


Collection of basic knowledge of javascript (1) Recommended collection_Basic knowledge
次へ: js 正規表現、クロージャー、適用、呼び出し先、イベント関連関数、クッキーなど。
1.javascript 配列 API
//配列を定義
var pageIds = new Array();
pageIds.push('A');
Array length
pageIds.length;
//shift: 元の配列の最初の項目を削除し、削除された要素の値を返します。配列が空の場合、未定義を返します
var a = [1,2,3,4,5]
var b = a.shift(); //a: [2,3,4,5]; b: 1
//unshift: 元の配列の先頭にパラメータを追加し、配列の長さを返します
var a = [1,2,3,4,5]; a.unshift(-2,- 1); //a: [-2,-1,1,2,3,4,5] b: 7
//注: IE6.0 でのテスト戻り値FF2.0 では常に未定義です。テストの戻り値は 7 なので、このメソッドの戻り値は信頼できません。戻り値を使用する必要がある場合は、このメソッドの代わりに splice を使用できます。
//pop: 元の配列の最後の項目を削除し、削除された要素の値を返します。配列が空の場合は、未定義の
var a = [1,2,3,4,5] を返します。
var b = a.pop(); //a: [1,2,3,4] b: 5
//push: 元の配列の末尾にパラメータを追加し、配列の長さを返します。配列
var a = [1,2,3,4,5];
var b = a.push(6,7); //a: [1,2,3,4,5,6] ,7] b: 7
//concat: 元の配列にパラメータを追加して形成された新しい配列を返します
var a = [1,2,3,4,5]; b = a.concat(6 ,7); //a: [1,2,3,4,5] b: [1,2,3,4,5,6,7]
//splice( start,deleteCount,val1,val2 ,): deleteCount 項目を開始位置から削除し、この位置から val1、val2 を挿入します。
var a = [1,2,3,4,5]; = a.splice(2 ,2,7,8,9); //a:[1,2,7,8,9,5] b:[3,4]
var b = a.splice( 0,1); / /shift
a.splice(0,0,-2,-1) と同じ; //unshift
var b = a.splice() と同じa.length-1,1) ; //pop
a.splice(a.length,0,6,7) と同じ //push
//リバースと同じ: 配列の順序を逆にします
var a = [1,2,3,4,5]
var b = a.reverse(); //a: [5,4,3,2, 1] b: [5,4,3,2 ,1]
//sort(orderfunction): 指定されたパラメーターに従って配列を並べ替えます
var a = [1,2,3,4,5] ;
var b = a.sort() ; //a: [1,2,3,4,5] b: [1,2,3,4,5]
//スライス(開始, end): 元の配列で指定された開始添字を返します。 終了添字間の項目で構成される新しい配列。
var a = [1,2,3,4,5];
var b = a.slice( 2,5); //a: [ 1,2,3,4,5] b: [3,4,5]
//join(separator): を使用して、配列の要素を文字列に結合します。 separator を区切り文字として使用します。省略した場合はデフォルトが使用されます。区切り文字としてカンマを使用します。
var a = [1,2,3,4,5]; //a: [1,2,3,4 ,5] b: "1|2|3|4|5"
2.dom で最もよく使用される API
ドキュメント メソッド:
getElementById(id) ノードは指定されたノードへの参照を返します
getElementsByTagName(name) NodeList はドキュメント内のすべての一致する要素のコレクションを返します
createElement(name) ノード ノード
createTextNode(text) ノードはプレーンを作成しますtext ノード
ownerDocument Document は、このノードが属するドキュメントを指します
documentElement ノード 戻り値 html ノード
document.body ノード 戻り値 body ノード
要素メソッド:
getAttribute(attributeName) 文字列指定された属性の値
setAttribute(attributeName,value) String 属性に値を割り当てます
removeAttribute(attributeName) String 指定された属性とその値を削除します
getElementsByTagName(name) NodeList 一致するすべてのコレクションを返しますノード内の要素
node メソッド:
appendChild(child) ノードは指定されたノードを与えます クリックして新しい子ノードを追加します
removeChild(child) Node 指定されたノードの子ノードを削除します
replaceChild( newChild,oldChild) ノード 指定したノードの子ノードを置き換えます
insertBefore(newChild, refChild) ノードは同じレベルのノードの前に新しいノードを挿入します
hasChildNodes() ノードに子がある場合、ブール値は true を返しますノード
ノード属性:
nodeName 文字列はノードを文字列形式で格納します。 name
nodeType 文字列はノードのタイプを整数データ形式で格納します。
nodeValue 文字列はノードの値を使用可能な形式で格納します
parentNode ノードはノードの親ノードへの参照を指します
childNodes NodeList 子ノードを指す参照のコレクション
firstChild Node 子ノードの組み合わせの最初の子ノードを指す参照
lastChild ノード 子ノードの組み合わせの最後の子ノードを指す参照
