In The Evolution of the Event Module I used dispatchEvent (standard) and fireEvent (IE) to actively trigger events. As follows
...
dispatch = w3c ?
function(el, type){
try{
var evt = document.createEvent('Event');
evt.initEvent(type,true,true);
el.dispatchEvent( evt);
}catch(e){alert(e)};
} :
function(el, type){
try{
el.fireEvent('on' type) ;
}catch(e){alert(e)}
};
...
jQuery does not use the dispatchEvent/fireEvent method at all. It uses another mechanism.
The core method of jQuery triggering events is jQuery.event.trigger. It provides two trigger event methods for client programmers: .trigger/.triggerHandler
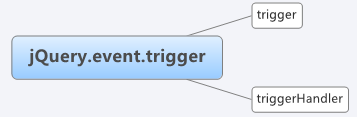
The occurrence of an event in some elements may cause two actions, one is the default behavior , one is event handler. For example, link A
Sina Mail After clicking, 1 pops up (event handler), click OK to jump (default behavior) to mail.sina.com.cn. Therefore, the function that is designed to trigger the event must take these two situations into consideration.
jQuery uses .trigger and .triggerHandler to distinguish between these two situations:
.trigger executes the event handler/executes bubbling/executes the default behavior
.triggerHandler executes the event handler/does not bubble/does not execute the default behavior
The source code of
.trigger/.triggerHandler is as follows
trigger : function( type, data ) {
return this.each(function() {
jQuery.event.trigger( type, data, this );
});
},
triggerHandler: function( type, data ) {
if ( this[0] ) {
return jQuery.event.trigger( type, data, this[0], true );
}
} ,
It can be seen that both call jQuery.event.trigger. When calling, one did not pass true and the other did. Passing true triggerHander means that only the event handler will be executed.
In addition, there is one difference to note: .trigger operates on a collection of jQuery objects, while .triggerHandler only operates on the first element of the jQuery object. As follows
p1
p1
p1
<script> <br>$('p').click(function(){alert(1)} ); <br>$('p').trigger('click'); // Play 3 times, that is, the clicks of three p's are triggered. ; // Only play once, that is, only trigger the click of the first p <br></script>
Okay, it’s time to post the code of jQuery.event.trigger
trigger: function( event, data, elem, onlyHandlers ) {
// Event object or event type
var type = event.type || event,
namespaces = [],
exclusive;
......
}
This is the definition of jQuery.event.trigger, with most of it omitted. Listed below are
if ( type.indexOf("! ") >= 0 ) {
// Exclusive events trigger only for the exact event (no namespaces)
type = type.slice(0, -1);
exclusive = true;
}
This paragraph is to handle the situation of .trigger('click!'), that is, triggering non-namespace events. The variable exclusive is used within jQuery.event.handle after being hung on the event object. For example
function fn1() {
console .log(1)
}
function fn2() {
console.log(2)
}
$(document).bind('click.a', fn1);
$(document).bind('click', fn2);
$(document).trigger('click!'); // 2
Added two click events to the document, one with namespace "click.a" and one without "click". When using trigger, add an exclamation mark "!" after the click parameter. It can be seen from the output result of 2 that the event of the namespace is not triggered. To summarize:
.trigger('click') triggers all click events
.trigger('click.a') only triggers the click event of "click.a"
.trigger('click!' ) Trigger non-namespace click events
Then look at
if ( type.indexOf(".") >= 0 ) {
// Namespaced trigger; create a regexp to match event type in handle()
namespaces = type.split(".");
type = namespaces.shift();
namespaces.sort();
}
This paragraph is easy to understand, that is, .trigger('click.a ') processing, that is, processing of events with namespaces.
Then look at
if ( (!elem || jQuery.event.customEvent[ type ]) && !jQuery.event.global[ type ] ) {
// No jQuery handlers for this event type, and it can't have inline handlers
return;
}
Return directly for some special events such as "getData" or for events that have been triggered.
Go down
event = typeof event === "object" ?
