = window, prefix = "", fixIE = NaN;
search.addClass("search_target") ;
search.input(function(){//Monitor input
var
input = this.value,//Original value
val = input.slice( prefix.length),//Compare Value
output = []; //Used to place the output content
if( fixIE === input){
return //IE fix will be triggered even if the value in the input box is changed through the program The propertychange event prevents us from flipping up and down
}
for(var prop in hash){
if( prop.indexOf( val ) === 0 ){//Get the index starting with the input value API
if( output.push( '
' input " " (prefix prop ).slice( input.length ) "" ) == 10){
break;
}
}
}
//If a dot is encountered forward, or a dot is canceled backwards
if( val.charAt(val.length - 1) === "." || (input && !val) ){
var arr = input.split("."); hash = window;
for(var j = 0; j < arr.length; j ){
var el = arr[j ];
if(el && hash[ el ]){
hash = hash[ el ];//Reset the object to traverse the API
}
}
prefix = input == "." ? "" : input;
for( prop in hash){
if( output.push( '
' input "" (prefix prop ).slice( prefix.length ) "break;
}
}
}
$("#suggest_list").html( output.join("") );
if(!input){//Reset all
hash = window;
fixIE = prefix = output = [];
}
});
});
When the prompt list comes out, we monitor the up and down effect. That is, when you click the direction key on the keyboard, the prompted item will be highlighted up and down, and it will be filled in the search box. At this time, you need to bind the keyup event and check its keyCode. Standard browsers call it which. You can read my blog post "
Javascript Keyboard Event Summary". The implementation principle is very simple. Define a peripheral variable to store the highlighted position (index value), then decrease it by one when scrolling up, and increase it by one when scrolling down. Then get all a tags in the prompt list. Use the index value to locate a certain a tag, highlight it, and then remove the originally highlighted a tag.
//by Situ Zhengmei
$.require("ready,event,attr",function(){
var search = $("#search"), hash = window, prefix = "";
search.input(function(){//Monitor input
//.....
});
var glowIndex = -1;
$(document) .keyup(function(e){//Monitor up and down
if(/search_target/i.test( e.target.className)){//Only proxy specific elements to improve performance
var upOrdown = 0
if(e.which === 38 || e.which === 104){ //up 8
upOrdown --;
}else if(e.which === 40 || e .which === 98){//down 2
upOrdown ;
}
if(upOrdown){
var list = $("#suggest_list a");
//Transfer Highlighted column
list.eq(glowIndex).removeClass("glow_suggest");
glowIndex = upOrdown;
var el = list.eq( glowIndex ).addClass("glow_suggest");
fixIE = el.attr("data-value")
search.val( fixIE )
if(glowIndex === list.length - 1){
glowIndex = -1;
}
}
}
});
});
Finally, press Enter to submit. I wrote another keyup event. Of course, you can try to combine two keyups into one (monitoring window). I wrote it this way purely for teaching purposes.
//by Situ Zhengmei
$.require( "ready,event,attr",function(){
var search = $("#search"), hash = window, prefix = "";
search.input(function(){//Listen to input
//.....
});
var glowIndex = -1;
$(window).keyup(function(e){//Listen for up and down scrolling
// .....
});
search.keyup(function(e){//Listen for submission
var input = this.value;
if(input && (e.which == 13 || e.which == 108)){ //If you press the ENTER key
alert(input)//In the actual project, the page should jump and go to the search results page }
});
});
At this point, the suggestion effect is completed. If you have downloaded my framework, you can see this effect by turning on the server and opening the document homepage. In actual projects, suggestion is actually simpler. When the input box text changes, AJAX requests an array in the background, and then splices it into the format of the li element.