This is an original translated article. Original address.
We often use iframes to load third-party content, ads or plug-ins. Iframe is used because it can be loaded in parallel with the main page and will not block the main page. Of course, there are pros and cons to using iframes: Steve Souders explains it in his blog: Using Iframes Sparingly:
- iframe will block the onload event of the main page
- The main page and iframe share the same connection pool
Blocking the onload of the main page is the most performance-impacting aspect of these two problems. Generally speaking, we want the onload time to be triggered as early as possible. On the one hand, users have experienced it, and more importantly, Google scores the loading speed of the website: users can use the Google toolbar in IE and FF to time the time.
So in order to improve page performance, how can we load the iframe without blocking the onload event of the main page?
This article talks about four methods of loading iframe: normal iframe, loading iframe after onload, setTimeout() iframe and asynchronous loading iframe. I use the IE8 timeline to display the loading results of each method. I recommend paying more attention to the dynamic asynchronous loading method, because it has the best performance. In addition, there is also a friendly iframe (friendly iframe) technology. It may not be considered an iframe loading technology, but iframe must be used because it loads without blocking.
Load iframe using the normal method
This is a well-known normal loading method, which has no browser compatibility issues.
Use this loading The method will behave as follows in each browser:
iframe will be loaded before the onload of the main page
iframe will trigger the onload of the iframe after all iframe contents have been loaded
of the main page onload will be triggered after the onload of iframes is triggered, so the iframe will block the loading of the main page
When the iframe is loading, the browser will indicate that it is loading something and is in a busy state.
Here is a demo page. The timeline shows that iframe blocks the loading of the main page.

My suggestion: pay attention to onload blocking. If the content of the iframe only takes a short time to load and execute, then it is not a big problem, and using this method has the advantage that it can be loaded in parallel with the main page. But if it takes a long time to load this iframe, the user experience will be very poor. You have to test it yourself and then do some testing at http://www.webpagetest.org/ to see if other loading methods are needed based on the onload time.
Load the iframe after onload
If you want to load some content in the iframe, but the content is not that important to the page. Or the content does not need to be displayed to the user immediately, but needs to be clicked to trigger. Then you can consider loading the iframe after the main page loads.
<script> <br>//doesn't block the load event <br>function createIframe() { <br>var i = document.createElement("iframe"); <br>i.src = "path/to/file"; <br>i.scrolling = " auto"; <br>i.frameborder = "0"; <br>i.width = "200px"; <br>i.height = "100px"; <br>document.getElementById("div-that-holds- the-iframe").appendChild(i); <br>}; <br>// Check for browser support of event handling capability <br>if (window.addEventListener) window.addEventListener("load", createIframe, false) ; <br>else if (window.attachEvent) window.attachEvent("onload", createIframe); <br>else window.onload = createIframe; <br></script>
这种加载方法也是没有浏览器的兼容性问题的:
iframe会在主页面onload之后开始加载
主页面的onload事件触发与iframe无关,所以iframe不会阻塞加载
当iframe加载的时候,浏览器会标识正在加载
这是是一个测试页面,时间线图如下

