10 quirks and secrets about JavaScript_javascript tips
Original author: Andy Croxall
Original link: Ten Oddities And Secrets About JavaScript
Translation editor: Zhang Xinxu
Data types and definitions
1. Null is an object
Among the many types of JavaScript, there is the Null type, which has a unique value of null, that is, its literal, defined as A value that makes no sense at all. It behaves like an object, as shown in the following detection code:
alert (typeof null); //Pop up 'object'
as shown below:
Although the typeof value shows "object", null is not considered an object instance. You know, the values in JavaScript are object instances, each value is a Number object, and each object is an Object object. Because null has no value, it is obvious that null is not an instance of anything. Therefore, the value below equals false.
alert(null instanceof Object); // is false
Translator’s Note: null can also be understood as an object placeholder
2. NaN is a numerical value
The original meaning of NaN is to represent a certain The value is not a numerical value, but it is a numerical value and is not equal to itself. It is strange. Look at the following code:
alert(typeof NaN); //pop up 'Number'
alert(NaN === NaN); //is false
The results are as follows:
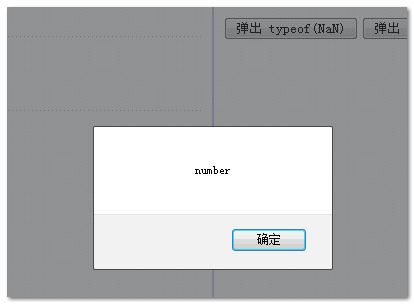
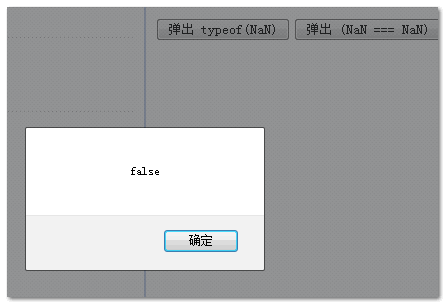
Actually NaN is not equal to anything. To confirm whether something is NaN, you can only use isNaN.
3. Arrays without keywords are equivalent to false (about Truthy and Falsy)
Here is another great quirk of JavaScript:
alert(new Array() == false); // is true
The result is as follows:
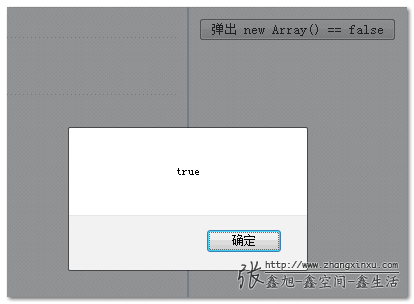
To understand what is going on here, you need to understand the concepts of truthy and falsy. They are a true/flase literal. In JavaScript, all non-Boolean values have a built-in boolean flag. When the value is required to have boolean behavior, this built-in Boolean value will appear, such as when you want to compare with a Boolean value.
Since apples cannot be compared with pears, when JavaScript requires a comparison between two different types of values, it will first weaken them into the same type. false, undefined, null, 0, "", NaN are all weakened to false. This coercion does not always exist, only when used as an expression. Look at the following simple example:
var someVar =0;
alert(someVar == false); //display true
The results are as follows:
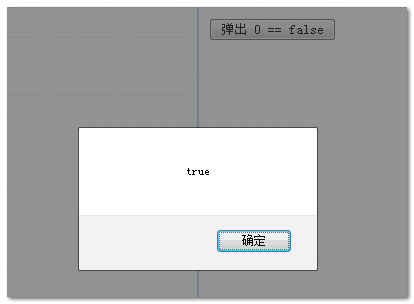
In the above test, we tried to compare the value 0 with the boolean value false. Since the two data types are incompatible, JavaScript automatically forced conversion into unified and equivalent truthy and falsy, where 0 is equivalent to false (as mentioned above) mentioned).
You may have noticed that there are no empty arrays in some of the above values that are equivalent to false. Just because the empty array is a strange thing: it itself is actually truthy, but when the empty array is compared with the Boolean type, its behavior is falsy. Not sure? There are reasons for this. Let’s take an example to verify the strange behavior of empty arrays:
var someVar = []; //Empty array
alert(someVar == false); //The result is true
if (someVar) alert('hello'); //The alert statement is executed, so someVar is treated as true
The result is as shown in the screenshot below, with two boxes popping up in succession:
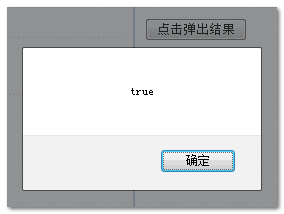
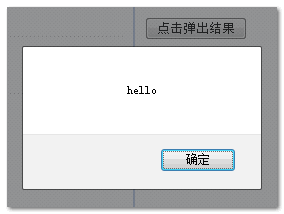
Translator’s Note: The reason for this difference is that, according to the author, the array has a built-in toString() method. For example, when alerting directly, a string will pop up in the form of join(“,”), and an empty array will naturally It is an empty string, so it is equivalent to false. For details, please refer to the author's other article, "Twisted logic: understanding truthy & falsy". But what I personally find strange is that when empty objects, empty functions, weak equals true or false, false is displayed. Why? Is it really because arrays are a weirdo and need special considerations?
To avoid comparison problems with casts, you can use strong equals (===) instead of weak equals (==).
var someVar = 0;
alert(someVar = = false); //The result true – 0 belongs to falsy
alert(someVar === false); //The result false – zero is a numerical value, not a Boolean value
The result is as screenshot below (win7 FF4):
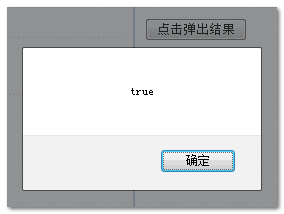
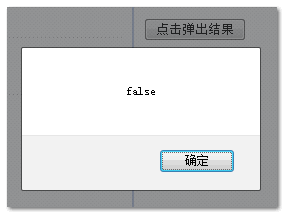
If you want to delve deeper into some unique quirks such as type coercion in JavaScript, you can refer to the official relevant document specifications:
section 11.9.3 of the ECMA-262
Regular Expression Formula
4. replace() can accept callback function
This is one of the least known secrets of JavaScript, first introduced in v1.3. In most cases, the use of replace() is similar to the following:
alert('10 13 21 48 52'.replace(/d /g, '*')); //Replace all numbers with *
This is a simple replacement, one character String, an asterisk. But what if we want to have more control over when replacement occurs? We only want to replace values below 30, what should we do? At this point, it is beyond your reach to rely solely on regular expressions. We need to use the callback function to process each match.
alert('10 13 21 48 52'.replace( /d /g, function(match) {
return parseInt(match) <30?'*' : match;
}));
When each match is completed, JavaScript applies a callback function and passes the matching content to the match parameter. Then, depending on the filtering rules in the callback function, either an asterisk is returned, or the match itself is returned (no replacement occurs).
The screenshot below:
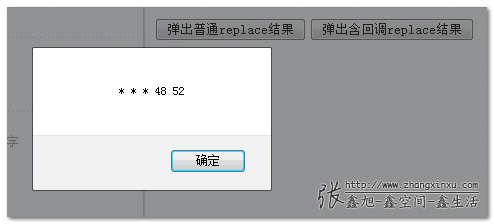
alert(/w{3,}/.test( 'Hello')); //Pop up 'true'
The above line of code is used to verify whether the string has more than three ordinary characters. Obviously "hello" meets the requirements, so true pops up.
