Several functions under jQuery that you may not have used_jquery
Speaking of dropdown list, in html you will think of
But Its display will not look good. We can use div ul to make a drop down list ourselves, and it has very painful slidedown and slideup functions.
Effect comparison under IE8:

First let’s talk about the idea, a very simple idea,
a. A Div needs to be used instead of drop down list Select the container where the record is displayed, and use offset to get the position where the Div should be displayed, offset.top and offset.left.
b. Simulate a drop-down box through a UL and its children li. There are several issues that need to be noted here.
i: The UL must be placed in a newly created Div, and the position of this Div is far from the top of the Div in the previous step (we call it iDiv) of the top data. iDiv.height;
ii: Be sure to clear it every time after clicking on an li element, otherwise your drop down list will become longer and longer. . .
iii: When the mouse is clicked elsewhere, the dropdown list must be hidden.
Let me explain how to implement this function step by step with the code:
1. Create an iDiv as a container for the selected value of the drop down list.
Before creating iDiv we need to first get the position where we want to display this drop down list:
// get the select list 's position using offset,width and height
var offset = $(".select_css").offset();
var width = $(".select_css").width();
var height = $(".select_css").height();
The next step is to create the iDivb and use css() Method to add formatting to iDiv.
var iDiv = $("
'top': offset.top,
'left': offset.left,
'width': width,
' height': height,
'border': '1px solid #aaaaaa',
'fontSize': '12px',
'textIndent': '4px',
'cursor': 'default ' }).text("hello");
iDiv also added a class='iDiv', which was originally unnecessary, but then I found that jQuery's css() cannot handle the background. For the no-repeat attribute of the image, there is no example for foreigners after searching for a long time, so I can only set it through this clas='iDiv':
.iDiv
{
background-image:url('images/select_right.gif');
background-position:right;
background-repeat:no-repeat;
}
The effect is as follows;
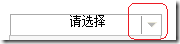
2. When a click event occurs on iDiv, create a Drop down box and use slidedown effect.
First we need to create a cDiv and add it to the body of the html. Its position is just below the iDiv, so the cDiv needs to be created as follows:
var cDiv = $("
'position': 'absolute',
'width': width,
'top': offset.top 22,
'left': offset.left,
'background': '# f7f7f7',
'border': '1px solid silver'
}).hide();
And by default we want it to be hidden.
With this cDiv, we only need to create a UL list when the iDiv Click event occurs, and append it to the cDiv.
var UL = $("
for (var i = 1; i < 10; i ) {
$("
$(this).css(
{
'color': 'white',
'background' : 'gray'
}
);
}).mouseout(function () {
$(this).css(
{
'color': 'black',
'background': 'white'
});
}).click(function () {
// disvisualble the cDiv and set the selected crrent li's text as iDiv's text
$ ("#cDiv").slideUp().hide();
$("#iDiv").html($(this).html());
});
}
// slide show the cDiv now
$("#cDiv").slideDown('slow');
You can see that each li record is added when it is added mouseover, mouseout and click events.
When the click event occurs, we not only need to give the cDiv to slideUp but also hide it and clear the cDiv before clicking the iDiv next time. These two points are very important. You can try what will happen if you don't do these two things.
Don’t forget to copy the html content of the current li to iDiv when clicking li, otherwise our control will have no practical effect. . . . .
3. Use Ajax to get the value of the drop-down list from the server.
Many times we need to dynamically obtain the value of the drop-down list from the server, so when clicking on the iDiv, we need to first load the data from the server through jQuey's ajax method (or other ajax method), and when the data load is completed We just started creating the UL list.
I am using WCF Servece as the data source for ajax requests.
In order to increase user-friendliness, when fetching data from the server, we let the iDiv display as a load image. . . . . . . . . . . .
Code download: http://xiazai.jb51.net/201008/yuanma/jQueryAJAXCallWCFService.rar2. Use jQuery’s append function to switch the played video file (flash or Silverlight player) without refreshing.
There was a minisite that needed this thing before. I tried researching it, and it really works.
http://haokan.lafaso.com/pretty.html Everyone can take a look. I don’t think this is an advertisement. Most of us are men and will not read this. hehe. It’s just that I think you might be able to use this method in the future.
Since these players are all embed controls, we can play different videos by replacing the src attribute of embed. For example:
Using jQuery's append() method, we can change the src of embed and replace the html of div1 again. It is like using ajax technology on the page.