PreviousSibling Node は前の兄弟ノードを指します。このノードが兄弟ノードの場合、値は null になります。
nextSibling Node は、そのノードを指します。次の兄弟ノード; このノードが兄弟ノードの場合、値は null
3. オンライン検索 非表示のマップ オブジェクト:
function HashMap()
{
/**マップサイズ ** /
var サイズ = 0;
/**物体 **/
var エントリ = new Object();
/**ライブ **/
this.put = 関数(キー , 値)
{
if(!this.containsKey(key))
{
サイズ ;
}
エントリ [キー] = 値; 🎜>/**選ぶ **/
this.get = function (key)
{
return this.containsKey(key) ? エントリ[key] :
}
/**消去 **/
this.remove = function ( key )
{
if( this.containsKey(key) && ( delete エントリ[key] ) )
{
サイズ --;
}
}
/**キーを含めるかどうか **/
this.containsKey = function ( key )
{
return (エントリのキー); >}
/**値を含めるかどうか **/
this.containsValue = function ( value )
{
for(エントリの var prop)
{
if(entry[prop ] == 値)
{
true を返す
}
}
/**すべての値 **/
this.values = function ()
{
var 値 = new Array();
for (エントリの var prop)
{
values.push(entry[prop]);
}
戻り値;
}
/**すべてのキー **/
this.keys = function ()
{
var key = new Array();
for(エントリ内の変数 prop)
{
keys.push(prop);
}
キーを返す
}
/**マップサイズ **/
this.size = function ()
{
サイズを返す;
}
/ * Clear*/
this.clear = function ()
{
size = 0;
エントリ = 新しいオブジェクト()
}
var map = new HashMap();
/*
map.put("A","1");
map.put("B","2");
map.put("A","5");
map.put("C","3");
map.put("A","4");
*/
/*
alert(map.containsKey("XX"));
alert(map.size());
alert(map.get("A"));
alert(map.get("XX"));
map.remove("A");
alert(map.size());
alert(map.get("A"));
*/
/**You can also use the object as Key **/
/*
var arrayKey = new Array("1","2","3","4");
var arrayValue = new Array("A","B","C","D");
map.put(arrayKey,arrayValue);
var value = map.get(arrayKey);
for(var i = 0 ; i < value.length ; i )
{
//alert(value[i]);
}
*/
/**When an object is used as a Key, the toString() method of the object is automatically called. In fact, the String object is ultimately used as the Key**/
/**If it is a custom object, you have to override the toString() method. Otherwise, . will be the following result **/
function MyObject(name)
{
this.name = name;
}
/**
function MyObject(name)
{
this.name = name;
this.toString = function ()
{
return this.name;
}
}
**/
var object1 = new MyObject("小张");
var object2 = new MyObject("小名");
map.put(object1,"小张");
map.put(object2,"小名");
alert(map.get(object1));
alert(map.get(object2));
map.remove("xxxxx");
alert(map.size());
/**Running result Nickname Nickname size = 1 **/
/**If you change it to an object that overrides the toString() method, the effect will be completely different **/
4.常用的数字函数:
·数字型(Number)
1.声明
var i = 1;
var i = new Number(1);
2.字符串与数字间的转换
var i = 1;
var str = i.toString(); //结果: "1"
var str = new String(i); //结果: "1"
i = parseInt(str); //结果: 1
i = parseFloat(str); //结果: 1.0
//注意: parseInt,parseFloat会把一个类似于"32G"的字符串,强制转换成32
3.判断是否为有效的数字
var i = 123; var str = "string";
if( typeof i == "number" ){ } //true
//某些方法(如:parseInt,parseFloat)会返回一个特殊的值NaN(Not a Number)
//请注意第2点中的[注意],此方法不完全适合判断一个字符串是否是数字型!!