The results are as follows:
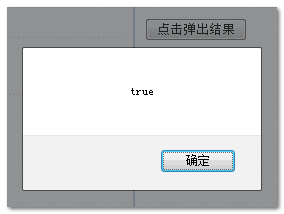
We should also pay attention to the RegExp object, which you can use to create dynamic regular expression objects, for example:
function findWord(word, string) {
var instancesOfWord = string.match(new RegExp('\b' word '\b', 'ig'));
alert(instancesOfWord);
}
findWord('car', 'Carl went to buy a car but had forgotten his credit card.');
Here, we dynamically create a matching verification based on the parameter word. The function of this test code is to select the word car without distinguishing between large and small selections. At a quick glance, the only word in the test English sentence is car, so the performance here is only one word. is used to represent word boundaries.
The results are as follows:
Function and Scope
6. You can pretend to be a scope
Scope is used to determine what variables are available, independent JavaScript (such as JavaScript is not in a running function) in the window object Operating in the global scope, the window object can be accessed under any circumstances. However, local variables declared in a function can only be used within that function.
var animal ='dog';
function getAnimal (adjective) { alert(adjective '' this.animal); }
getAnimal('lovely'); //pop up 'lovely dog'
Here our variables and functions are declared in in global scope. Because this points to the current scope, which is window in this example. Therefore, this function looks for window.animal, which is 'dog'. So far, so good. However, in practice, we can make a function run in a different scope and ignore its own scope. We can use a built-in method called call() to achieve scope impersonation.
var animal ='dog';
function getAnimal (adjective) { alert(adjective '' this.animal); };
var myObj = {animal: 'camel'};
getAnimal.call(myObj, 'lovely'); //pop up 'lovely camel' '
The first parameter in the call() method can pretend to be this in the function. Therefore, this.animal here is actually myObj.animal, which is 'camel'. The subsequent parameters are passed to the function body as ordinary parameters.
Another related one is the apply() method, which works the same as call(). The difference is that the parameters passed to the function are represented in the form of an array instead of independent variables. Therefore, if the above test code is represented by apply(), it is:
getAnimal.apply(myObj, ['lovely']); //Function parameters are sent in the form of array
In the demo page, the result of clicking the first button is as follows:
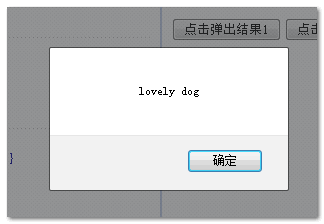
The following is very OK:
(function() { alert('hello'); })(); // Pop up 'hello'
The parsing here is simple enough: declare a function, and then execute it immediately because of () parsing . You may wonder why we do this (referring to the direct () call), which seems a bit contradictory: functions usually contain code that we want to execute later, rather than parsing and executing it now, otherwise , we don’t need to put the code in the function.
Another good use of self-executing functions (SEFs) is to use bound variable values in deferred code, such as event callbacks, timeouts and intervals. Execution(intervals). The following example:
var someVar ='hello';
setTimeout(function() { alert(someVar); }, 1000);
var someVar ='goodbye';
Newbies always ask in the forum why the timeout popup here is goodbye instead Not hello? The answer is that the callback function in timeout does not assign the value of the someVar variable until it is run. At that time, someVar had been rewritten by goodbye for a long time.
SEFs provide a solution to this problem. Instead of implicitly specifying the timeout callback as above, the someVar value is directly passed in as a parameter. The effect is significant, which means that we pass in and isolate the someVar value, protecting it from changing whether there is an earthquake, tsunami, or the girlfriend is roaring.
var someVar = 'hello';
setTimeout( (function(someVar) {
returnfunction() { alert(someVar); }
})(someVar), 1000);
var someVar ='goodbye';
Things have changed, and this time, the pop-up here is hello. This is the difference between function parameters and external variables.
For example, the popup after clicking the last button is as follows:
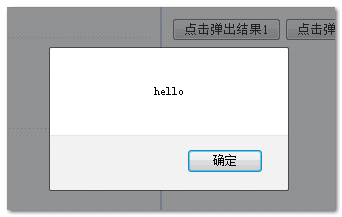
Hello, world!
< ;script>
var ie = navigator.appVersion.indexOf('MSIE') !=-1;
var p = document.getElementById('somePara');
alert(ie ? p.currentStyle. color : getComputedStyle(p, null).color);
The pop-up result of most browsers is ff9900, but the result of FireFox is rgb(255, 153, 0), in the form of RGB. Often, when dealing with colors, we need to spend a lot of code to convert RGB colors to Hex.
The following are the results of the above code in different browsers:
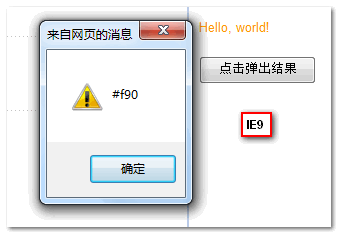
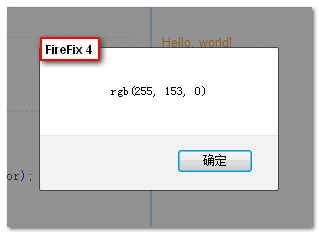
9. 0.1 0.2 !== 0.3
This weird problem does not only appear in JavaScript , which is a common problem in computer science and affects many languages. The title equation outputs 0.30000000000000004.
This is a problem called machine precision. When JavaScript tries to execute the (0.1 0.2) line of code, it converts the value into its preferred binary flavor. This is where the problem starts, 0.1 is not actually 0.1, but its binary form. Essentially, when you write these values down, they are bound to lose precision. You might just want a simple two decimal places, but what you get (according to Chris Pine's comment) is a binary floating point calculation. For example, if you want to translate a paragraph into simplified Chinese, but the result is traditional, there are still differences.
There are generally two ways to deal with problems related to this:
Convert it to an integer and then calculate it, and then convert it to the desired decimal content after the calculation is completed
Adjust your logic and set the permission The range is not the specified result.
For example, we should not look like this:
var num1=0.1, num2=0.2, shouldEqual=0.3;
alert(num1 num2 == shouldEqual); //false
And you can try this:
alert(num1 num2 > shouldEqual - 0.001&& num1 num2 < shouldEqual 0.001); // true
10. Undefined can be defined
We end with a gentle and drizzly little quirk. As strange as it may sound, undefined is not a reserved word in JavaScript, although it has a special meaning and is the only way to determine whether a variable is undefined. Therefore:
var someVar;
alert(someVar = = undefined); //Show true
So far, everything seems calm and normal, but the plot is always bloody:
undefined ="I'm not undefined!";
var someVar;
alert(someVar == undefined ); // Display false!
This is why the outermost closure function in the jQuery source code has an undefined parameter that is not passed in. The purpose is to protect undefined from being taken advantage of by external bad actors. And enter.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
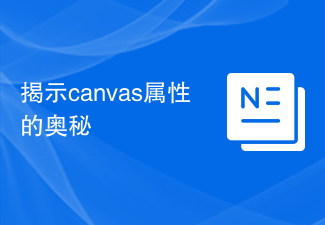
To explore the secrets of the canvas attribute, you need specific code examples. Canvas is a very powerful graphics drawing tool in HTML5. Through it, we can easily draw complex graphics, dynamic effects, games, etc. in web pages. However, in order to use it, we must be familiar with the related properties and methods of Canvas and master how to use them. In this article, we will explore some of the core properties of Canvas and provide specific code examples to help readers better understand how these properties should be used.
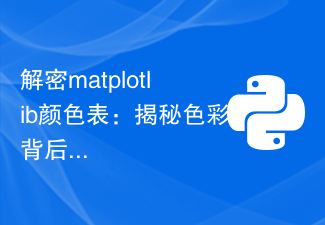
Detailed explanation of matplotlib color table: Revealing the secrets behind colors Introduction: As one of the most commonly used data visualization tools in Python, matplotlib has powerful drawing functions and rich color tables. This article will introduce the color table in matplotlib and explore the secrets behind colors. We will delve into the color tables commonly used in matplotlib and give specific code examples. 1. The color table in Matplotlib represents the color in matplotlib.
