


Organize javascript code under jquery (js functionalization)_Basic knowledge
Starting with the magical "$" function
The "$" function will bind an event to a specified button after the document is loaded. These codes work fine in a single web page. But if we have other pages, we will have to repeat this process.
What if we need another action for the button? For example, like this:
Next, more questions arise. We need a lot of buttons like this, which seems not difficult.
However, not all pages will use these two kinds of buttons. In order not to To use additional selectors on the page, we need to make some necessary adjustments, because the performance of class-based selectors is very expensive compared to id selectors. It requires traversing all DOM elements and using regular expressions to match the class attribute to select elements that satisfy the condition.
if($page == 'A' ){?>
} ?>
if($page == 'B'){?>
} ?>
Our project functions are becoming more and more complex. After a period of time, it became like this, quick but dirty...
if($page == 'A' or $page == "C" and $page is not "D"){ ?> ;
} ?>
if ($page == "B" or $page == "E" and $page is not "X"){ ?>
} ?>
if($page == "B" or $page == "E" or $page == "C "){ ?>
} ?>
This is really bad. We need to load many code snippets on one page to bind all events. If we load different codes into multiple js files, this will increase the resource consumption of multiple pages. HTTP requests will face challenges both in management and user experience, and we need to find a better solution.
Since the overhead of class selector is so high, can we bind all events in one scan? We can try it:
In more complex scenarios, we can use the inline code on the page to pass some necessary information to the component.
Yottaa.globalConst = {
User:{
familyName: "Jhone",
givenName: 'bruce'
},
Url:{
siteName: 'yottaa.com',
score: 98
}
}
Yottaa.componentMetaData = {
compoment_id_1:{ ...... },
component_id_2:{ ...... }
};
The above discusses a possible code organization method, but it is not suitable for all projects. What we have to do is: find a relatively low-cost refactoring solution based on the current status quo. We consider the following points:
Separate the event binding code and component code of the element: the component code includes the jquery library, related extension plug-ins, and widgets written by ourselves, such as chartbox and other content.
Event binding and processing logic: divided into multiple modules according to different components, and each module is placed in a function.
The page needs to specify which modules are to be initialized on this page, and provide a list for unified processing by the global event binder.
Let’s demonstrate part of the code:
< script type="text/javascript">
function init_loginPanel = function(){
var container = $('login_panel');
$('#login_button').click(function(){
......
});
}
function init_chart = function(){
......
}
//global static init method
Yottaa.initComponents = function(components){
for(var i = 0;i
window[components[i]]();
}
}
}
// above is in the 'all-in-one' assets file which is compressed to one file in production.
var components = ['init_loginPanel', 'init_chart'];
var metaData = {
loginPanel: {},
chart: {},
.... ..
};
$(function(){
Yottaa.initComponents(components);
});
//here is inline script on the page.
< /script>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


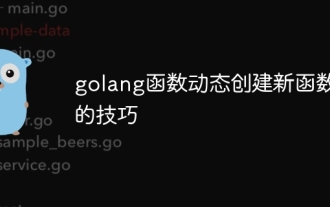
Go language provides two dynamic function creation technologies: closure and reflection. closures allow access to variables within the closure scope, and reflection can create new functions using the FuncOf function. These technologies are useful in customizing HTTP routers, implementing highly customizable systems, and building pluggable components.
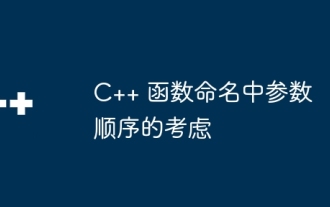
In C++ function naming, it is crucial to consider parameter order to improve readability, reduce errors, and facilitate refactoring. Common parameter order conventions include: action-object, object-action, semantic meaning, and standard library compliance. The optimal order depends on the purpose of the function, parameter types, potential confusion, and language conventions.
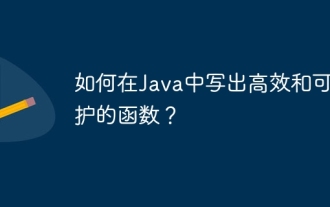
The key to writing efficient and maintainable Java functions is: keep it simple. Use meaningful naming. Handle special situations. Use appropriate visibility.
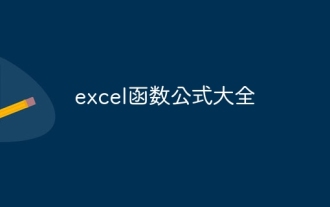
1. The SUM function is used to sum the numbers in a column or a group of cells, for example: =SUM(A1:J10). 2. The AVERAGE function is used to calculate the average of the numbers in a column or a group of cells, for example: =AVERAGE(A1:A10). 3. COUNT function, used to count the number of numbers or text in a column or a group of cells, for example: =COUNT(A1:A10) 4. IF function, used to make logical judgments based on specified conditions and return the corresponding result.
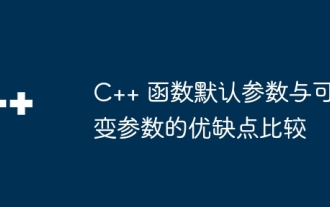
The advantages of default parameters in C++ functions include simplifying calls, enhancing readability, and avoiding errors. The disadvantages are limited flexibility and naming restrictions. Advantages of variadic parameters include unlimited flexibility and dynamic binding. Disadvantages include greater complexity, implicit type conversions, and difficulty in debugging.
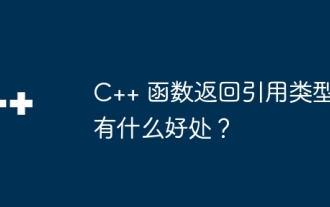
The benefits of functions returning reference types in C++ include: Performance improvements: Passing by reference avoids object copying, thus saving memory and time. Direct modification: The caller can directly modify the returned reference object without reassigning it. Code simplicity: Passing by reference simplifies the code and requires no additional assignment operations.
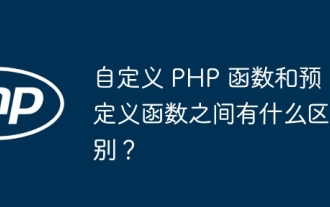
The difference between custom PHP functions and predefined functions is: Scope: Custom functions are limited to the scope of their definition, while predefined functions are accessible throughout the script. How to define: Custom functions are defined using the function keyword, while predefined functions are defined by the PHP kernel. Parameter passing: Custom functions receive parameters, while predefined functions may not require parameters. Extensibility: Custom functions can be created as needed, while predefined functions are built-in and cannot be modified.
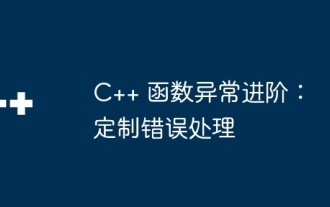
Exception handling in C++ can be enhanced through custom exception classes that provide specific error messages, contextual information, and perform custom actions based on the error type. Define an exception class inherited from std::exception to provide specific error information. Use the throw keyword to throw a custom exception. Use dynamic_cast in a try-catch block to convert the caught exception to a custom exception type. In the actual case, the open_file function throws a FileNotFoundException exception. Catching and handling the exception can provide a more specific error message.