$("#div1 embed").empty() ;
var placeHolder = $("");
var tempParent = $("");
var embed = $("#div1 embed" );
embed.replaceWith(placeHolder);
tempParent.append(embed);
embed.attr("src", http://player.ku6.com/refer/DMFZdNYzKDEosiPG/v.swf&auto =1);
placeHolder.replaceWith(tempParent.html());
3. Use jQuery to implement header and footer functions for HTML.
In php and asp.net, there are controls such as header and footer. In php, we use include, but in asp.net, we use master or ascx. What about
in html? I believe there never has been. But the customer asked us to make the page must be html, saying that we were afraid of too many users. . . . .
The advantage of using footer and header is that when we need to modify the content of these parts, we only need to modify one page, and all pages will change.
Later I found a way to use jquery’s load() method.
First we need to add two Divs in the html, one at the top of and one at the bottom. It is best to have an Id='header' and an id='footer'.
Then on the server side we only need to create a header.html and a footer.html.
When the page is loaded, we will use jquery's load method to load header.html and footer.html.
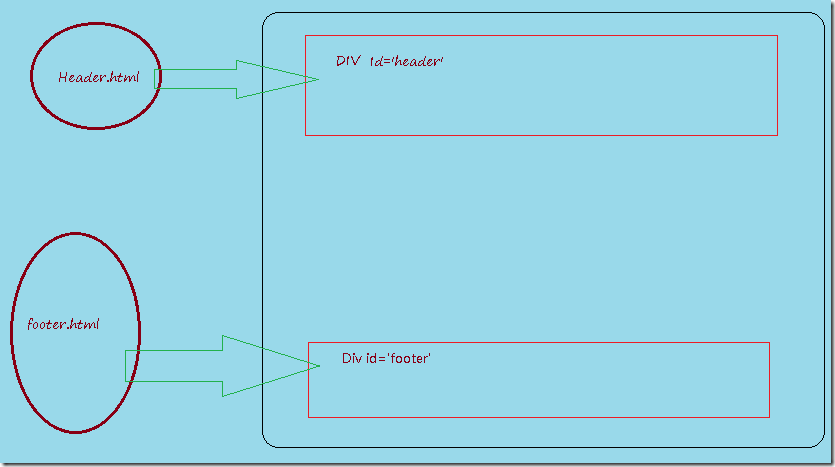
Code:
$("#header").load("controls/header.html", function (response, status, xhr) {
if (status == "error") {
var msg = "Server data transmission error, please refresh the page";
// $("#error").html(msg xhr.status " " xhr.statusText);
alert(msg);
}
});
// load footer from server
$("#footer").load("controls/footer.html", function (response, status, xhr) {
if (status == "error") {
var msg = "Server data transmission error, please refresh the page";
// $("#error").html(msg xhr.status " " xhr .statusText);
alert(msg);
}
});
If possible, I will summarize some jQuery techniques and share them with you later. . . . . . . . I will also provide you with the download address for the code for the drop-down box later.
Cheers
Nic
Code download: http://xiazai.jb51.net/201008/yuanma/jQueryAJAXCallWCFService.rar

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


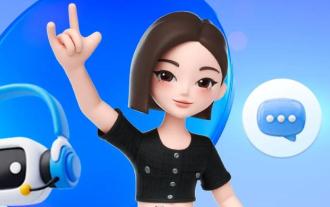
There will be many AI creation functions in the Doubao app, so what functions does the Doubao app have? Users can use this software to create paintings, chat with AI, generate articles for users, help everyone search for songs, etc. This function introduction of the Doubao app can tell you the specific operation method. The specific content is below, so take a look! What functions does the Doubao app have? Answer: You can draw, chat, write articles, and find songs. Function introduction: 1. Question query: You can use AI to find answers to questions faster, and you can ask any kind of questions. 2. Picture generation: AI can be used to create different pictures for everyone. You only need to tell everyone the general requirements. 3. AI chat: can create an AI that can chat for users,
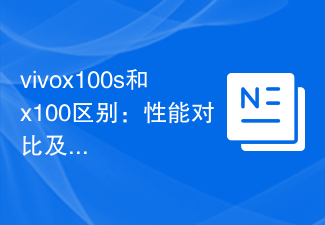
Both vivox100s and x100 mobile phones are representative models in vivo's mobile phone product line. They respectively represent vivo's high-end technology level in different time periods. Therefore, the two mobile phones have certain differences in design, performance and functions. This article will conduct a detailed comparison between these two mobile phones in terms of performance comparison and function analysis to help consumers better choose the mobile phone that suits them. First, let’s look at the performance comparison between vivox100s and x100. vivox100s is equipped with the latest
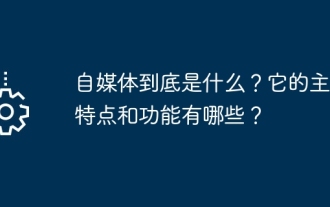
With the rapid development of the Internet, the concept of self-media has become deeply rooted in people's hearts. So, what exactly is self-media? What are its main features and functions? Next, we will explore these issues one by one. 1. What exactly is self-media? We-media, as the name suggests, means you are the media. It refers to an information carrier through which individuals or teams can independently create, edit, publish and disseminate content through the Internet platform. Different from traditional media, such as newspapers, television, radio, etc., self-media is more interactive and personalized, allowing everyone to become a producer and disseminator of information. 2. What are the main features and functions of self-media? 1. Low threshold: The rise of self-media has lowered the threshold for entering the media industry. Cumbersome equipment and professional teams are no longer needed.