i = parseInt(str);
if( isNaN(i) ){ }
4.数字型比较
//此知识与[字符串比较]相同
5.小数转整数
var f = 1.5;
var i = Math.round(f); //结果:2 (四舍五入)
var i = Math.ceil(f); //结果:2 (返回大于f的最小整数)
var i = Math.floor(f); //结果:1 (返回小于f的最大整数)
6.格式化显示数字
var i = 3.14159;
//格式化为两位小数的浮点数
var str = i.toFixed(2); //结果: "3.14"
//格式化为五位数字的浮点数(从左到右五位数字,不够补零)
var str = i.toPrecision(5); //结果: "3.1415"
7.X进制数字的转换
//不是很懂 -.-
var i = parseInt("0x1f",16);
var i = parseInt(i,10);
var i = parseInt("11010011",2);
8.随机数
//返回0-1之间的任意小数
var rnd = Math.random();
//返回0-n之间的任意整数(不包括n)
var rnd = Math.floor(Math.random() * n)
5.网上搜藏的js堆栈:
function stack(){
if(this.top==undefined){
//初始化堆栈的顶部指针和数据存放域
this.top=0;
this.unit=new Array();
}
this.push=function(pushvalue){
//定义压入堆栈的方法
this.unit[this.top]=pushvalue;
this.top =1;
}
this.readAllElements=function(){
//定义读取所有数据的方法
if(this.top==0){
alert("当前栈空,无法读取数据");
return("");
}
var count=0;
var outStr="";
for(count=0;count
}
return(outStr);
}
this.pop=function(){
//定义弹出堆栈的方法
if(this.top==0){
alert("当前栈空,无法弹出数据");
return("");
}
var popTo=this.unit[this.top-1];
this.top--;
return(popTo);
/* 从堆栈弹出数据,顶部指针减一,不过这里没有做到资源的释放,也
就是说数据仍然存在于this.unit的数组中,只不过无法访问罢了。目前
我也没想到好的办法解决。*/
}
}
6. The most commonly used JavaScript date functions:
·Date type (Date)
1. Declare
var myDate = new Date() ; //Current time of the system
var myDate = new Date(yyyy, mm, dd, hh, mm, ss);
var myDate = new Date(yyyy, mm, dd);
var myDate = new Date("monthName dd, yyyy hh:mm:ss");
var myDate = new Date("monthName dd, yyyy");
var myDate = new Date(epochMilliseconds);
2. Get a part of the time
var myDate = new Date();
myDate.getYear(); //Get the current year (2 digits)
myDate.getFullYear(); //Get the complete year (4 digits, 1970-????)
myDate.getMonth(); //Get the current month (0-11, 0 represents January)
myDate.getDate(); //Get the current day ( 1-31)
myDate.getDay(); //Get the current week X (0-6, 0 represents Sunday)
myDate.getTime(); //Get the current time (milliseconds since 1970.1.1 number) timestamp! !
myDate.getHours(); //Get the current number of hours (0-23)
myDate.getMinutes(); //Get the current number of minutes (0-59)
myDate.getSeconds(); / /Get the current number of seconds (0-59)
myDate.getMilliseconds(); //Get the current number of milliseconds (0-999)
myDate.toLocaleDateString(); //Get the current date
myDate.toLocaleTimeString (); //Get the current time
myDate.toLocaleString( ); //Get the date and time
3. Calculate the previous or future time
var myDate = new Date();
myDate. setDate(myDate.getDate() 10); //The current time plus 10 days
//Similar methods are basically the same, starting with set, for details, refer to point 2
4. Calculate the offset of two dates Amount
var i = daysBetween(beginDate,endDate); //Return the number of days
var i = beginDate.getTimezoneOffset(endDate); //Return the number of minutes
5. Check the valid date
//checkDate () Only allows dates in the two formats of "mm-dd-yyyy" or "mm/dd/yyyy"
if( checkDate("2006-01-01") ){ }
//regular expression (Written by myself to check yyyy-mm-dd, yy-mm-dd, yyyy/mm/dd, yy/mm/dd)
var r = /^(d{2}|d{4}) [/-]d{1,2}[/-]d{1,2}$/;
if( r.test( myString ) ){ }
7. The most commonly used string functions API:
·String (String)
1. Statement
var myString = new String("Every good boy does fine.");
var myString = "Every good boy does fine." ;
2. String concatenation
var myString = "Every " "good boy " "does fine.";
var myString = "Every "; myString = "good boy does fine.";
3. Intercept the string
//Intercept the characters starting from the 6th position
var myString = "Every good boy does fine.";
var section = myString.substring(6); //Result: "good boy does fine."
//Intercept characters starting from digit 0 to digit 10
var myString = "Every good boy does fine.";
var section = myString.substring(0 ,10); //Result: "Every good"
//Intercept the characters from the 11th to the 6th from the bottom
var myString = "Every good boy does fine.";
var section = myString.slice(11,-6); //Result: "boy does"
//Truncate characters with a length of 4 starting from the 6th position
var myString = "Every good boy does fine.";
var section = myString.substr(6,4); //Result: "good"
4. Convert case
var myString = "Hello";
var lcString = myString.toLowerCase( ); //Result: "hello"
var ucString = myString.toUpperCase(); //Result: "HELLO"
5. String comparison
var aString = "Hello!";
var bString = new String("Hello!");
if( aString == "Hello!" ){ } //Result: true
if( aString == bString ){ } //Result: true
if( aString === bString ){ } //Result: false (the two objects are different, although their values are the same)
6. Retrieve the string
var myString = "hello everybody.";
// If it cannot be retrieved, it will return -1. If it is retrieved, it will return the starting position in the string
if( myString.indexOf("every") > -1 ){ }//Result: true
7. Find and replace the string
var myString = "I is your father.";
var result = myString.replace("is","am"); //Result : "I am your father."
8. Special characters:
b: back character t: horizontal tab character
n: newline character v: vertical tab character
f: page break r : Carriage return character
" : Double quote ' : Single quote
\ : Backslash
9. Convert characters to Unicode encoding
var myString = "hello";
var code = myString.charCodeAt(3); //Return the Unicode encoding (integer type) of "l"
var char = String.fromCharCode(66); //Return the character with Unicode 66
10. Convert the string Into URL encoding
var myString = "hello all";
var code = encodeURI(myString); //Result: "hello all"
var str = decodeURI(code); //Result: "hello all"
//The corresponding ones are: encodeURIComponent() decodeURIComponent()
8. Mathematical functions:
·Math object
1. Math.abs(num): Returns the absolute value of num
2. Math.acos(num): Returns the arcsine value of num
3. Math.asin(num): Returns the arcsine value of num
4. Math.atan(num): Returns the arcsine value of num Arctangent
5. Math.atan2(y,x): Returns the arctangent of the quotient of y divided by x
6. Math.ceil(num): Returns the smallest integer greater than num
7 . Math.cos(num): Returns the cosine value of num
8. Math.exp(x): Returns the number raised to the power of x with the natural number as the base
9. Math.floor(num): Returns less than The largest integer of num
10.Math.log(num): Returns the natural logarithm of num
11.Math.max(num1,num2): Returns the larger of num1 and num2
12. Math.min(num1,num2): Returns the smaller of num1 and num2
13.Math.pow(x,y): Returns the value of x raised to the power of y
14.Math.random() : Returns a random number between 0 and 1
15.Math.round(num) : Returns the rounded value of num
16.Math.sin(num) : Returns the sine value of num
17 .Math.sqrt(num): Returns the square root of num
18.Math.tan(num): Returns the tangent value of num
19.Math.E: Natural number (2.718281828459045)
20.Math.LN2 : Natural logarithm of 2 (0.6931471805599453)
21.Math.LN10 : Natural logarithm of 10 (2.302585092994046)
22.Math.LOG2E : Natural number with base log 2 (1.4426950408889634)
23 .Math .LOG10E : Log 10 base natural number (0.4342944819032518)
24.Math.PI : π(3.141592653589793)
25.Math.SQRT1_2 : Square root of 1/2 (0.7071067811865476)
26.Math.SQRT2 : Square root of 2 (1.4142135623730951)
9. Browser feature function:
1. Browser name
//IE : "Microsoft Internet Explorer"
//NS : "Netscape"
var browserName = navigator.appName;
2. Browser version
bar browserVersion = navigator.appVersion;
3. Client operating system
var isWin = ( navigator.userAgent.indexOf("Win" ) != -1 );
var isMac = ( navigator.userAgent.indexOf("Mac") != -1 );
var isUnix = ( navigator.userAgent.indexOf("X11") != - 1 );
4. Determine whether an object, method, or attribute is supported
//When an object, method, or attribute is undefined, undefined or null will be returned. These special values are false
if( document.images ){ }
if( document.getElementById ){ }
5. Check the browser’s current language
if( navigator.userLanguage ){ var l = navigator.userLanguage.toUpperCase(); }
6. Check whether the browser supports Cookies
if( navigator.cookieEnabled ){ }
10. JavaScript object-oriented method implementation inheritance: call method
// animal class animal
function animal(bSex){
this.sex = bSex
this .getSex = function(){
return this.sex
}
}
// Class static variable (if you don’t modify it~~)
animal.SEX_G = new Object( ); // Female
animal.SEX_B = new Object(); // Male
// Animal subclass bird
function bird(bSex){
animal.call(this, bSex);
this.fly = function(iSpeed){
alert("Flying speed up to" iSpeed);
}
}
//Animal sub-category fish
function fish(bSex) {
animal.call(this, bSex);
this.swim = function(iSpeed){
alert("Swimming speed up to" iSpeed)
}
}
/ / Fish-bird hybrid. . .
function crossBF(bSex){
bird.call(this, bSex);
fish.call(this, bSex);
}
var oPet = new crossBF(animal.SEX_G) ; // Female fish bird
alert(oPet.getSex() == animal.SEX_G ? "female" : "male");
oPet.fly(124)
oPet.swim(254)
11. Write JavaScript using object-oriented programming:
MyTool = new function(){
/**
* Returns a non-empty string. If there is a default value, return the default string.
*/
this.notNull = function(str,defaultStr){
if(typeof(str)== "undefined"||str==null||str==''){
if(defaultStr)
return defaultStr;
else
return '';
}
else {
return str;
}
}
}
rootId = MyTool.notNull(rootId,'001000');
12. Commonly used js methods, including some methods for form verification, commonly used methods for drop-down menus, etc.:
/**
* JSON 개체를 문자열로 변환합니다.
* @param {json object} json
* @return {json string}
*/
function jsonObj2Str(json) {
var str = "{";
for (prop in json) {
str = prop ":" json[prop] ",";
}
str = str.substr(0, str.length - 1);
str = "}";
str을 반환합니다;
}
/**
* json 문자열을 json 객체로 변환합니다.
* @param {json string} jsonstr
* @return {json object}
*/
function jsonStr2Obj(jsonstr) {
return eval("(" jsonstr ")");
}
/**
* 요소의 왼쪽 좌표 값을 가져옵니다.
