Understanding and examples of javascript closures_javascript skills
By the way, a reminder:
Lexical scope: The scope of a variable is determined when it is defined rather than when it is executed. That is to say, the lexical scope depends on the source code and can be determined through static analysis. Therefore, the lexical scope is also called static Scope. Except for with and eval, it can only be said that the scope mechanism of JS is very close to lexical scope (Lexical scope).
The following is a simple closure example using global variables:
var sWord="Hello, Welcome to the blog of a web front-end development engineer, please give us your advice."
function disWord(){
alert(sWord);
}
disWord();
Analysis: When the script is loaded into the memory, disWord does not calculate the value of sWord, but performs the calculation of sWord when the function disWord is called.
The following is an example of a closure within a function that defines another function:
var iNum=10;
function add(num1,num2){
function doAdd(){return num1 num2 iNum;}
return doAdd();
}
Analysis: The internal function doAdd is a closure. It will get the value of the incoming parameters num1, num2 and the global variable iNum. doAdd does not accept parameters. The last step of add calls doAdd. Please take two parameters and the global variable iNum. The variables are summed and returned. It can be seen that the value used by doAdd is obtained in the execution environment.
The following are a few examples found on the Internet, understanding lexical scope and closure
, Case 1
/*A piece of code under the global (window) domain*/
function a(i) {
var i ;
alert(i);
};
a(10);
Question: What will the above code output?
Answer: 10.
Specific execution process
The a function has a formal parameter i. When calling the a function, the actual parameter 10 is passed in, and the formal parameter i=10
Then define a local variable i with the same name, which is not assigned a value
alert Output 10
Thinking: Are local variable i and formal parameter i the same storage space?
, Case 2
1 /*Global (window ) A piece of code under the domain */
2 function a(i) {
3 alert(i);
4 alert(arguments[0]); //arguments[0] should be the formal parameter i
5 var i = 2;
6 alert(i);
7 alert(arguments[0]);
8 };
9 a(10);
Question: What will the above code output?
Answer: 10,10,2,2
Specific execution process
The function has a formal parameter i, and the actual value is passed in when calling the a function Parameter 10, formal parameter i=10
The first alert outputs the value 10 of the formal parameter i
The second alert outputs arguments[0], which should also be i
Then define a local variable i and The assigned value is 2. At this time, the local variable i=2
The third alert outputs the value 2 of the local variable i
The fourth alert outputs argumentsa[0] again
Thinking: This can explain the local Are the values of variable i and formal parameter i the same?
, case three
/*Global A piece of code under the (window) domain*/
function a(i) {
var i = i;
alert(i);
};
a(10)
Question: What will the above code output?
Answer: 10
Specific execution process
The first sentence declares a local variable i with the same name as the formal parameter i. According to the result, we know that the latter i points to the
formal parameter i, so here It is equivalent to assigning the value 10 of the formal parameter i to the local variable i
The second alert will of course output 10
Thinking: Combined with Case 2, this basically shows that the local variable i and the formal parameter i point to the same Store address!
, Case 4
/*Global (window) A piece of code under the domain*/
var i=10;
function a() {
alert(i);
var i = 2;
alert(i);
};
a();
Question: What will the above code output?
Answer: undefined, 2
Specific execution process
The first alert outputs undefined
The second alert outputs 2
Thinking: What’s going on?
Seeing the above examples, you may wonder how to implement them? What are the implementation details? How does the JS engine work?
Parsing process
, execution sequence
Compiled language, the compilation steps are divided into: lexical analysis, syntax analysis, semantic checking, code optimization and byte generation.
In interpreted languages, after obtaining the syntax analysis tree through lexical analysis and syntax analysis, interpretation and execution can begin. Here is a simple and original principle about the parsing process. It is only for reference. The detailed parsing process (various JS engines are different) requires further study.
The execution process of javascript. If a document stream contains multiple script code segments (js code separated by script tags or imported js files), their running order is:
Step 1. Read the first code segment (the js execution engine does not execute the program line by line, but It is analyzed and executed section by section)
Step 2. Do lexical analysis and syntax analysis. If there are errors, a syntax error will be reported (such as mismatched brackets, etc.) and jump to step 5.
Step 3. Correct [var 】Variables and [function] definitions are "pre-parsed" (errors will never be reported, because only correct declarations are parsed)
Step 4. Execute the code segment, and an error will be reported if there is an error (for example, the variable is undefined)
Steps 5. If there is another code segment, read the next code segment and repeat step 2
Step 6. End
, special instructions
All JS codes in the global domain (window) domain can be viewed It is an "anonymous method", which will be executed automatically, while other methods in this "anonymous method" will only be executed when they are explicitly called
, key steps
In the above process, we mainly It is divided into two stages
Parsing: it constructs a legal syntax analysis tree through syntax analysis and pre-parsing.