这种方法比普通方法有什么好处呢?load事件会马上触发,有两个好处:
其他等待主页面onload事件的代码可以尽早执行
Google Toolbar计算你页面加载的时间会大大减少
但是,当iframe加载的时候,还是会看到浏览器的忙碌状态,相对于普通加载方法,用户看到忙碌状态的时间更长。还有就是用户还没等到页面完全加载完的时候就已经离开了。有些情况下这是个问题,比如广告。
setTimeout()来加载iframe
这种方法的目的是不阻塞onload事件。
Steve Souders(又是他?)有一个这种方法的测试页面(http://stevesouders.com/efws/iframe-onload-nonblocking.php)。他写道:“src通过setTimeout动态的设置,这种方法可以再所有的浏览器中避免阻塞”。
<script> <br>function setIframeSrc() { <br>var s = "path/to/file"; <br>var iframe1 = document.getElementById('iframe1'); <br>if ( - 1 == navigator.userAgent.indexOf("MSIE")) { <br>iframe1.src = s; <br>} else { <br>iframe1.location = s; <br>} <br>} <br>setTimeout(setIframeSrc, 5); <br></script>
在除了IE8以外的所有浏览器中会有如下表现:
iframe会在主页面onload之前开始加载
iframe的onload事件会在iframe的内容都加载完毕之后触发
iframe不会阻塞主页面的onload事件(IE8除外)
为什么不会阻塞主页面的onload呢(IE8除外)?因为setTimeout()
当iframe加载的时候,浏览器会显示忙碌状态
下面是时间线图
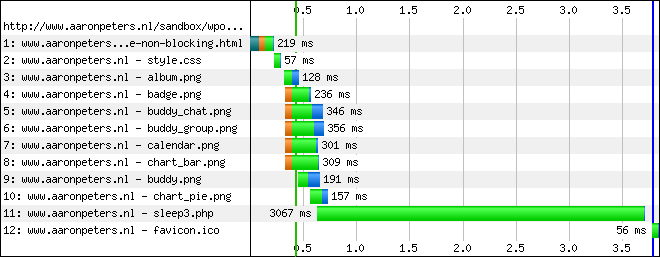
因为IE8的问题,这种技术就不适合很多网站了。如果有超过10%的用户使用IE8,十分之一的用户体验就会差。你会说那也只是比普通加载差一点点,其实普通加载性能上也不差。onload事件对于10%的用户来说都更长。。。。额,你自己考虑吧。但是最好在看了下面这个很赞的异步加载方法之后再决定吧。
我在参加Velocity 2010的时候,Meebo的两个工程师(@marcuswestin and Martin Hunt)做了一个关于他们的Meebo Bar的演讲。他们使用iframe来加载一些插件,并且真正做到了无阻塞加载。对于有的开发者来说,他们的做法还比较新鲜。很赞,超级赞。但是一些原因导致这种技术没有得到相应的关注,我希望这篇blog能把它发扬光大。
<script> <br>(function(d) { <br>var iframe = d.body.appendChild(d.createElement('iframe')), <br>doc = iframe.contentWindow.document; <br>// style the iframe with some CSS <br>iframe.style.cssText = "position:absolute;width:200px;height:100px;left:0px;"; <br>doc.open().write('<body onload="' + 'var d = document;d.getElementsByTagName(\'head\')[0].' + 'appendChild(d.createElement(\'script\')).src' + '=\'\/path\/to\/file\'">'); <br>doc.close(); //iframe onload event happens <br>})(document); <br></script>
神奇的地方就在:这个iframe一开始没有内容,所以onload会立即触发。然后你创建一个script元素,用他来加载内容、广告、插件什么的,然后再把这个script添加到HEAD中去,这样iframe内容的加载就不会阻塞主页面的onload!你应该看看他在个浏览器中的表现:
iframe会在主页面onload之前开始加载
iframe的onload会立即触发,因为iframe的内容一开始为空
主页面的onload不会被阻塞
为什么这个iframe不会阻塞主页面的onload?因为
如果你不在iframe使用onload监听,那么iframe的加载就会阻塞主页面的onload
当iframe加载的时候,浏览器终于不显示忙碌状态了(非常好)
我的测试页给出下面的时间线:

Escape characters make the code look a little uncomfortable, but that’s not a problem. Give it a try.
Friendly iframe loading
This is used to load ads. Although this is not an iframe loading technology, it uses iframes to hold advertisements. The highlight is not how the iframe loads, but how the main page, iframe, and ads work together. As follows:
- Create an iframe first. Set his src to a static html file under the same domain name
- In this iframe, set the js variable inDapIF=true to tell the ad that it has been loaded in this iframe.
- In this iframe, create a script element and add the URL of the ad as src, and then load it like a normal ad code
- When the ad is loaded, reset the iframe size to fit the ad
- This method also has no browser compatibility issues.
I didn’t create the relevant test page, so I don’t have the information for the first song. From the results of my research:
This method is not very useful if you only want to call an iframe with a certain src address on your web page.
If you want to display multiple ads on a web page, a more flexible method is: load one ad, and then update the iframe to load another main page. The DOMContentLoaded time will not be blocked, and page rendering will not be blocked. Of course, , the onload event of the main page will still be blocked.
Please indicate when reprinting: Author:
BeiYuu