* @param {dom object} obj
* @return {position value}
*/
function getLeft(obj){
var offset=e.offsetLeft;
if(e.offsetParent!=null) offset =getLeft(e.offsetParent);
반품 오프셋;
}
/**
* 요소의 절대 위치의 상단 좌표 값을 가져옵니다.
* @param {dom object} obj
* @return {position value}
*/
function getTop(obj){
var offset=e.offsetTop;
if(e.offsetParent!=null) offset =getTop(e.offsetParent);
반품 오프셋;
}
/**
* 문자열에서 왼쪽 및 오른쪽 공백을 제거합니다.
* @param {original string} str
* @return {공백 제거 후 문자열}
*/
함수 다듬기(str)
{
return str.replace(/(^s*)|(s*$)/g, "");
}
/**
* id를 기준으로 요소를 가져옵니다.
* @param {element id value} str
* @return {dom object}
*/
function $(str) {
return document.getElementById(str);
}
/**
* 이름으로 객체를 가져옵니다.
* @param {요소 이름 값} str
* @return {이름에 따라 반환된 첫 번째 객체}
*/
function $byName(str) {
var arr = document.getElementsByName(str);
if (arr)
return arr[0];
그렇지 않으면
null을 반환합니다.
}
/*************다음 방법은 양식 확인과 관련이 있습니다******************************** * ******************/
/**
* 비어 있지 않은 문자열을 반환합니다. 기본값이 있으면 기본 문자열을 반환합니다.
* @param {변환할 원래 문자열} str
* @param {기본값} defaultStr
* @return {반환 결과}
*/
function notNull(str, defaultStr) {
if (typeof(str) == "정의되지 않음 " || str == null || str == '') {
if (defaultStr)
return defaultStr;
그렇지 않으면
''를 반환합니다.
} else {
str을 반환;
}
}
/**
* 두 날짜의 크기를 비교합니다.
* @param {작은 날짜의 텍스트 상자 ID} smallDate
* @param {큰 날짜의 텍스트 상자 ID} bigDate
* @param {오류 프롬프트 메시지} msg
*/
function CompareTwoDate(smallDate, bigDate, msg) {
var v1 = $(smallDate).value;
var v2 = $(bigDate).value;
if (v1 >= v2) {
경고(msg);
v2.focus();
거짓을 반환합니다.
}
true를 반환합니다.
}
/**
* 두 금액을 비교하는 방법
* @param {더 작은 금액} smallNum
* @param {큰 금액} bigNum
* @param {오류 메시지} msg
* @return { 부울}
*/
function CompareTwoNum(smallNum, bigNum, msg) {
var v1 = $(smallNum).value;
var v2 = $(bigNum).value;
if (parseFloat(v1) >= parseFloat(v2)) {
alert(msg);
v2.focus();
거짓을 반환합니다.
}
true를 반환합니다.
}
/**
* 텍스트 상자의 길이가 지정된 길이를 초과하는지 확인하세요.