// jQuery.Event object
event[ jQuery.expando ] ? event :
// Object literal
new jQuery.Event( type, event ) :
// Just the event type (string)
new jQuery.Event( type );
There are three situations
, event itself is an instance of jQuery.Event class
, event is an ordinary js object (not an instance of the jQuery.Event class)
, event is a string, such as "click"
continued
event.type = type;
event.exclusive = exclusive;
event .namespace = namespaces.join(".");
event.namespace_re = new RegExp("(^|\.)" namespaces.join("\.(?:.*\.)?") "( \.|$)");
It should be noted that exclusive/namespace/namespace_re is linked to event and can be used in jQuery.event.handle (event namespace).
The following is
// triggerHandler() and global events don't bubble or run the default action
if ( onlyHandlers || !elem ) {
event.preventDefault();
event.stopPropagation();
}
onlyHandlers is only used in .triggerHandler, that is, it does not trigger the default behavior of the element and stops bubbling.
The following is
// Handle a global trigger
if ( !elem ) {
// TODO: Stop taunting the data cache; remove global events and always attach to document
jQuery.each( jQuery.cache, function() {
// internalKey variable is just used to make it easier to find
// and potentially change this stuff later; currently it just
// points to jQuery.expando
var internalKey = jQuery.expando,
internalCache = this[ internalKey ];
if ( internalCache && internalCache.events && internalCache.events[ type ] ) {
jQuery.event.trigger( event, data, internalCache.handle.elem );
}
});
return;
}
This is a recursive call. If the elem element is not passed, it will be taken from jQuery.cache.
Followed by
// Don't do events on text and comment nodes
if ( elem.nodeType === 3 || elem.nodeType === 8 ) {
return;
}
Attribute, text node Return directly.
The following is the copy code
// Clone any incoming data and prepend the event, creating the handler arg list
data = data != null ? jQuery.makeArray( data ) : [];
data.unshift( event );
First put the parameter data into the array, and put the event object at the first position of the array.
Followed by
// Fire event on the current element, then bubble up the DOM tree
do {
var handle = jQuery._data( cur, "handle" );
event.currentTarget = cur;
if ( handle ) {
handle.apply( cur, data );
}
// Trigger an inline bound script
if ( ontype && jQuery.acceptData( cur ) && cur[ ontype ] && cur[ ontype ].apply( cur , data ) === false ) {
event.result = false;
event.preventDefault();
}
// Bubble up to document, then to window
cur = cur .parentNode || cur.ownerDocument || cur === event.target.ownerDocument && window;
} while ( cur && !event.isPropagationStopped() );
This code is very Important, do the following things
, get handle
, execute
, execute events added through onXXX (such as onclick="fun()")
, get the parent element
while loop Repeat these four steps to simulate event bubbling. Until the window object.
The following is
// If nobody prevented the default action, do it now
if ( !event.isDefaultPrevented() ) {
var old,
special = jQuery.event.special[ type ] || {};
if ( (! special._default || special._default.call( elem.ownerDocument, event ) === false) &&
!(type === "click" && jQuery.nodeName( elem, "a" )) && jQuery. acceptData( elem ) ) {
// Call a native DOM method on the target with the same name name as the event.
// Can't use an .isFunction)() check here because IE6/7 fails that test.
// IE<9 dies on focus to hidden element (#1486), may want to revisit a try/catch.
try {
if ( ontype && elem[ type ] ) {
// Don't re-trigger an onFOO event when we call its FOO() method
old = elem[ ontype ];
if ( old ) {
elem[ ontype ] = null;
}
jQuery.event.triggered = type;
elem[ type ]();
}
} catch ( ieError ) {}
if ( old ) {
elem [ ontype ] = old;
}
jQuery.event.triggered = undefined;
}
}
This section is the trigger for the browser's default behavior. Such as form.submit(), button.click(), etc.
Note that due to the security restrictions of links in Firefox, jQuery’s default behavior for links is unified to not trigger. That is, the link cannot be jumped through .trigger().