Table of Contents
jQuery)扩展jQuery系列之一 模拟alert,confirm(一)_jquery
May 16, 2016 pm 06:14 PM
alert
效果图
复制代码 代码如下:
/**
* @author xing
*/
(function($){
$.extend({
alert:function(html,callback){
var dialog=new Dialog();
dialog.build('alert',callback,html);
},
confirm:function(html,callback){
var dialog=new Dialog();
dialog.build('confirm',callback,html);
}
});
var Dialog=function(){
var render={
template:'
系统提示
你的操作出现错误!
templateConfirm:'
系统提示
你的操作出现错误!
/**
* 根据需要生成对话框
* @param {Object} type
* @param {Object} callback
* @param {Object} html
*/
renderDialog:function(type,callback,html){
if(type=='alert'){
this.renderAlert(callback,html);
}else{
this.renderConfirm(callback,html);
}
},
/**
* 生成alert
* @param {Object} callback
* @param {Object} html
*/
renderAlert:function(callback,html){
var temp=$(this.template).clone().hide();
temp.find('div.alertHtml').html(html);
$(document.body).append(temp);
this.setPosition(temp);
temp.fadeIn();
this.bindEvents('alert',temp,callback);
},
/**
* 生成confirm
* @param {Object} callback
* @param {Object} html
*/
renderConfirm:function(callback,html){
var temp=$(this.templateConfirm).clone().hide();
temp.find('div.alertHtml').html(html);
$(document.body).append(temp);
this.setPosition(temp);
temp.fadeIn();
this.bindEvents('confirm',temp,callback);
},
/**
* 设定对话框的位置
* @param {Object} el
*/
setPosition:function(el){
var width=el.width(),
height=el.height(),
pageSize=this.getPageSize();
el.css({
top:(pageSize.h-height)/2,
left:(pageSize.w-width)/2
});
},
/**
* 绑定事件
* @param {Object} type
* @param {Object} el
* @param {Object} callback
*/
bindEvents:function(type,el,callback){
if(type=="alert"){
if($.isFunction(callback)){
$('#sure').click(function(){
callback();
$('div.alertParent').remove();
});
}else{
$('#sure').click(function(){
$('div.alertParent').remove();
});
}
}else{
if($.isFunction(callback)){
$('#sure').click(function(){
callback();
$('div.alertParent').remove();
});
}else{
$('#sure').click(function(){
$('div.alertParent').remove();
});
}
$('#cancel').click(function(){
$('div.alertParent').remove();
});
}
},
/**
* 获取页面尺寸
*/
getPageSize:function(){
return {
w:document.documentElement.clientWidth,
h:document.documentElement.clientHeight
}
}
}
return {
build:function(type,callback,html){
render.renderDialog(type,callback,html);
}
}
}
})(jQuery);
因为我们的alert,并不需要选择器的支持,所以我们采用$.extend这样声明
复制代码 代码如下:
$.extend({
alert:function(html,callback){
},
confirm:function(html,callback){
}
});
其次我们声明一个单体来生成这个组件到页面,这个单体返回一个公共的方法build来创建这个组件
复制代码 代码如下:
var Dialog=function(){
return {
build:function(type,callback,html){
render.renderDialog(type,callback,html);
}
}
}
接下来我们分别声明组件的HTML字符串
复制代码 代码如下:
var render={
template:'
系统提示
你的操作出现错误!
templateConfirm:'
id="confirmPanel">
'}系统提示
你的操作出现错误!
id="cancel"/>
向里面填充方法
复制代码 代码如下:
/**
* 根据需要生成对话框
* @param {Object} type
* @param {Object} callback
* @param {Object} html
*/
renderDialog:function(type,callback,html){
if(type=='alert'){
this.renderAlert(callback,html);
}else{
this.renderConfirm(callback,html);
}
},
/**
* 生成alert
* @param {Object} callback
* @param {Object} html
*/
renderAlert:function(callback,html){
var temp=$(this.template).clone().hide();
temp.find('div.alertHtml').html(html);
$(document.body).append(temp);
this.setPosition(temp);
temp.fadeIn();
this.bindEvents('alert',temp,callback);
},
/**
* 生成confirm
* @param {Object} callback
* @param {Object} html
*/
renderConfirm:function(callback,html){
var temp=$(this.templateConfirm).clone().hide();
temp.find('div.alertHtml').html(html);
$(document.body).append(temp);
this.setPosition(temp);
temp.fadeIn();
this.bindEvents('confirm',temp,callback);
},
/**
* 设定对话框的位置
* @param {Object} el
*/
setPosition:function(el){
var width=el.width(),
height=el.height(),
pageSize=this.getPageSize();
el.css({
top:(pageSize.h-height)/2,
left:(pageSize.w-width)/2
});
},
/**
* 绑定事件
* @param {Object} type
* @param {Object} el
* @param {Object} callback
*/
bindEvents:function(type,el,callback){
if(type=="alert"){
if($.isFunction(callback)){
$('#sure').click(function(){
callback();
$('div.alertParent').remove();
});
}else{
$('#sure').click(function(){
$('div.alertParent').remove();
});
}
}else{
if($.isFunction(callback)){
$('#sure').click(function(){
callback();
$('div.alertParent').remove();
});
}else{
$('#sure').click(function(){
$('div.alertParent').remove();
});
}
$('#cancel').click(function(){
$('div.alertParent').remove();
});
}
},
/**
* 获取页面尺寸
*/
getPageSize:function(){
return {
w:document.documentElement.clientWidth,
h:document.documentElement.clientHeight
}
}
接下来就是对话框的实现
复制代码 代码如下:
$.extend({
alert:function(html,callback){
var dialog=new Dialog();
dialog.build('alert',callback,html);
},
confirm:function(html,callback){
var dialog=new Dialog();
dialog.build('confirm',callback,html);
}
});
调用方法:
复制代码 代码如下:
$.confirm('确定删除?',function(){
alert('cccc');
});
复制代码 代码如下:
div.alertParent{
padding:6px;
background:#ccc;
background:rgba(201,201,201,0.8);
width:auto;
position:absolute;
-moz-border-radius:3px;
font-size:12px;
top:50px;
left:50px;
}
div.alertContent{
background:#fff;
width:350px;
height:auto;
border:1px solid #999;
}
h2.title{
width:100%;
height:28px;
background:#0698E9;
text-indent:10px;
font-size:12px;
color:#fff;
line-height:28px;
margin:0;
}
div.alertHtml{
background:url(dtips.gif) 0 0 no-repeat;
height:50px;
margin:10px;
margin-left:30px;
text-indent:50px;
line-height:50px;
font-size:14px;
}
div.btnBar{
border-top:1px solid #c6c6c6;
background:#f8f8f8;
height:30px;
}
div.btnBar input{
background:url(sure.png) no-repeat;
border:0;
width:63px;
height:28px;
float:right;
margin-right:5px;
}
html
复制代码 代码如下:
系统提示
你的操作出现错误!
type="button" value="确定"/>
高手勿笑,为了说明实现的方式,我并没有仔细的去完善这段代码,仅仅是在写作的半小时内写出的,所以有很多地方没有去思考,有很多的纰漏,并且以一个比较笨的方式实现了这个模拟的alert,不过下次我们将通过构建Button的方式实现一个组件,会加入遮罩,ajax调用,iframe宽度高度自适应等更强大的功能。
Statement of this Website
The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn

Hot Article
Repo: How To Revive Teammates
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
How Long Does It Take To Beat Split Fiction?
3 weeks ago
By DDD
R.E.P.O. Energy Crystals Explained and What They Do (Yellow Crystal)
1 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
Hello Kitty Island Adventure: How To Get Giant Seeds
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌

Hot tools Tags

Hot Article
Repo: How To Revive Teammates
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
How Long Does It Take To Beat Split Fiction?
3 weeks ago
By DDD
R.E.P.O. Energy Crystals Explained and What They Do (Yellow Crystal)
1 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌
Hello Kitty Island Adventure: How To Get Giant Seeds
3 weeks ago
By 尊渡假赌尊渡假赌尊渡假赌

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
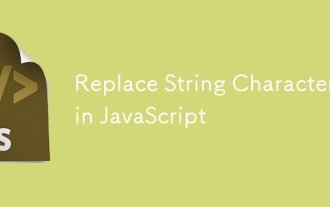
Replace String Characters in JavaScript
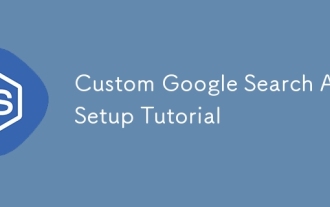
Custom Google Search API Setup Tutorial
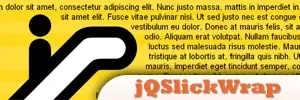
8 Stunning jQuery Page Layout Plugins
