jQuery source code analysis notes (7) Queue_jquery
Each Element can have multiple queues, but basically only one is used, which is the default fn queue. Queues allow a series of functions to be called asynchronously without blocking the program. For example: $("#foo").slideUp().fadeIn(); In fact, this is a chain call commonly used by all of us. In fact, this is a Queue. Therefore, queues are similar to Deferreds and are an internal infrastructure. When slideUp is running, fadeIn is placed in the fx queue. When slideUp is completed, it is taken out of the queue and run. The queue function allows direct manipulation of the behavior of this chain of calls. At the same time, queue can specify the queue name to obtain other capabilities, not limited to the fx queue.
// General usage:
$("# foo").slideUp(function() {
alert("Animation complete.");
});
// Equivalent to:
$("#foo").slideUp() ; // Does not provide a callback, just triggers the event
$("#foo").queue(function() { // Add the callback function
alert("Animation complete.");
$( this).dequeue(); // Must be taken out of the queue, then the next function in the queue has a chance to be called
});
queue internally uses data or JavaScript array API to save data. The push and shift operations on arrays are inherently a set of queue APIs. Data can be used to save any data.
queue: function(elem, type, data) {
if(elem) {
// The default is fn
type = (type || "fx") "queue";
// data internal API: data(element, key, value, pvt );
// Data is not passed in here for the sake of efficiency. If data is not passed in, it is just a get queue, so the following judgment is not needed
var q = jQuery.data(elem, type, undefined, true);
if(data) {
if (!q || jQuery.isArray(data)) {
q = jQuery.data(elem, type, jQuery.makeArray(data), true);
} else {
q.push(data ); // Push
}
}
return q || [];
}
}
dequeue: function(elem, type) { type = type || " fx"; // Get this queue var queue = jQuery.queue(elem, type), // Dequeue an element fn = queue.shift(), defer;
// "inprogress" sentry
if( fn === "inprogress") {
fn = queue.shift();
}
if(fn) {
// Add a sentry to prevent automatic dequeueing
if( type === "fx") {
queue.unshift("inprogress");
}
// Execute
fn.call(elem, function() {
jQuery.dequeue (elem, type);
});
}
if(!queue.length) {
jQuery.removeData(elem, type "queue", true);
handleQueueMarkDefer(elem , type, "queue");
}
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


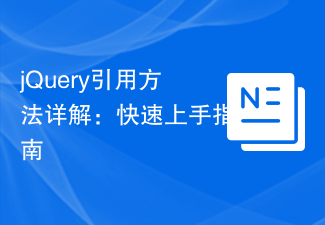
Detailed explanation of jQuery reference method: Quick start guide jQuery is a popular JavaScript library that is widely used in website development. It simplifies JavaScript programming and provides developers with rich functions and features. This article will introduce jQuery's reference method in detail and provide specific code examples to help readers get started quickly. Introducing jQuery First, we need to introduce the jQuery library into the HTML file. It can be introduced through a CDN link or downloaded
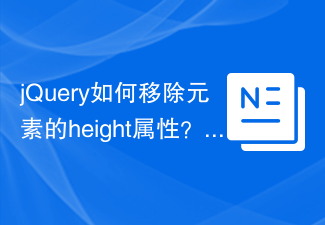
How to remove the height attribute of an element with jQuery? In front-end development, we often encounter the need to manipulate the height attributes of elements. Sometimes, we may need to dynamically change the height of an element, and sometimes we need to remove the height attribute of an element. This article will introduce how to use jQuery to remove the height attribute of an element and provide specific code examples. Before using jQuery to operate the height attribute, we first need to understand the height attribute in CSS. The height attribute is used to set the height of an element
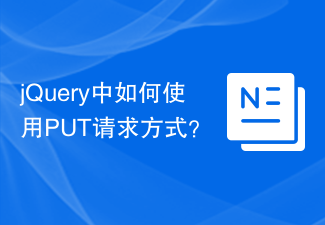
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
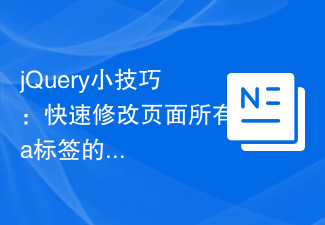
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
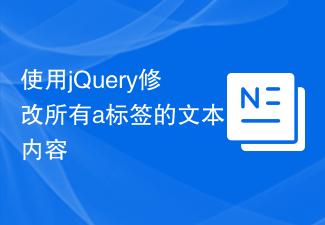
Title: Use jQuery to modify the text content of all a tags. jQuery is a popular JavaScript library that is widely used to handle DOM operations. In web development, we often encounter the need to modify the text content of the link tag (a tag) on the page. This article will explain how to use jQuery to achieve this goal, and provide specific code examples. First, we need to introduce the jQuery library into the page. Add the following code in the HTML file:
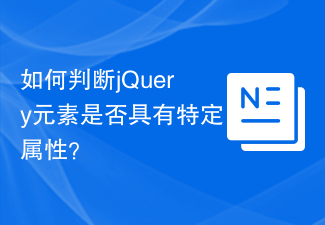
How to tell if a jQuery element has a specific attribute? When using jQuery to operate DOM elements, you often encounter situations where you need to determine whether an element has a specific attribute. In this case, we can easily implement this function with the help of the methods provided by jQuery. The following will introduce two commonly used methods to determine whether a jQuery element has specific attributes, and attach specific code examples. Method 1: Use the attr() method and typeof operator // to determine whether the element has a specific attribute
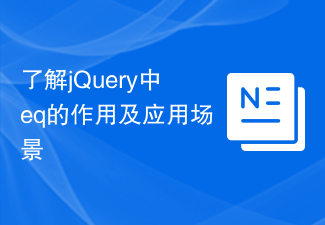
jQuery is a popular JavaScript library that is widely used to handle DOM manipulation and event handling in web pages. In jQuery, the eq() method is used to select elements at a specified index position. The specific usage and application scenarios are as follows. In jQuery, the eq() method selects the element at a specified index position. Index positions start counting from 0, i.e. the index of the first element is 0, the index of the second element is 1, and so on. The syntax of the eq() method is as follows: $("s
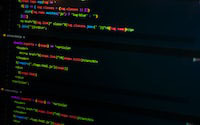
目录1:basename()2:copy()3:dirname()4:disk_free_space()5:disk_total_space()6:file_exists()7:file_get_contents()8:file_put_contents()9:filesize()10:filetype()11:glob()12:is_dir()13:is_writable()14:mkdir()15:move_uploaded_file()16:parse_ini_file()17:
