


Javascript object-oriented design 1 factory pattern_js object-oriented
1. The factory pattern abstracts the process of creating specific objects, but classes cannot be created in ECMAScript, so a function is used to encapsulate the details of creating objects with a specific interface. Take the following situation as an example,
has an employee class with name, age, and position attributes,
var Emp = new Object();
Emp.name = name;
Emp.age = age;
Emp .job = job;
Emp.sayName = function () {
alert(this.name);
};
return Emp;
}
Use the above method to define two employees, Jim, Sun
var Jim = CreateEmployee("jim", 22, "SoftWare Engineer");
var Sun = CreateEmployee("Sun",24,"Doctor");
Then use the SayName method respectively, Let two employees sign up
Jim.sayName( );
Sun.sayName();
The function CreateEmployee can construct an Employee object containing necessary information based on the parameters, and this function can be called unlimited times. Although the factory pattern solves the problem of creating multiple similar objects, it does not solve the problem of how to know the type of an object.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


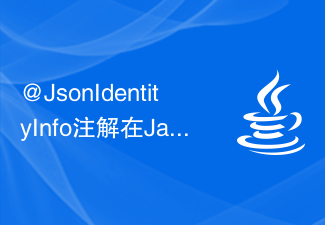
The @JsonIdentityInfo annotation is used when an object has a parent-child relationship in the Jackson library. The @JsonIdentityInfo annotation is used to indicate object identity during serialization and deserialization. ObjectIdGenerators.PropertyGenerator is an abstract placeholder class used to represent situations where the object identifier to be used comes from a POJO property. Syntax@Target(value={ANNOTATION_TYPE,TYPE,FIELD,METHOD,PARAMETER})@Retention(value=RUNTIME)public
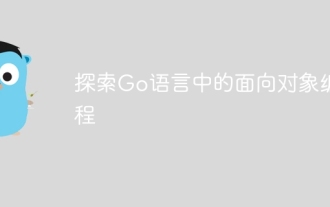
Go language supports object-oriented programming through type definition and method association. It does not support traditional inheritance, but is implemented through composition. Interfaces provide consistency between types and allow abstract methods to be defined. Practical cases show how to use OOP to manage customer information, including creating, obtaining, updating and deleting customer operations.
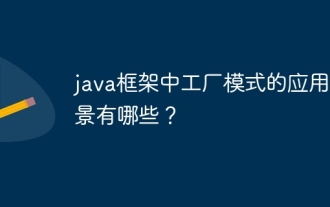
The factory pattern is used to decouple the creation process of objects and encapsulate them in factory classes to decouple them from concrete classes. In the Java framework, the factory pattern is used to: Create complex objects (such as beans in Spring) Provide object isolation, enhance testability and maintainability Support extensions, increase support for new object types by adding new factory classes
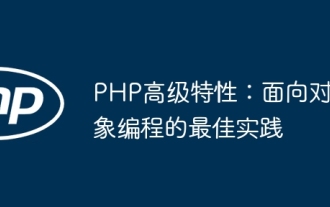
OOP best practices in PHP include naming conventions, interfaces and abstract classes, inheritance and polymorphism, and dependency injection. Practical cases include: using warehouse mode to manage data and using strategy mode to implement sorting.

The Go language supports object-oriented programming, defining objects through structs, defining methods using pointer receivers, and implementing polymorphism through interfaces. The object-oriented features provide code reuse, maintainability and encapsulation in the Go language, but there are also limitations such as the lack of traditional concepts of classes and inheritance and method signature casts.
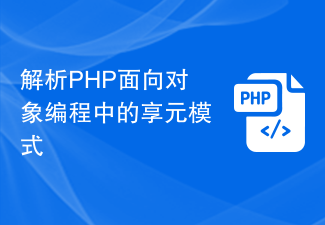
Analyzing the Flyweight Pattern in PHP Object-Oriented Programming In object-oriented programming, design pattern is a commonly used software design method, which can improve the readability, maintainability and scalability of the code. Flyweight pattern is one of the design patterns that reduces memory overhead by sharing objects. This article will explore how to use flyweight mode in PHP to improve program performance. What is flyweight mode? Flyweight pattern is a structural design pattern whose purpose is to share the same object between different objects.
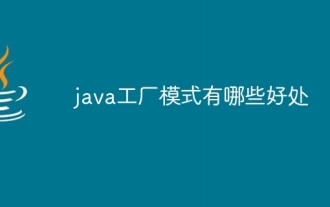
The benefits of the Java factory pattern: 1. Reduce system coupling; 2. Improve code reusability; 3. Hide the object creation process; 4. Simplify the object creation process; 5. Support dependency injection; 6. Provide better performance; 7. Enhance testability; 8. Support internationalization; 9. Promote the open and closed principle; 10. Provide better scalability. Detailed introduction: 1. Reduce the coupling of the system. The factory pattern reduces the coupling of the system by centralizing the object creation process into a factory class; 2. Improve the reusability of code, etc.
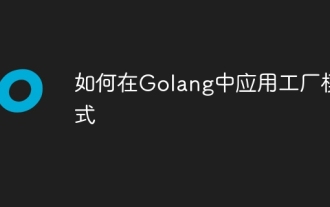
Factory Pattern In Go, the factory pattern allows the creation of objects without specifying a concrete class: define an interface (such as Shape) that represents the object. Create concrete types (such as Circle and Rectangle) that implement this interface. Create a factory class to create objects of a given type (such as ShapeFactory). Use factory classes to create objects in client code. This design pattern increases the flexibility of the code without directly coupling to concrete types.