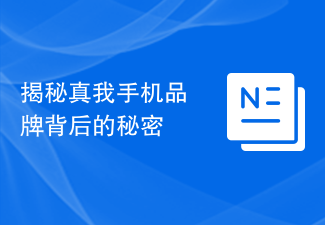
As a first-line mobile phone brand, Realme mobile phone brand has always attracted the attention and attention of consumers. However, as we all know, behind every successful brand there is an unknown journey and story. This article will reveal the secrets behind the Realme mobile phone brand and conduct an in-depth discussion from the origin, development process and market strategy of the brand. The real mobile phone brand originates from OPPO Electronics Corp., a Chinese mobile communications equipment company, and was officially launched in 2018, adhering to the brand concept of "real mobile phone". True self brand is committed to
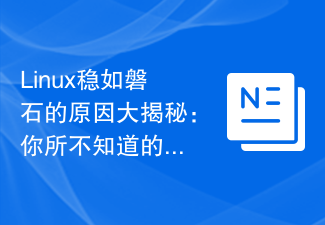
As an open source operating system, Linux system has always been known for its stability and reliability, and is widely used in servers, embedded devices and other fields. So, how exactly does a Linux system remain rock solid? What secrets are hidden in this? This article will reveal the reasons for Linux system stability and reveal these secrets through specific code examples. 1. Open source code Linux system is an open source project, and its source code is open to the public and anyone can view and modify it.
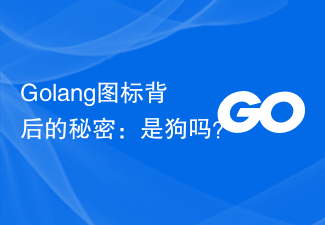
The secret behind the Golang icon: Is it a dog? Golang, the Go language, is an open source programming language developed by Google. It has efficient concurrent processing capabilities, concise syntax, and fast compilation speed, so it has received widespread attention and use. The official logo of Golang has also attracted much attention. Its design is simple and layered, which makes people think of a mysterious animal. What kind of secret is hidden behind this logo? Some people guess that this logo is actually a picture of a dog, so this guess

PHP is a very popular programming language that is easy to learn, powerful and highly flexible. However, when processing large amounts of data and high concurrent requests, PHP's performance issues often become bottlenecks that limit application performance. To solve this problem, developers often use caching techniques to improve the performance and scalability of PHP applications. Caching is a technique for saving data in memory so that applications can quickly obtain already calculated results without having to calculate them again. In PHP, caching technology
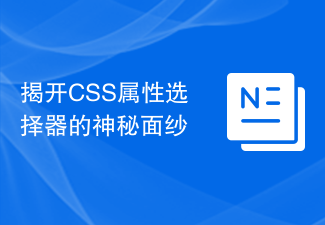
Secrets of CSS Attribute Selectors Revealed CSS attribute selectors are a very useful and powerful tool that allow us to select and style specific elements by their attribute values. These attribute selectors can match and select based on the element's attribute value, where the attribute value appears, and specific characters in the attribute value. This article will reveal the secrets of CSS attribute selectors through specific code examples. First, let's learn about some basic CSS attribute selectors. The most common attribute selector is "[attribute]
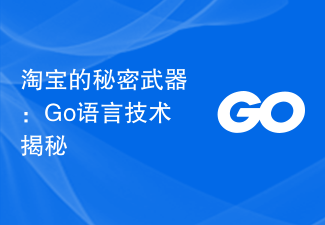
Taobao is China's largest online shopping platform, with hundreds of millions of users purchasing goods every day. In supporting the operation of such a huge platform, technology plays a vital role. In recent years, with the popularity of Go language in the Internet field, some people have begun to speculate whether Taobao's success is related to Go language. This article will start from a technical perspective and specifically explore the application of Go language in Taobao and the reasons why it may become Taobao's secret weapon. First, let’s analyze the technical challenges Taobao faces. as a huge
