Summary of js function calling patterns_js object-oriented
When a function is saved as a property of an object, we call it a method of the object, then this is bound to the object.
var myObject={
name : "myObject" ,
value : 0 ,
increment : function(num){
this.value = typeof(num) === 'number' ? num : 0;
} ,
toString : function(){
return '[Object:' this.name ' {value:' this.value '}]';
}
}
alert(myObject);//[Object: myObject {value:0}]
Function calling mode
When a function is not a function of an object, then it is called as a function, and this is Bind to the global object. This is a mistake in language design. If the language design is correct, when the inner function is called, this should still be bound to the this variable of the outer function. For example:
var myObject={
name : " myObject" ,
value : 0 ,
increment : function(num){
this.value = typeof(num) === 'number' ? num : 0;
} ,
toString : function(){
return '[Object:' this.name ' {value:' this.value '}]';
},
getInfo:function(){
return ( function(){
return this.toString();//this in the internal anonymous function points to the global object window
})();
}
}
alert(myObject.getInfo ());//[object Window]
Luckily, there is an easy solution: define a variable and assign it the value this, then the internal function can access it through this variable This points to the object, such as:
var myObject={
name : "myObject" ,
value : 0 ,
increment : function(num){
this.value = typeof(num) === 'number' ? num : 0;
} ,
toString : function(){
return '[Object:' this.name ' {value:' this.value '}]';
},
getInfo:function() {
var self=this;
return (function(){
return self.toString(); // Point to the myObject object through the variable self
})();
}
}
alert(myObject.getInfo());//[Object:myObject {value:0}]
Constructor calling mode
JavaScript is A language based on prototypal inheritance. This means that objects can inherit properties directly from other objects. The language is classless.
If a function is called with new in front, a new object will be created that hides the prototype member connected to the function, and this will be bound to the instance of the constructor.
function MyObject(name){
this.name =name || 'MyObject';
this.value=0;
this.increment=function(num){
this.value = typeof(num) === 'number' ? num : 0 ;
};
this.toString=function(){
return '[Object:' this.name ' {value:' this.value '}]';
}
this .target=this;
}
MyObject.prototype.getInfo=function(){
return this.toString();
}
/*
Create a MyObject.prototype at the same time Object, myObject inherits all properties of MyObject.prototype,
this is bound to the instance of MyObject
*/
var myObject=new MyObject();
var otherObject=new MyObject() ;
//alert(myObject.target===myObject);//ture
//alert(myObject.target.getInfo());//[Object:MyObject {value:0}]
myObject.increment(10);
otherObject.increment(20);
alert(myObject.value);//10
alert(otherObject.value);//20
Apply calling mode
JavaScript is a functional object-oriented programming language, so functions can have methods.
The apply method of the function, as if the object has this method, makes the object have this method. At this time this points to the object.
apply receives two parameters, the first is the object to be bound (the object pointed to by this), and the second is the parameter array.
function MyObject(name){
this.name=name || 'MyObject';
this.value=0;
this.increment=function(num){
this.value = typeof(num) === 'number' ? num : 0;
};
this.toString=function(){
return '[Object:' this.name ' {value:' this.value '}]';
}
this.target=this;
}
function getInfo(){
return this.toString();
}
var myObj=new MyObject();
alert(getInfo.apply(myObj));//[Object:MyObject {value:0}],this指向myObj
alert(getInfo.apply(window));//[object Window],this指向window

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


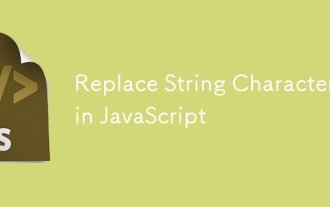
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
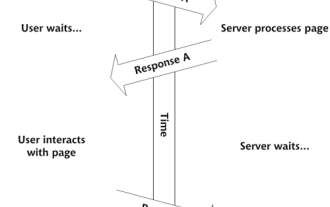
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
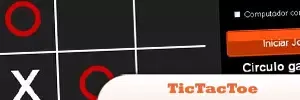
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
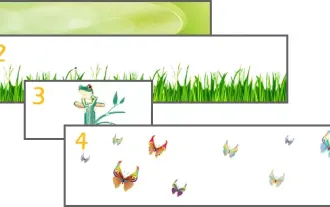
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
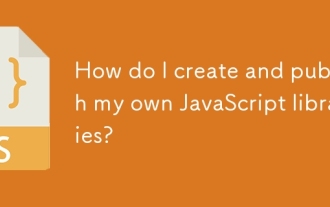
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
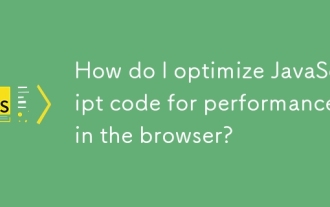
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
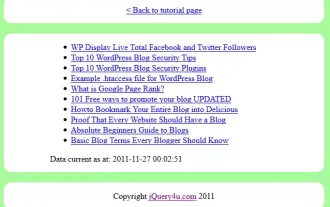
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona
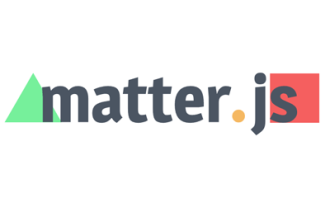
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