Execution: Execute a specific function. When the JS engine executes each function instance, it will create an execution environment (ExecutionContext) and active object (activeObject) (they belong to the host object and are consistent with the life cycle of the function instance) )
Here are more detailed example analysis materials: http://www.jb51.net/article/24547.htm

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
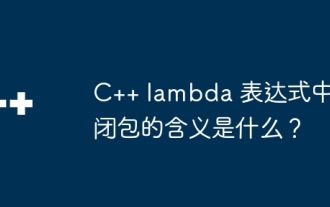
In C++, a closure is a lambda expression that can access external variables. To create a closure, capture the outer variable in the lambda expression. Closures provide advantages such as reusability, information hiding, and delayed evaluation. They are useful in real-world situations such as event handlers, where the closure can still access the outer variables even if they are destroyed.
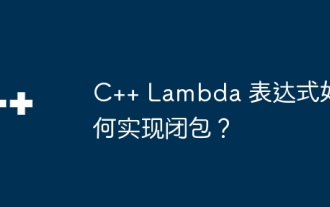
C++ Lambda expressions support closures, which save function scope variables and make them accessible to functions. The syntax is [capture-list](parameters)->return-type{function-body}. capture-list defines the variables to capture. You can use [=] to capture all local variables by value, [&] to capture all local variables by reference, or [variable1, variable2,...] to capture specific variables. Lambda expressions can only access captured variables but cannot modify the original value.
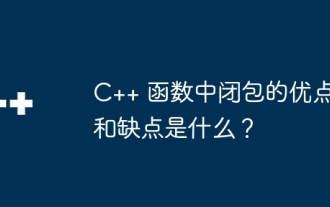
A closure is a nested function that can access variables in the scope of the outer function. Its advantages include data encapsulation, state retention, and flexibility. Disadvantages include memory consumption, performance impact, and debugging complexity. Additionally, closures can create anonymous functions and pass them to other functions as callbacks or arguments.
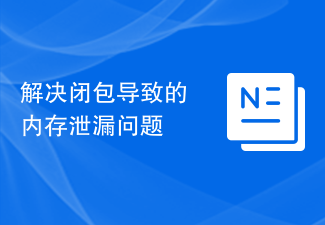
Title: Memory leaks caused by closures and solutions Introduction: Closures are a very common concept in JavaScript, which allow internal functions to access variables of external functions. However, closures can cause memory leaks if used incorrectly. This article will explore the memory leak problem caused by closures and provide solutions and specific code examples. 1. Memory leaks caused by closures The characteristic of closures is that internal functions can access variables of external functions, which means that variables referenced in closures will not be garbage collected. If used improperly,
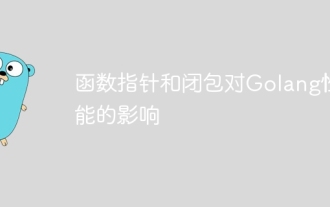
The impact of function pointers and closures on Go performance is as follows: Function pointers: Slightly slower than direct calls, but improves readability and reusability. Closures: Typically slower, but encapsulate data and behavior. Practical case: Function pointers can optimize sorting algorithms, and closures can create event handlers, but they will bring performance losses.
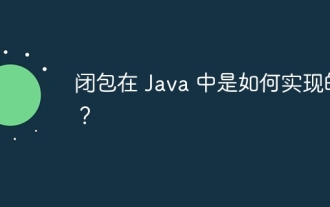
Closures in Java allow inner functions to access outer scope variables even if the outer function has exited. Implemented through anonymous inner classes, the inner class holds a reference to the outer class and keeps the outer variables active. Closures increase code flexibility, but you need to be aware of the risk of memory leaks because references to external variables by anonymous inner classes keep those variables alive.
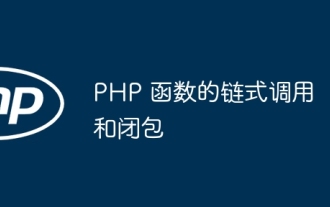
Yes, code simplicity and readability can be optimized through chained calls and closures: chained calls link function calls into a fluent interface. Closures create reusable blocks of code and access variables outside functions.
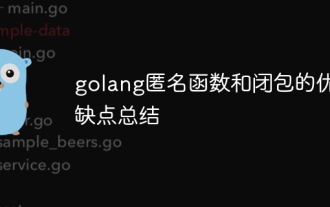
Anonymous functions are concise and anonymous, but have poor readability and are difficult to debug; closures can encapsulate data and manage state, but may cause memory consumption and circular references. Practical case: Anonymous functions can be used for simple numerical processing, and closures can implement state management.