* @param {text id} textId
* @param {텍스트 상자의 최대 길이} len
* @param { 텍스트 상자 설명 내용 } msg
* @return {오류가 있으면 false, 없으면 true를 반환}
*/
function checkLength(textId, len, msg) {
obj = $(textId);
str = obj.value;
str = str.replace(/[^x00-xff]/g, "**");
realLen = str.length;
if (realLen > len) {
alert("[" msg "]" "长島最大为" len "位," "请重新输入!n注意:一个汉字 2位。");
obj.focus();
return false;
} else
return true;
}
/**
* テキスト ボックスを空にできないかどうかを決定します。
* @param {テキスト ボックス ID} textId
* @param {テキスト ボックスの説明内容} msg
* @return {存在する場合は false を返します。エラー、それ以外の場合は true を返します}
*/
function checkIfEmpty( textId, msg) {
var textObj = $(textId);
var textValue = textObj.value
if (trim(textValue) == '') {
alert('[' msg '] は空であってはなりません! ');
return false;
}
/* *
* 指定されたテキスト ボックスの内容が電子メールである必要があることを決定します。
* @param {テキスト ボックス ID} textId
* @param {テキスト ボックスの説明} msg
* @return {Ifこれは電子メールのコンテンツです。そうでない場合は true を返し、それ以外の場合は false を返します。}
*/
function checkIsMail(textId, msg) {
var obj = $(textId);
if (!_isEmail(obj.value)) {
alert('[' msg '] は有効な電子メール アドレスではありません! ');
return false;
} else
return true; 🎝> */
function _isEmail(strEmail) {
//次の検証は 2 つ以上の '.' 数字があるかどうかです。ある場合は、それは間違っています。
var first = strEmail.indexOf ('. ');
if (strEmail.indexOf('@')== -1) {
return false;
}
var tempStr = strEmail.substring(最初の 1); 🎜>if (tempStr.indexOf('.') != -1) {
return false;
}
if (strEmail
.search(/^w ((-w )|( .w ) )*@[A-Za-z0-9] ((.|-)[A-Za-z0-9] )*.[A-Za-z0-9] $/) != -1) {
return true;
} else
return false
}
/**
* メールであるかどうかを確認します。
* @param {検証する文字列} strEmail
* @return {Boolean}
*/
function checkIsNum(textId, msg) {
obj = $ (textId) ;
if (isNaN(obj.value)) {
alert('[' msg '] は数値でなければなりません。 ');
obj.focus();
return
} else
return true;
/**
* テキスト ボックスが数値かどうかを判断します。
* @param {テキスト ボックス ID} textId
* @param {テキスト ボックスの説明内容} msg
* @return {Boolean}
*/
関数textId, msg) {
obj = $(textId);
if (!_isValidString(obj.value, '[' msg '] には不正な文字が含まれていてはなりません。')) {
obj.focus();
return false;
}
return true;
}
/**
* Determine whether it is a legal string.
* @param {String to be judged} szStr
* @param {Text description} errMsg
* @return {Return true if it is legal, otherwise return false}
*/
function _isValidString(szStr,errMsg) {
voidChar = "'"><`~!@#$%^&()()!¥……??“”‘'*";
for (var i = 0; i < voidChar.length; i ) {
aChar = voidChar.substring(i, i 1);
if (szStr.indexOf(aChar) > -1){
alert(errMsg)
return false;
}
}
return true;
}
/*************** The following methods are related to drop-down menus****************************** ******************/
/**
* The control drop-down menu cannot be -1 (value=-1 when not selected)
* @param {drop-down menu id} selectId
* @param {drop-down menu description content} msg
* @ param {value corresponding to the null value of the drop-down menu, the default is -1} nullValue
* @return {Boolean}
*/
function checkChooseSelect(selectId, msg ,nullValue) {
var obj = $(selectId);
if (obj.value == notNull(nullValue,'-1')) {
alert('[' msg ']必选!');
obj.focus();
return false;
} else
return true;
}
/**
* Get the displayed text of the drop-down menu.
* @param {drop-down menu dom object} selectObj
* @return {return the displayed "text" of the drop-down menu}
*/
function getSelectText(selectObj) {
return selectObj.options[selectObj.selectedIndex].text;
}
/**
* Get the displayed value of the drop-down menu.
* @param {drop-down menu dom object} selectObj
* @return {Get the displayed "value" of the drop-down menu}
*/
function getSelectValue(selectObj) {
return selectObj.options[selectObj.selectedIndex].value;
}
/**
* Set the selection state of the drop-down menu to the specified value.
* @param {drop-down menu object} obj
* @param {value to be selected} value
*/
function setSelectValue(obj, value) {
/*for (i = obj.options.length - 1; i >= 0; i--) {
if (obj.options[i].value == value) {
obj.options[i].selected = true;
return;
}
}
*/
obj.value= value;
}
/**
* Dynamically assemble the drop-down menu based on the content of the key-value string
* @param {DOM object to be assembled for the drop-down menu} obj
* @param {Key-value pairs are divided by, and;, for example '1,Male;2,Female;3,Unknown'} valAndText
*/
function setSelectContent(obj,valAndText){
if(trim(valAndText)==''){
alert('没有要进行组装下拉菜单的数据!');
return false;
}
clearSelect(obj);
var keyandvalues = valAndText.split(';');
for(var i=0;i
if(arr){
var value =arr[0];
var text =arr[1];
var objOption = new Option(text,value);
obj.add(objOption);
}
}
}
/**
* Clear the contents of the drop-down menu.
* @param {drop-down menu object} obj
*/
function clearSelect(obj) {
for (var i=obj.options.length; i >0; i--) {
obj.remove(0);
}
}
/*************** The following methods are related to the multi-select box****************************** *******************/
/**
* Returns a string consisting of the IDs of the selected checks, separated by commas.
* @param {checks array} checks
* @return A string consisting of the selected IDs
*/
function getCheckedIds(checks){
var selectedValue = '';
var len = checks.length;
for(var index=0; index
selectedValue = checks[index].value ",";
}
}
if(selectedValue.length>0)
return selectedValue.substring(0,selectedValue.length-1);
return selectedValue;
}
将上面的js保存之后,使用下面的html进行测试:
Not empty : | checkIfEmpty('a','non-empty check') < /td> |
Email: | checkIsMail('b','Email verification') |
Number: | checkIsNum('c','Number check') |
Legal characters : | checkIsValid(' d','validity check') |
Small date: Large date: | compareTwoDate('e','f','The relationship between the small date and the large date is wrong!') |
Small number: Large number: | compareTwoNum('k','l','The size and number are not related Correct!') |
Character length check (<5): | checkLength('g',5,'length check') |
Drop-down menu non-empty check: | checkChooseSelect ('h','The drop-down menu is not empty','-1') |
| |
< /td> | getSelectText(sss) and getSelectValue(sss) |
setSelectContent($( 'h'),sss) | |
| getCheckedIds(document.getElementsByName('j')) |

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
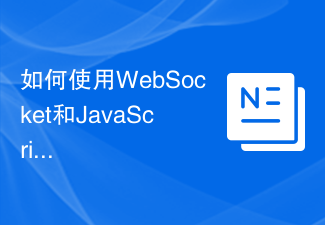
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
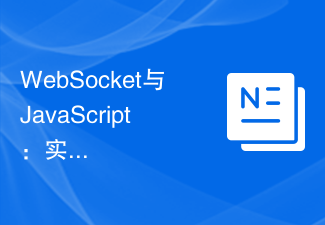
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
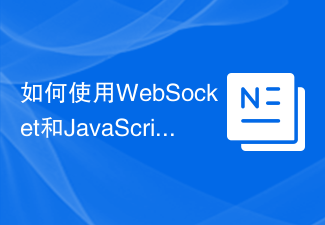
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
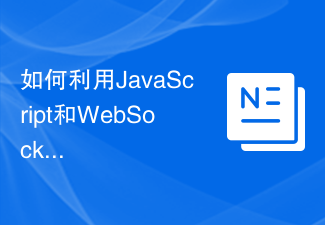
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
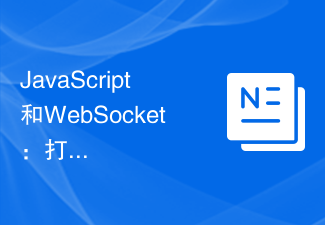
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
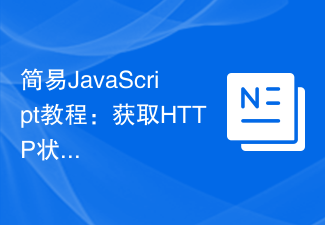
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
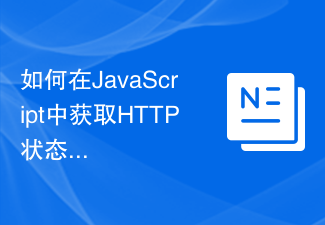
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
