Collection of some practical jQuery code snippets_jquery
The jQuery snippets below are only a small part. If you have encountered some commonly used jQuery codes during the learning process, please share them. Let's take a look at these code snippets.
1.jQuery gets the user IP:
$.getJSON("http://jsonip.appspot.com?callback=?", function (data) {
alert("Your ip: " data.ip);
});
2.jQuery to view the width and height of the image:
var theImage = new Image();
theImage.src = $('#imageid').attr("src");
alert("Width: " theImage.width);
alert("Height: " theImage.height);
3.jQuery finds the specified string:
var str = $('*:contains("the string")');
4.js Determine whether the browser enables cookies:
$(document).ready(function () {
document.cookie = "cookieid=1; expires=60";
var result = document.cookie.indexOf("cookieid=") != -1 ;
if (!result) {
alert("Browser does not enable cookies");
}
});
5.jQuery detects keyboard keys:
$(document).ready(function () {
$(this).keypress(function (e) {
switch (e.which) {
case 13:
alert("You pressed the Enter key");
break ;
//more detect
}
});
});
Okay, this article is summarized here, I hope this jQuery code snippet article It can be helpful to everyone
1. jQuery scrolling to the top/bottom
Regarding jQuery scrolling, this site has published similar articles before, such as: jQuery back to the top, and they are organized again below Here it is:
//Scroll to the top
$( "html, body").animate({ scrollTop: "0px" }, 1000);
//Scroll to the bottom
//$("#container"): The element to be scrolled
$( "html, body").animate({
scrollTop: $("#container").height()
}, 1000);
2.jQuery determines whether the element exists
How to use jQuery to determine whether an element exists? I believe many jQuery learners will ask this question. The method is very simple, as follows:
if ($(" #elementid").length) {
//Element exists
}
3 .Use the abort() method to cancel the Ajax request
Use the abort() method to cancel the last request when executing a js asynchronous request. The method is as follows:
var req = $.ajax({
type: "post",
url: "/article/form/ comment.aspx",
data: { "id": 1 },
success: function() {
//handle
}
});
//Cancel Ajax Request
if (req) {
req.abort()
}
jQuery Ajax is an important part of using jQuery. If you are a beginner of jQuery, you may be interested in it. The above code feels unfamiliar, maybe you can take a look at jQuery Learning Summary (5) jQuery Ajax.
4.jQuery disables the right mouse button
$(document).ready(function() {
$(document).bind("contextmenu", function() {
return false;
});
});
5. Pass parameters to the method called by setTimeout()
$(document).ready(function() {
timeout = setTimeout(function() {
showMess("succeed")
}, 2000);
} );
function showMess(m) {
alert(m);
}
1.jQuery Countdown
$(document). ready(function () {
var count = 10;
countdown = setInterval(function () {
$("p.countdown").html(count "Will jump in seconds!");
if (count == 0) {
clearInterval(countdown)
window.location = 'http://google.com';
}
count--;
} , 1000);
});
2.jQuery determines the browser type and version number
jQuery determines the browser type and version number is very simple, you can directly use the $.browser method Make judgments. But when I tested it myself, I found that when I judged the Chrome browser, Safari was returned. After searching on the Internet, I got the following code:
var browserName = navigator.userAgent.toLowerCase();
mybrowser = {
version: (browserName.match(/. (? :rv|it|ra|ie)[/: ]([d.] )/) || [0, '0'])[1],
safari: /webkit/i.test(browserName) && !this.chrome,
opera: /opera/i.test(browserName),
firefox: /firefox/i.test(browserName),
msie: /msie/i.test(browserName) && !/opera/.test(browserName),
mozilla: /mozilla/i.test(browserName) && !/(compatible|webkit)/.test(browserName) && !this.chrome,
chrome: / chrome/i.test(browserName) && /webkit/i.test(browserName) && /mozilla/i.test(browserName)
}
$(document).ready(function () {
if (mybrowser.msie) {
alert("The browser is: Internet Explorer and the version number is: " $.browser.version);
}
else if (mybrowser.mozilla) {
alert( "The browser is: Firefox and the version number is:" $.browser.version);
}
else if (mybrowser.opera) {
alert("The browser is: Opera and the version number is:" $ .browser.version);
}
else if (mybrowser.safari) {
alert("The browser is: Safari and the version number is: " $.browser.version);
}
else if (mybrowser.chrome) {
alert("The browser is: Chrome and the version number is: " mybrowser.version);
}
else {
alert("神马");
} ( See below how it is relatively simple to use jQuery to center elements.
Copy code
this.css('left', ($(window).width() - this.width() ) / 2 $(window).scrollLeft() 'px');
return this;
}
})(jQuery);
$(document).ready(function () {
//Call
$("#somediv").center();
});
4.jQuery determines whether the image is fully loaded
Copy code
If you have any useful jQuery code snippets, please post them in jQuery Share your learning with everyone!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
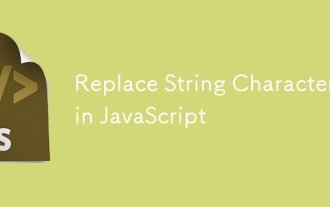
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
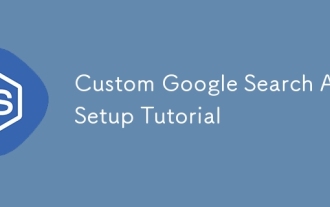
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
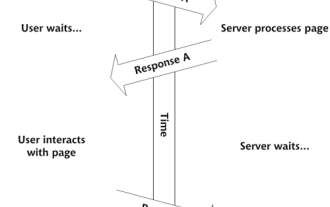
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
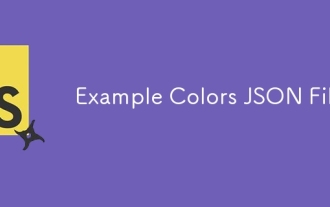
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
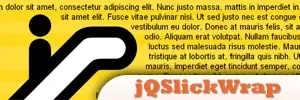
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
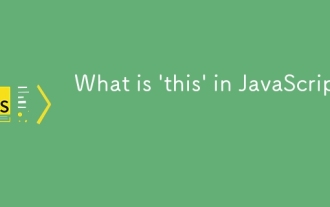
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was
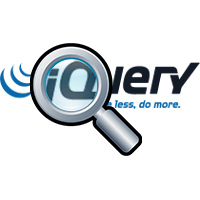
jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
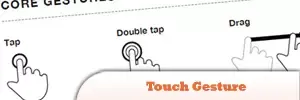
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig
