Implementation of standard Get and Set accessors
function Field( val){
this.value = val;
}
Field.prototype = {
get value(){
return this._value;
},
set value (val){
this._value = val;
}
};
var field = new Field("test");
field.value="test2";
//field.value will now return "test2"
Can work normally in the following browsers:

Our commonly used implementation method may be like this:
function Field(val){
var value = val;
this.getValue = function(){
return value;
};
this.setValue = function(val){
value = val;
};
}
var field = new Field("test");
field.setValue("test2")
field .getValue() // return "test2"
Implementation of Get and Set accessors on DOM elements
HTMLElement.prototype.__defineGetter__("description", function () {
return this.desc;
});
HTMLElement .prototype.__defineSetter__("description", function (val) {
this.desc = val;
});
document.body.description = "Beautiful body";
// document. body.description will now return "Beautiful body";
can work normally in the following browsers:

Implement the accessor through Object.defineProperty
In the future, the ECMAScript standard method of extending objects will be implemented through Object.defineProperty, which is why IE8 implements get and set accessors through this method. It seems that Microsoft is still very far-sighted, unfortunately. Currently, only IE8 and Chrome 5.0 support it, and other browsers do not support it. Moreover, IE8 only supports DOM elements, but future versions will support ordinary Javascript objects like Chrome.
Implementation of Get and Set accessors on DOM elements
Object.defineProperty(document.body, "description", {
get : function () {
return this.desc;
},
set : function (val) {
this.desc = val;
}
});
document.body.description = "Content container";
// document.body.description will now return "Content container"
Can work normally in the following browsers:
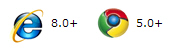
Implementation of Get and Set accessors for ordinary objects
var lost = {
loc : "Island"
};
Object.defineProperty(lost, "location", {
get : function () {
return this.loc;
},
set : function (val) {
this.loc = val;
}
});
lost.location = "Another island" ;
// lost.location will now return "Another island"
can work normally in the following browsers:

Summary of this article
Although Microsoft's IE only supports Object.defineProperty and does not perfectly implement the Get and Set accessors, we have seen great progress in IE, especially the new javascript engine used by the just-released IE9. It supports HTML5 and CSS3, supports hardware acceleration, etc. I believe that one day all browsers will be able to fully embrace standards and bring a perfect WEB world.
Reference:
1. Getters and setters with JavaScript
2. JavaScript Getters and Setters
Author: Dream