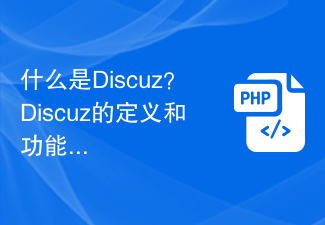
"Exploring Discuz: Definition, Functions and Code Examples" With the rapid development of the Internet, community forums have become an important platform for people to obtain information and exchange opinions. Among the many community forum systems, Discuz, as a well-known open source forum software in China, is favored by the majority of website developers and administrators. So, what is Discuz? What functions does it have, and how can it help our website? This article will introduce Discuz in detail and attach specific code examples to help readers learn more about it.
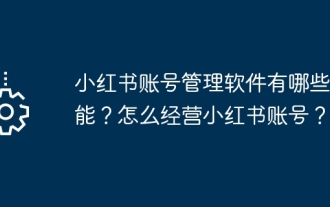
As Xiaohongshu becomes popular among young people, more and more people are beginning to use this platform to share various aspects of their experiences and life insights. How to effectively manage multiple Xiaohongshu accounts has become a key issue. In this article, we will discuss some of the features of Xiaohongshu account management software and explore how to better manage your Xiaohongshu account. As social media grows, many people find themselves needing to manage multiple social accounts. This is also a challenge for Xiaohongshu users. Some Xiaohongshu account management software can help users manage multiple accounts more easily, including automatic content publishing, scheduled publishing, data analysis and other functions. Through these tools, users can manage their accounts more efficiently and increase their account exposure and attention. In addition, Xiaohongshu account management software has
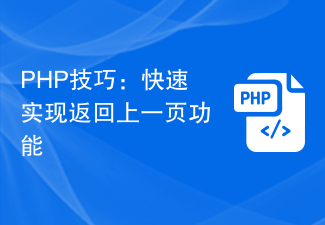
PHP Tips: Quickly implement the function of returning to the previous page. In web development, we often encounter the need to implement the function of returning to the previous page. Such operations can improve the user experience and make it easier for users to navigate between web pages. In PHP, we can achieve this function through some simple code. This article will introduce how to quickly implement the function of returning to the previous page and provide specific PHP code examples. In PHP, we can use $_SERVER['HTTP_REFERER'] to get the URL of the previous page
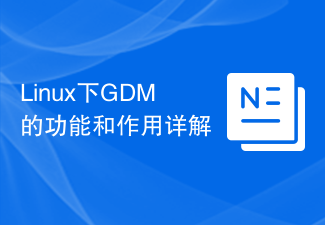
Detailed explanation of the functions and functions of GDM under Linux In the Linux operating system, GDM (GNOMEDisplayManager) is a graphical login manager that provides an interface for users to log in and log out of the system. GDM is usually part of the GNOME desktop environment, but can be used by other desktop environments as well. The role of GDM is not only to provide a login interface, but also includes user session management, screen saver, automatic login and other functions. The functions of GDM mainly include the following aspects:
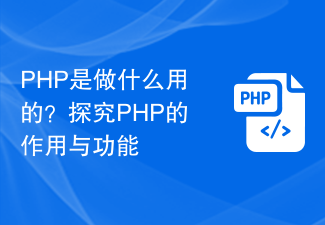
PHP is a server-side scripting language widely used in web development. Its main function is to generate dynamic web content. When combined with HTML, it can create rich and colorful web pages. PHP is powerful. It can perform various database operations, file operations, form processing and other tasks, providing powerful interactivity and functionality for websites. In the following articles, we will further explore the role and functions of PHP, with detailed code examples. First, let’s take a look at a common use of PHP: dynamic web page generation: P
