Jquery operates cookies to remember username_jquery
1. Introduction to jquery.cookie.js
jquery.cookie.js is a jquery-based plug-in, a lightweight cookie plug-in that can read, write, and delete cookies.
jquery.cookie.js can get the source code from Github https://github.com/carhartl/jquery-cookie
2. Introduction to the basic usage of jquery.cookie.js
jQuery plug-in to operate cookies, the approximate usage method is as follows:
1. Read Cookie value
$.cookie('the_cookie'); //Return cookieValue if it exists, otherwise return null.
2. Set cookie value
(1) Default setting. When the cookie time is not specified, the created cookie is valid until the user's browser is closed by default, so it is called a session cookie.
$.cookie('the_cookie', 'the_value');
(2) Set a cookie with time. When the time is specified, it is called a persistent cookie, and the validity time is days.
$.cookie('cookieName','cookieValue', {expires:7});
(3) Set cookie with path. If a valid path is not set, by default, the cookie can only be read on the current page of cookie settings. The path of the cookie is used to set the top-level directory that can read the cookie.
$.cookie('cookieName','cookieValue', {expires:7, path:'/'});
(4) Set cookies for specific websites.
$.cookie('cookieName','cookieValue',{expires:7, path:'/' , domain: 'souvc.com' , secure: false , raw:false});
Parameter explanation:
1).expires: 365
Define the validity time of the cookie. The value can be a number (in days from the time the cookie is created) or a Date object. If omitted, the cookie created is a session cookie and will be deleted when the user exits the browser.
//Note: By default, only the web page that sets the cookie can read the cookie. If you want a page to read the cookie set by another page, you must set the cookie path. The path to the cookie is used to set the top-level directory that can read the cookie. Setting this path to the root directory of the website allows all web pages to read each other's cookies (generally do not set this to prevent conflicts).
expires: (Number | Date) Validity period, you can set an integer as the validity period (unit: days), or you can set a date object as the expiration date of the cookie. If the specified date is a negative number, then this cookie will be deleted; if not set or set to null, then this cookie will be treated as a Session Cookie and deleted after the browser is closed.
var COOKIE_NAME = 'username'; if( $.cookie(COOKIE_NAME) ){ $("#username").val( $.cookie(COOKIE_NAME) ); } $("#check").click(function(){ if(this.checked){ $.cookie(COOKIE_NAME, $("#username").val() , { path: '/', expires: 10 }); //var date = new Date(); //date.setTime(date.getTime() + (3 * 24 * 60 * 60 * 1000)); //三天后的这个时候过期 //$.cookie(COOKIE_NAME, $("#username").val(), { path: '/', expires: date }); }else{ $.cookie(COOKIE_NAME, null, { path: '/' }); //删除cookie } });
2).path: '/'
Default: Only the webpage that sets the cookie can read the cookie. Defines the valid path of the cookie. By default, the value of this parameter is the path to the web page that created the cookie (standard browser behavior). If you want to access this cookie throughout the website, you need to set the effective path like this: path: '/'.
If you want to delete a cookie that defines a valid path, you need to include this path when calling the function: $.cookie('the_cookie', null, { path: '/' });. domain: 'example.com' Default value: The domain name of the web page that created the cookie.
3). domain: The domain name owned by the webpage where the cookie is created;
4). secure: the default is false, if true, the cookie transmission protocol needs to be https; raw: the default is false, automatic encoding and decoding when reading and writing (use encodeURIComponent to encode, use decodeURIComponent to decode) , to turn off this function, please set it to true.
3. Delete cookies.
$.cookie('the_cookie', null); //Delete a cookie
$.cookie('cookieName',null,{path:'/'}); //Note: If you want to delete a cookie with a valid path
3. How to use
Include the jQuery library file first, and then include the jquery.cookie.js library file.
<script type="text/javascript" src="js/jquery-1.6.2.min.js"></script> <script type="text/javascript" src="js/jquery.cookie.js"></script>
4. Brief description.
1. Page effect
2. jsp page:
<input type="text" class="lr-input" placeholder="手机号码/用户名" style="width:255px" id="username" name="username" value=""/> <input type="password" class="lr-input" placeholder="请输入登录密码" style="width:255px" id="password" name="password" /> <div class="lr-formWrap fn-clear"> <p class="lr-remUser fn-left" id="remUserSelect"><i class="icon-check"></i>记住用户</p> <a href="javascript:void(0)" id="login-submit" class="lr-submit">登录</a>
3. css style:
.lr-remUser { color: #9d9d9d; cursor: pointer; font-size: 14px; line-height: 25px; padding-left: 30px; }
4. js implementation
//按照状态读取是否显示昵称 if ($.cookie("rmbUser") == "true") { $("#remUserSelect").addClass("active");//如果是选中,那么给上选中的标志 $("#username").val($.cookie("nickName"));//记录账号 } //验证记住帐号 function vailRememberNickName(){ if($("#remUserSelect").hasClass("active")){ var nickName = $("#username").val(); $.cookie("rmbUser", "true", { expires: 7 }); // 存储一个带7天期限的 cookie $.cookie("nickName", nickName, { expires: 7 }); // 存储一个带7天期限的 cookie }else { $.cookie("rmbUser", "false", { expires: -1 }); $.cookie("nickName", '', { expires: -1 }); } }
5. Then call this method when you click to log in.
//登录提交表单 $("#login-submit").on("click",function(){ var form = $("#loginForm"); if(!vailPhone())return; if(!vailPwd())return; vailRememberNickName(); form.submit(); });
6. Log in to view the browser console effect as follows:
7. When logging out, you can see the effect of the login box:
The above content is the relevant instructions of Jquery operating cookies to remember user names introduced by the editor. I hope it will be helpful to everyone!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
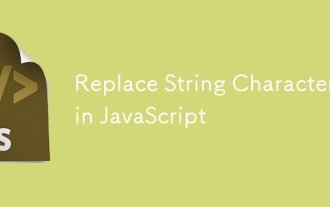
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
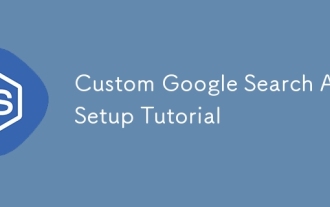
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
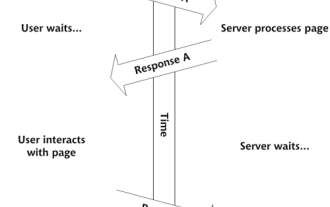
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
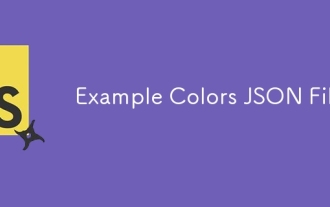
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
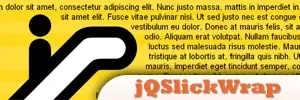
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
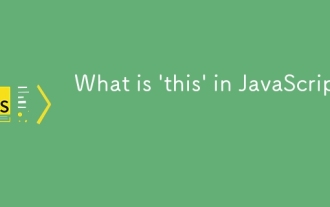
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was
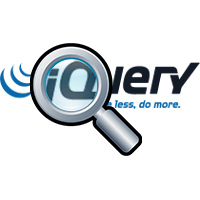
jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
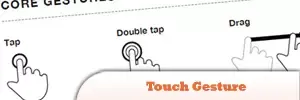
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig
